Array Questions - Part 1
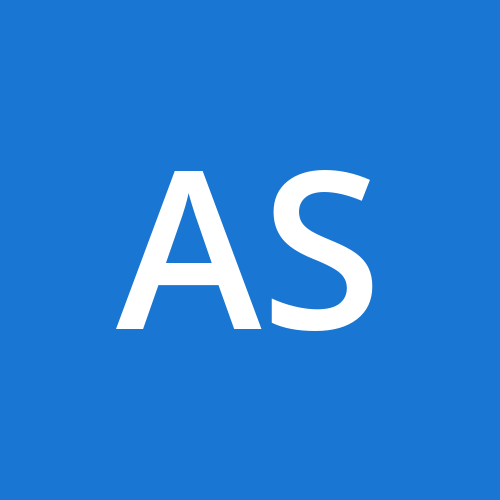
Value equal to index value
Note: There can be more than one element in the array which have the same value as its index. You need to include every such element’s index. Follows 1-based indexing of the array.
Example 1:
Input:
N = 5
Arr[] = {15, 2, 45, 12, 7}
Output: 2
Explanation: Only Arr[2] = 2 exists here.
Example 2:
Input:
N = 1
Arr[] = {1}
Output: 1
Explanation: Here Arr[1] = 1 exists.
vector<int> valueEqualToIndex(int arr[], int n) {
// code here
vector<int> v1;
for(int i=0;i<n;i++)
{
if (i+1==arr[i] )
v1.push_back(arr[i]);
}
return v1;
}
Print alternate elements of an array
Example 1:
Input:
N = 4
A[] = {1, 2, 3, 4}
Output:
1 3
void print(int ar[], int n)
{
// code here
for(int i=0;i<n;i+=2)
cout<<ar[i]<<" ";
}
Palindromic Array
Example 1:
Input:
5
111 222 333 444 555
Output:
1Explanation:
A[0] = 111 //which is a palindrome number.
A[1] = 222 //which is a palindrome number.
A[2] = 333 //which is a palindrome number.
A[3] = 444 //which is a palindrome number.
A[4] = 555 //which is a palindrome number.
As all numbers are palindrome so This will return 1.
for(int i=0 ;i<a.length ;i++)
{
int rev =0, num=a[i];
while(num > 0)
{
rev = rev * 10 + (num % 10);
num /=10;
}
if( a[i] != rev)
return 0;
}
return 1;
Count of smaller elements
Example 1:
Input:
N = 6
A[] = {1, 2, 4, 5, 8, 10}
X = 9
Output:
5
long count =0;
for(int i =0;i<n;i++)
{
if(arr[i] <= x)
count++;
}
return count;
Sum of Array
Given an integer array arr[] of size n. The task is to find sum of it.
Example 1:
Input:
n = 4
arr[] = {1, 2, 3, 4}
Output: 10
Explanation: 1 + 2 + 3 + 4 = 10.
Example 2:
Input:
n = 3
arr[] = {1, 3, 3}
Output: 7
Explanation: 1 + 3 + 3 = 7.
int sum(int arr[], int n) {
// code here
int total=0;
for(int i=0;i<n;i++)
total += arr[i];
return total;
}
Print Elements of Array
Print Elements of Array | Practice | GeeksforGeeks
Given an array arr of size n, print all its elements space-separated. Note: You don't need to move to the next line…
Given an array arr of size n, print all its elements space-separated.
Note: You don’t need to move to the next line after printing all elements of the array (space-separated)
Example 1:
Input:
n = 5
arr[] = {1, 2, 3, 4, 5}
Output: 1 2 3 4 5
Example 2:
Input:
n = 4
arr[] = {2, 3, 5, 5}
Output: 2 3 5 5
class Solution{
public:
//Just print the space seperated array elements
void printArray(int arr[], int n) {
// code here
for(int i=0;i<n;i++)
cout<<arr[i]<<" ";
}
};
Find Index
Given an unsorted array arr[] of n integers and a key which is present in this array. You need to write a program to find the start index( index where the element is first found from left in the array ) and end index( index where the element is first found from right in the array ).(0 based indexing is used)
If the key does not exist in the array then return -1 for both start and end index in this case.
Example 1:
Input:
n = 6
arr[] = { 1, 2, 3, 4, 5, 5 }
key = 5
Output: {4, 5}
Explanation:
5 appears first time at index 4 and appears last time at index 5.
