Advanced Linux Shell Scripting: Managing System Logs, Processes, Networking, and Security
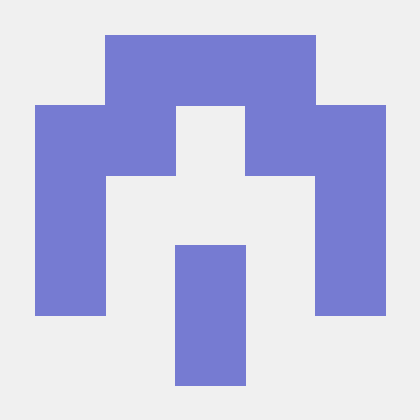
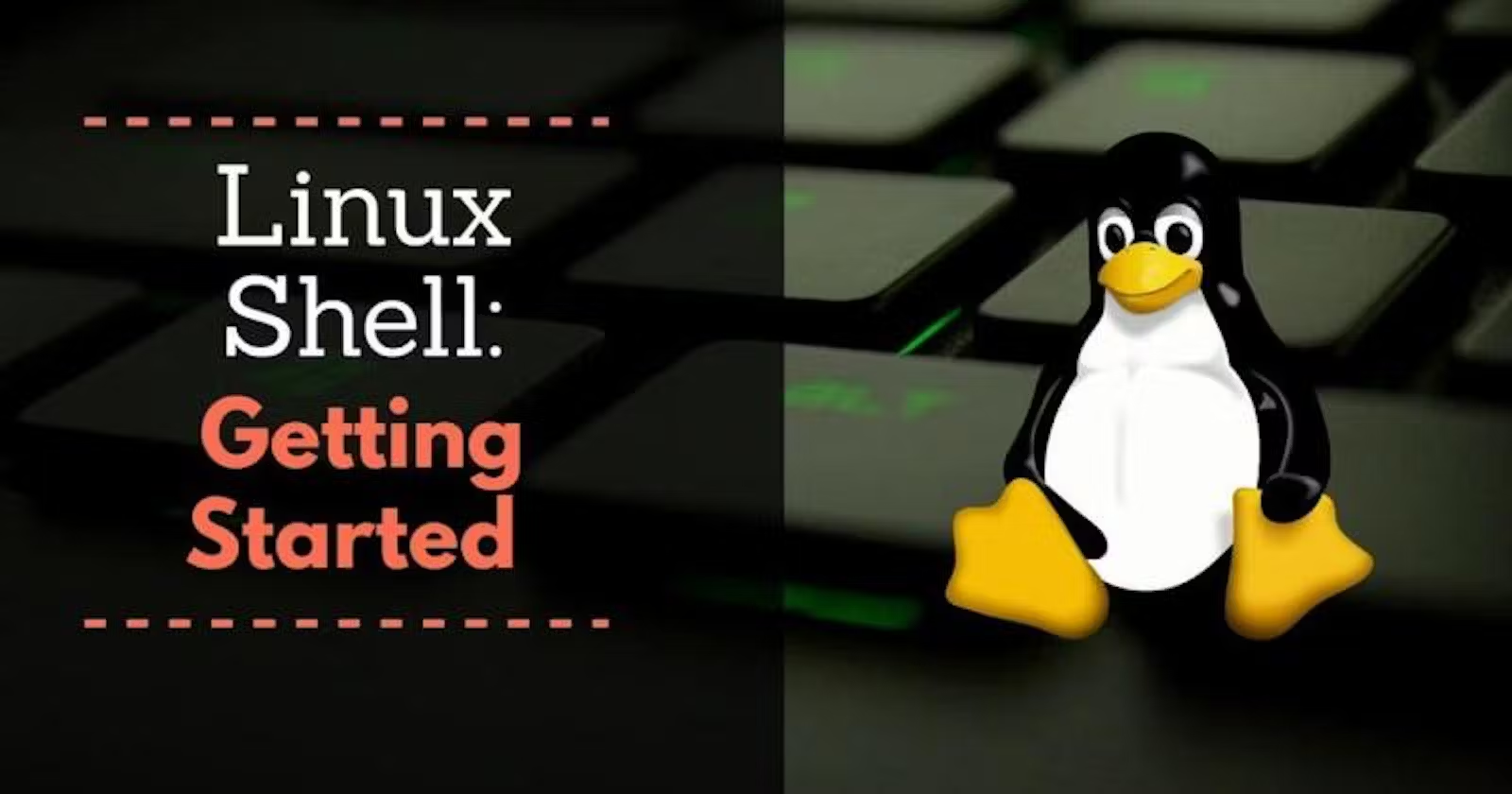
Introduction
Linux shell scripting is not just limited to basic automation; it also plays a crucial role in advanced system administration tasks such as managing system logs, processes, networking, and security. This article explores advanced shell scripting techniques to handle these tasks efficiently, enabling system administrators to maintain healthy and optimized systems.
