Getting Started with Lists and Dictionaries in Python
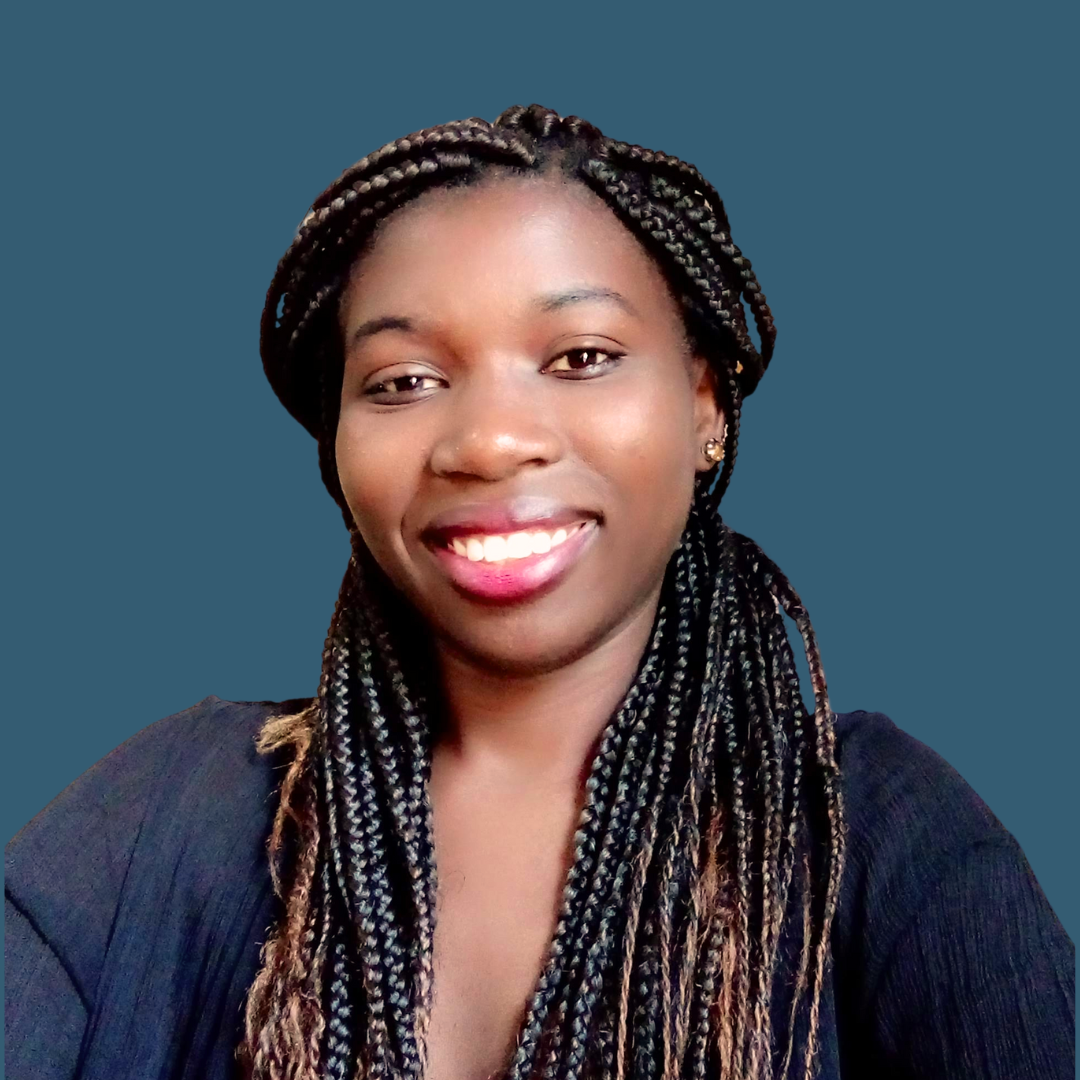
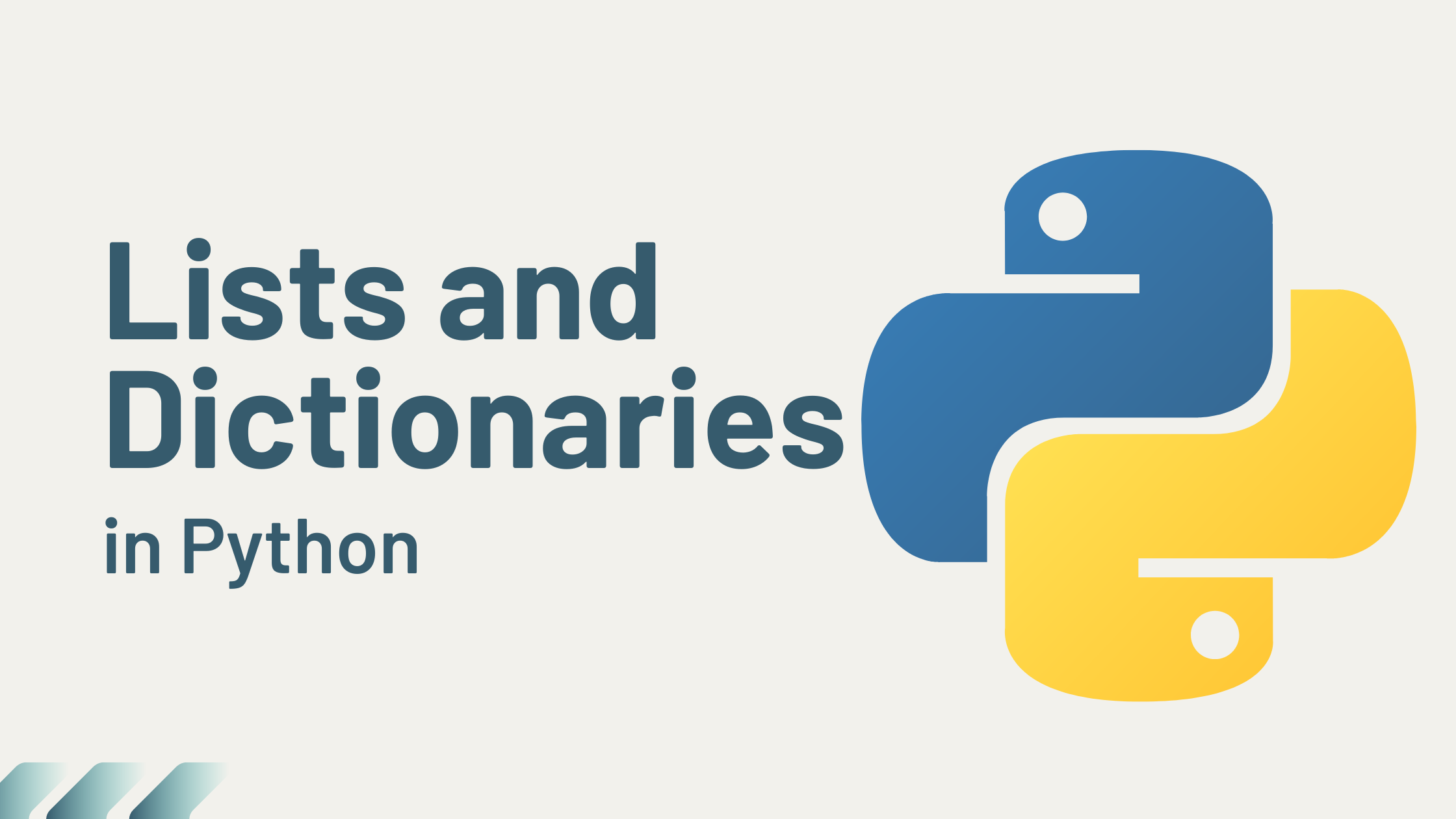
Hey there! Today, I’m diving into two fundamental data structures in Python: lists and dictionaries. If you're just starting out like me, understanding these two can be a game-changer for managing and organizing data in your programs.
So, let's explore lists and dictionaries together, step by step!
What are Lists?
