Understanding the Inner Workings of Python

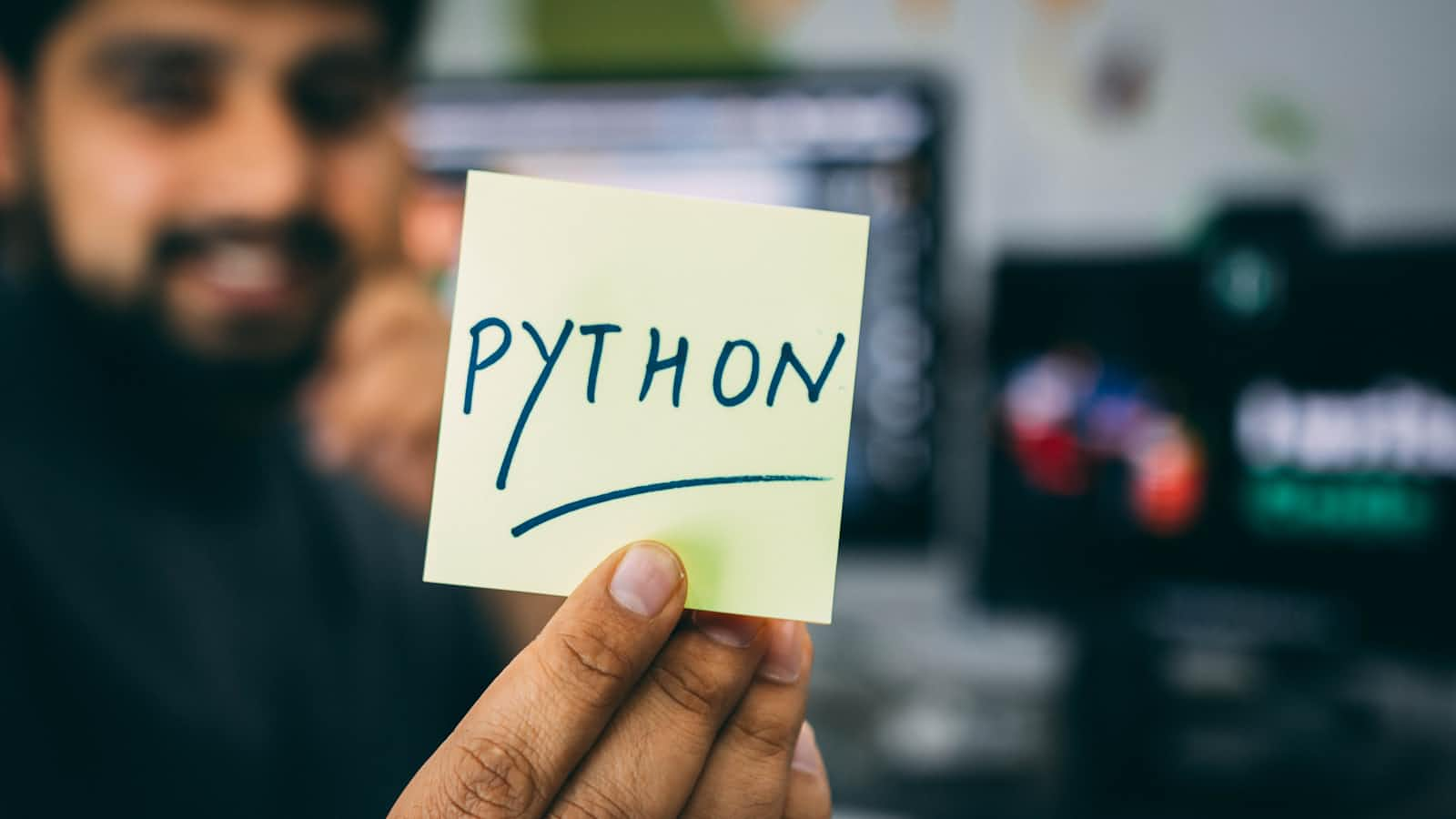
Python, a high-level programming language known for its readability and simplicity, has become a cornerstone in fields ranging from web development to data science and artificial intelligence. While Python’s external simplicity is one of its greatest strengths, the internal mechanisms that power Python are equally fascinating. This article delves into how Python works internally, providing a glimpse into the engine that drives this versatile language.