Managing System Logs
System logs are vital for monitoring and troubleshooting system activities. Shell scripts can automate the process of managing logs, including log rotation, archiving, and analyzing log files.
Log Rotation
Log rotation involves rotating out old logs and compressing or deleting them to prevent disk space issues.
Example Script: Log Rotation
#!/bin/bash
# Define log directory and rotation parameters
log_dir="/var/log/myapp"
archive_dir="/var/log/myapp/archive"
days_to_keep=7
# Create archive directory if it doesn't exist
mkdir -p $archive_dir
# Find and move old logs to the archive directory
find $log_dir -type f -name "*.log" -mtime +$days_to_keep -exec mv {} $archive_dir \;
# Compress archived logs
gzip $archive_dir/*.log
# Remove logs older than a certain number of days
find $archive_dir -type f -name "*.gz" -mtime +$days_to_keep -exec rm {} \;
echo "Log rotation completed."
Log Analysis
Analyzing logs helps identify patterns and troubleshoot issues. Shell scripts can parse logs to extract meaningful information.
Example Script: Log Analysis
#!/bin/bash
# Define log file and search parameters
log_file="/var/log/syslog"
search_term="ERROR"
# Count the number of occurrences of the search term
error_count=$(grep -c $search_term $log_file)
# Print the results
echo "The term '$search_term' occurred $error_count times in $log_file."
# Extract and display the lines containing the search term
grep $search_term $log_file
Managing Processes
Process management is another critical task for system administrators. Shell scripts can automate the monitoring and control of system processes.
Monitoring Processes
Monitoring processes involves checking their status, resource usage, and taking action if necessary.
Example Script: Process Monitoring
#!/bin/bash
# Define the process name to monitor
process_name="apache2"
# Check if the process is running
if pgrep $process_name > /dev/null; then
echo "$process_name is running."
else
echo "$process_name is not running. Starting the process..."
sudo systemctl start $process_name
fi
Resource Usage
Monitoring the resource usage of processes helps ensure that the system is not overburdened.
Example Script: Resource Usage Monitoring
#!/bin/bash
# Define the process name to monitor
process_name="mysqld"
# Get CPU and memory usage of the process
cpu_usage=$(ps -C $process_name -o %cpu=)
mem_usage=$(ps -C $process_name -o %mem=)
# Print the resource usage
echo "$process_name CPU usage: $cpu_usage%"
echo "$process_name Memory usage: $mem_usage%"
# Check if resource usage exceeds a threshold and take action
cpu_threshold=80.0
mem_threshold=50.0
if (( $(echo "$cpu_usage > $cpu_threshold" | bc -l) )); then
echo "CPU usage of $process_name is above threshold. Taking action..."
# Add actions to be taken (e.g., restart the process, alert admin)
fi
if (( $(echo "$mem_usage > $mem_threshold" | bc -l) )); then
echo "Memory usage of $process_name is above threshold. Taking action..."
# Add actions to be taken (e.g., restart the process, alert admin)
fi
Networking
Networking is an essential aspect of system administration, involving tasks such as monitoring network status, managing firewall rules, and configuring network interfaces.
Network Monitoring
Shell scripts can automate the monitoring of network interfaces and traffic to ensure optimal performance and detect potential issues.
