Is Your Data Lying to You? Uncover the Zod of Validation
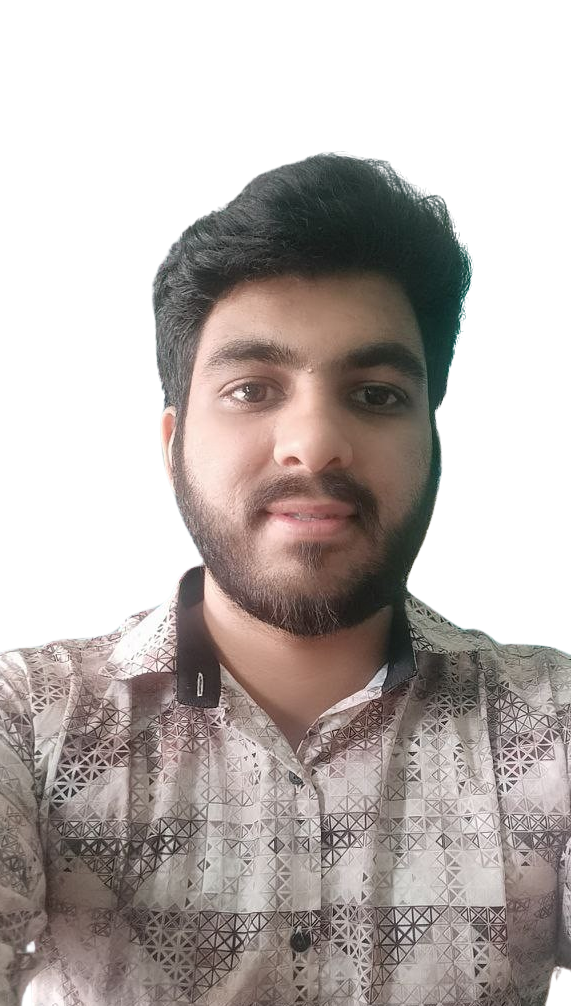
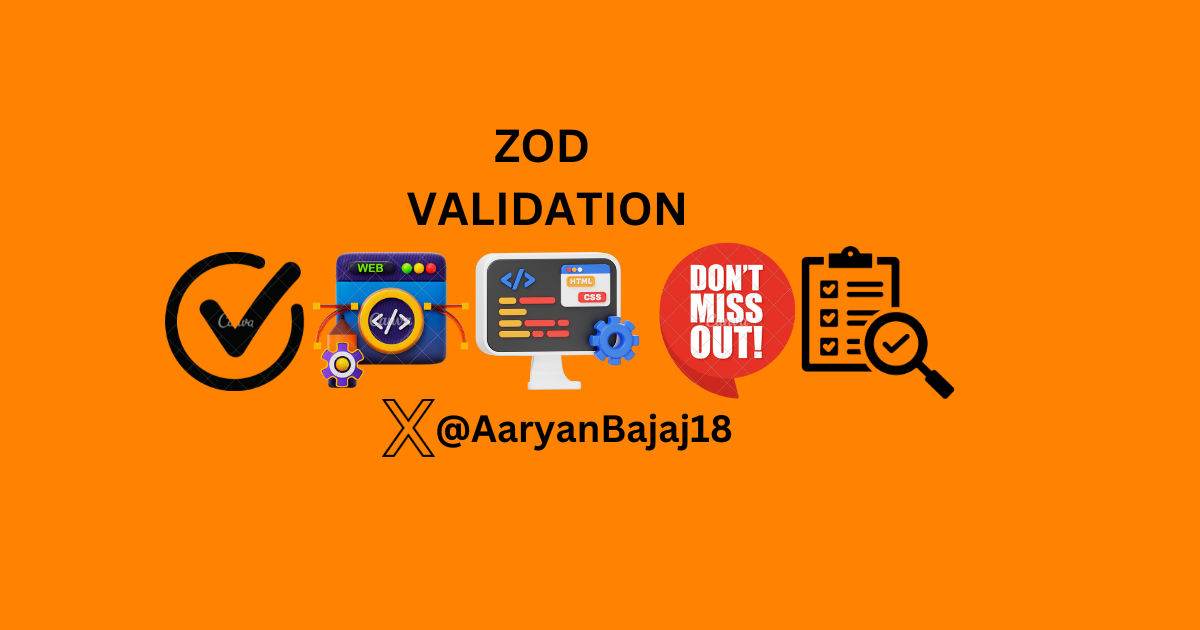
Introduction
Zod is a TypeScript-first library designed to ensure your data adheres to specific schemas. This means that whether you're working on the front end or the back end, Zod can help maintain data integrity and reduce bugs.
Why Use Zod?
TypeScript Integration: Zod works seamlessly with TypeScript, allowing you to define and infer types directly from schemas.
Declarative Syntax: The syntax is straightforward, making it easy to declare validation rules.
Comprehensive Validation: Zod handles various data structures, from simple types to complex nested objects.
Installation Of Zod
npm install zod
# or
yarn add zod
Real Life Example: User Registration Form
Let's consider a common scenario: validating a user registration form. This form collects a user's name, email, password, and address. Here's how you can use Zod to ensure that the data entered by users meets your requirements.
