Exploring the Mark and Sweep Method in Garbage Collection

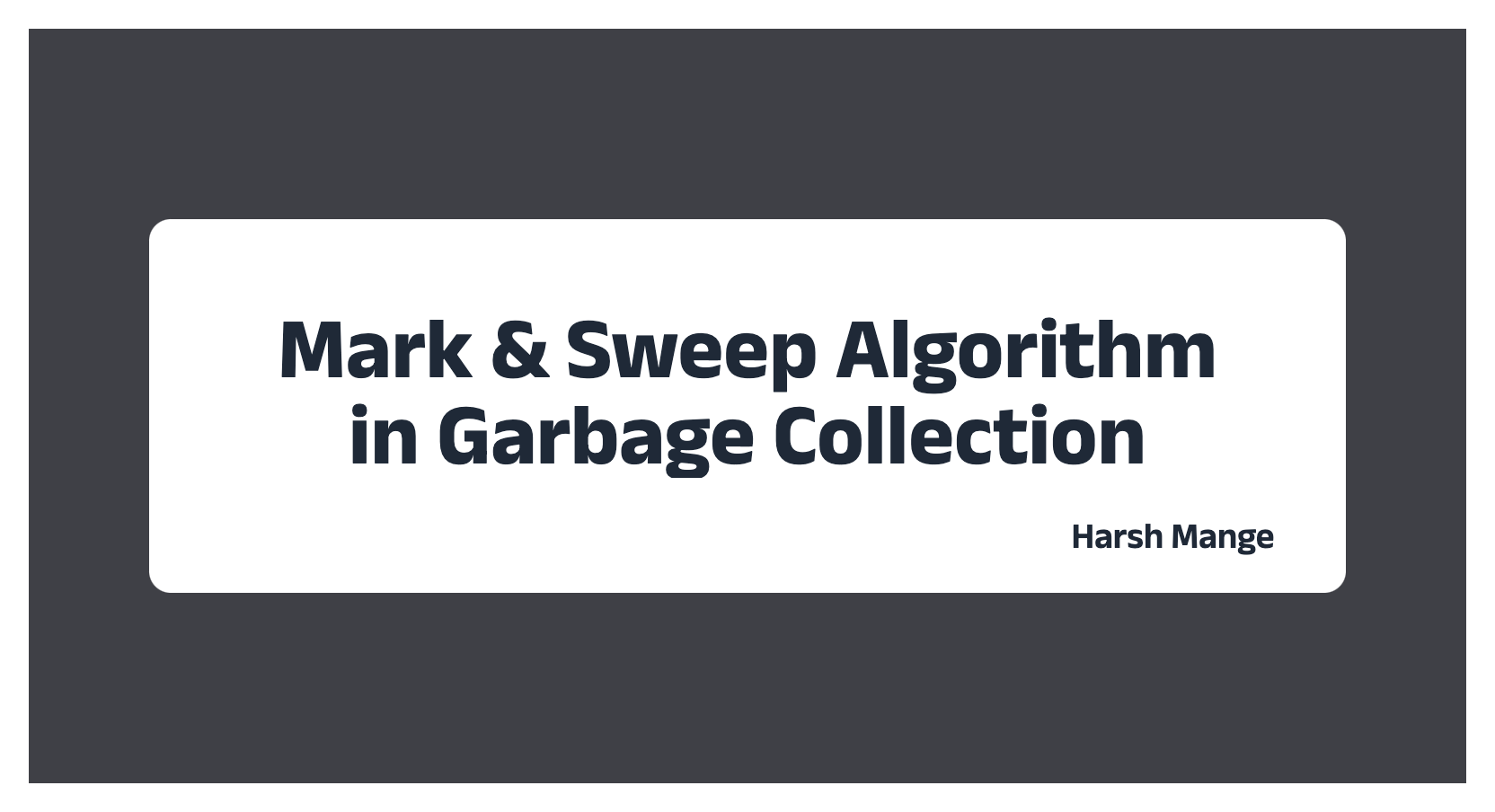
Garbage collection (GC) is an essential feature in many modern programming languages, ensuring efficient memory management by automatically reclaiming memory allocated to objects no longer in use. One of the most foundational and widely used garbage collection algorithms is the Mark and Sweep method. This blog will delve into its internal workings, use cases, and implementation in modern frameworks.
Understanding Garbage Collection
Before we explore the Mark and Sweep method, let's briefly understand the role of garbage collection. In managed languages like Java, C#, and Python, garbage collection automates the process of freeing up memory that is no longer needed by the program. This helps prevent memory leaks and other related issues, contributing to more robust and maintainable software.
Mark & Sweep Algorithm
The mark-and-sweep algorithm is a garbage-collection technique used in memory management. It involves two main steps: marking and sweeping. The basic idea behind this algorithm is to identify all the objects that are still in use by the program and reclaim the memory occupied by the objects that are no longer needed.
Internal Working of the Mark and Sweep Method
Let's break down the internal workings of the Mark and Sweep method step-by-step:
Initialization
- The garbage collector initializes by setting all object markers to "unmarked."
Root Identification
- It identifies the root set, which includes objects directly accessible by the program (global variables, stack variables, registers).
Mark Phase
Starting from the root set, the collector recursively traverses each referenced object.
Each visited object is marked as "reachable."
This traversal continues until all reachable objects from the root set are marked.
Sweep Phase
The collector scans the entire heap.
Objects that are not marked as reachable are considered garbage and are reclaimed.
The reclaimed memory is added back to the free list, making it available for future allocations.
Example
Suppose we have a program that creates the following objects:
var obj1 = {name: "John", age: 30};
var obj2 = {name: "Mary", age: 25};
var obj3 = {name: "Tom", age: 35};
var obj4 = {name: "Jane", age: 28};
These objects are initially allocated in memory and referenced by variables obj1
, obj2
, obj3
, and obj4
. At this point, the program is using all these objects and they should not be garbage collected.
Now, suppose the program executes the following code:
obj1 = null;
obj3 = null;
These lines of code remove the references to obj1
and obj3
, which means that these objects are no longer being used by the program and can be garbage collected.
To reclaim the memory occupied by these objects, the mark-and-sweep algorithm performs the following steps:
Marking: The algorithm first marks all the objects that are still in use by the program. It starts from the root objects, which are the objects that are directly referenced by the program, and recursively traverses the object graph to mark all the objects that are reachable from the root objects. In our example, the root objects are
obj2
andobj4
, since they are the only objects that are still referenced by the program. The algorithm marks these objects as live.Sweeping: The algorithm then sweeps through the entire memory heap and frees the memory occupied by all the unmarked objects, which are the objects that are no longer in use by the program. In our example, the algorithm identifies
obj1
andobj3
as unmarked objects and frees the memory occupied by them.
After the garbage collection process is complete, the program is left with the following objects:
obj2 = {name: "Mary", age: 25};
obj4 = {name: "Jane", age: 28};
The mark-and-sweep algorithm is an effective way to manage memory in a program, as it ensures that the memory occupied by unused objects is freed up, which can help prevent memory leaks and improve the overall performance of the program.
Subscribe to my newsletter
Read articles from Harsh Mange directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Harsh Mange
Harsh Mange
This is Harsh Mange, working as a Software Engineer - Backend at Argoid. I love building apps. Working on contributing to open-source projects and the dev community.