How to Use Red-Amber-Green (RAG) Status Indicators for Table Selection in PostgreSQL
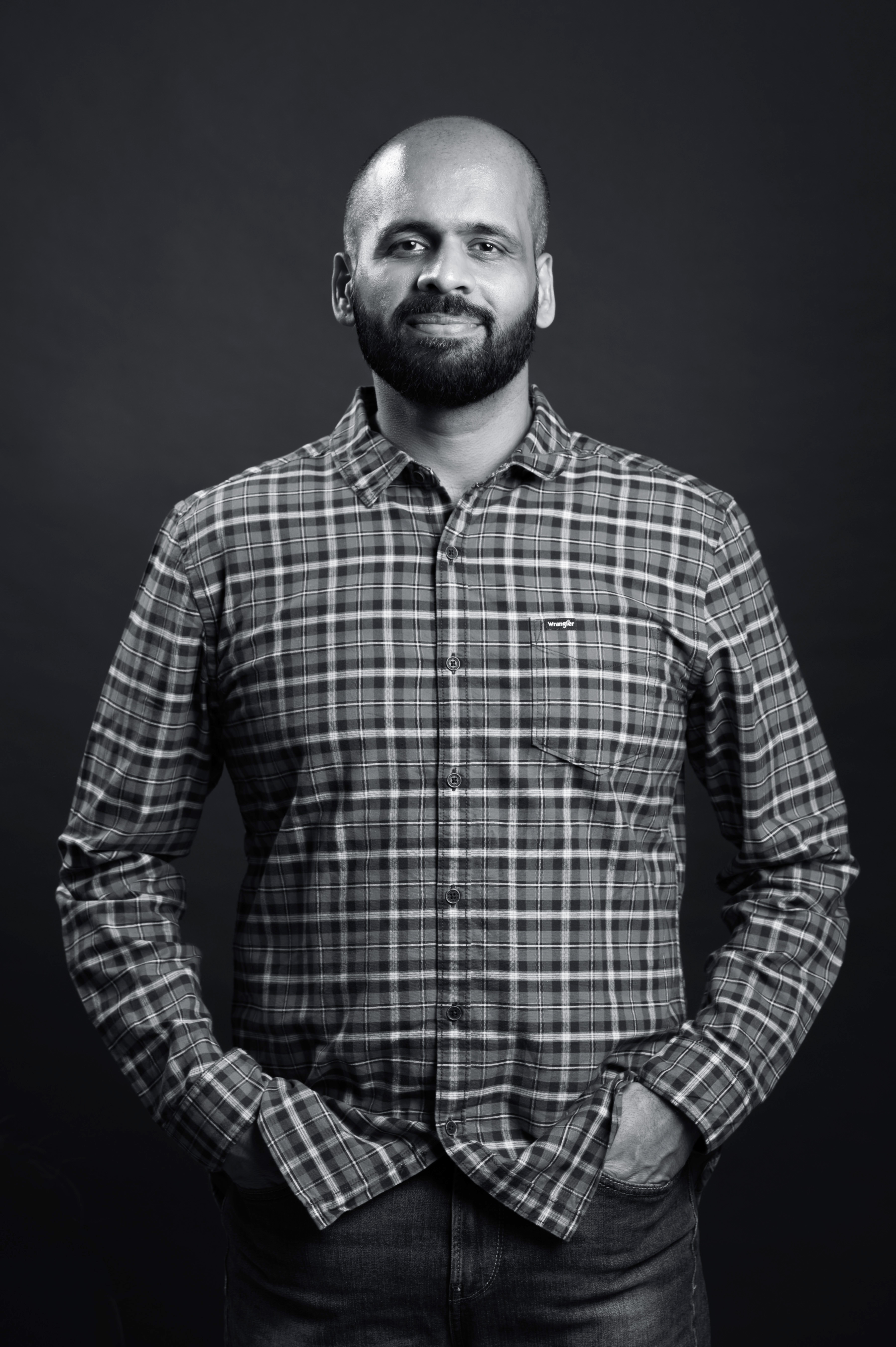
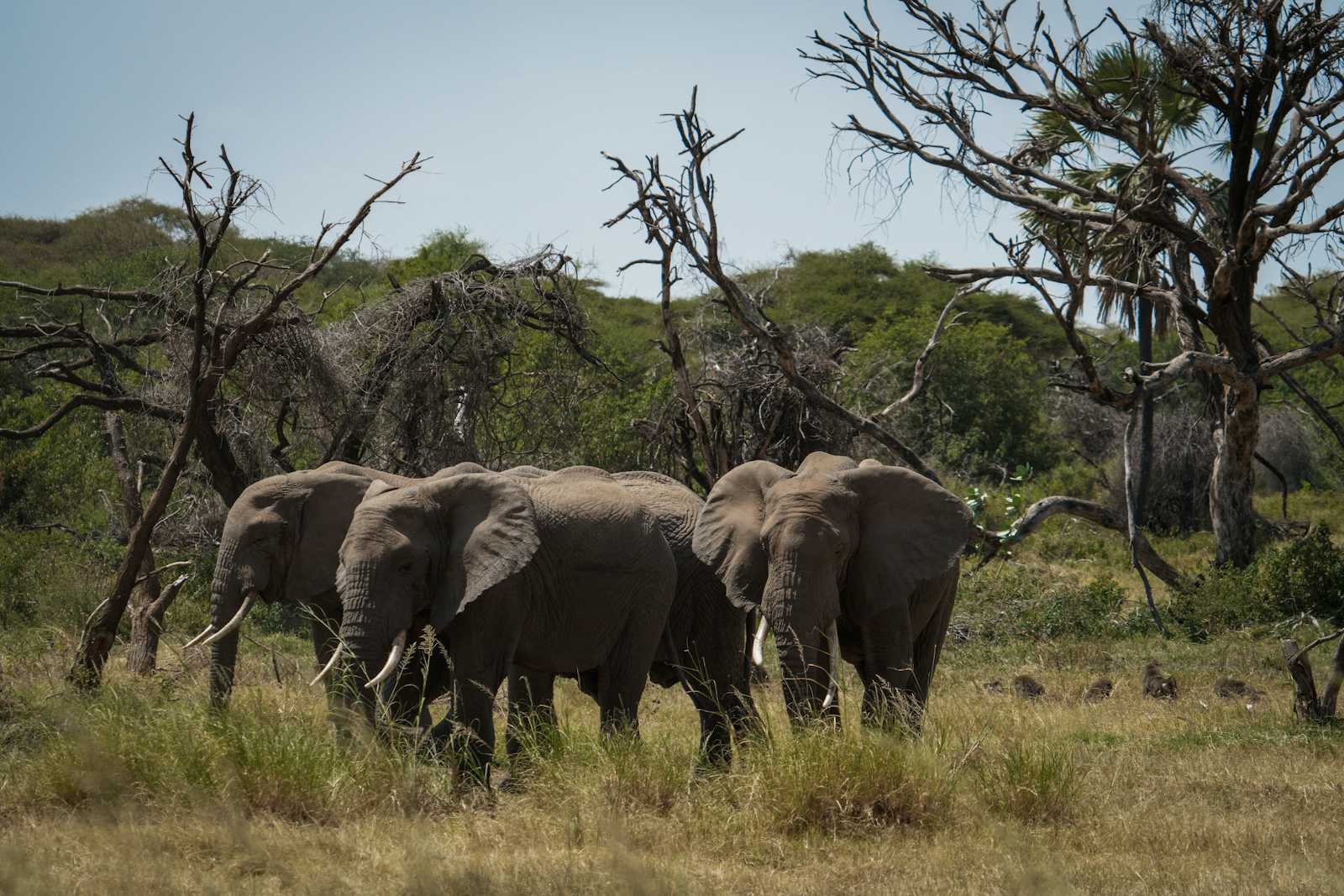
Incorporating Red-Amber-Green (RAG) status indicators for table selection in PostgreSQL involves using color-coded statuses to represent different states or conditions of records. This can be particularly useful in dashboards or reports where visual cues help quickly identify critical, warning, and normal statuses.
