Palindrome Partitioning
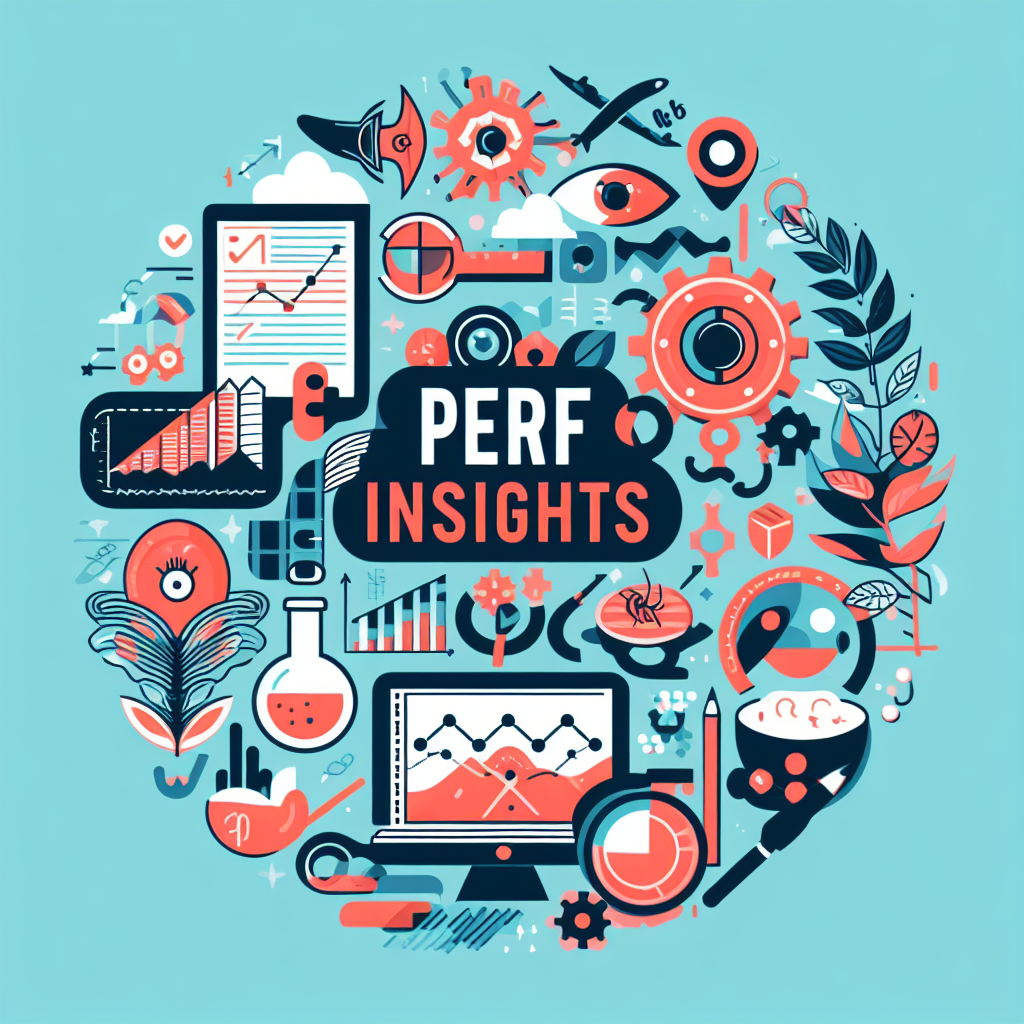
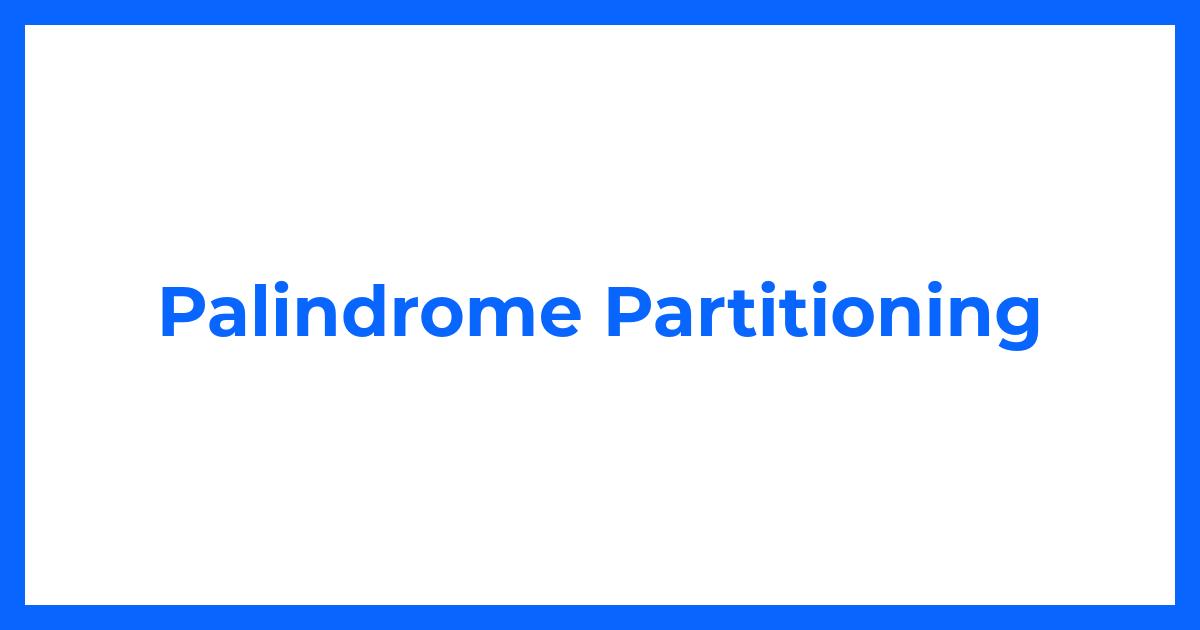
Given a string s
, partition s
such that every substringof the partition is a palindrome. Return all possible palindrome partitioning of s
.
LeetCode Problem - 131
class Solution {
// Method to partition the input string into all possible palindromic substrings
public List<List<String>> partition(String s) {
// List to store the result of all possible palindromic partitions
List<List<String>> result = new ArrayList<>();
// List to store the current partition being constructed
List<String> currentPartition = new ArrayList<>();
// Start the backtracking process from the first character
backtrack(s, 0, currentPartition, result);
// Return the final result list
return result;
}
// Helper method to perform backtracking
private void backtrack(String s, int start, List<String> currentPartition, List<List<String>> result) {
// If we've reached the end of the string, add the current partition to the result list
