JavaScript setTimeout() Function explained.
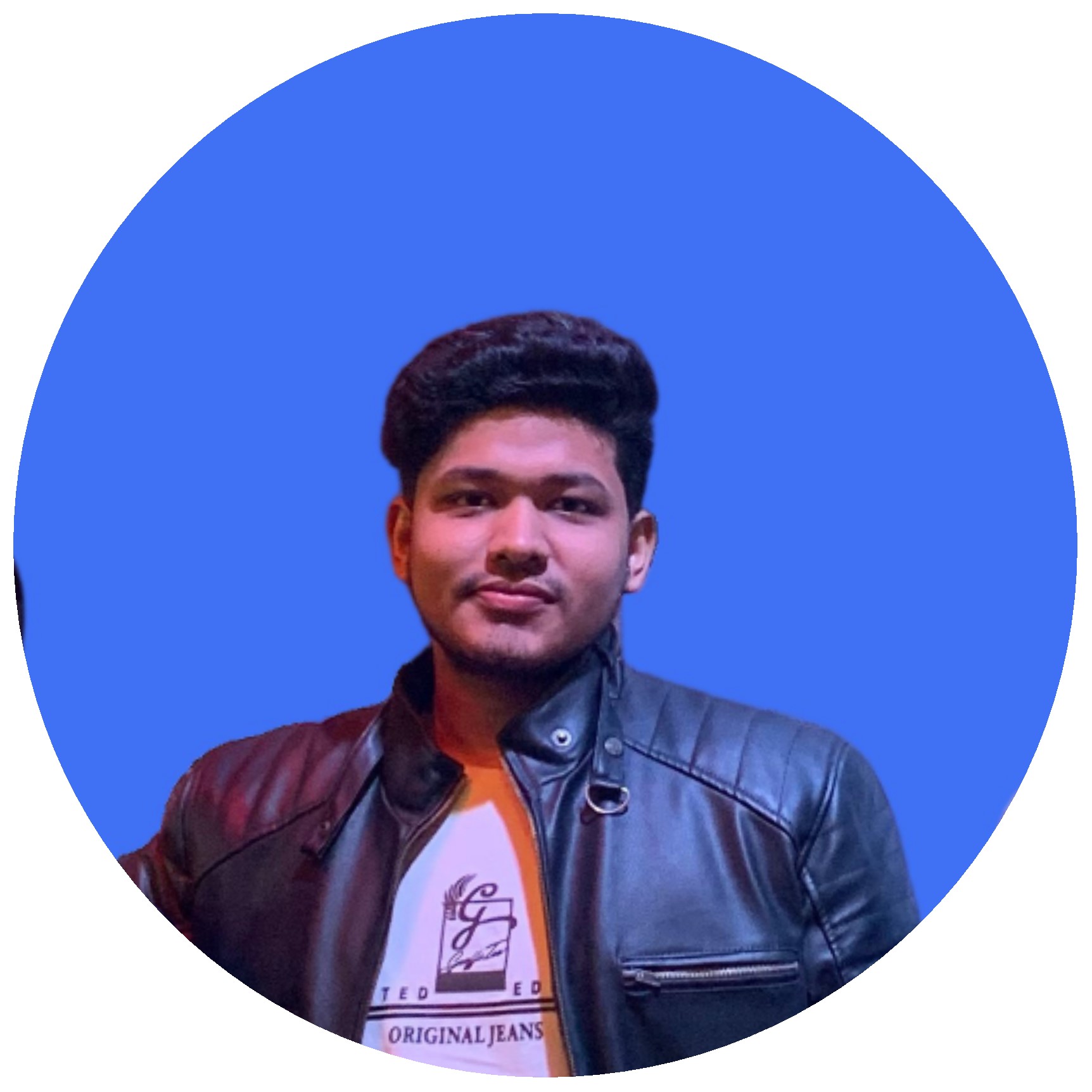
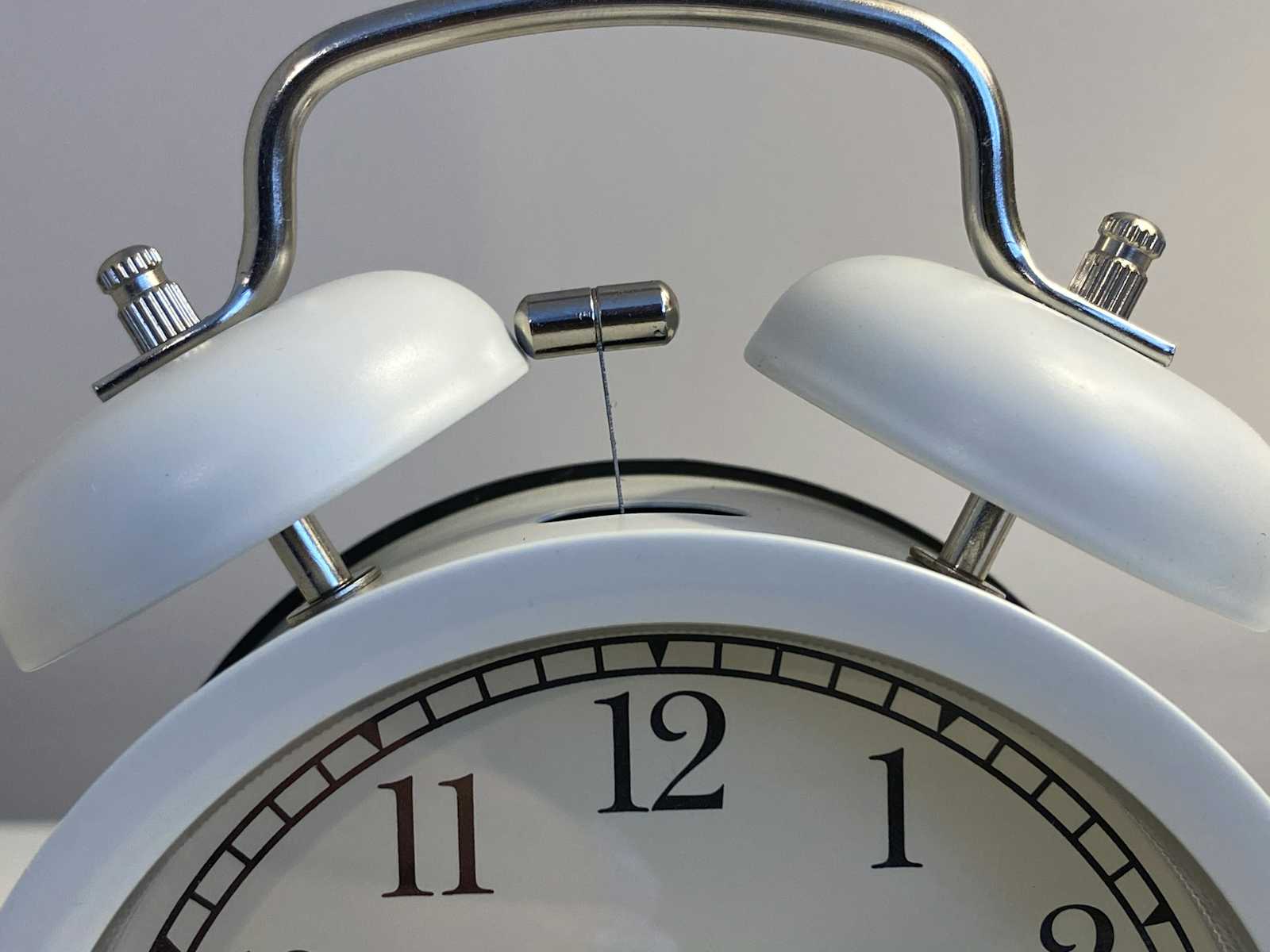
Prerequisites
Before direclty jumping into the setTimeout()
, it's good to have a basic understanding of Javascript and its fundamental concepts. Being familiar with variables
, basic control flow (such as if-else statements
and loops
) and functions
, will make it easier to understand the concepts discussed in this article.