A list in Python is like a dynamic array that can hold multiple items. These items can be of any data type, and you can change them after creating the list, making lists a versatile and powerful tool.
Creating a List
Creating a list is simple. You just use square brackets []
and separate your items with commas. Here’s an example:
fruits = ["apple", "banana", "cherry"]
print(fruits)
In this example, fruits
is a list containing three strings: "apple," "banana," and "cherry."
Accessing List Elements
You can access elements in a list using their index. Remember, Python indices start at 0. So, to get the first element, you use:
print(fruits[0]) # Output: apple
Modifying List Elements
Lists are mutable, meaning you can change their elements. Let’s change "banana" to "blueberry":
fruits[1] = "blueberry"
print(fruits) # Output: ['apple', 'blueberry', 'cherry']
Adding Elements to a List
You can add elements to a list using methods like append()
and insert()
. The append()
method adds an element at the end of the list:
fruits.append("date")
print(fruits) # Output: ['apple', 'blueberry', 'cherry', 'date']
The insert()
method allows you to add an element at a specific position:
fruits.insert(1, "blackberry")
print(fruits) # Output: ['apple', 'blackberry', 'blueberry', 'cherry', 'date']
Removing Elements from a List
To remove elements, you can use methods like remove()
, pop()
, and del
. The remove()
method removes the first occurrence of a specified value:
fruits.remove("cherry")
print(fruits) # Output: ['apple', 'blackberry', 'blueberry', 'date']
The pop()
method removes the element at the specified position and returns it:
popped_fruit = fruits.pop(2)
print(popped_fruit) # Output: blueberry
print(fruits) # Output: ['apple', 'blackberry', 'date']
The del
statement can also remove elements by index:
del fruits[1]
print(fruits) # Output: ['apple', 'date']
What are Dictionaries?
Dictionaries in Python are collections of key-value pairs. They are incredibly useful for storing related data and are much like real-world dictionaries where you look up a word (the key) to get its definition (the value).
Creating a Dictionary
You can create a dictionary using curly braces {}
and separating keys and values with a colon :
. Here’s an example:
person = {
"name": "Alice",
"age": 25,
"city": "New York"
}
print(person)
In this example, person
is a dictionary with three key-value pairs.
Accessing Dictionary Elements
You can access values by referring to their keys:
print(person["name"]) # Output: Alice
print(person["age"]) # Output: 25
Modifying Dictionary Elements
Dictionaries are mutable, so you can change their values:
person["age"] = 26
print(person) # Output: {'name': 'Alice', 'age': 26, 'city': 'New York'}
Adding Elements to a Dictionary
You can add new key-value pairs to a dictionary simply by assigning a value to a new key:
person["email"] = "alice@example.com"
print(person) # Output: {'name': 'Alice', 'age': 26, 'city': 'New York', 'email': 'alice@example.com'}
Removing Elements from a Dictionary
To remove elements, you can use the pop()
method or the del
statement. The pop()
method removes the item with the specified key and returns its value:
email = person.pop("email")
print(email) # Output: alice@example.com
print(person) # Output: {'name': 'Alice', 'age': 26, 'city': 'New York'}
The del
statement can also remove key-value pairs:
del person["city"]
print(person) # Output: {'name': 'Alice', 'age': 26}
Putting It All Together
Lists and dictionaries are essential tools in Python that help you manage data collections efficiently. Here’s a quick example of how you might use both in a simple program:
# List of dictionaries
students = [
{"name": "John", "grade": "A"},
{"name": "Jane", "grade": "B"},
{"name": "Doe", "grade": "C"}
]
# Adding a new student
students.append({"name": "Mary", "grade": "A"})
# Modifying a student's grade
students[2]["grade"] = "B+"
# Printing all students
for student in students:
print(f"Name: {student['name']}, Grade: {student['grade']}")
This script maintains a list of students, each represented by a dictionary. You can see how flexible and powerful combining lists and dictionaries can be!
Conclusion
Learning about lists and dictionaries has been a fascinating part of my Python journey. These data structures make it easy to organize and manipulate data, which is a fundamental skill for any programmer.
I hope this guide helps you start with lists and dictionaries in Python.
