Day 9 of #100DaysOfCode - Secret Auction Program
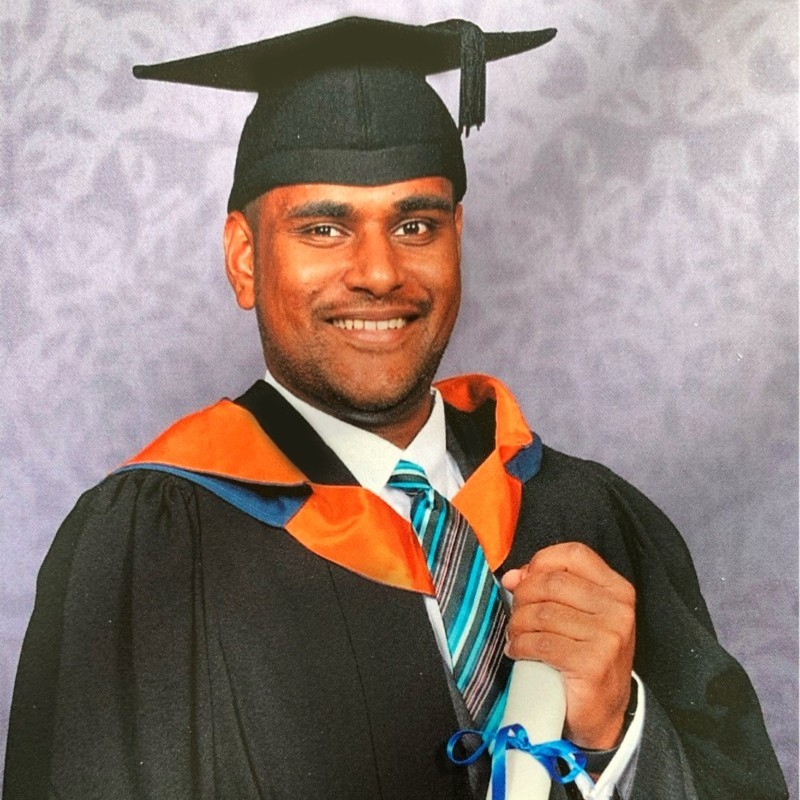
On Day 9 of #100DaysOfCode, this mini project I have created a secret auction program using the dictionaries and nested lists, skills which I have learned from Dr Angela Yu's 100DaysOfCode: The Complete Python Bootcamp Udemy course.
Secret auction program works by asking the people to enter their name, the price and requests whether there are other bidders that want to bid. If the response is yes, it clears the screen and repeats the process for the new bidders. Once all the information is collected, the program announces the highest bidder as the winner.
Python Concepts Used:
Dictionary
In the secret auction program, I used a dictionary called user_bidders = {}
to manage the bids. Each time a person enter their name and bid amount, it gets stored into the user_bidders
dictionary with the person's name as the key and bid amount as the value.
Here's how the bids are stored:
user_bidders[name] = bid_price
After retrieving all the bids, a for loop iterates through all the entries in the user_bidders dictionary. For each iteration, it checks if the current bid amount is higher than the highest bid recorded so far. If it is, the program updates the highest bid and the corresponding bidder's name. By the end of the loop the highest_bid
variable contains the highest bid and the bid_winner
variable contains the name of the person with the highest bid.
Here's the relevant code:
for user_bid in bid_database:
bid_amount = bid_database[user_bid]
if bid_amount > highest_bid:
highest_bid = bid_amount
bid_winner = user_bid
Reflections on the Secret Auction Program
During this mini project, I had learned how to effectively use dictionaries in Python to store and collect user inputs. By storing each bid with the bidders name as key and bid price as the value, I was able to simply iterate through all the entries to determine the highest bid. This project helped me hugely improve my understanding of dictionaries and their practical applications in handling and processing data.
Access to full code - https://github.com/keiransystem14/Python100daysofcode/tree/main/Day%209%20Project%20-%20Secret%20Auction%20Program
Subscribe to my newsletter
Read articles from Keiran Krishnavenan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
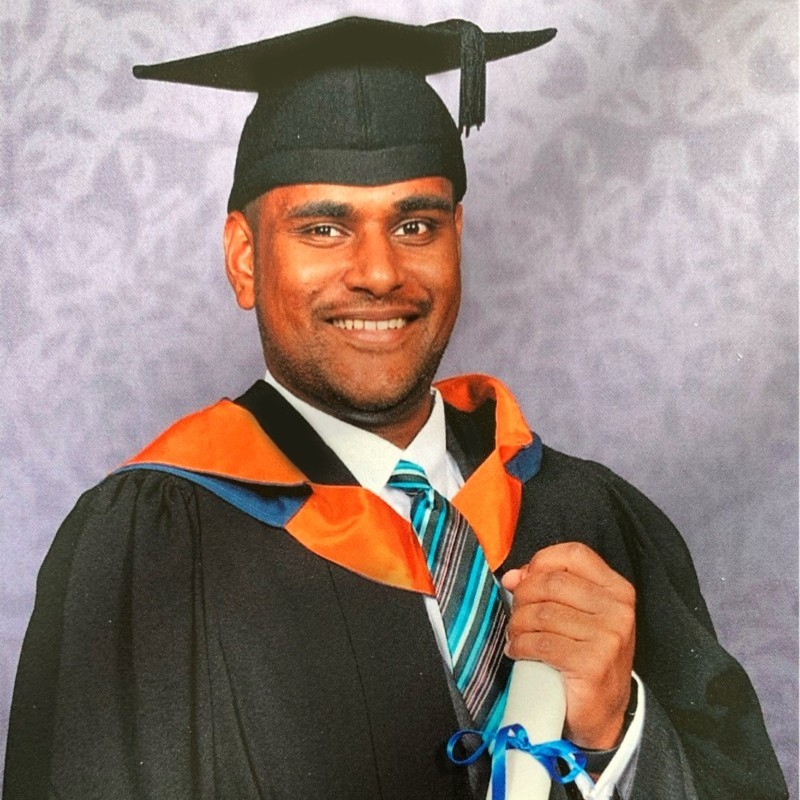