Mastering Angular Change Detection: Understanding OnPush Strategy

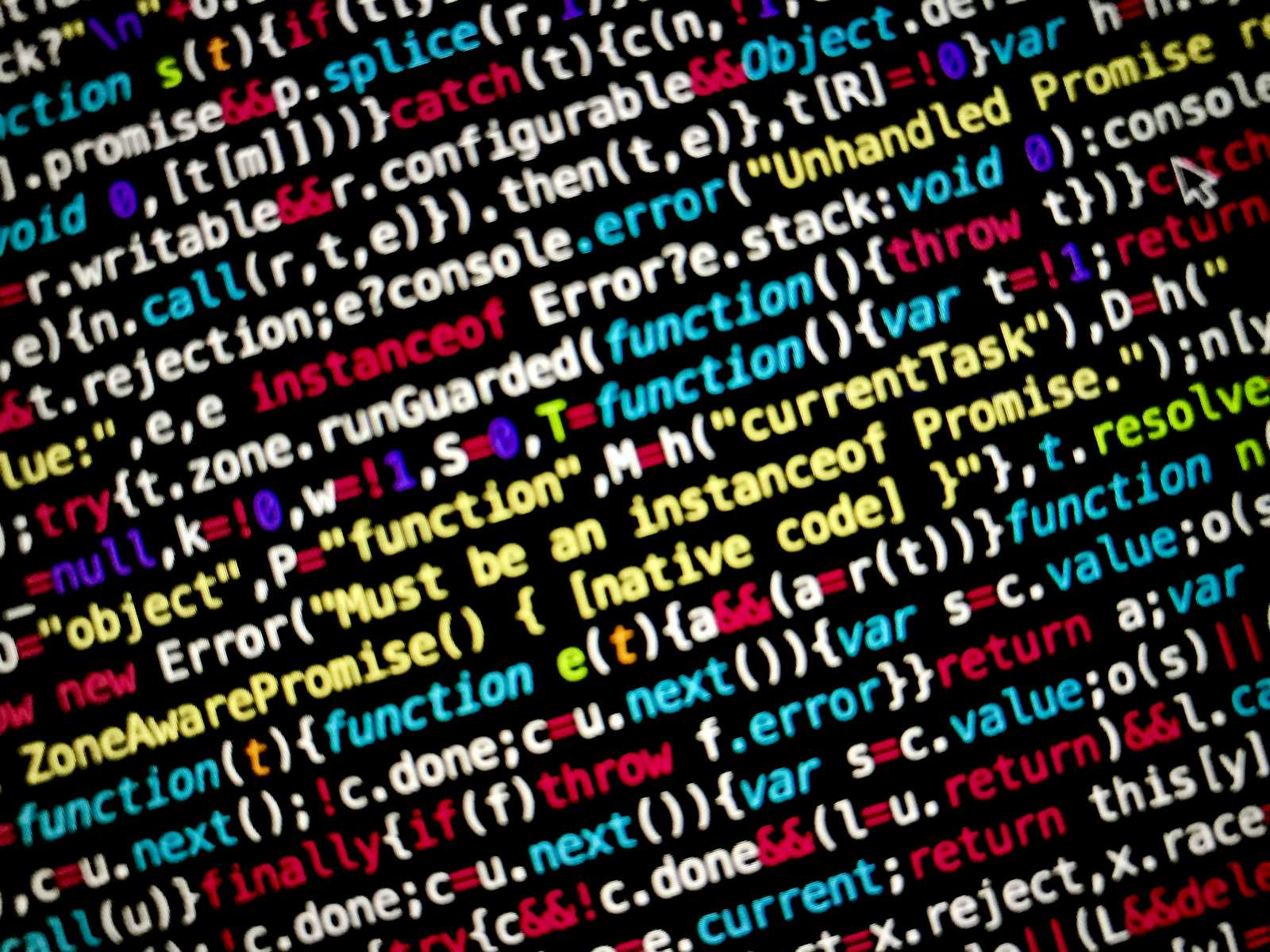
In the vibrant world of Angular development, mastering change detection is key to creating efficient and high-performance applications. One of the important aspects of this is understanding the ChangeDetectionStrategy.OnPush
. Let's delve into this topic with an example that resonates with the meticulous and detail-oriented style.
Introduction to Change Detection
Angular’s change detection mechanism is the magic that updates the user interface (UI) whenever the underlying data changes. By default, Angular uses the ChangeDetectionStrategy.Default
, which checks every component’s bindings on each change detection cycle. While this ensures that all changes are detected, it can lead to performance bottlenecks, especially in large applications.
To address this, Angular provides an alternative: ChangeDetectionStrategy.OnPush
. This strategy optimizes change detection by restricting it to specific scenarios, leading to significant performance improvements.
When Change Detection Occurs with OnPush
When a component is set to use ChangeDetectionStrategy.OnPush
, Angular only runs change detection for that component in the following scenarios:
Input Reference Change: When any of the
@Input
properties change by reference.Event Binding: When an event bound in the template is triggered.
Manual Triggering: When you manually trigger change detection using methods like
ChangeDetectorRef.detectChanges()
orChangeDetectorRef.markForCheck()
.Observable Async Pipe: When an observable used in the template emits a new value.
Propagation of Change Detection: Parent to Child
Initial Rendering
Consider the initialization phase. When a parent component initializes, it renders the child component as part of its template. Let's consider an example to understand this better.
The Example Scenario
Suppose we have two components, ParentComponent
and ChildComponent
, both using ChangeDetectionStrategy.OnPush
.
// Parent component
@Component({
selector: 'app-parent',
template: `
<div>
<button (click)="changeValue()">Change Value</button>
<app-child [value]="value"></app-child>
</div>
`,
changeDetection: ChangeDetectionStrategy.OnPush
})
export class ParentComponent {
value = { data: 'initial' };
changeValue() {
this.value = { data: 'changed' }; // Changing the reference
}
}
// Child component
@Component({
selector: 'app-child',
template: `
<div>{{ value.data }}</div>
`,
changeDetection: ChangeDetectionStrategy.OnPush
})
export class ChildComponent {
@Input() value: any;
}
Breaking Down the Example
Initialization:
- When the
ParentComponent
initializes, it rendersChildComponent
with the input propertyvalue
set to{ data: 'initial' }
.
- When the
Input Changes:
Clicking the "Change Value" button in
ParentComponent
changes the reference ofvalue
.Since
value
is an input property ofChildComponent
, Angular detects the reference change and updates the child component's view accordingly.
Event Binding:
- If an event such as a button click occurs in the child component, Angular will run change detection for the child component and propagate any necessary updates to the parent.
Manual Triggering:
- Developers can manually trigger change detection using methods like
markForCheck()
ordetectChanges()
to ensure that the UI updates as expected.
- Developers can manually trigger change detection using methods like
Conclusion
Understanding ChangeDetectionStrategy.OnPush
is crucial for optimizing Angular applications. This strategy limits change detection to specific conditions, such as input reference changes, event bindings, and manual triggers. By adopting OnPush
, developers can significantly enhance performance, especially in complex and large-scale applications.
In summary, OnPush
change detection ensures that Angular applications remain responsive and efficient. Embrace this strategy to harness the true power of Angular's change detection, and enjoy the seamless and snappy performance of your applications.
Subscribe to my newsletter
Read articles from Akanksha Saxena directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
