Increasing Decreasing String
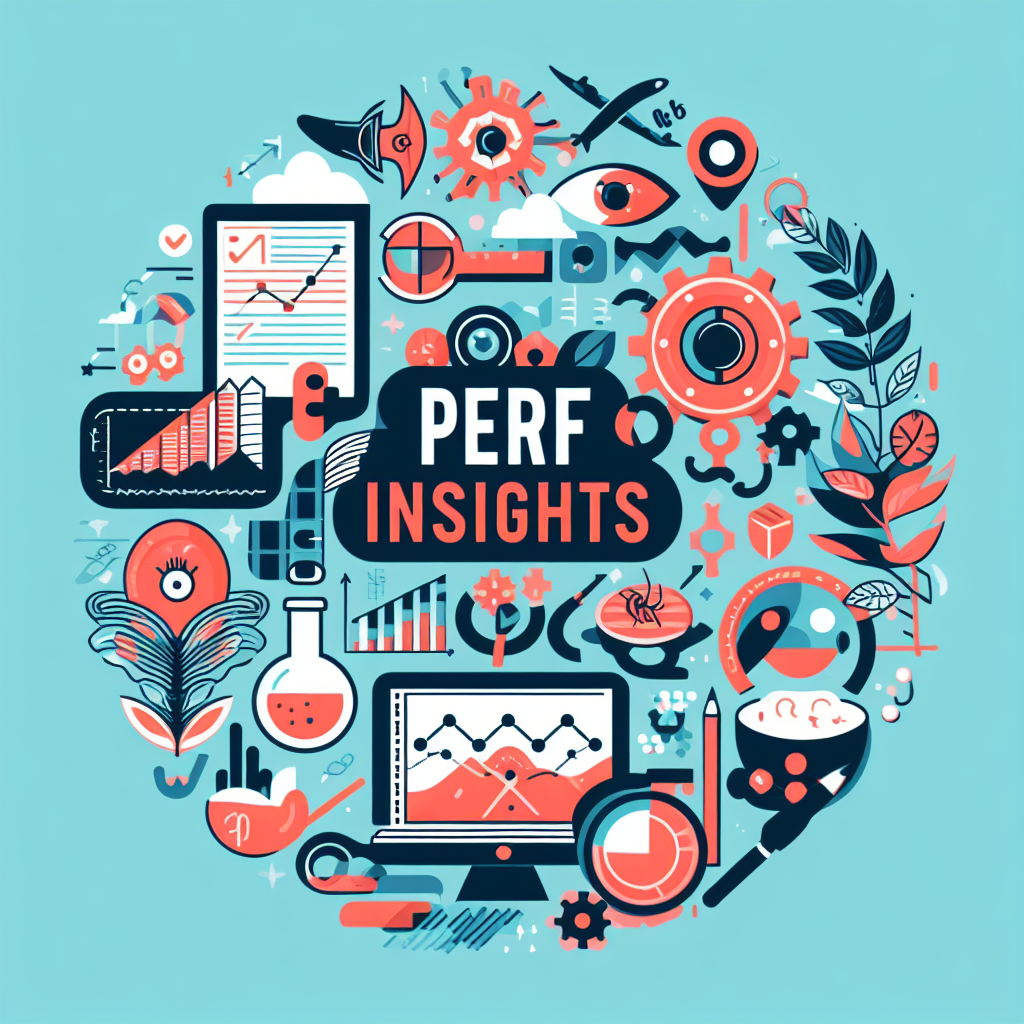
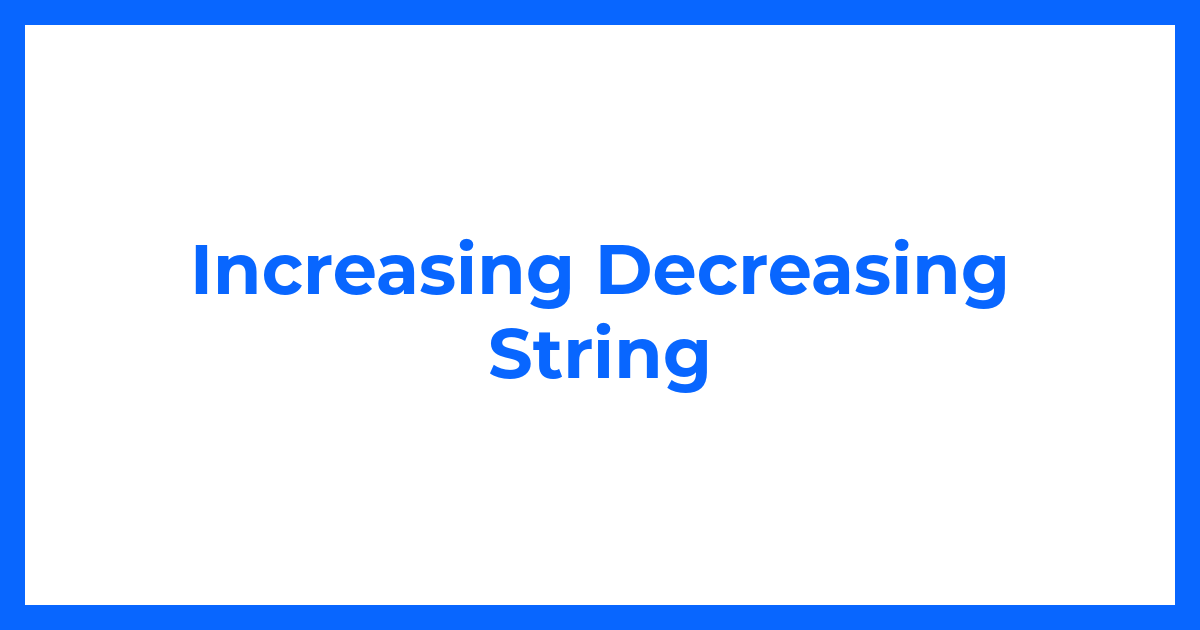
You are given a string s
. Reorder the string using the following algorithm:
Pick the smallest character from
s
and append it to the result.Pick the smallest character from
s
which is greater than the last appended character to the result and append it.Repeat step 2 until you cannot pick more characters.
Pick the largest character from
s
and append it to the result.Pick the largest character from
s
which is smaller than the last appended character to the result and append it.Repeat step 5 until you cannot pick more characters.
Repeat the steps from 1 to 6 until you pick all characters from
s
.
In each step, If the smallest or the largest character appears more than once you can choose any occurrence and append it to the result.
Return the result string after sorting s
with this algorithm.
LeetCode Problem - 1370
class Solution {
// Function to sort a string in ascending and descending order of characters alternately
public String sortString(String s) {
// Map to store character frequencies
Map<Character, Integer> map = new HashMap<>();