if (start == s.length()) {
result.add(new ArrayList<>(currentPartition));
return;
}
// Iterate over possible end indices for substrings starting from 'start'
for (int end = start; end < s.length(); end++) {
// If the substring s[start:end+1] is a palindrome, proceed with the current partition
if (isPalindrome(s, start, end)) {
// Add the palindromic substring to the current partition
currentPartition.add(s.substring(start, end + 1));
// Recur to continue partitioning the remaining substring
backtrack(s, end + 1, currentPartition, result);
// Backtrack by removing the last added substring
currentPartition.remove(currentPartition.size() - 1);
}
}
}
// Helper method to check if a substring s[left:right+1] is a palindrome
private boolean isPalindrome(String s, int left, int right) {
// Compare characters from the ends towards the center
while (left < right) {
// If characters don't match, it's not a palindrome
if (s.charAt(left) != s.charAt(right)) {
return false;
}
left++;
right--;
}
// If all characters match, it's a palindrome
return true;
}
}
Subscribe to my newsletter
Read articles from Gulshan Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
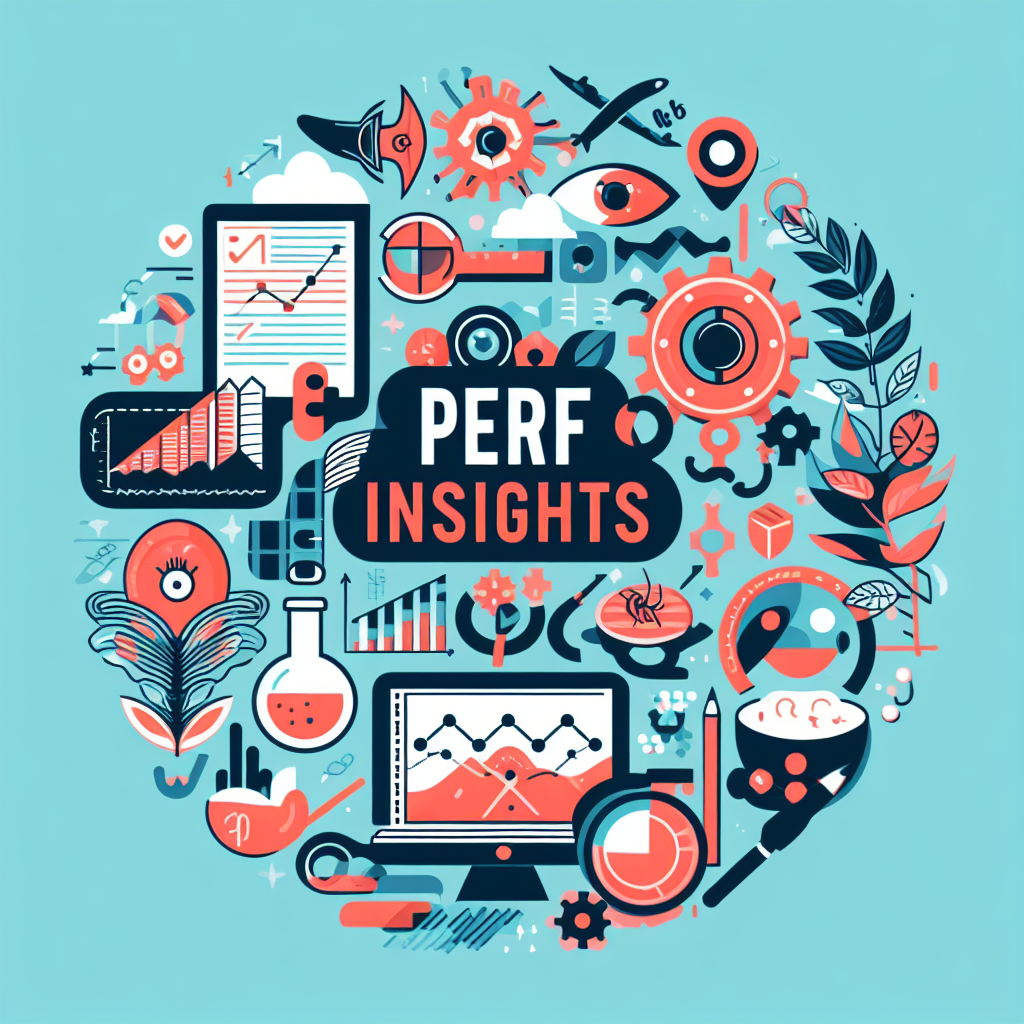
Gulshan Kumar
Gulshan Kumar
As a Systems Engineer at Tata Consultancy Services, I deliver exceptional software products for mobile and web platforms, using agile methodologies and robust quality maintenance. I am experienced in performance testing, automation testing, API testing, and manual testing, with various tools and technologies such as Jmeter, Azure LoadTest, Selenium, Java, OOPS, Maven, TestNG, and Postman. I have successfully developed and executed detailed test plans, test cases, and scripts for Android and web applications, ensuring high-quality standards and user satisfaction. I have also demonstrated my proficiency in manual REST API testing with Postman, as well as in end-to-end performance and automation testing using Jmeter and selenium with Java, TestNG and Maven. Additionally, I have utilized Azure DevOps for bug tracking and issue management.