Creating an E-commerce Website with Django: A Step-by-Step Guide

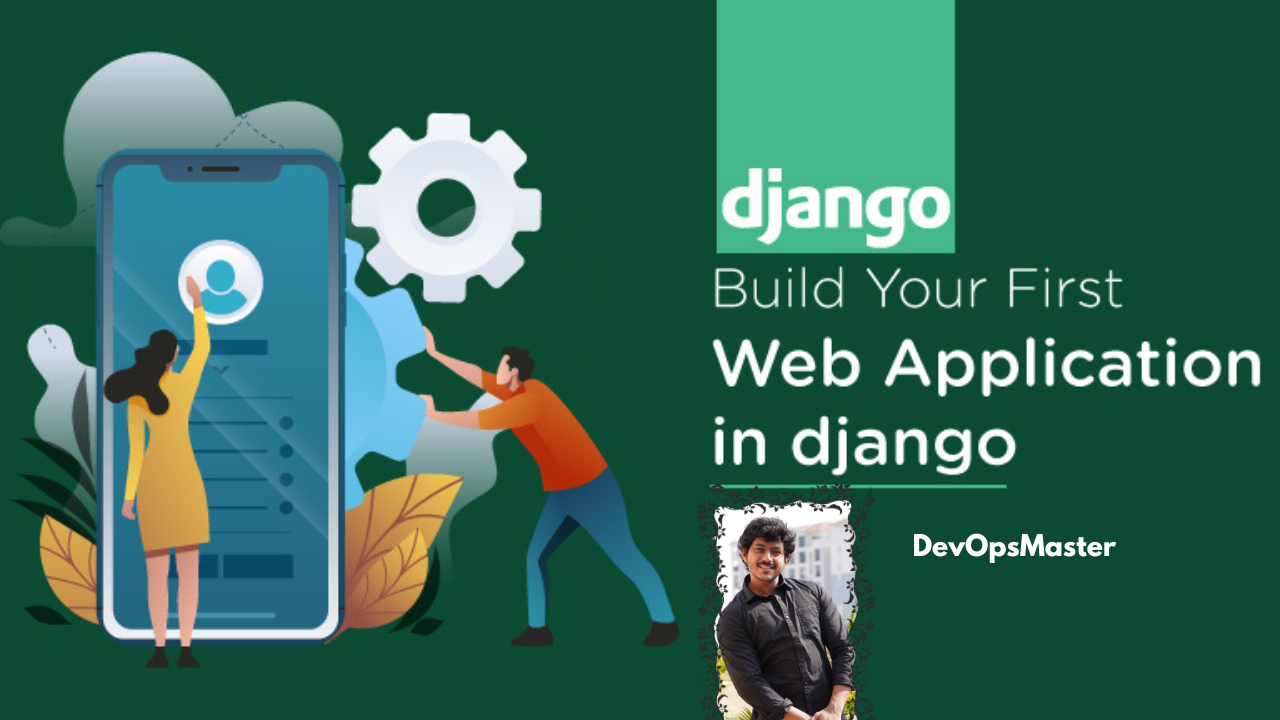
Django is a powerful and flexible web framework that simplifies the process of building web applications. In this guide, we will walk you through the process of creating a basic e-commerce website using Django, focusing on adding products with images. By the end of this tutorial, you'll have a functional e-commerce site with an admin interface for managing products.
Step 1: Setting Up Your Django Project
First, ensure you have Django installed. If not, you can install it using pip:
pip install django
Next, create a new Django project and navigate into the project directory:
django-admin startproject ecommerce
cd ecommerce
Step 2: Create a Products App
Within your project, create a new app called products
:
python manage.py startapp products
Add the new app to your INSTALLED_APPS
in settings.py
:
"""
Django settings for ecommerce project.
Generated by 'django-admin startproject' using Django 5.0.6.
For more information on this file, see
https://docs.djangoproject.com/en/5.0/topics/settings/
For the full list of settings and their values, see
https://docs.djangoproject.com/en/5.0/ref/settings/
"""
from pathlib import Path
# Build paths inside the project like this: BASE_DIR / 'subdir'.
BASE_DIR = Path(__file__).resolve().parent.parent
# Quick-start development settings - unsuitable for production
# See https://docs.djangoproject.com/en/5.0/howto/deployment/checklist/
# SECURITY WARNING: keep the secret key used in production secret!
SECRET_KEY = 'django-insecure-0_&92rew+2iwqw0!ry8wb=2&#n9xlfz#_j%lng@)m_14!=h5ln'
