CSRF Protection in Laravel: Shielding Your App from Unintended Actions
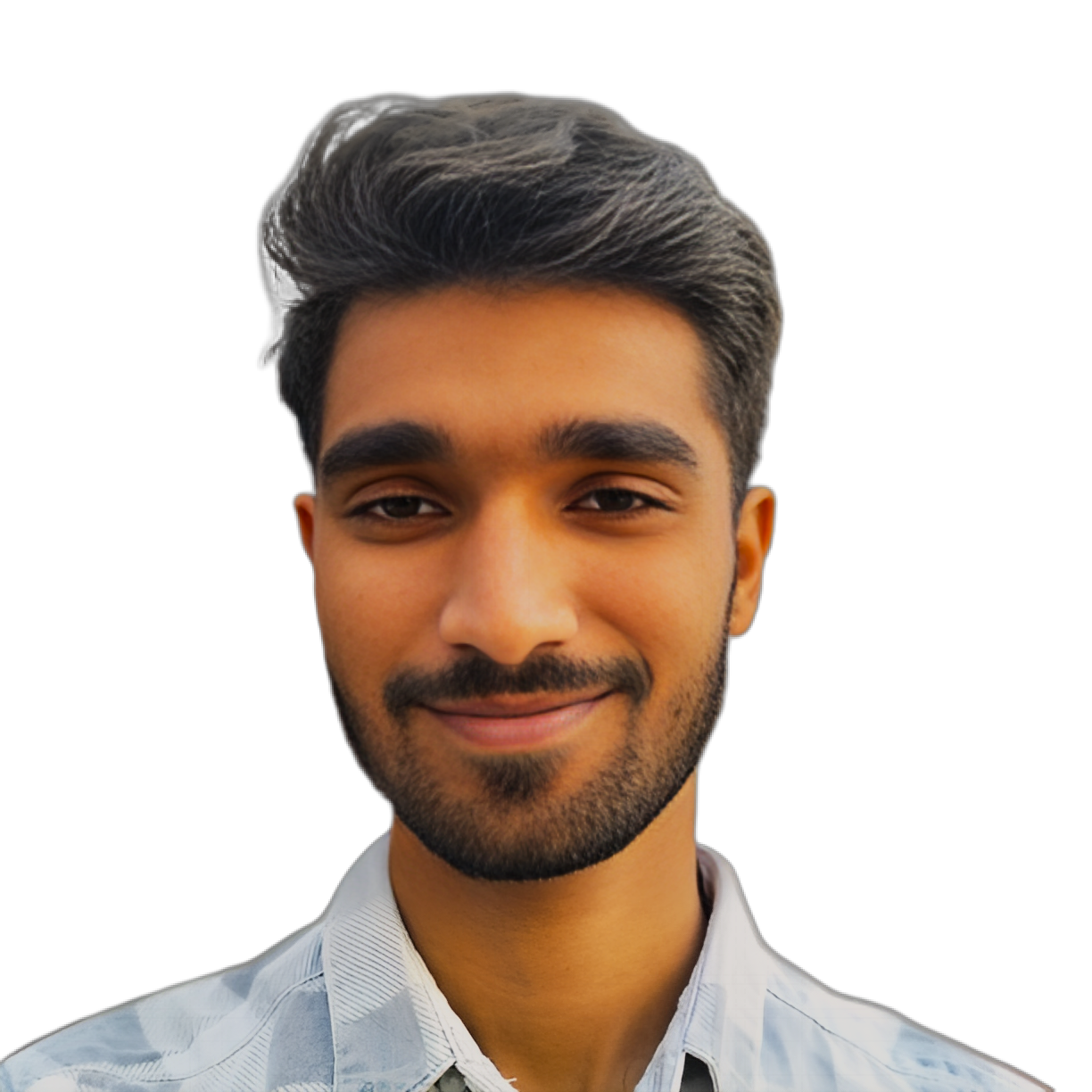
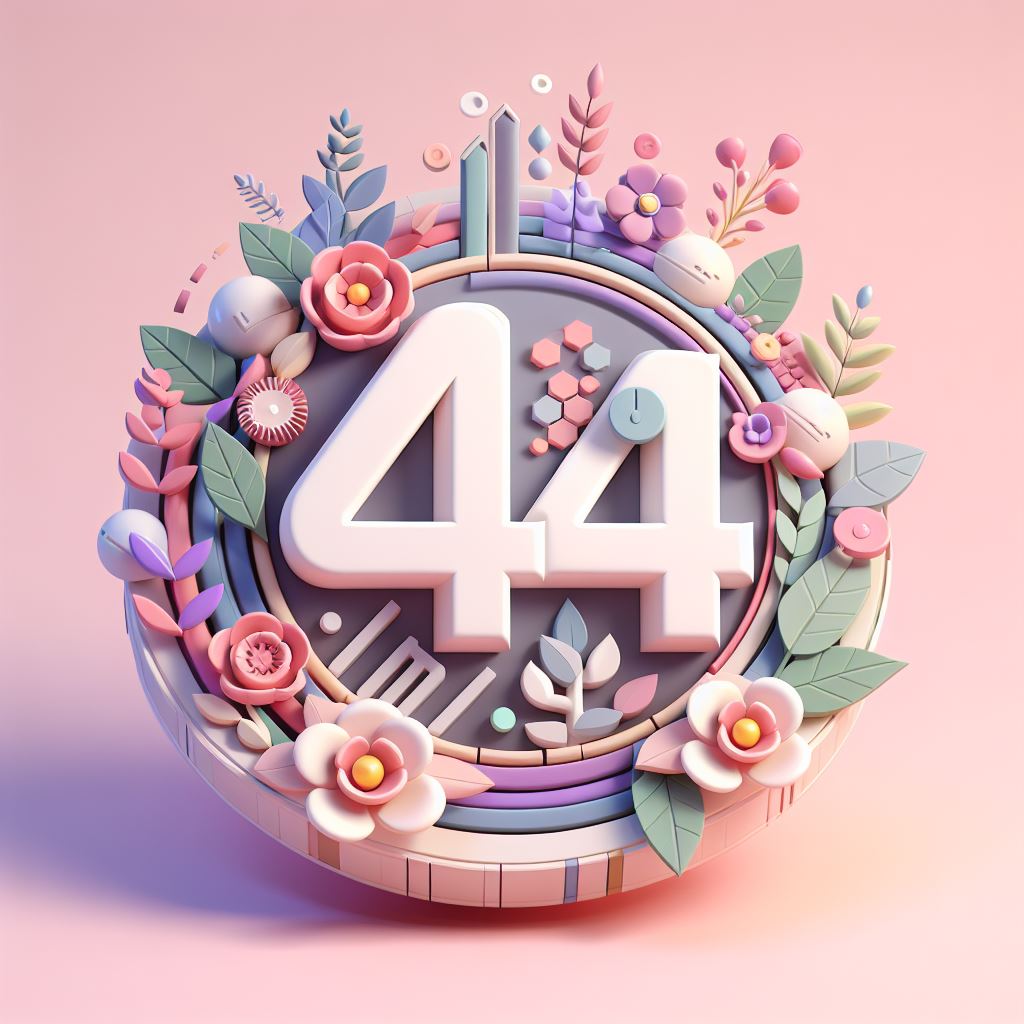
Introduction
Have you ever encountered a scenario where someone else's account settings mysteriously changed, or unauthorized actions were performed on a website you were logged in to? This could be a sign of a Cross-Site Request Forgery (CSRF) attack. In this blog post, we'll delve into CSRF attacks, their implications, and how Laravel's built-in CSRF protection safeguards your web applications. We'll also provide a step-by-step tutorial to ensure your forms are CSRF-protected.
