JavaScript Essentials: Understanding Scope, Lexical Environment, and Hoisting

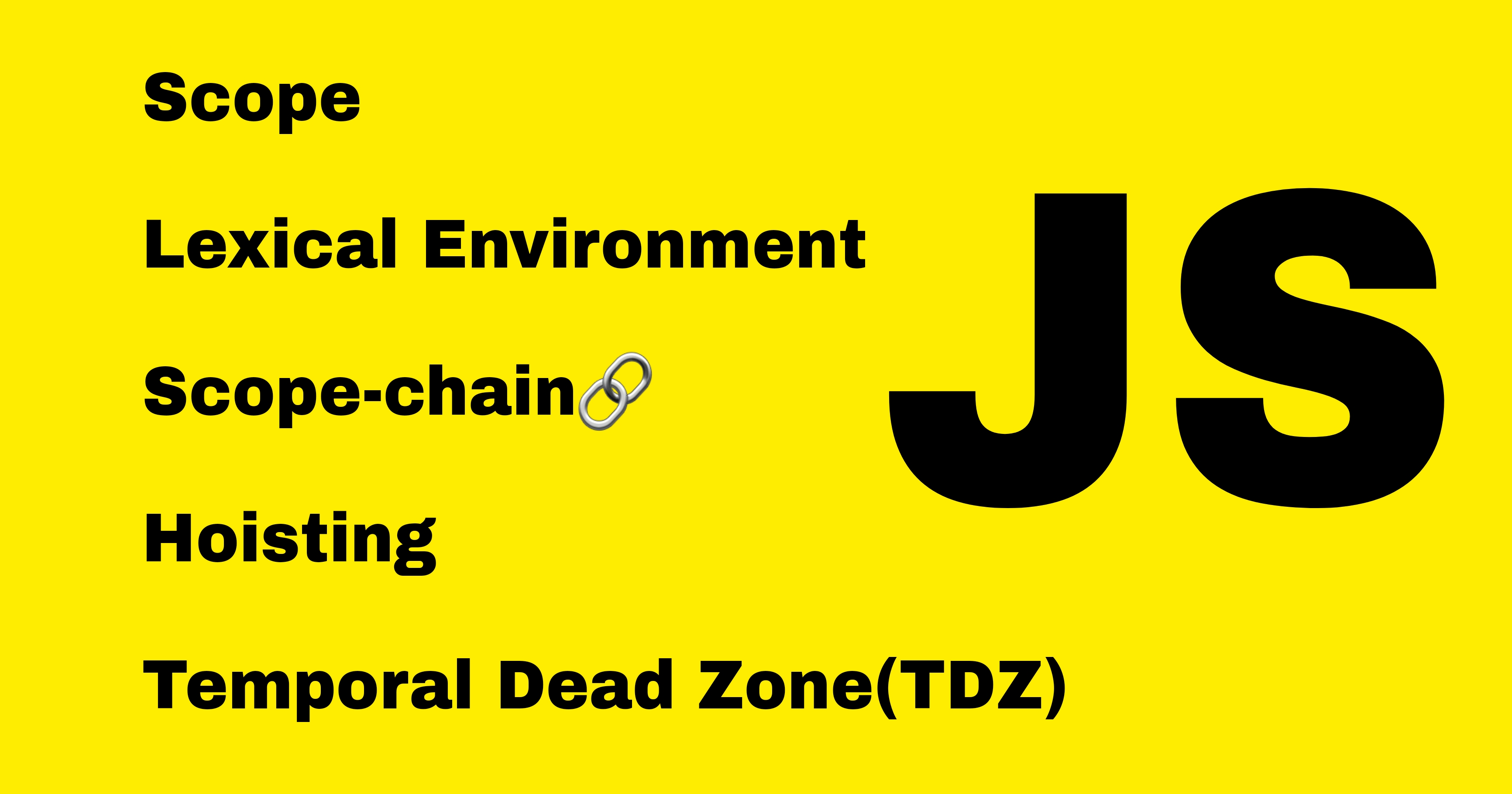
Unveiling JavaScript Scope, Lexical Environment, and Hoisting: Demystifying Key Concepts
Scope: Where Variables and Functions Live
Scope determines where you can access a specific variable or function in your code. It is directly dependent on the lexical environment.
Types of Scope
Global Scope:
Variables declared outside any function are in the global scope.
Accessible from anywhere in the code.
var globalVar = "I'm global";
function foo() {
console.log(globalVar); // Accessible here
}
foo();
console.log(globalVar); // Accessible here too
Local/Function Scope:
Variables declared within a function are in the local scope.
Accessible only within that function.
function bar() {
var localVar = "I'm local";
console.log(localVar); // Accessible here
}
bar();
console.log(localVar); // ReferenceError: localVar is not defined
Block Scope:
Variables declared with
let
orconst
within a block ({}
) are in the block scope.Accessible only within that block.
if (true) {
let blockVar = "I'm block scoped";
console.log(blockVar); // Accessible here
}
console.log(blockVar); // ReferenceError: blockVar is not defined
What is a Lexical Environment?
A lexical environment is created whenever an execution context is established. It includes the local memory and the lexical environment of its lexical parent. Lexical here means the order or hierarchy in which code is written. For instance:
function a() {
c();
function c() {
console.log(b);
}
}
var b = 10;
a();
In this example:
c()
is lexically insidea()
, meaning it is physically within thea()
function.a()
is lexically inside the global scope.
Call Stack and Lexical Environment
Let's create a call stack for the above code:
Global Execution Context: Created first, with
b
being assigned a value of10
.Function
a()
Execution Context: Created whena()
is called.Function
c()
Execution Context: Created whenc()
is called withina()
.
Each execution context has its lexical environment, which contains its local variables and a reference to its lexical parent's environment.
Scope Chain
The scope chain is the chain of all lexical environments and their parent references. This allows functions to access variables from their lexical parents.
Hoisting: var
, let
, and const
var
Variables declared with var
are hoisted to the top of their scope and are attached to the global object if declared globally.
let
and const
Variables declared with let
and const
are also hoisted but to a separate memory space than the global object. They are not accessible until they have been initialized, resulting in a concept called the Temporal Dead Zone (TDZ).
Temporal Dead Zone (TDZ)
The TDZ is the time between the hoisting of a let
or const
variable and its initialization. During this time, accessing the variable will result in a Reference Error.
Example:
console.log(a); // Uncaught ReferenceError: Cannot access 'a' before initialization
let a = 5;
In this example, a
is in the TDZ until it is initialized with the value 5
.
Understanding these concepts is crucial for writing predictable and bug-free JavaScript code. It helps you grasp how variables are stored, accessed, and manipulated in different scopes and contexts within your program.
Subscribe to my newsletter
Read articles from Bhavesh Jadhav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Bhavesh Jadhav
Bhavesh Jadhav
I am a passionate web developer from India, currently pursuing an MCA from Government College of Engineering, Aurangabad. With a strong foundation in HTML, CSS, JavaScript, and expertise in frameworks like React and Node.js, I create dynamic and responsive web applications. My skill set extends to backend development with PHP, MySQL, and MongoDB. I also have experience with AJAX, jQuery, and I am proficient in Python and Java. As an AI enthusiast, I enjoy leveraging AI tools and LLMs to enhance my projects. Eager to expand my horizons, I am delving into DevOps to optimize development workflows and improve deployment processes. Always keen to learn and adapt, I strive to integrate cutting-edge technologies into my work. Let's connect and explore the world of technology together!