Scenario
Let’s consider a scenario where you have a projects
table, and you want to assign a RAG status based on the project’s progress and deadlines.
Steps to Implement RAG Status
Define Criteria for RAG Status: Decide the rules for assigning Red, Amber, and Green statuses. For example:
Green: Project is on track (progress >= 80% and deadline is not near).
Amber: Project is slightly delayed (progress between 50% and 80% or deadline is approaching).
Red: Project is significantly delayed (progress < 50% or past deadline).
Create the
projects
Table: This table will store the project details including progress and deadline.Write a Query to Calculate RAG Status: Use a CASE statement to evaluate each project and assign the appropriate RAG status.
Example
Step 1: Create the projects
Table
CREATE TABLE projects (
project_id SERIAL PRIMARY KEY,
project_name VARCHAR(255) NOT NULL,
progress INT NOT NULL, -- Progress percentage
deadline DATE NOT NULL,
status VARCHAR(10) -- To store the RAG status
);
Step 2: Insert Sample Data
INSERT INTO projects (project_name, progress, deadline) VALUES
('Project A', 90, '2024-12-31'),
('Project B', 60, '2024-07-01'),
('Project C', 40, '2024-05-15'),
('Project D', 85, '2024-06-30');
Step 3: Query to Calculate and Display RAG Status
SELECT
project_id,
project_name,
progress,
deadline,
CASE
WHEN progress >= 80 AND deadline > CURRENT_DATE + INTERVAL '1 month' THEN 'Green'
WHEN progress >= 50 AND progress < 80 AND deadline > CURRENT_DATE THEN 'Amber'
WHEN deadline <= CURRENT_DATE THEN 'Red'
ELSE 'Red'
END AS rag_status
FROM
projects;
Explanation of the Query
CASE Statement: The
CASE
statement is used to evaluate each project and determine its RAG status based on the specified criteria.Green Status: Projects with progress >= 80% and a deadline more than a month away are considered on track.
Amber Status: Projects with progress between 50% and 80% or those nearing their deadline (but not past it) are given a warning status.
Red Status: Projects with progress < 50% or those past their deadline are flagged as critical.
Result
Running the above query would yield a result set where each project is assigned a RAG status:
project_id | project_name | progress | deadline | rag_status
------------+--------------+----------+--------------+------------
1 | Project A | 90 | 2024-12-31 | Green
2 | Project B | 60 | 2024-07-01 | Amber
3 | Project C | 40 | 2024-05-15 | Red
4 | Project D | 85 | 2024-06-30 | Amber
Step 4: Update the Table with RAG Status
If you need to store the RAG status in the table:
UPDATE projects
SET status = CASE
WHEN progress >= 80 AND deadline > CURRENT_DATE + INTERVAL '1 month' THEN 'Green'
WHEN progress >= 50 AND progress < 80 AND deadline > CURRENT_DATE THEN 'Amber'
WHEN deadline <= CURRENT_DATE THEN 'Red'
ELSE 'Red'
END;
Conclusion
Incorporating RAG status in PostgreSQL involves using a CASE
statement to evaluate each row in your table based on predefined criteria. This approach allows you to dynamically assess and visualize the status of each record, making it easier to identify issues and take action. This technique is highly useful for project management, risk assessment, and various other monitoring applications.