Example Script: Network Monitoring
#!/bin/bash
# Define network interface to monitor
interface="eth0"
# Get network statistics
rx_bytes=$(cat /sys/class/net/$interface/statistics/rx_bytes)
tx_bytes=$(cat /sys/class/net/$interface/statistics/tx_bytes)
# Print network statistics
echo "Network interface: $interface"
echo "Received bytes: $rx_bytes"
echo "Transmitted bytes: $tx_bytes"
# Monitor network traffic over a period of time
sleep 5
rx_bytes_new=$(cat /sys/class/net/$interface/statistics/rx_bytes)
tx_bytes_new=$(cat /sys/class/net/$interface/statistics/tx_bytes)
echo "New received bytes: $((rx_bytes_new - rx_bytes))"
echo "New transmitted bytes: $((tx_bytes_new - tx_bytes))"
Firewall Management
Managing firewall rules is crucial for maintaining system security. Shell scripts can simplify the process of adding, removing, and listing firewall rules.
Example Script: Firewall Management
#!/bin/bash
# Define firewall rule parameters
port=8080
action="allow"
# Add a firewall rule
sudo ufw $action $port/tcp
# Enable the firewall if not already enabled
sudo ufw enable
# List current firewall rules
sudo ufw status verbose
echo "Firewall rule added: $action $port/tcp"
Security
Security is a top priority for system administrators. Shell scripts can help automate security tasks such as user management, file permissions, and system updates.
User Management
Automating user management tasks ensures consistency and reduces the risk of human error.
Example Script: User Management
#!/bin/bash
# Define user parameters
username="newuser"
password="password123"
# Create a new user
sudo useradd -m $username
# Set user password
echo "$username:$password" | sudo chpasswd
# Add user to a group
sudo usermod -aG sudo $username
echo "User $username created and added to sudo group."
File Permissions
Managing file permissions ensures that only authorized users have access to sensitive data.
Example Script: File Permissions
#!/bin/bash
# Define file and permission parameters
file_path="/home/newuser/important_file.txt"
user="newuser"
permissions="600"
# Set file ownership
sudo chown $user:$user $file_path
# Set file permissions
sudo chmod $permissions $file_path
echo "File permissions for $file_path set to $permissions."
System Updates
Regular system updates are crucial for security and stability. Automating this process ensures that the system remains up-to-date.
Example Script: System Updates
#!/bin/bash
# Update the package list
sudo apt update
# Upgrade all installed packages
sudo apt upgrade -y
# Clean up unnecessary packages
sudo apt autoremove -y
echo "System update completed."
Advanced Techniques
Using Cron Jobs:
- Automate the execution of scripts at scheduled intervals using
cron
.
- Automate the execution of scripts at scheduled intervals using
# Open the crontab editor
crontab -e
# Add a job to run the script every day at midnight
0 0 * * * /path/to/your/script.sh
Using
awk
for Advanced Text Processing:awk
is a powerful tool for pattern scanning and processing.
# Example: Summarize disk usage by directory
du -sh * | awk '{print $2 ": " $1}'
Using
sed
for Stream Editing:sed
is a stream editor for filtering and transforming text.
# Example: Replace all instances of 'foo' with 'bar' in a file
sed -i 's/foo/bar/g' filename.txt
Error Handling and Debugging:
- Use set commands to handle errors and debug scripts.
# Exit script on any error
set -e
# Print each command before executing it
set -x
Conclusion
Advanced shell scripting is a crucial skill for managing and automating system administration tasks on Linux. From managing logs and processes to networking and security, shell scripting enhances efficiency and reliability. By mastering these advanced techniques, system administrators can ensure their systems run smoothly and respond quickly to issues.
Continued learning and practice are key to becoming proficient in shell scripting. Explore more advanced concepts and integrate these scripts into your daily workflow to maximize their benefits.
For further reading and resources, consider books like "The Linux Command Line" by William Shotts or online tutorials and forums dedicated to Linux system administration and shell scripting.
Subscribe to my newsletter
Read articles from Vikram directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
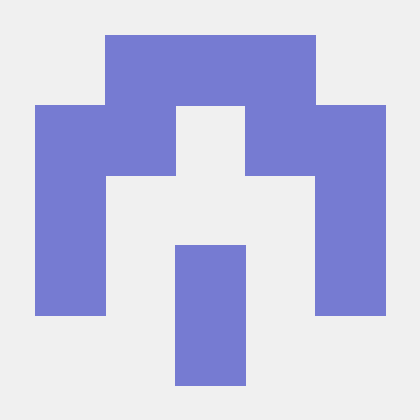