If you're new to Javascript or need a refresher, there are multiple online resources available to learn. Websites like W3Schools
, freeCodeCamp
and yes how can we forget MDN Web Docs
, offer comprehensive tutorials and guides that can help you get started with Javascript.
Syntax and input parameters of setTimeout()
The basic syntax of setTimeout is as follows:
setTimeout(function, delay, param1, param2, ...);
Let's break down the different components:
The first parameter is the
function
or code snippet that you want to execute after the specified delay. It can be a named function or an anonymous arrow function.The second parameter
delay
represents the time interval inmilliseconds
before the code execution begins. It determines the wait duration before the specified function is invoked.Additional parameters, such as
param1
,param2
, and so on, are optional. You can use them to pass arguments to the function specified in the first parameter.
These setTimeout parameters allow you to customize the behavior of the function when it is executed.
Let's see few examples:
Example 1:
console.log("Start");
function greeting(name){
console.log("Have a good day "+ name + "!");
}
setTimeout(greeting, 2000, "Alice");
Output:
The output for above code will be:
Start
Have a good day Alice!
Explanation:
On console :
"Start" will get printed as the code gets executed.
After 2000 milli seconds or (2 seconds) "Have a good day Alice!" will be printed on console.
Example 2:
setTimeout(()=>{
console.log("Hello there!");
}, 0);
console.log("End");
Output:
The output for above code will be:
End
Hello there!
Explanation:
On console :
Even though a delay timer
of 0 milliseconds was passed in the setTimeout(), the JavaScript engine treats it as a waiting period of 0 milliseconds, and the function passed inside setTimeout will act as asynchronous code.
And console.log("End")
will act as synchronous code.
Since Javascript is a synchronous, single-threaded, object-oriented programming language.
Therefore, synchronous code will be executed first, and "End" will be printed on the console.
And after the 0 milli seconds timer has completed, "Hello there!" will get printed.
Working of setTimeout()
Consider the following program:
console.log("Start");
setTimeout( function callback(){
console.log("Callback function called.");
}, 5000);
console.log("End");
As we try to execute any Javascript prоgram an Execution Context is created and pushed inside the Call Stack.
The
console.log("Start");
of the program calls Web API of the browser [The super power of Browser] & the console Web API calls the console of brower to log some output.The console Web API is connected to the program via global
window object
which is executed inside the Global Execution Context.The JS Engine (interpreter) encounters setTimeout() of the program, this setTimeout will call the Web API which provides us the Time feature of the browser.
This set Timeout takes a
callback() function
and adelay timer
. This call back function is registered in the environment of Web API and a timer starts for5000 ms.
The JS Engine moves to next line and finds
console.log()
which will print "End" on the console.After "End" gets printed on the console, the Global Execution Context is poped out of the Call Stack.
After the
5000 ms
timer completes, the reference of async callback function is then pushed into Callback queue.Here comes Event Loop into the picture. Event loop is a single threaded loop which is always ON and running.
Event Loop:
The job of Event loop is to continiously observe the
Call Stack
,Callback Queue
andMicro-task Queue
and when the Call Stack is empty, the Event Loop pushes the reference of tasks present in Micro-task Queue & Callback Queue into the Call Stack.The Event Loop checks the Call Stack, Micro-task Queue and Callback queue.
If the Call stack is empty and any function is left to be executed in Microtask queue or Callback queue then, that function is pushed into the Call stack.
Hence the Call stack executes the callback function by creating a new Execution context and code will be executed line by line.
Output:
Start
End
Callback function called.
That's it for now. For those of you who didn't know, hope you learned something. And if you're already an expert, I would appreciate a critique - let me know if my understanding is correct.
