[TWIL] Week of May 12, 2024
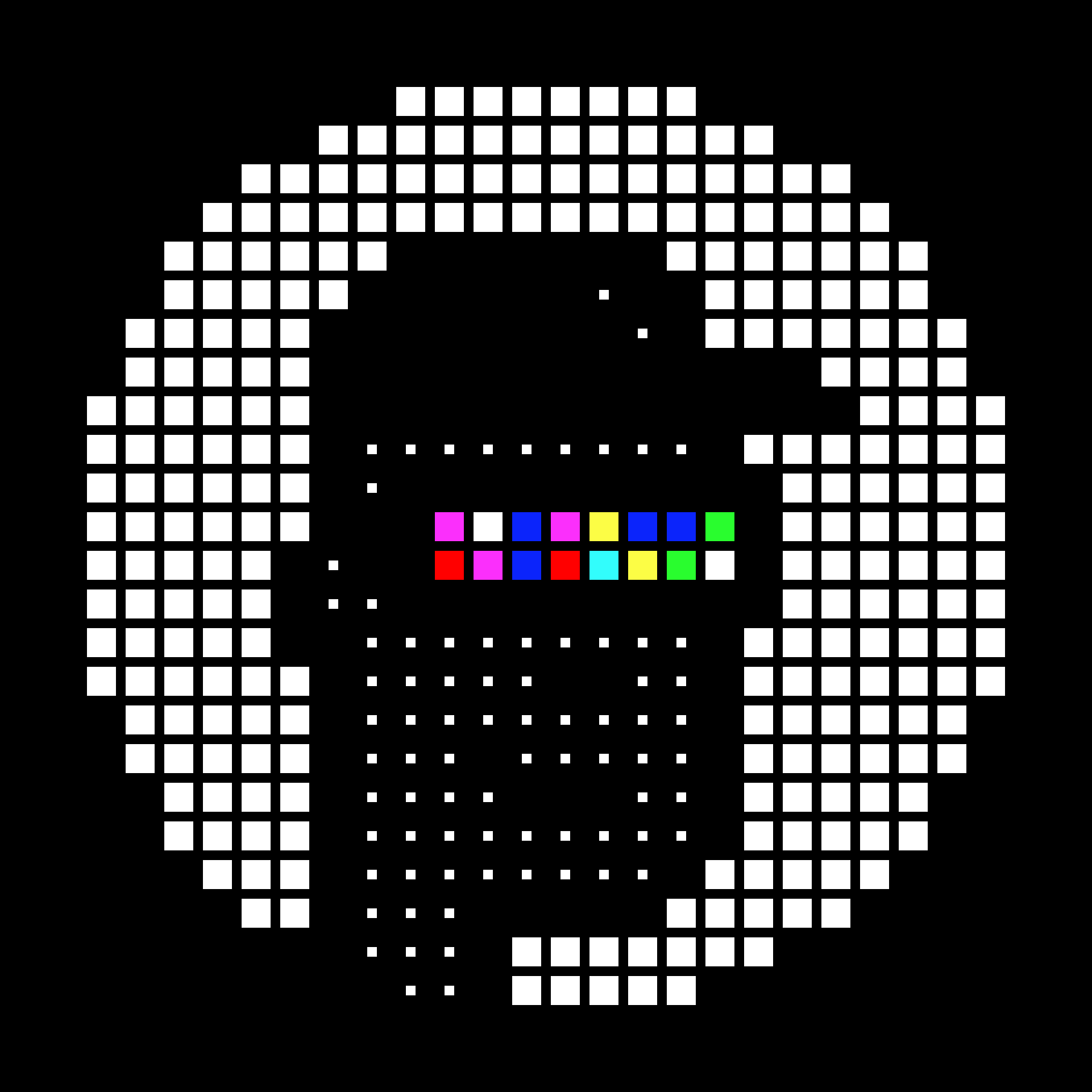
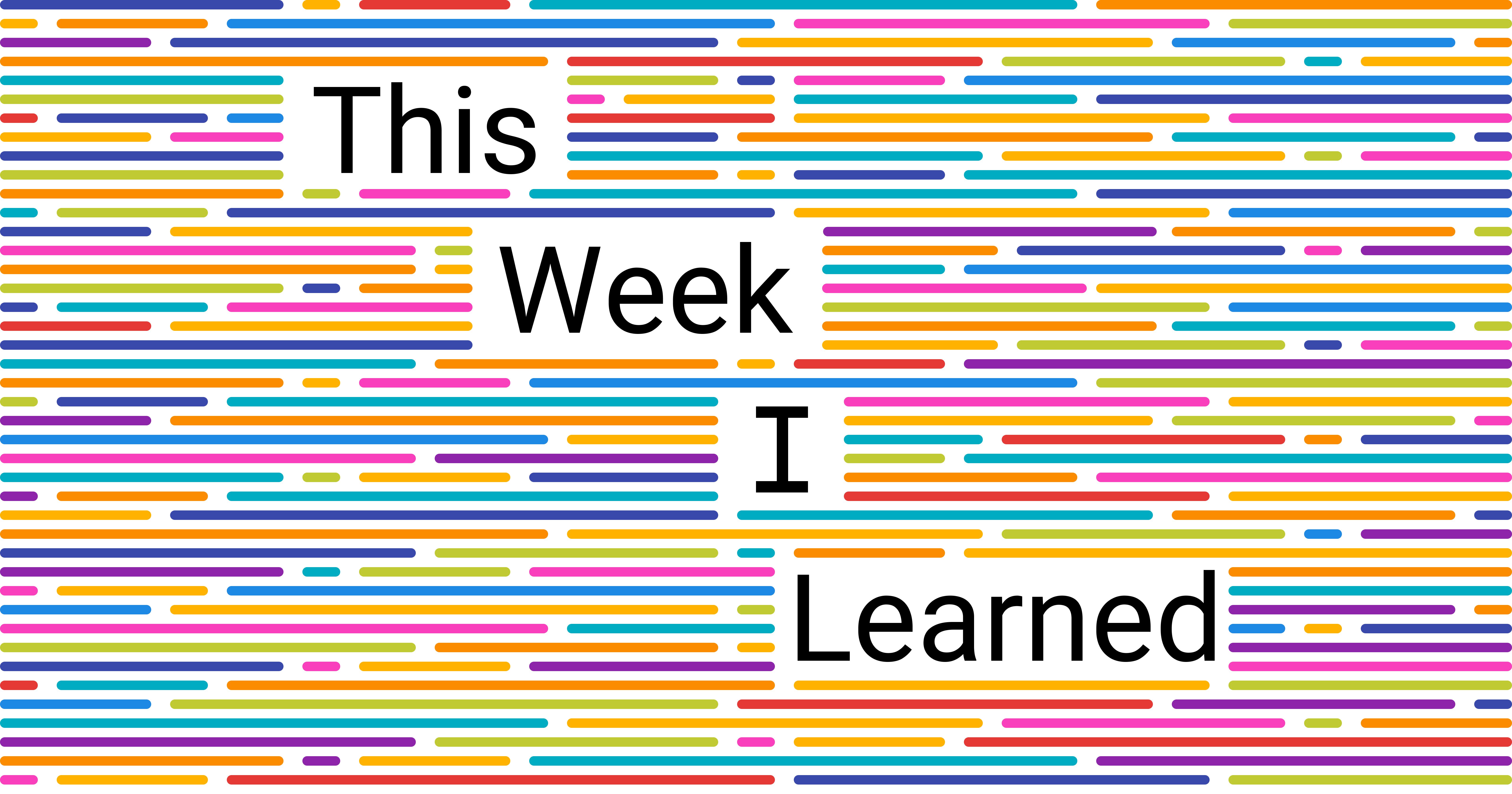
Similar to last week, this week was mainly about deploying and upgrading smart contracts. Here are a couple of things I learned:
Usage of unchecked
Looking at many Solidity smart contracts deployed on various mainnets, I noticed the use of the unchecked
keyword in several instances, especially within for loops. I looked it up on Google and read this Stack Overflow answer. In simple terms, it is a gas-efficient way of handling arithmetic operations by ignoring any possible underflow or overflow errors. In other words, if you know that underflow and overflow will never occur for an arithmetic operation, wrapping it with the unchecked
keyword will use less gas than the default checked operation. Here's a quick example:
contract SmartContract {
uint[] public intArray;
function contractMethod() external {
require(intArray.length < 5, "Length must be less than 5");
for (uint i; i < intArray.length;) {
// perform some operation...
unchecked {
i++;
}
}
}
}
OpenZeppelin's Proxy Admin Issue
While upgrading and deploying several smart contracts using Hardhat, I encountered an error: Proxy admin is not the one registered in the network manifest
. I searched online for a solution but found none, except for deleting the entire manifest file in the .openzeppelin
directory.
