Material on Development and Implementation of Applications in Organizations

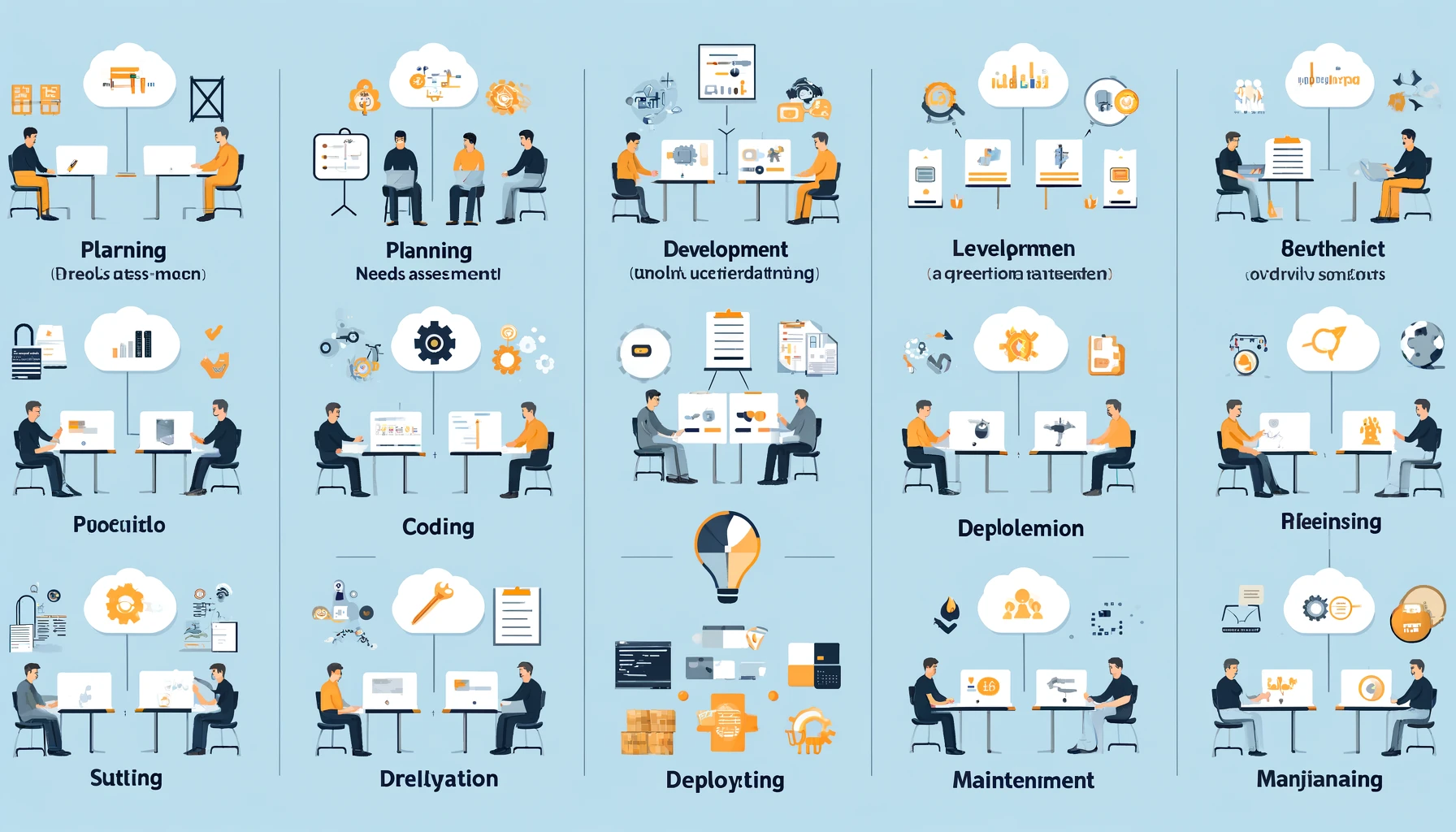
Introduction
Developing and implementing applications in organizations, such as bureaucracy, services, companies, and SMEs, is a complex process that requires a deep understanding of business needs and relevant technology. In this material, we will discuss the basic theory, creation steps, development flow, text visualization, and implementation examples using the Dart language and Serverpod backend.
Basic Theory
Requirement Analysis
Identifying business and user needs.
Collecting and analyzing data from stakeholders.
Creating a requirements specification document.
System Design
Designing the system architecture.
Designing the database.
