A Comprehensive Guide to Linux Shell Scripting
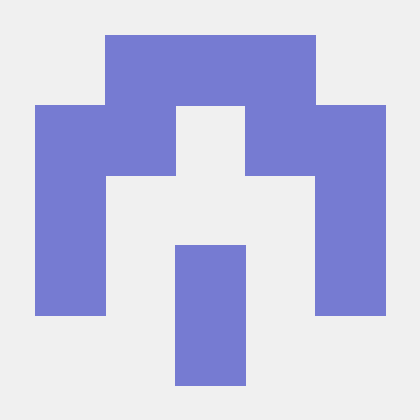
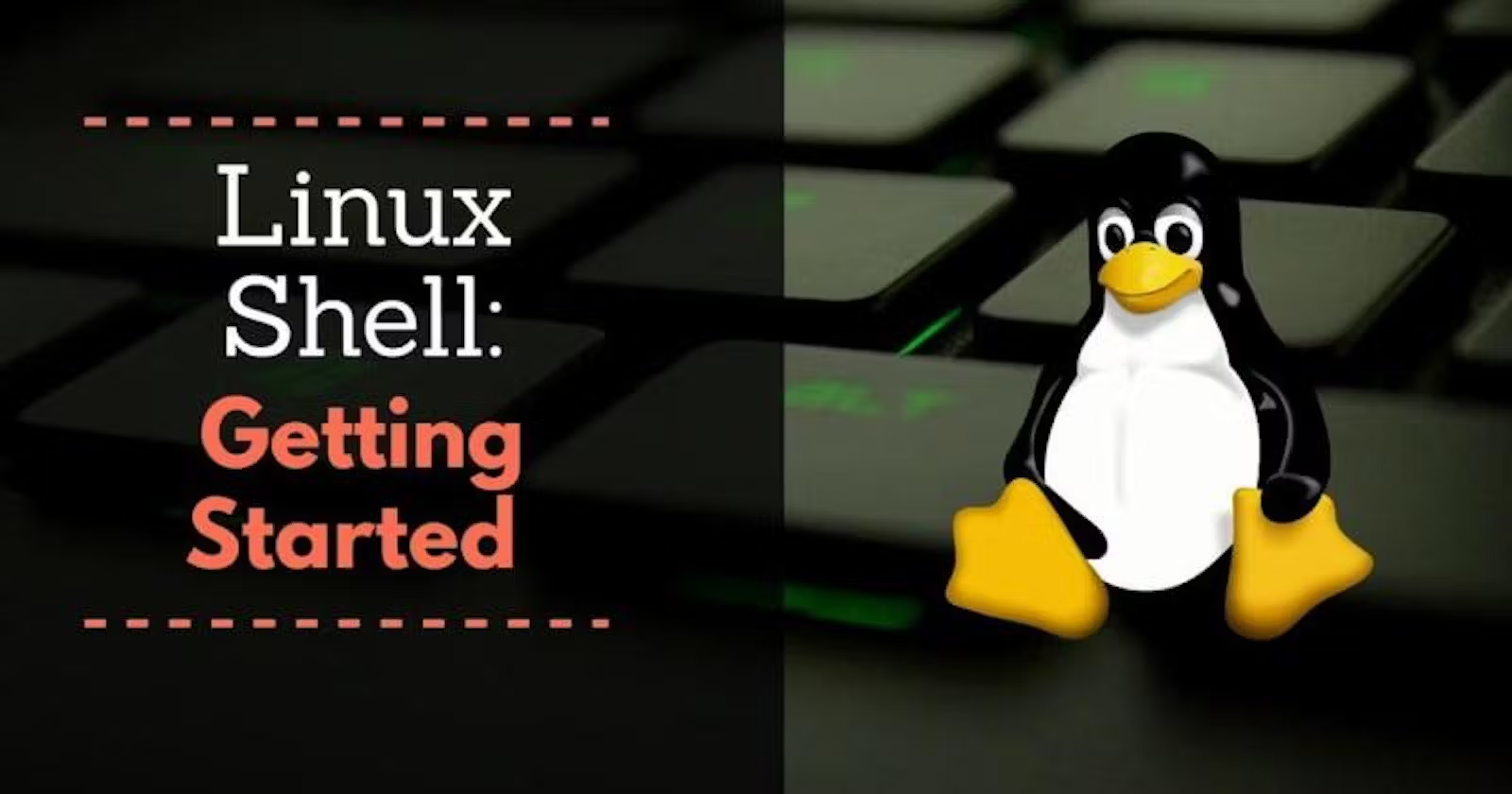
Introduction
Linux shell scripting is a powerful way to automate repetitive tasks, manage system operations, and perform complex operations efficiently. Shell scripts are text files containing a series of commands that the shell interpreter reads and executes. This guide covers the basics of shell scripting, essential commands, and best practices to help you get started.