Instead, here's how I resolved it:
I went to Polygonscan, searched for the contract I was trying to upgrade, and retrieved the proxy admin contract's address by interacting with the website.
I then checked the
.openzeppelin > polygon.json
file and noticed that the address underadmin
was different from the one I got from Polygonscan.I replaced the address in the
polygon.json
file with the one from Polygonscan.Finally, I reran the code to upgrade the smart contract, and it worked.
So, if you encounter the same issue, find the proxy admin's address from the relevant block explorer and replace the one in the .openzeppelin > <network>.json
file with it.
Subscribe to my newsletter
Read articles from Sean Kim directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
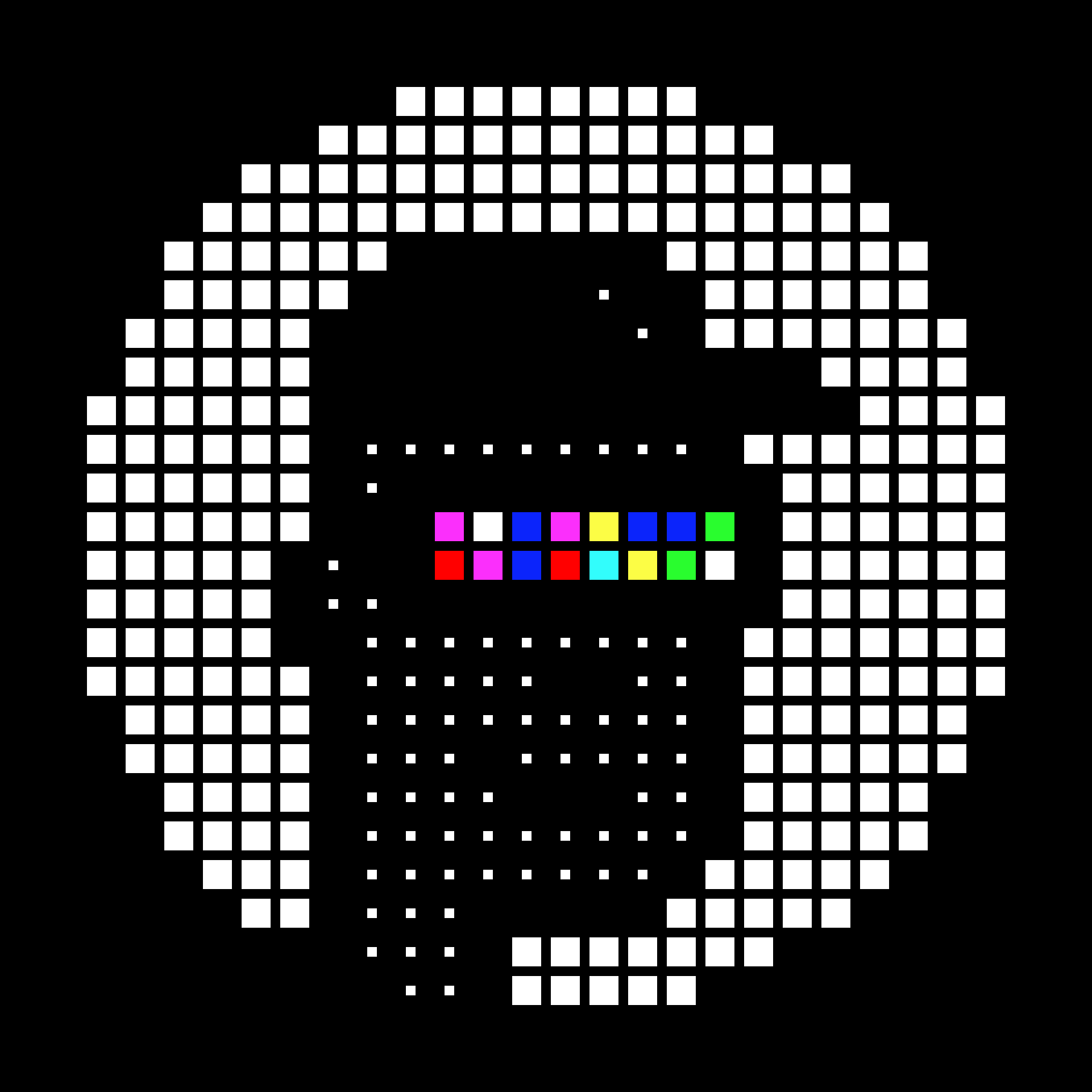
Sean Kim
Sean Kim
Engineer @ ___ | Ex-Ourkive | Ex-Meta