Take care and Happy coding.
Subscribe to my newsletter
Read articles from Ayush Rastogi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
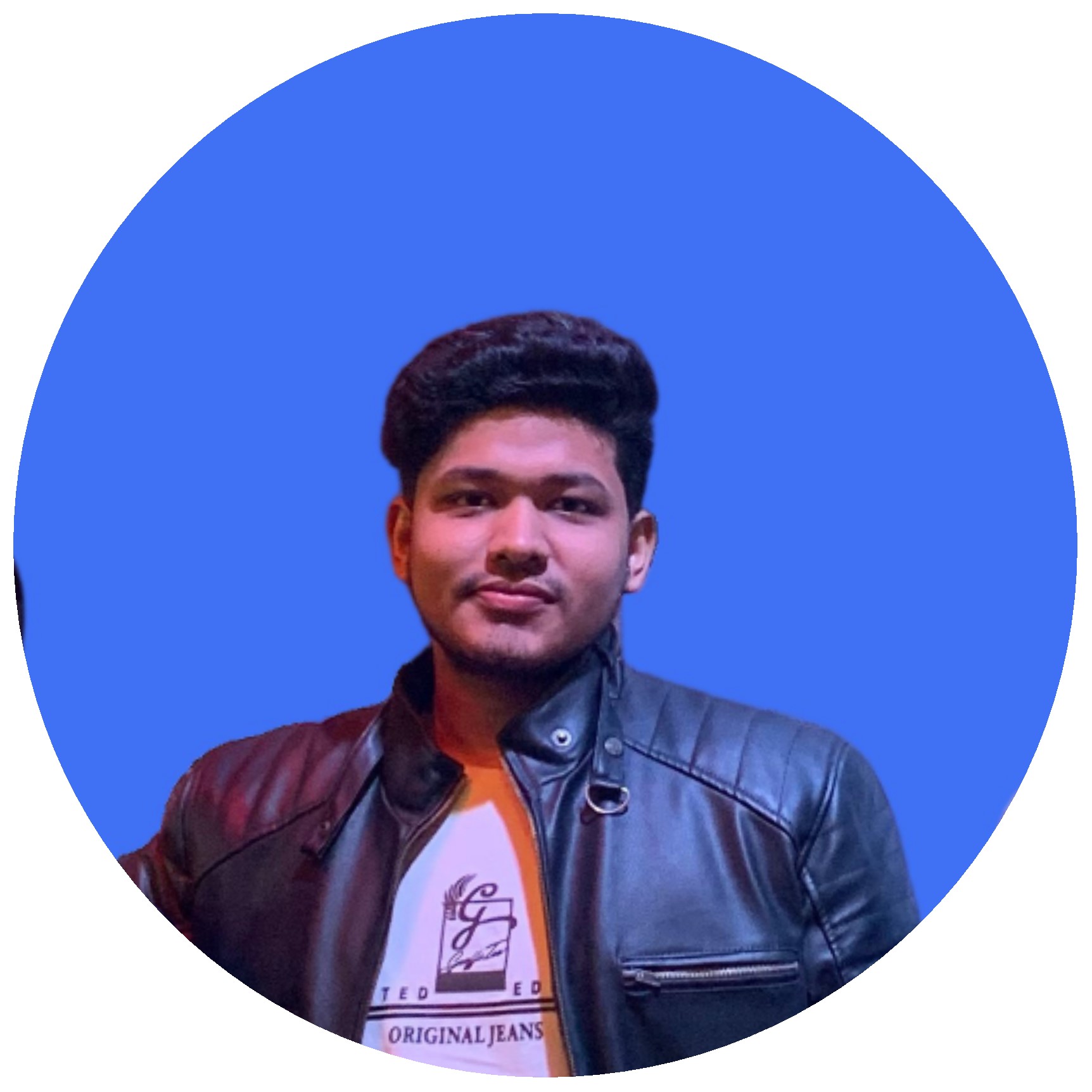