Understanding CSRF Attacks
Imagine a malicious website that tricks your browser into unknowingly sending unauthorized requests to another website where you're logged in. This is the essence of a CSRF attack. Hackers exploit the trust your browser has for legitimate websites to manipulate it into performing actions on your behalf without your knowledge.
Here's a breakdown of how a CSRF attack might unfold:
An Attacker's Webpage: The attacker creates a webpage containing a seemingly harmless image or link.
Embedded Request: This image or link embeds a specially crafted request that targets a specific action on another website (e.g., updating your profile, transferring money).
Unsuspecting User: You, the unsuspecting user, visit the attacker's webpage.
Unwitting Submission: Your browser, unaware of the malicious intent, sends the embedded request to the targeted website along with your cookies (which contain your authentication credentials).
Unauthorized Action: The targeted website receives the request and, assuming it's coming from your trusted browser, processes it. This can lead to unauthorized actions on your account.
The Importance of CSRF Protection
CSRF attacks pose a significant threat to the security of web applications. Without proper protection, attackers can potentially:
Steal sensitive data from your accounts
Change your account settings or preferences
Perform unauthorized actions (e.g., transferring funds, making purchases)
Disrupt normal application functionality by flooding it with illegitimate requests
Laravel's CSRF "Shield": How It Works
Thankfully, Laravel provides a robust built-in mechanism to guard against CSRF attacks. Here's an overview of how it functions:
CSRF Token Generation: When a user initiates a session in your Laravel application, a unique CSRF token is generated. This token is a random string that serves as an additional security layer.
Hidden Form Field: Laravel's Blade templating engine includes the
@csrf
directive within your application's forms. This directive automatically generates a hidden form field named_token
and embeds the CSRF token within it.CSRF Token Validation: When a user submits a form, Laravel automatically retrieves the CSRF token from the
_token
hidden field and validates it against the one stored in the user's session.Protection Against Mismatches: If the tokens don't match, Laravel throws an exception, preventing the intended form action from being executed. This effectively prevents CSRF attacks.
Tutorial: Securing Your Laravel Forms with CSRF Protection
Now, let's walk through a step-by-step tutorial on how to ensure your Laravel forms are CSRF-protected:
1. Include the @csrf
Directive:
In all your Laravel forms, make sure to include the @csrf
directive within the opening form tag. This will automatically generate the hidden _token
field with the CSRF token:
HTML
<form method="POST" action="{{ route('update-profile') }}">
@csrf
<button type="submit">Update Profile</button>
</form>
Beyond the Basics: Additional Considerations
While the @csrf
directive offers excellent protection for most scenarios, there are a few additional points to consider:
1. Excluding Specific Routes:
In rare cases, you might need to disable CSRF protection for certain routes, such as API endpoints that receive data from external applications. To achieve this, you can modify the VerifyCsrfToken
middleware in app/Http/Middleware/VerifyCsrfToken.php
.
2. Handling AJAX Requests:
For AJAX requests, you'll need to include the CSRF token in the request headers.
3. Customizing the CSRF Token Field Name:
By default, Laravel uses the _token
field name. If you need to change this for specific forms, you can modify the $except
property in the VerifyCsrfToken
middleware.
4. Session Management:
Remember that CSRF protection relies on session cookies. Ensure your Laravel application is configured with appropriate session settings.