The Python Interpreter
At the heart of Python’s functionality is the Python interpreter. Unlike compiled languages such as C or Java, Python is an interpreted language, meaning that the source code is executed line by line, rather than being compiled into machine code beforehand. Python’s standard implementation is CPython, written in C, which makes it the most widely used Python interpreter.
Bytecode Compilation
When you execute a Python script, the interpreter first compiles the source code (.py files) into an intermediate format known as bytecode. This compilation step is implicit and happens behind the scenes. The bytecode is a low-level set of instructions that is portable and can be executed on any machine with a compatible Python interpreter. The bytecode is stored in .pyc files within the __pycache__
directory to speed up subsequent executions.
The Role of the Python Virtual Machine (PVM)
The compiled bytecode is then executed by the Python Virtual Machine (PVM). The PVM is a stack-based virtual machine that interprets the bytecode instructions one at a time. Each instruction is processed by the PVM's main loop, which handles operations such as arithmetic calculations, variable management, function calls, and control flow.
Garbage Collection and Memory Management
Python handles memory management automatically through a process known as garbage collection. Python uses a reference counting mechanism as its primary garbage collection method. Each object in Python maintains a reference count, which keeps track of the number of references pointing to it. When the reference count drops to zero, the memory occupied by the object is deallocated.
Additionally, Python employs a cyclic garbage collector to detect and collect reference cycles—situations where a group of objects reference each other, preventing their reference counts from ever reaching zero. The cyclic garbage collector periodically searches for and eliminates these cycles, ensuring efficient memory usage.
Data Structures and Memory Allocation
Python’s built-in data structures, such as lists, dictionaries, and sets, are implemented in C for efficiency. For example, lists are dynamic arrays that can grow and shrink as needed, while dictionaries are implemented as hash tables for fast lookup operations. These data structures manage memory allocation through internal strategies that minimize overhead and fragmentation.
Dynamic Typing and Objects
Python is a dynamically typed language, meaning that variable types are determined at runtime rather than at compile time. Every variable in Python is a reference to an object, and these objects carry type information with them. This dynamic typing allows for greater flexibility but requires a robust and efficient object model.
Method Resolution Order (MRO)
Python supports multiple inheritance, allowing a class to inherit attributes and methods from multiple parent classes. The Method Resolution Order (MRO) is a mechanism that Python uses to determine the order in which base classes are searched when executing a method. Python uses the C3 linearization algorithm to compute the MRO, ensuring a consistent and predictable method resolution order.
Just-in-Time Compilation: PyPy
While CPython remains the standard Python interpreter, alternative implementations like PyPy offer significant performance improvements through Just-in-Time (JIT) compilation. PyPy translates Python code into machine code at runtime, allowing for optimizations that can dramatically speed up execution. PyPy’s JIT compiler analyzes the running program, identifies hotspots, and compiles these parts into machine code for faster execution.
The Standard Library and Beyond
Python’s extensive standard library is one of its most powerful features, providing modules and packages for everything from file I/O and networking to data manipulation and mathematical operations. This library is written in a mix of Python and C, enabling both ease of use and performance.
Extending Python with C/C++
For performance-critical applications, Python allows integration with C/C++ code through extensions and API interfaces like the Python C API. This capability enables developers to write performance-intensive parts of their application in C/C++ while leveraging Python’s simplicity for higher-level operations.
Conclusion
Understanding the internal workings of Python reveals the complexity and sophistication underlying its deceptively simple syntax. From bytecode compilation and the PVM to dynamic typing and garbage collection, Python’s design balances ease of use with powerful features and performance. Whether you are a beginner or an experienced developer, appreciating these internal mechanisms can enhance your ability to write efficient, effective Python code and deepen your understanding of this remarkable programming language.
Subscribe to my newsletter
Read articles from Utkarsh Roy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