(0 based indexing)
class Solution
{
public:
vector<int> findIndex(int arr[], int n, int key)
{
//code here.
vector <int>v1;
for(int i=0;i<n;i++)
{
if (arr[i] == key){
v1.push_back(i); break;
}
}
for(int i=n;i>=0;i--)
{
if (arr[i] == key){
v1.push_back(i); break;}
}
if (v1.size() == 0 )
return {-1,-1};
return v1;
}
};
Swap kth elements
Given an array arr of size n, swap the kth element from the beginning with kth element from the end.
Example 1:
Input:
n = 8
k = 3
arr[] = {1, 2, 3, 4, 5, 6, 7, 8}
Output: {1, 2, 6, 4, 5, 3, 7, 8}
Explanation:
3rd element from beginning is 3 and from end is 6.
class Solution {
public:
void swapKth(int n, int k, vector<int> &arr) {
int temp = arr[k-1];
arr[k-1] = arr[n-k];
arr[n-k] = temp;
}
};
Display longest name
Example1 :
Input:
n = 5
names[] = { "Geek", "Geeks", "Geeksfor", "GeeksforGeek", "GeeksforGeeks" }
Output: GeeksforGeeks
Explanation: name "GeeksforGeeks" has maximum length among all names.
lass Solution {
public:
string longest(int n, vector<string> &names) {
// code here
string longest = "";
int length = 0;
for(int i=0; i<n; i++){
if(names[i].size()>length){
longest = names[i];
length = names[i].size();
}
}
return longest;
}
};
C++ Array (print an element) | Set 2
Example 1:
Input:
n = 5
key = 2
arr = {10, 20, 30, 40, 50}
Output: 30
Explanation: The value of arr[2] is 30 .
class Solution {
public:
int findElementAtIndex(int n, int key, vector<int> &arr) {
// code here
return arr[key];
}
};
Perfect Arrays
Perfect Arrays | Practice | GeeksforGeeks
Given an array arr of size n and you have to tell whether the arr is perfect or not. An array is said to be perfect if…
Given an array arr of size n and you have to tell whether the arr is perfect or not. An array is said to be perfect if its reverse array matches the original array. If the arr is perfect then return True else return False.
Example 1:
Input :
n = 5
arr = {1, 2, 3, 2, 1}
Output : PERFECT
Explanation:
Here we can see we have [1, 2, 3, 2, 1] if we reverse it we can find [1, 2, 3, 2, 1]
which is the same as before.So, the answer is PERFECT.
class Solution {
public:
bool isPerfect(int n, vector<int> &arr) {
// code here
for(int i=0, j=arr.size()-1; i<j;i++,j--)
{
if (arr[i] != arr[j])
return false;
}
return true;
}
};
Smaller and Larger
Example 1:
Input:
N = 7, X = 0
Arr[] = {1, 2, 8, 10, 11, 12, 19}
Output: 0 7
Explanation: There are no elements less or
equal to 0 and 7 elements greater or equal
to 0.
//User function template for C++
class Solution{
public:
vector<int> getMoreAndLess(int arr[], int n, int x) {
int c1=0, c2=0;
for(int i=0;i<n;i++)
if (x >=arr[i])
c1++;
for(int i=n-1;i>=0;i--)
if (x <=arr[i])
c2++;
return {c1,c2};
}
};
Subscribe to my newsletter
Read articles from Akshima Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
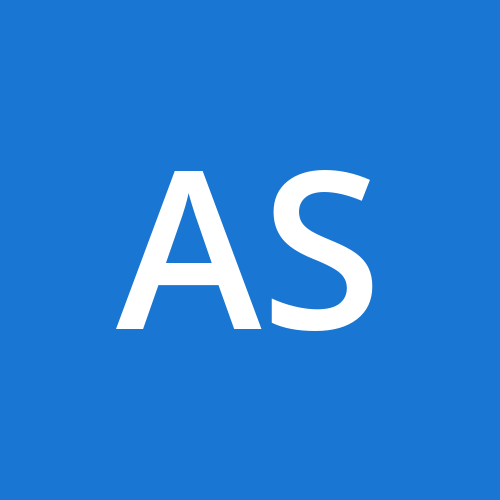