Subscribe to my newsletter
Read articles from Shiv Iyer directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
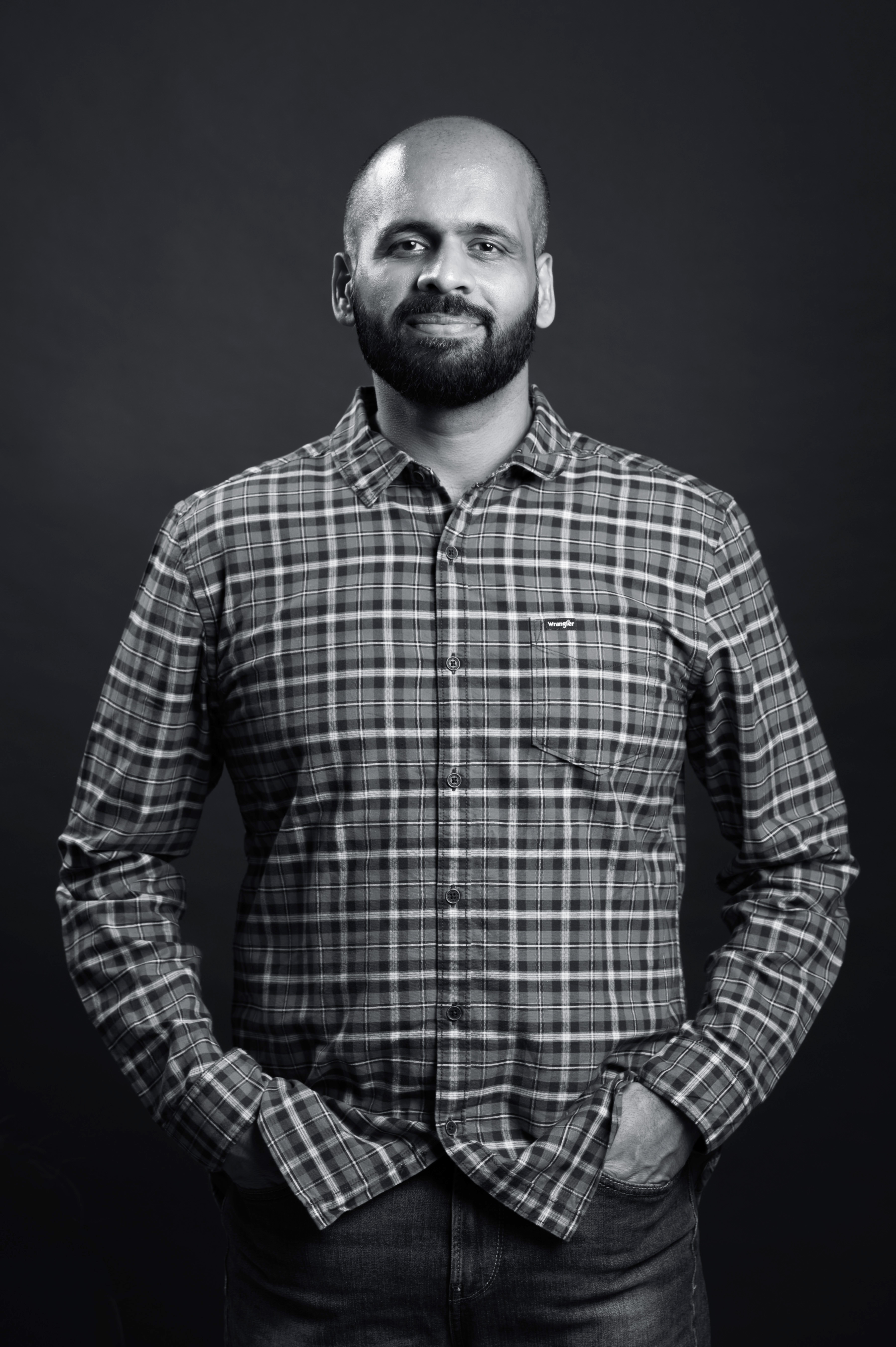
Shiv Iyer
Shiv Iyer
Over two decades of experience as a Database Architect and Database Engineer with core expertize in Database Systems Architecture/Internals, Performance Engineering, Scalability, Distributed Database Systems, SQL Tuning, Index Optimization, Cloud Database Infrastructure Optimization, Disk I/O Optimization, Data Migration and Database Security. I am the founder CEO of MinervaDB Inc. and ChistaDATA Inc.