Mastering List Comprehension in Python

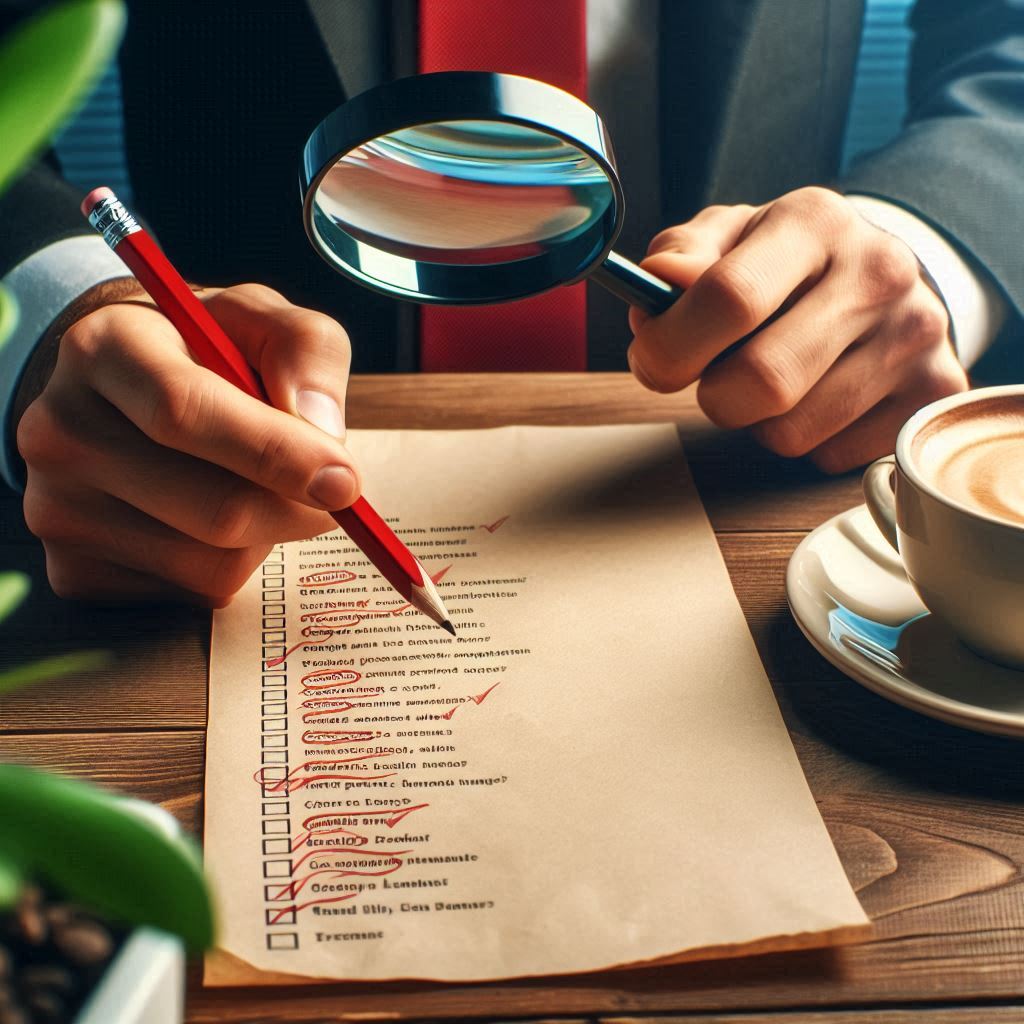
List comprehension is one of Python's most powerful and readable features. It offers a concise way to create lists and is often more compact and faster than traditional for-loops or the map()
function. In this blog post, we'll explore the syntax and various use cases of list comprehension, illustrating its importance and efficiency in Python programming.
Understanding List Comprehension
The basic syntax for list comprehension is as follows:
[expression-involving-loop-variable for loop-variable in sequence]
This structure steps over every element in a sequence, setting the loop variable to each element one at a time. It then builds up a list by evaluating the expression for each element. This approach eliminates the need for lambda functions and typically results in more readable and compact code compared to using map()
or a traditional for-loop.
Basic Example
Let's start with a simple example where we create a list of integers from 0 to 9.
ListOfNumbers = [x for x in range(10)]
print(ListOfNumbers)
# Output: [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
Nested Loops in List Comprehension
List comprehension can also handle nested loops. Here's an example that generates pairs of numbers:
pairs = [[x, y] for x in [1, 2, 3] for y in [4, 5, 6]]
print(pairs)
# Output: [[1, 4], [1, 5], [1, 6], [2, 4], [2, 5], [2, 6], [3, 4], [3, 5], [3, 6]]
This is equivalent to the following nested for-loop:
results = []
for x in [1, 2, 3]:
for y in [4, 5, 6]:
results.append([x, y])
print(results)
# Output: [[1, 4], [1, 5], [1, 6], [2, 4], [2, 5], [2, 6], [3, 4], [3, 5], [3, 6]]
Conditional List Comprehension
List comprehension supports conditional expressions to filter items. Here's an example that creates a list of multiples of 3 below 10:
ListOfThreeMultiples = [x for x in range(10) if x % 3 == 0]
print(ListOfThreeMultiples)
# Output: [0, 3, 6, 9]
Multiple Conditions in List Comprehension
You can also add multiple conditions in a list comprehension. For instance, to create a list of even numbers greater than 10:
numbers = [1, 4, 12, 15, 22, 30, 5, 8, 11]
result = [num for num in numbers if num % 2 == 0 if num > 10]
print(result)
# Output: [12, 22, 30]
Real-World Example
Let's consider a practical application of list comprehension. Suppose we have a dictionary of parks and a list of squirrels in each park. We want to get the primary fur color of squirrels in a specific park.
squirrels_by_park = {
'Central Park': [{'primary_fur_color': 'Gray'}, {'primary_fur_color': 'Black'}, {}],
'Prospect Park': [{'primary_fur_color': 'Cinnamon'}, {}]
}
park_name = 'Central Park'
fur_colors = [squirrel.get('primary_fur_color', 'N/A') for squirrel in squirrels_by_park[park_name]]
print(fur_colors)
# Output: ['Gray', 'Black', 'N/A']
Conclusion
List comprehension is a powerful feature that simplifies the creation and manipulation of lists in Python. Its ability to replace traditional for-loops with a more readable and concise syntax makes it an essential tool for any Python programmer. By mastering list comprehension, you can write cleaner, more efficient code, making your programs easier to understand and maintain. So, start incorporating list comprehensions into your coding practice and experience the elegance and efficiency they bring to your Python projects!
Subscribe to my newsletter
Read articles from Emeron Marcelle directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Emeron Marcelle
Emeron Marcelle
As a doctoral scholar in Information Technology, I am deeply immersed in the world of artificial intelligence, with a specific focus on advancing the field. Fueled by a strong passion for Machine Learning and Artificial Intelligence, I am dedicated to acquiring the skills necessary to drive growth and innovation in this dynamic field. With a commitment to continuous learning and a desire to contribute innovative ideas, I am on a path to make meaningful contributions to the ever-evolving landscape of Machine Learning.