Conclusion
Laravel's built-in CSRF protection is a powerful tool for safeguarding your web applications against unauthorized actions. By understanding how it works and implementing the provided techniques, you can effectively secure your
Subscribe to my newsletter
Read articles from Asis Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
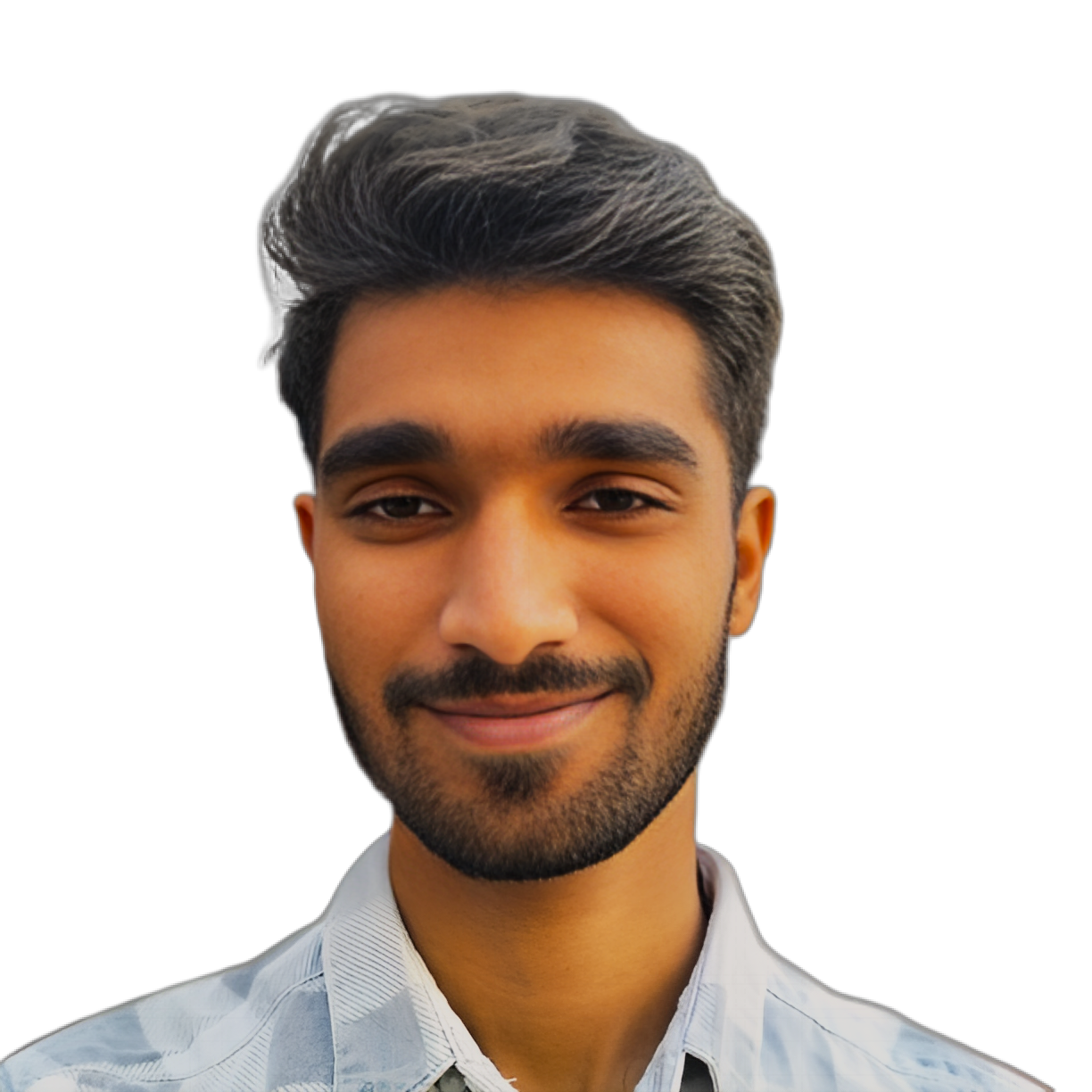
Asis Sharma
Asis Sharma
I'm an ambitious and motivated software developer, eager to apply my knowledge and skills to contribute to the development of innovative and cutting-edge software solutions. I'm well-versed in a variety of programming languages, including Javascript, C++, and Node.js, and have a understanding of object-oriented programming and algorithms. I'm passionate about developing and I am eager to learn and collaborate with others.