TypeSafe Navigation or Traditional Intent Passing?
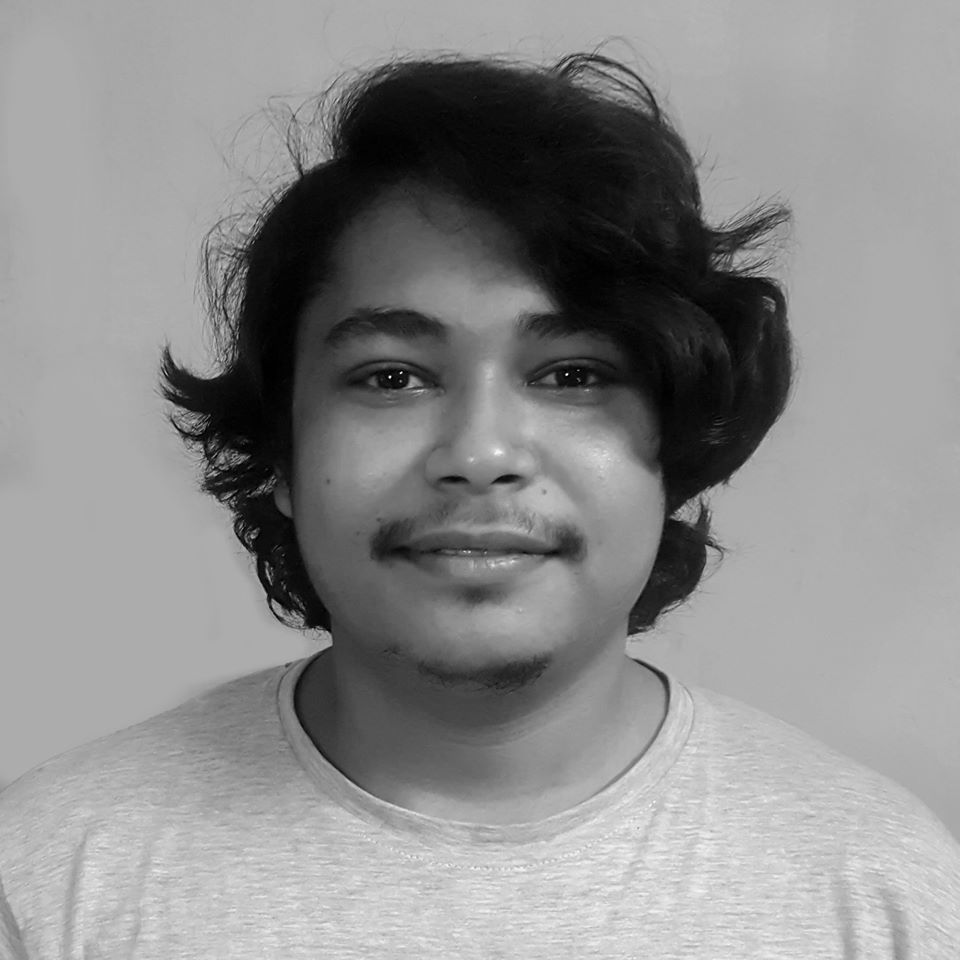
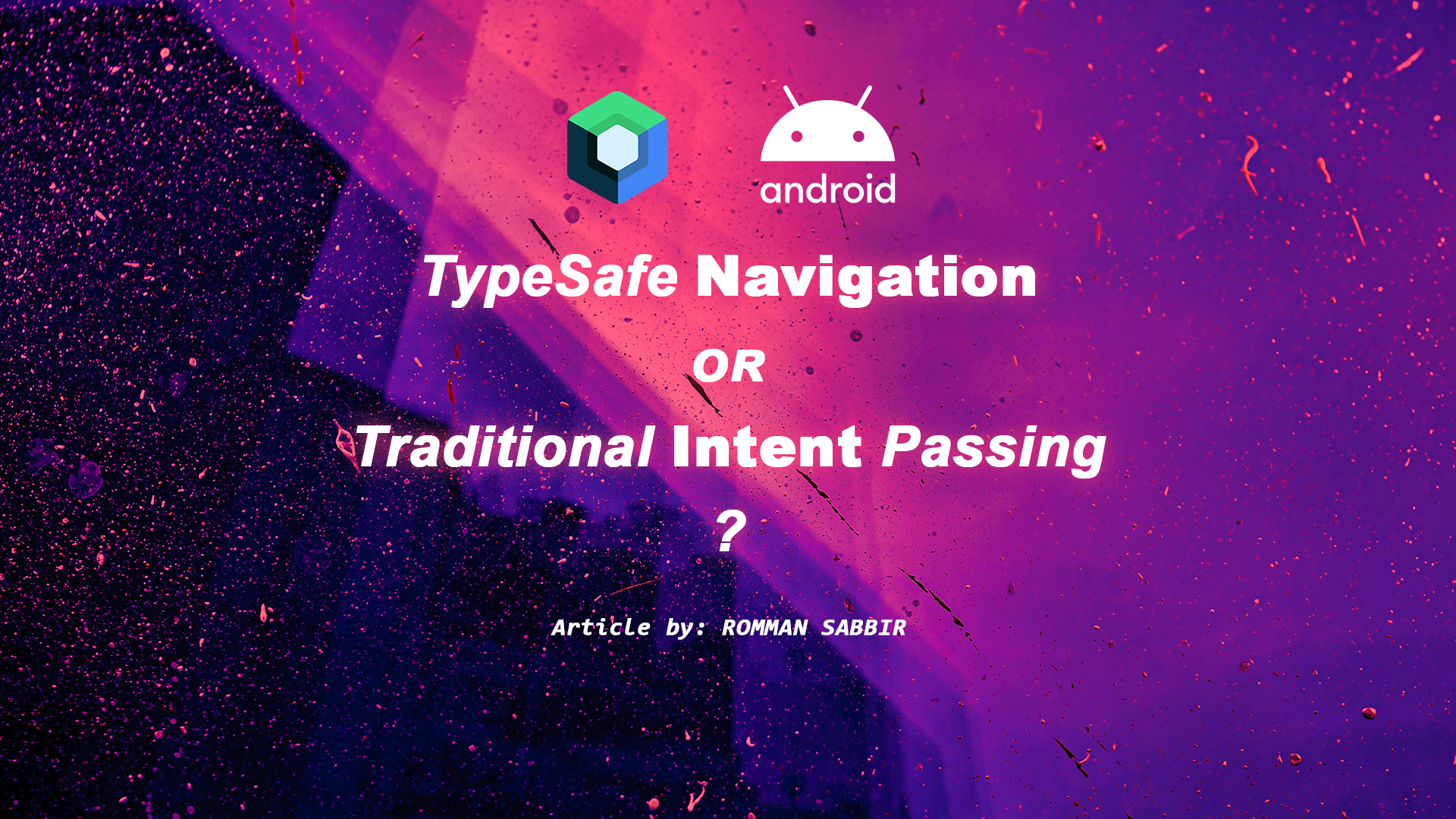
Introduction
Navigating between screens is a fundamental aspect of mobile app development. In the Android ecosystem, there are two primary methods for handling navigation: the traditional intent passing and the modern TypeSafe navigation in Jetpack Compose.
This article we will compare these two methods, highlighting their advantages and disadvantages, and providing Kotlin code examples to illustrate their usage.
TypeSafe Navigation in Jetpack Compose
Jetpack Compose introduces a TypeSafe navigation mechanism that integrates seamlessly with its declarative UI framework. This approach offers several benefits:
Pros:
Type Safety: Ensures compile-time checks for argument types, reducing runtime errors.
Ease of Use: Simplifies navigation logic with clear and concise APIs.
Consistency: Provides a uniform approach to navigation across the app.
Deep Integration with Compose: Efficiently manages UI state and navigation within Compose.
Scoping and Lifecycle: Better handles composable lifecycles and state management.
Argument Passing: Safely and easily passes arguments between composables.
Cons:
Learning Curve: Requires learning new APIs and concepts.
Limited Documentation and Resources: As a newer framework, it may have less community support.
Compatibility: Limited to projects using Jetpack Compose.
Example in Kotlin:
First, add the necessary dependencies to our build.gradle
file:
implementation "androidx.navigation:navigation-compose:2.4.0-alpha10"
Define your navigation routes and arguments:
sealed class Screen(val route: String) {
object Home : Screen("home")
object Details : Screen("details/{itemId}") {
fun createRoute(itemId: String) = "details/$itemId"
}
}
Set up the NavHost in our composable:
@Composable
fun AppNavHost(navController: NavHostController) {
NavHost(navController, startDestination = Screen.Home.route) {
composable(Screen.Home.route) {
HomeScreen(navController)
}
composable(Screen.Details.route) { backStackEntry ->
val itemId = backStackEntry.arguments?.getString("itemId")
DetailsScreen(navController, itemId)
}
}
}
Navigate between screens:
@Composable
fun HomeScreen(navController: NavController) {
Button(onClick = { navController.navigate(Screen.Details.createRoute("123")) }) {
Text("Go to Details")
}
}
@Composable
fun DetailsScreen(navController: NavController, itemId: String?) {
Text("Details for item: $itemId")
}
Traditional Intent Passing
The traditional method for navigation in Android involves using intents. This method, while familiar and flexible, comes with its own set of challenges:
Pros:
Familiarity: Well-known method with extensive documentation and community support.
Flexibility: Usable in any Android project, regardless of the UI framework.
Integration: Easily integrates with other components and libraries.
Cons:
Type Safety: Lacks compile-time type checks, leading to potential runtime errors.
Boilerplate Code: Requires more boilerplate for passing data and handling navigation.