What is Shell Scripting?
A shell script is a text file that contains a sequence of commands for a UNIX-based operating system. It is used to automate tasks such as file manipulation, program execution, and system administration. The shell is a command-line interpreter that provides a user interface for the UNIX operating system.
Getting Started
Creating a Shell Script:
Use a text editor (like
nano
,vim
, orgedit
) to create a new file.Start the script with the shebang (
#!
) followed by the path to the shell interpreter, usually/bin/bash
.
#!/bin/bash
Making the Script Executable:
- Change the file permissions to make the script executable using the
chmod
command.
- Change the file permissions to make the script executable using the
chmod +x script.sh
Running the Script:
- Execute the script by typing
./
script.sh
in the terminal.
- Execute the script by typing
Basic Shell Scripting Commands
Echo:
- Used to print text to the terminal.
echo "Hello, World!"
Variables:
- Used to store data that can be referenced and manipulated within the script.
name="John Doe"
echo "Hello, $name"
User Input:
- Read input from the user.
echo "Enter your name:"
read name
echo "Hello, $name"
Conditional Statements:
- Used to perform different actions based on different conditions.
if [ $name == "John" ]; then
echo "Welcome, John!"
else
echo "Who are you?"
fi
Loops:
- Used to repeat a block of code.
for i in 1 2 3 4 5
do
echo "Welcome $i times"
done
Functions:
- Used to group commands into a single command.
function greet {
echo "Hello, $1"
}
greet "Alice"
Practical Examples
Backup Script:
- A simple script to back up a directory.
#!/bin/bash
src="/home/user/documents"
dest="/home/user/backup"
mkdir -p $dest
cp -r $src $dest
echo "Backup completed."
System Monitoring:
- A script to check disk usage and alert if it exceeds a threshold.
#!/bin/bash
threshold=80
usage=$(df / | grep / | awk '{ print $5 }' | sed 's/%//g')
if [ $usage -gt $threshold ]; then
echo "Disk usage is above $threshold%. Please clean up."
else
echo "Disk usage is under control."
fi
Automated Updates:
- A script to update the system packages.
#!/bin/bash
sudo apt update
sudo apt upgrade -y
echo "System updated."
Best Practices
Use Comments:
- Add comments to your scripts to explain what each part of the script does.
# This script greets the user
echo "Hello, World!"
Error Handling:
- Handle errors gracefully by checking the exit status of commands.
cp /nonexistentfile /tmp
if [ $? -ne 0 ]; then
echo "Error: File not found."
fi
Use Meaningful Variable Names:
- Choose descriptive names for variables to make the script easier to understand.
username="Alice"
Keep Scripts Modular:
- Break down large scripts into smaller, reusable functions.
function backup {
# backup code
}
Test Your Scripts:
- Test scripts in a safe environment before using them in production.
Conclusion
Shell scripting is an invaluable skill for anyone working with UNIX-based systems. By automating repetitive tasks, you can save time and reduce errors. This guide has covered the basics, but there is much more to learn. Practice writing scripts, explore advanced features, and you’ll soon become proficient in shell scripting.
For more in-depth learning, consider exploring resources like the Advanced Bash-Scripting Guide, online tutorials, and community forums.
By mastering shell scripting, you can enhance your productivity and take full advantage of the powerful capabilities of the UNIX/Linux command line.
Subscribe to my newsletter
Read articles from Vikram directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
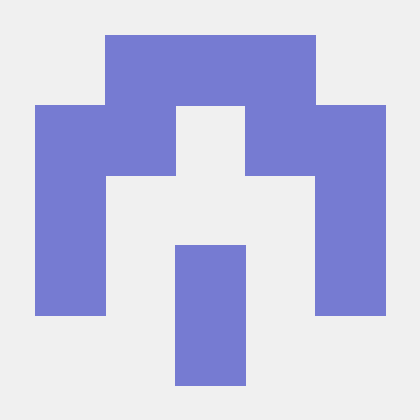