# SECURITY WARNING: don't run with debug turned on in production!
DEBUG = True
ALLOWED_HOSTS = []
# Application definition
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'products',
]
MIDDLEWARE = [
'django.middleware.security.SecurityMiddleware',
'django.contrib.sessions.middleware.SessionMiddleware',
'django.middleware.common.CommonMiddleware',
'django.middleware.csrf.CsrfViewMiddleware',
'django.contrib.auth.middleware.AuthenticationMiddleware',
'django.contrib.messages.middleware.MessageMiddleware',
'django.middleware.clickjacking.XFrameOptionsMiddleware',
]
ROOT_URLCONF = 'ecommerce.urls'
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [],
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
],
},
},
]
WSGI_APPLICATION = 'ecommerce.wsgi.application'
# Database
# https://docs.djangoproject.com/en/5.0/ref/settings/#databases
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': BASE_DIR / 'db.sqlite3',
}
}
# Password validation
# https://docs.djangoproject.com/en/5.0/ref/settings/#auth-password-validators
AUTH_PASSWORD_VALIDATORS = [
{
'NAME': 'django.contrib.auth.password_validation.UserAttributeSimilarityValidator',
},
{
'NAME': 'django.contrib.auth.password_validation.MinimumLengthValidator',
},
{
'NAME': 'django.contrib.auth.password_validation.CommonPasswordValidator',
},
{
'NAME': 'django.contrib.auth.password_validation.NumericPasswordValidator',
},
]
# Internationalization
# https://docs.djangoproject.com/en/5.0/topics/i18n/
LANGUAGE_CODE = 'en-us'
TIME_ZONE = 'UTC'
USE_I18N = True
USE_TZ = True
# Static files (CSS, JavaScript, Images)
# https://docs.djangoproject.com/en/5.0/howto/static-files/
STATIC_URL = '/static/'
MEDIA_URL = '/media/'
MEDIA_ROOT = BASE_DIR / 'media'
# Default primary key field type
# https://docs.djangoproject.com/en/5.0/ref/settings/#default-auto-field
DEFAULT_AUTO_FIELD = 'django.db.models.BigAutoField'
Step 3: Define the Product Model
In products/
models.py
, define a Product
model with fields for the product's name, price, description, and image:
from django.db import models
class Product(models.Model):
name = models.CharField(max_length=100)
price = models.DecimalField(max_digits=10, decimal_places=2)
description = models.TextField()
image = models.ImageField(upload_to='products/', null=True, blank=True)
def __str__(self):
return self.name
Step 4: Register the Product Model in the Admin Interface
To manage products via the Django admin, register the Product
model in products/
admin.py
:
from django.contrib import admin
from .models import Product
admin.site.register(Product)
Step 5: Configure Media Settings
To handle file uploads, configure media settings in settings.py
:
MEDIA_URL = '/media/'
MEDIA_ROOT = BASE_DIR / 'media'
Step 6: Update URL Configuration
Ensure your project’s urls.py
is configured to serve media files during development:
from django.contrib import admin
from django.urls import path
from django.conf import settings
from django.conf.urls.static import static
urlpatterns = [
path('admin/', admin.site.urls),
]
if settings.DEBUG:
urlpatterns += static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
Step 7: Run Migrations
Create the necessary database tables by running migrations:
python manage.py makemigrations
python manage.py migrate
Step 8: Add Products through the Admin Interface
Create a superuser to access the admin interface:
python manage.py createsuperuser
Run the development server:
python manage.py runserver
Log in to the admin interface at http://127.0.0.1:8000/admin/
and add a few products.
Step 9: Display Products on the Website
To display products on the front end, create a view and template. First, create a views.py
file in the products
app:
from django.shortcuts import render
from .models import Product
def product_list(request):
products = Product.objects.all()
return render(request, 'products/product_list.html', {'products': products})
Next, create a urls.py
file in the products
app:
from django.urls import path
from .views import product_list
urlpatterns = [
path('', product_list, name='product_list'),
]
Include the products
app's URLs in the project's urls.py
:
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('products/', include('products.urls')),
]
Create a templates/products/product_list.html
file to display the products:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Product List</title>
</head>
<body>
<h1>Product List</h1>
<ul>
{% for product in products %}
<li>
<h2>{{ product.name }}</h2>
<p>{{ product.description }}</p>
<p>${{ product.price }}</p>
{% if product.image %}
<img src="{{ product.image.url }}" alt="{{ product.name }}" width="200">
{% endif %}
</li>
{% endfor %}
</ul>
</body>
</html>
Conclusion
In this guide, we've walked through the steps to create a basic e-commerce site with Django, including adding and managing products with images. Django's powerful admin interface and straightforward configuration make it an excellent choice for building web applications quickly and efficiently. Expand on this foundation to add more features and create a fully-fledged e-commerce platform. Happy coding!
Feel free to leave any questions or feedback in the comments section below. If you enjoyed this tutorial, share it with your network!
Subscribe to my newsletter
Read articles from ANSAR SHAIK directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

ANSAR SHAIK
ANSAR SHAIK
AWS DevOps Engineer