Happy coding!
Subscribe to my newsletter
Read articles from Fanny Nyayic directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
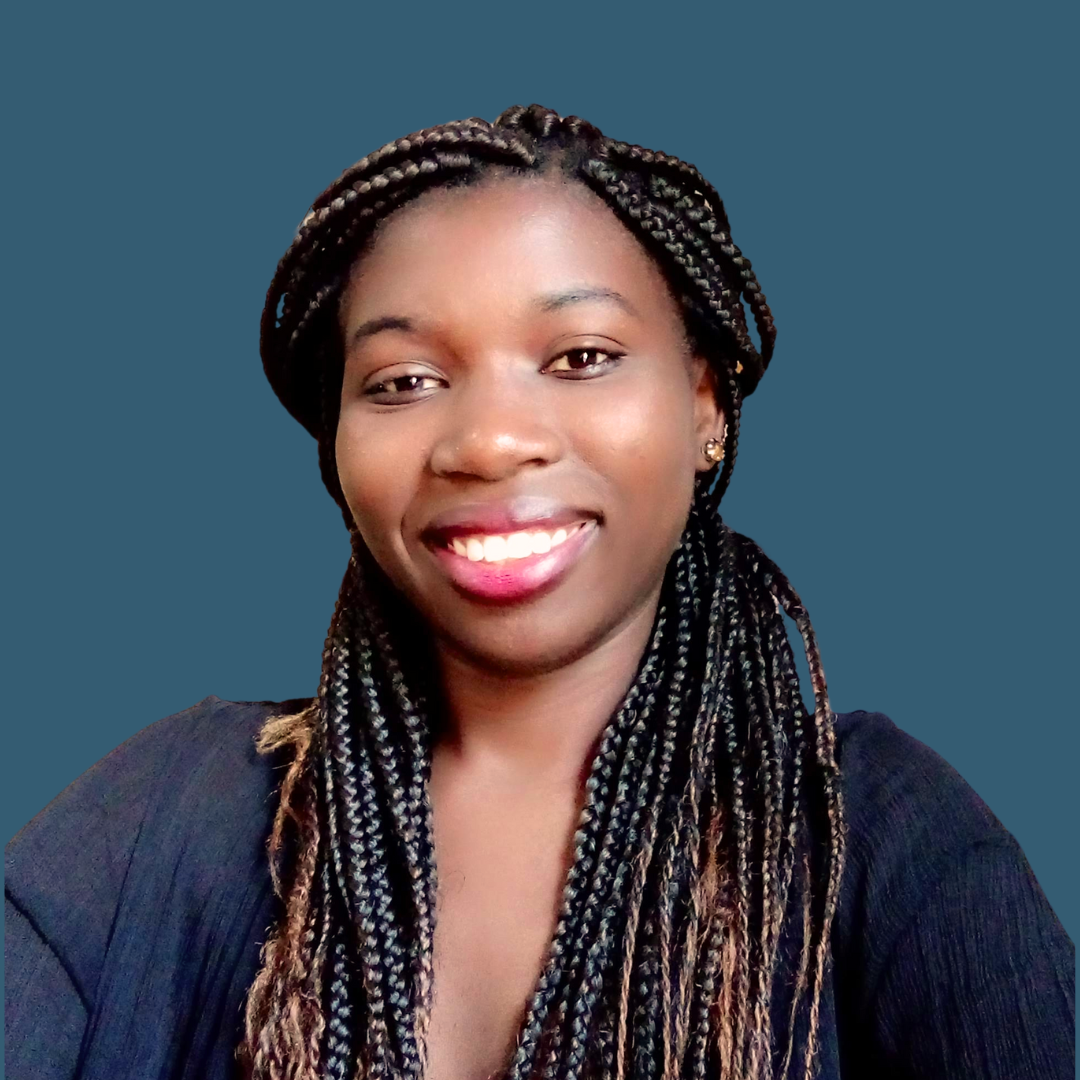
Fanny Nyayic
Fanny Nyayic
a passionate web developer, tech writer, open-source contributor & a life long learner.