Most Common Word
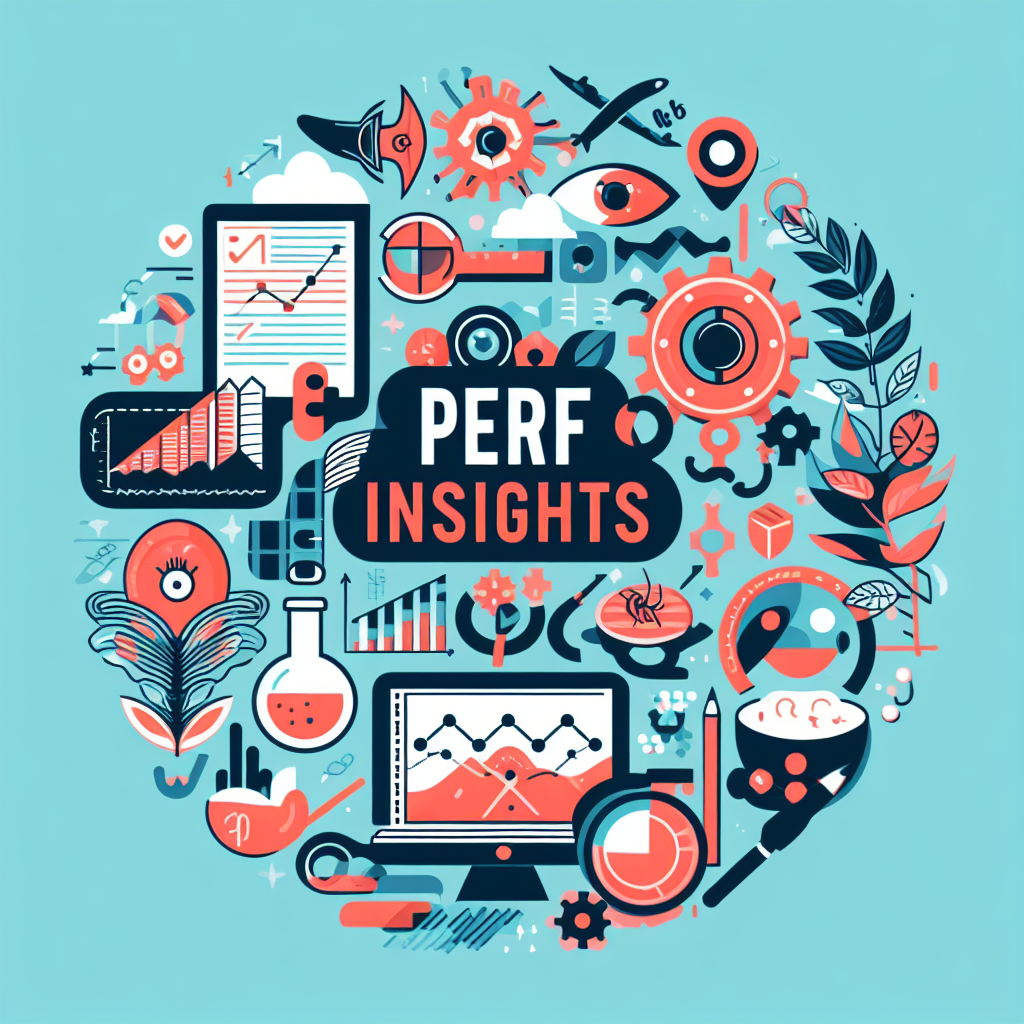
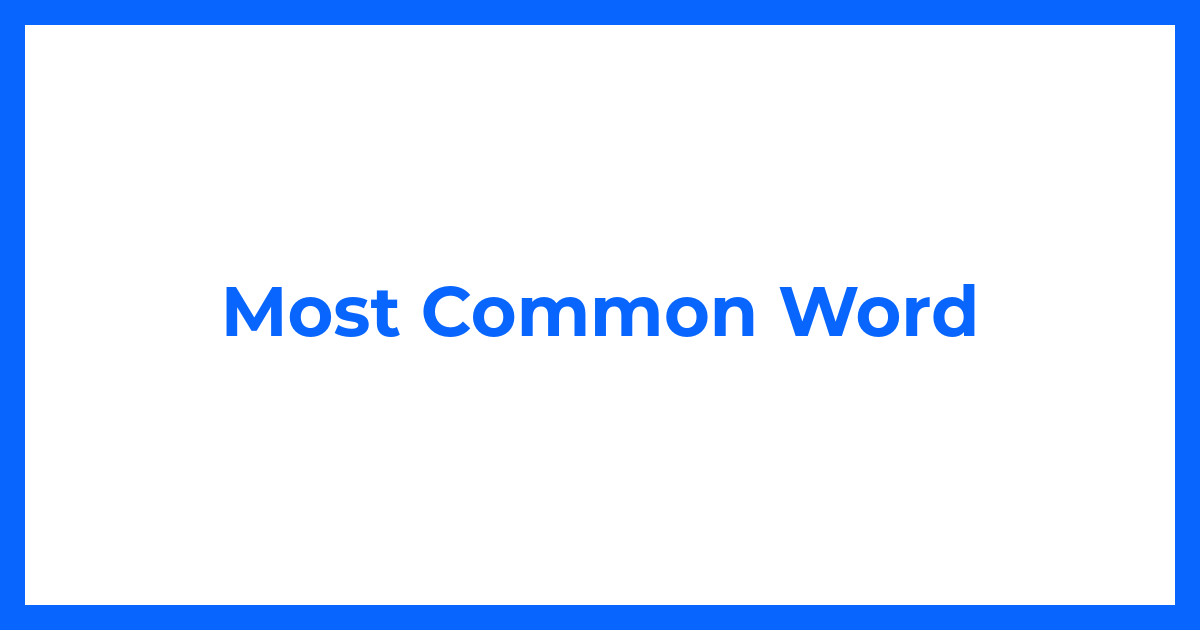
Given a string paragraph
and a string array of the banned words banned
, return the most frequent word that is not banned. It is guaranteed there is at least one word that is not banned, and that the answer is unique.
The words in paragraph
are case-insensitive and the answer should be returned in lowercase.
LeetCode Problem - 819
class Solution {
public String mostCommonWord(String paragraph, String[] banned) {
// Replace all non-letter characters with spaces and trim the result to clean up the paragraph
String filteredParagraph = paragraph.replaceAll("[^a-zA-Z]", " ").replaceAll("\\s+", " ").trim();
// Create a map to count the occurrences of each word
Map<String, Integer> mp = new HashMap<>();
// Split the cleaned paragraph into words
String[] paragraphArray = filteredParagraph.split(" ");