Designing UI/UX.
Development
Selecting technology.
Writing program code.
Testing and debugging.
Implementation
Application deployment.
Data migration.
User training.
Maintenance
Monitoring application performance.
Bug fixing.
Feature updates.
Creation Steps
Requirement Analysis
Hold meetings with stakeholders.
Document the requirements in a specification document.
System Design
Use tools like UML to design the system architecture.
Design the database schema using ERD (Entity-Relationship Diagram).
Create wireframes and UI prototypes using tools like Figma or Adobe XD.
Development
Choose the appropriate programming language and framework (e.g., Dart and Serverpod).
Write program code based on the design.
Conduct unit and integration testing.
Implementation
Prepare the deployment environment (e.g., server or cloud service).
Deploy the application using Docker or Kubernetes.
Perform data migration if necessary.
Provide training to end-users.
Maintenance
Monitor application performance using monitoring tools (e.g., Prometheus or Grafana).
Fix identified bugs.
Release feature updates periodically.
Development Flow
Project Initiation
Kick-off meeting.
Assign project team.
Requirement Analysis
Gathering and analyzing requirements.
Creating specification document.
System Design
Architecture design.
Database design.
UI/UX design.
Development
Coding.
Testing.
Implementation
Deployment.
Data migration.
User training.
Maintenance
Monitoring.
Bug fixing.
Feature updates.
Text Visualization
Development Flow Diagram
+------------------+
| Project Initiation|
+------------------+
|
v
+-------------------+
| Requirement Analysis|
+-------------------+
|
v
+------------------+
| System Design |
+------------------+
|
v
+------------------+
| Development |
+------------------+
|
v
+------------------+
| Implementation |
+------------------+
|
v
+------------------+
| Maintenance |
+------------------+
Implementation in Dart and Serverpod
Example of Simple CRUD API Implementation with Serverpod
Setup Serverpod
dart pub global activate serverpod_cli serverpod create my_project cd my_project serverpod generate
Design Data Model Create a file
lib/protocol/user.dart
with the following content:import 'package:serverpod/serverpod.dart'; class User extends TableRow { @override String get tableName => 'users'; late int id; late String name; late String email; }
Create CRUD Endpoint Create a file
lib/server/endpoints/user_endpoint.dart
with the following content:import 'package:serverpod/serverpod.dart'; import '../generated/user_class.dart'; class UserEndpoint extends Endpoint { Future<void> createUser(Session session, User user) async { await session.db.insert(user); } Future<User?> getUser(Session session, int id) async { return await session.db.findById<User>(id); } Future<void> updateUser(Session session, User user) async { await session.db.update(user); } Future<void> deleteUser(Session session, int id) async { await session.db.delete<User>(id); } }
Run the Server
serverpod run
Example Implementation in Organizations
1. Bureaucracy
Public Service Information System
Requirement Analysis: Identify services frequently requested by the public.
System Design: Create modules for registration, status tracking, and feedback.
Development: Use Dart and Serverpod for the backend, and Flutter for the frontend.
Implementation: Deploy on government servers and integrate with existing systems.
Maintenance: Monitor performance and fix bugs periodically.
2. Companies
Human Resource Management System
Requirement Analysis: Identify HR needs such as recruitment, attendance, and payroll.
System Design: Create modules for recruitment, attendance, and payroll.
Development: Use Dart and Serverpod for the backend, and Flutter for the frontend.
Implementation: Deploy on company servers and integrate with ERP systems.
Maintenance: Monitor performance and fix bugs periodically.
3. SMEs
Sales and Inventory System
Requirement Analysis: Identify needs such as product management, sales, and reporting.
System Design: Create modules for product management, sales, and reporting.
Development: Use Dart and Serverpod for the backend, and Flutter for the frontend.
Implementation: Deploy on servers or cloud services.
Maintenance: Monitor performance and fix bugs periodically.
Developing and implementing applications in organizations requires a deep understanding of business needs and technology. With the right approach, from requirement analysis to maintenance, applications can run effectively and efficiently. Using technologies like Dart and Serverpod can help speed up the development process by providing a strong and easy-to-use framework.
References
Books:
"Designing Data-Intensive Applications" by Martin Kleppmann.
"Clean Architecture: A Craftsman's Guide to Software Structure and Design" by Robert C. Martin.
Online:
Subscribe to my newsletter
Read articles from Unggul Cahya Saputra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Unggul Cahya Saputra
Unggul Cahya Saputra
I am a developer from Indonesia