// Count the frequency of each character
for(char c : s.toCharArray()){
map.put(c, map.getOrDefault(c, 0)+1);
}
// StringBuilder to store the sorted string
StringBuilder sb = new StringBuilder();
// Loop until all characters are processed
while(!map.isEmpty()){
try{
// Sort characters in ascending order
Set<Character> keySet = map.keySet();
List<Character> keyList = new ArrayList<>(keySet);
Collections.sort(keyList);
// Append characters in ascending order to the result string
for(char c : keyList){
sb.append(c);
map.put(c, map.getOrDefault(c, 0)-1);
// Remove the character if its frequency becomes 0
if (map.get(c) == 0){
map.remove(c);
}
}
// Sort characters in descending order
Set<Character> keySet2 = map.keySet();
List<Character> keyList2 = new ArrayList<>(keySet2);
Collections.sort(keyList2, Collections.reverseOrder());
// Append characters in descending order to the result string
for(char c : keyList2){
sb.append(c);
map.put(c, map.getOrDefault(c, 0)-1);
// Remove the character if its frequency becomes 0
if (map.get(c) == 0){
map.remove(c);
}
}
} catch (Exception e){
// Continue to the next iteration if an exception occurs (e.g., ConcurrentModificationException)
continue;
}
}
// Return the sorted string
return sb.toString();
}
}
Subscribe to my newsletter
Read articles from Gulshan Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
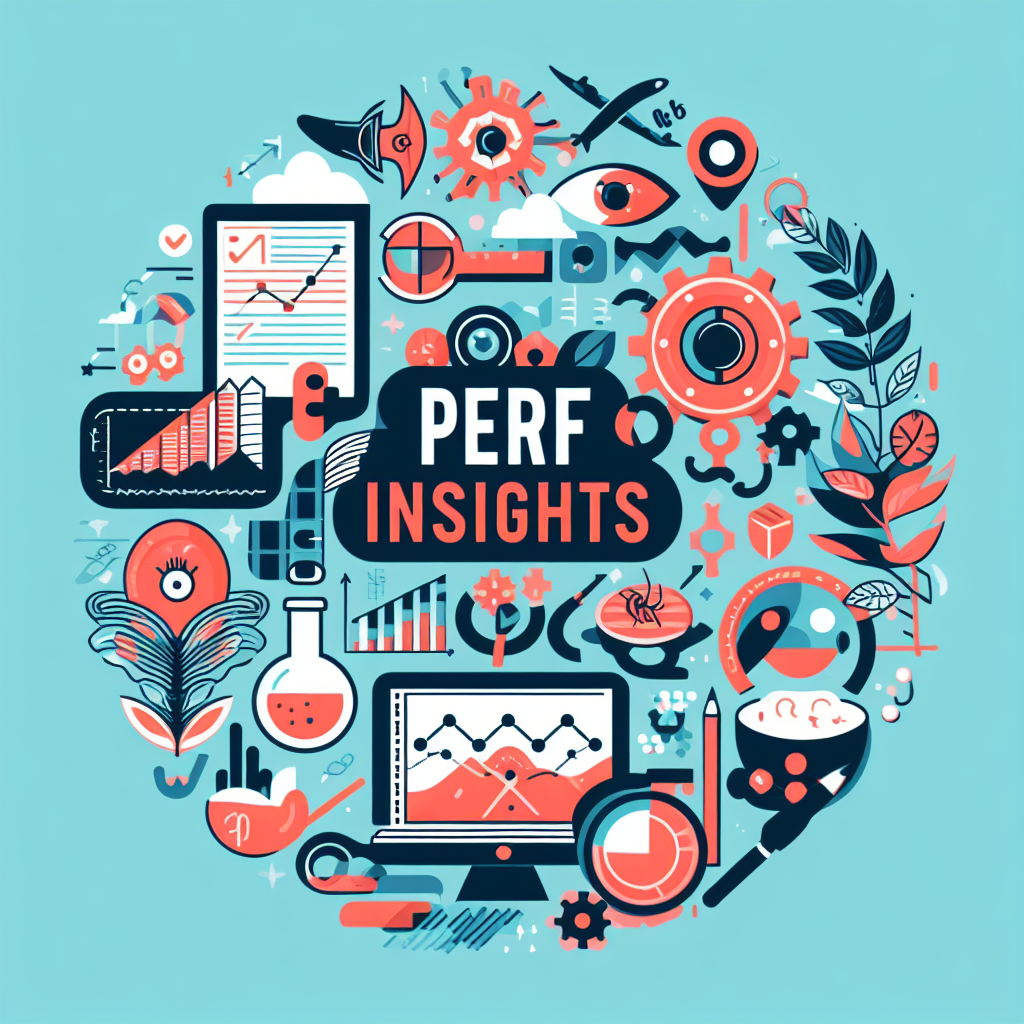
Gulshan Kumar
Gulshan Kumar
As a Systems Engineer at Tata Consultancy Services, I deliver exceptional software products for mobile and web platforms, using agile methodologies and robust quality maintenance. I am experienced in performance testing, automation testing, API testing, and manual testing, with various tools and technologies such as Jmeter, Azure LoadTest, Selenium, Java, OOPS, Maven, TestNG, and Postman. I have successfully developed and executed detailed test plans, test cases, and scripts for Android and web applications, ensuring high-quality standards and user satisfaction. I have also demonstrated my proficiency in manual REST API testing with Postman, as well as in end-to-end performance and automation testing using Jmeter and selenium with Java, TestNG and Maven. Additionally, I have utilized Azure DevOps for bug tracking and issue management.