Verbosity: Can become verbose and harder to manage with complex navigation flows.
Lifecycle Management: Manual handling of lifecycles and state management can be error-prone.
Example in Kotlin:
Define the intent and pass arguments:
// In HomeActivity
val intent = Intent(this, DetailsActivity::class.java).apply {
putExtra("itemId", "123")
}
startActivity(intent)
Retrieve arguments in the target activity:
// In DetailsActivity
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_details)
val itemId = intent.getStringExtra("itemId")
findViewById<TextView>(R.id.detailsTextView).text = "Details for item: $itemId"
}
Comparison
Type Safety: Jetpack Compose's TypeSafe navigation provides compile-time checks, reducing errors and improving reliability. Traditional intents rely on runtime checks, which can lead to crashes if not handled properly.
Ease of Use: Compose simplifies navigation with a declarative approach, whereas traditional intents can be more verbose and cumbersome.
Readability and Maintainability: Compose's navigation is generally more readable and maintainable, especially for complex apps, due to less boilerplate and more structured navigation code.
Integration: Compose is better integrated with modern Android architecture components, providing a more cohesive development experience.
Conclusion
TypeSafe navigation in Jetpack Compose is generally better for modern Android development due to its type safety, ease of use, and better integration with Compose. It is especially advantageous for new projects or those looking to leverage the benefits of Jetpack Compose. However, for projects deeply rooted in traditional Android development or those requiring extensive use of XML-based layouts, traditional intent passing might still be more practical due to its flexibility and familiarity.
Both methods have their place, and the choice between them should be guided by the specific needs and constraints of your project.
That's it for today. Happy Coding...
Subscribe to my newsletter
Read articles from Romman Sabbir directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
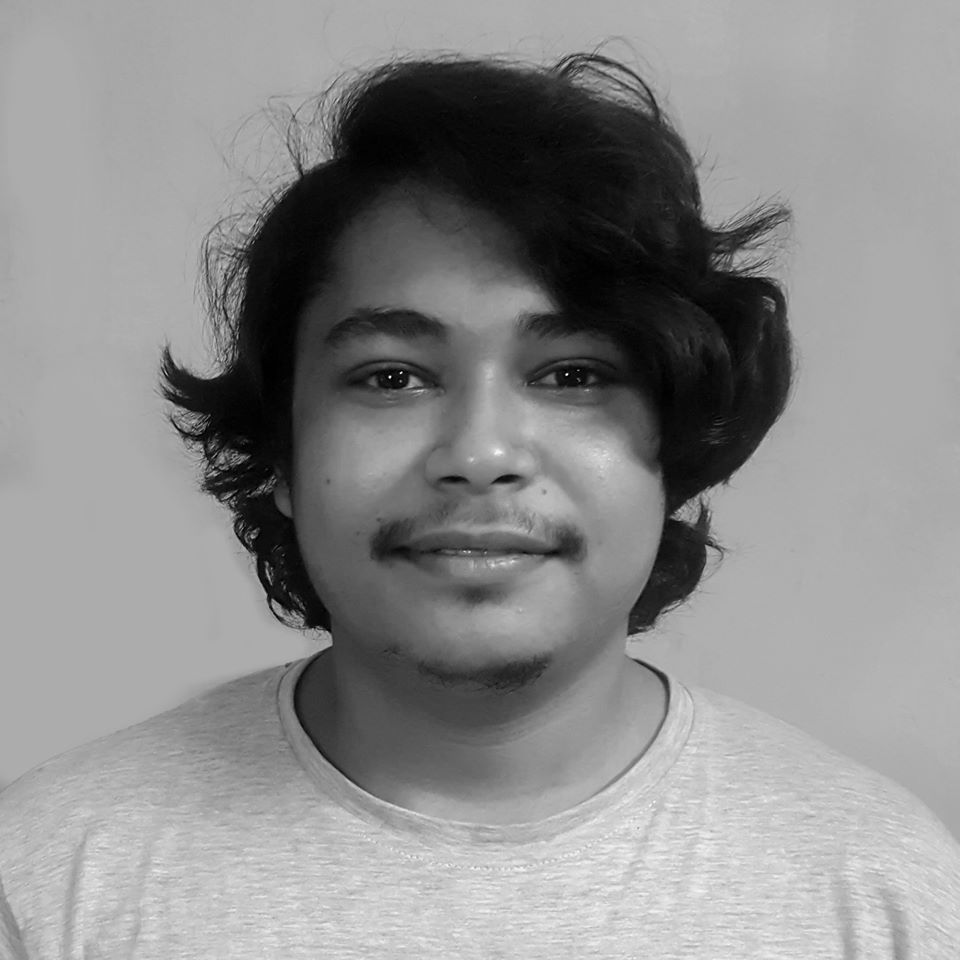
Romman Sabbir
Romman Sabbir
Senior Android Engineer from Bangladesh. Love to contribute in Open-Source. Indie Music Producer.