JavaScript Concepts: Hoisting, this Keyword, and Undefined vs. Not Defined

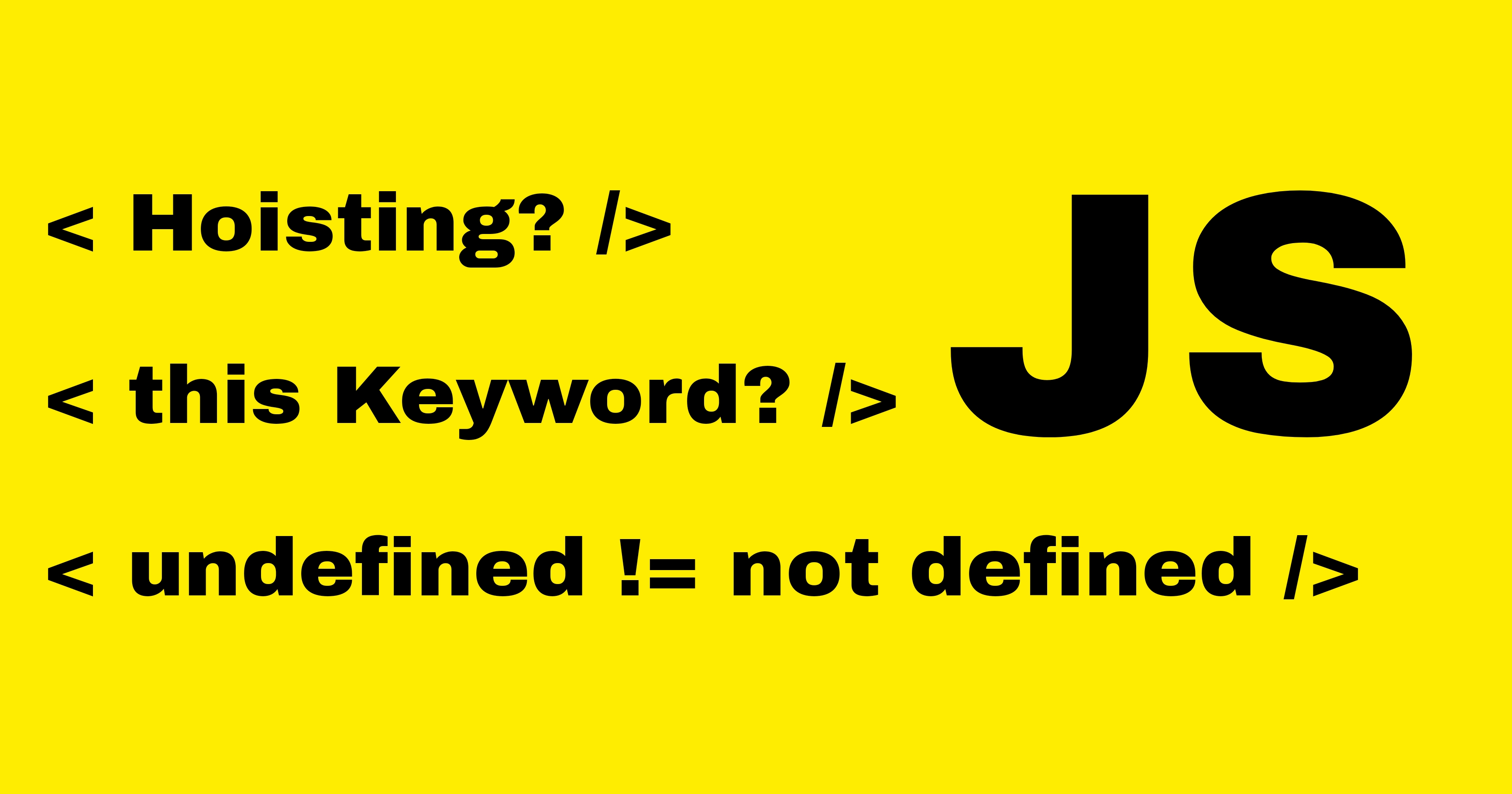
JavaScript is a versatile and powerful language, but it comes with some concepts that can be tricky to understand. In this blog, we’ll explore three important concepts: hoisting, the this
keyword, and the difference between undefined
and not defined
.
Hoisting in JavaScript
Hoisting is a phenomenon in JavaScript where you can access variables and functions even before you initialize them. This happens because during the compilation phase, the JavaScript engine moves the declarations of variables and functions to the top of their containing scope. However, only the declarations are hoisted, not the initializations.
Example:
console.log(myVar); // Output: undefined
var myVar = 5;
console.log(myVar); // Output: 5
myFunction(); // Output: "Hello, World!"
function myFunction() {
console.log("Hello, World!");
}
In the example above, the variable myVar
and the function myFunction
are hoisted. This means you can reference them before their actual declarations in the code.
The this
Keyword
The this
keyword in JavaScript is a bit tricky as its value depends on the context in which it is used. In a global execution context (outside of any function), this
refers to the global object, which is window
in the case of browsers.
Global Context:
console.log(this === window); // Output: true
Function Context:
When you create a function, this
inside the function refers to the object from which the function was called, unless the function is called as a method of an object.
function showThis() {
console.log(this);
}
showThis(); // Output: window (in a browser)
const obj = {
method: showThis
};
obj.method(); // Output: obj
Important Points:
At the global level,
this
points to the global object.Inside a function,
this
can vary depending on how the function is called.In strict mode,
this
will beundefined
if the function is called without an object.
Undefined vs. Not Defined
In JavaScript, undefined
and not defined
are different concepts.
undefined
:
When JavaScript allocates memory for variables and functions, it initializes them with the value undefined
. This is a placeholder indicating that the variable has been declared but not yet assigned a value.
var myVar;
console.log(myVar); // Output: undefined
not defined
:
A variable is not defined
when it has not been declared in any scope accessible to the code being executed.
console.log(myVar); // Output: Uncaught ReferenceError: myVar is not defined
Key Differences:
undefined
means a variable has been declared but has not yet been assigned a value.not defined
means the variable has not been declared in the current scope.
Conclusion
Understanding these core JavaScript concepts—hoisting, the this
keyword, and the difference between undefined
and not defined
—is crucial for mastering the language. These principles form the foundation of how JavaScript code is interpreted and executed, enabling you to write more predictable and error-free code.
Happy coding!
Subscribe to my newsletter
Read articles from Bhavesh Jadhav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Bhavesh Jadhav
Bhavesh Jadhav
I am a passionate web developer from India, currently pursuing an MCA from Government College of Engineering, Aurangabad. With a strong foundation in HTML, CSS, JavaScript, and expertise in frameworks like React and Node.js, I create dynamic and responsive web applications. My skill set extends to backend development with PHP, MySQL, and MongoDB. I also have experience with AJAX, jQuery, and I am proficient in Python and Java. As an AI enthusiast, I enjoy leveraging AI tools and LLMs to enhance my projects. Eager to expand my horizons, I am delving into DevOps to optimize development workflows and improve deployment processes. Always keen to learn and adapt, I strive to integrate cutting-edge technologies into my work. Let's connect and explore the world of technology together!