// Convert the banned array to a string for easier checking
String bannedString = Arrays.toString(banned);
// Iterate over each word in the paragraph
for(int i = 0; i < paragraphArray.length; i++) {
// Convert the word to lowercase
String key = paragraphArray[i].toLowerCase();
// If the word is not in the banned list, update its count in the map
if(!bannedString.contains(key)) {
mp.put(key, mp.getOrDefault(key, 0) + 1);
}
}
// Initialize variables to track the most common word and its count
String answer = "";
int count = 0;
// Iterate over the keys in the map
for(String str : mp.keySet()) {
// Get the count of the current word
int temp = mp.get(str);
// Update the most common word and its count if the current word's count is higher
if(temp > count) {
count = temp;
answer = str;
}
}
// Return the most common word
return answer;
}
}
Subscribe to my newsletter
Read articles from Gulshan Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
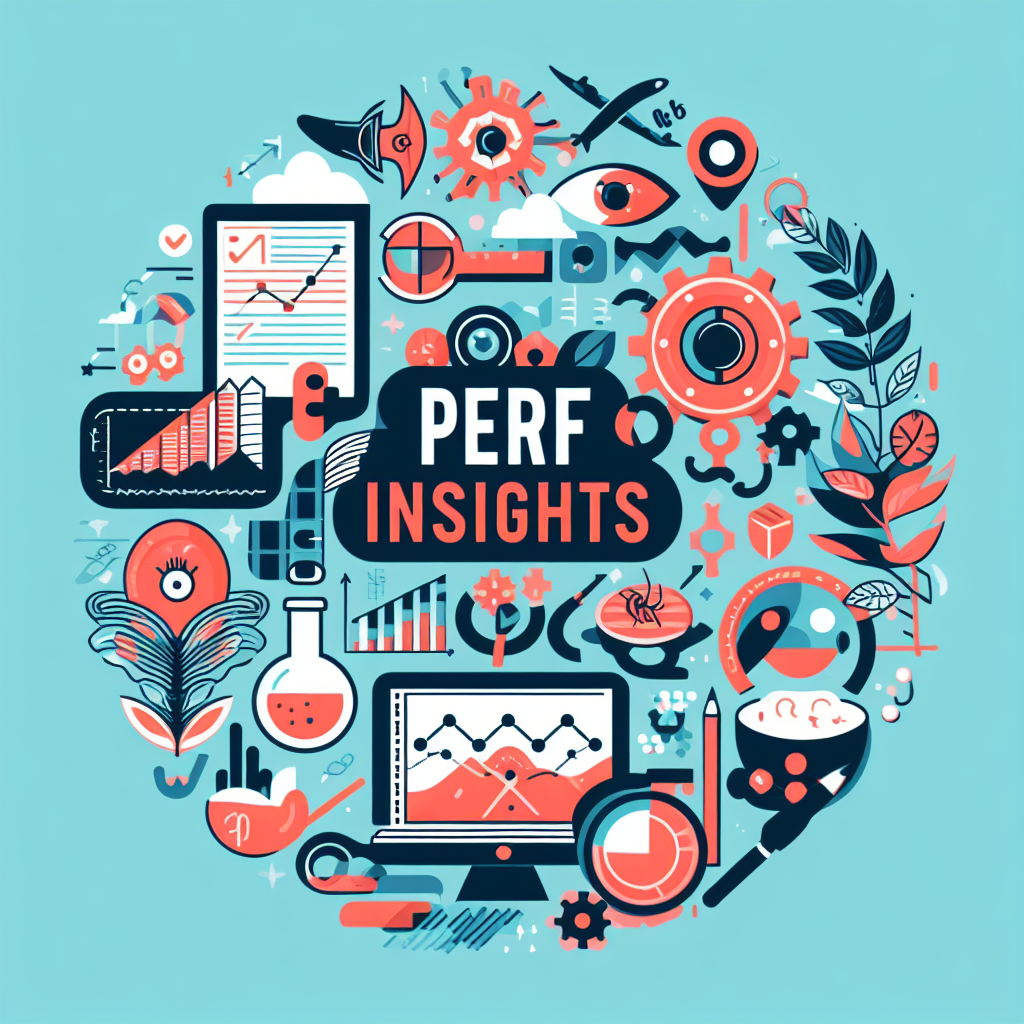
Gulshan Kumar
Gulshan Kumar
As a Systems Engineer at Tata Consultancy Services, I deliver exceptional software products for mobile and web platforms, using agile methodologies and robust quality maintenance. I am experienced in performance testing, automation testing, API testing, and manual testing, with various tools and technologies such as Jmeter, Azure LoadTest, Selenium, Java, OOPS, Maven, TestNG, and Postman. I have successfully developed and executed detailed test plans, test cases, and scripts for Android and web applications, ensuring high-quality standards and user satisfaction. I have also demonstrated my proficiency in manual REST API testing with Postman, as well as in end-to-end performance and automation testing using Jmeter and selenium with Java, TestNG and Maven. Additionally, I have utilized Azure DevOps for bug tracking and issue management.