Defining Schema
import { z } from 'zod';
const addressSchema = z.object({
street: z.string(),
city: z.string(),
zipCode: z.string().regex(/^\d{5}(-\d{4})?$/),
});
const userSchema = z.object({
name: z.string().min(1, "Name is required"),
email: z.string().email("Invalid email address"),
password: z.string().min(8, "Password must be at least 8 characters long"),
address: addressSchema,
});
In this schema:
name
is required and must be a string.email
must be a valid email address.password
must be at least 8 characters long.address
is a nested object with its own validation rules.
Validating User Input
const userInput = {
name: "John Doe",
email: "john.doe@example.com",
password: "securePass123",
address: {
street: "123 Main St",
city: "Springfield",
zipCode: "12345",
},
};
const result = userSchema.safeParse(userInput);
if (result.success) {
console.log("Validation passed:", result.data);
} else {
console.log("Validation failed:", result.error.errors);
}
Real-Life Example: API Request Validation
Another practical use case for Zod is validating API requests. Suppose we have an API endpoint that accepts order details. Here's how you can use Zod to validate the request body.
const orderSchema = z.object({
orderId: z.string().uuid("Invalid order ID"),
items: z.array(
z.object({
productId: z.string().uuid("Invalid product ID"),
quantity: z.number().min(1, "Quantity must be at least 1"),
})
),
totalAmount: z.number().min(0, "Total amount must be non-negative"),
userId: z.string().uuid("Invalid user ID"),
});
In this schema:
orderId
,productId
, anduserId
must be valid UUIDs.items
is an array of objects, each containing aproductId
andquantity
.totalAmount
must be a non-negative number.
Validating API Requests
const orderInput = {
orderId: "550e8400-e29b-41d4-a716-446655440000",
items: [
{ productId: "550e8400-e29b-41d4-a716-446655440001", quantity: 2 },
{ productId: "550e8400-e29b-41d4-a716-446655440002", quantity: 1 },
],
totalAmount: 59.99,
userId: "550e8400-e29b-41d4-a716-446655440003",
};
const result = orderSchema.safeParse(orderInput);
if (result.success) {
console.log("Validation passed:", result.data);
} else {
console.log("Validation failed:", result.error.errors);
}
Conclusion
Zod is an invaluable tool for full-stack web development, providing robust data validation capabilities. Whether you're validating user input on the front end or ensuring the integrity of API requests on the back end, Zod makes the process straightforward and reliable. By integrating Zod into your projects, you can enhance data integrity, reduce bugs, and improve overall application security.
Try incorporating Zod into your next project and experience the benefits of seamless data validation.
Thank You Everyone For The Read ....
Happy Coding! ๐ค๐ป
๐ Hello, I'm Aaryan Bajaj .
๐ฅฐ If you liked this article, consider sharing it.
Subscribe to my newsletter
Read articles from AARYAN BAJAJ directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
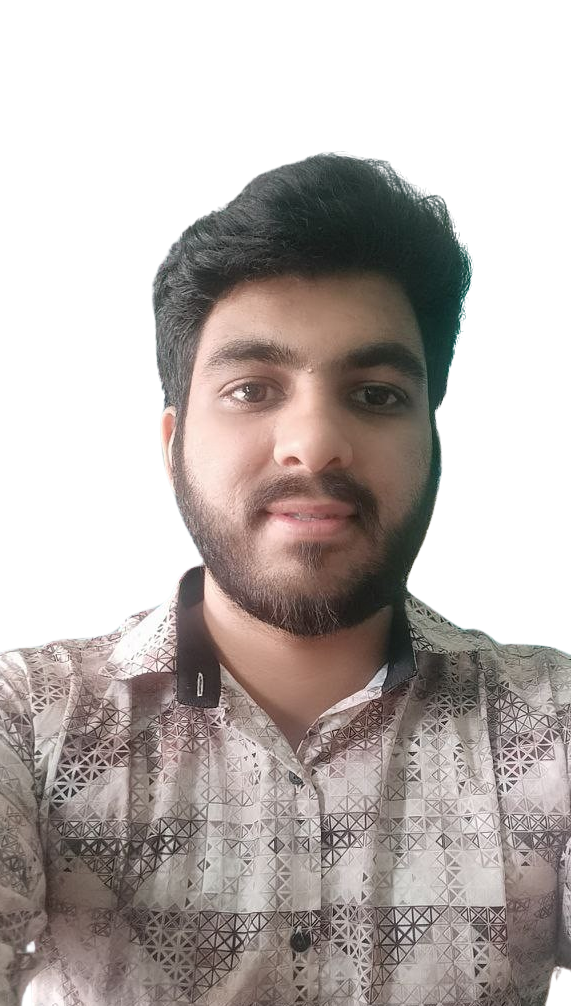
AARYAN BAJAJ
AARYAN BAJAJ
Hi Everyone ๐๐ป , I'm Aaryan Bajaj , a Full Stack Web Developer from India(๐ฎ๐ณ). I share my knowledge through engaging technical blogs on Hashnode, covering web development.