Python’s Built In Modules: The Math Module

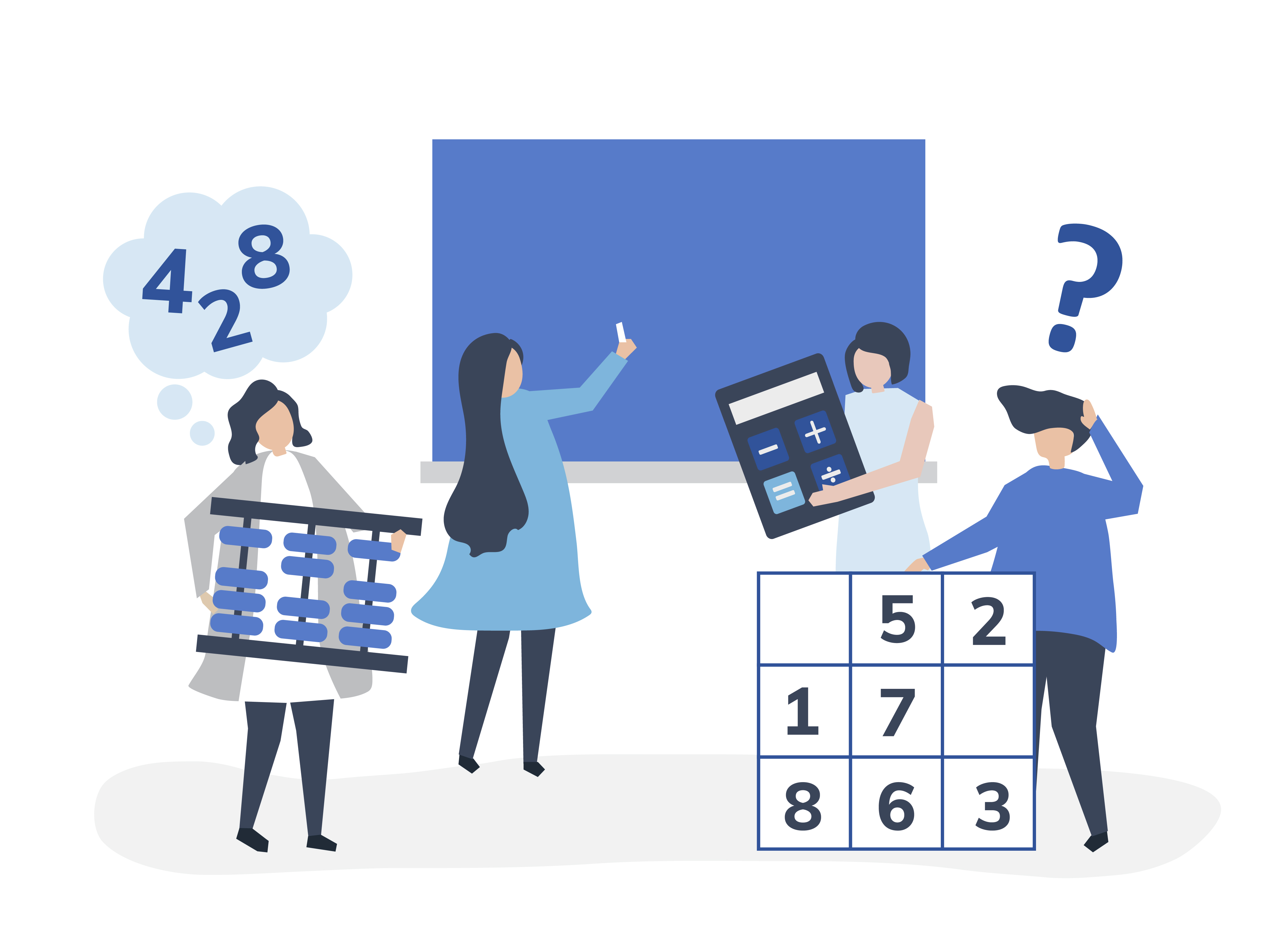
I was working on my personal project this week when I stopped to reflect on the number of Python Modules there are. I mean, they are quite a lot of them and there’s actually a difference between modules and libraries that I’ve been ignoring for so long making me wonder how often I’ve humiliated myself in tech conventions and if these modules would save me when AI ultimately takes over the job I’m still trying to make a mark in…
The point is, before the existential crisis and bucket of ice cream that followed, I took a moment to appreciate Python’s wealth of libraries and modules; and yes there is a difference between modules and libraries even though they are sometimes used interchangeably. You see, libraries are a collection of related modules and packages, whereas modules are .py files that contain code that do stuff. Now that’s cleared up, you can find out what all these python modules locally installed are using the code below:
help("modules")
Now let’s move on to the module we’ll be checking out today: The math module.
The Math Module
The math module makes it easy for us to perform complex ( or simple) mathematical computations. To use it we simply import it like so:
import math
You can find a variety of constants and functions in the math module, allowing you to do things like round up numbers, get square roots of numbers, get constants like pi or tau and so on. Below is a code snippet showing some of these constants and methods as well as their usage.
import math
someNumber = 3.555
x = 4
y = 6
# some math module constants
print(math.pi) #prints pi
print(math.tau) #prints tau (ratio of circle circumference to radius)
print(math.e) #Euler's
# some math module methods
print(math.cos(someNumber)) #prints cosine of number (in radians)
print(math.ceil(someNumber)) #rounds to the nearest integer upwards
print(math.floor(someNumber)) #rounds to the nearest integer downwards
print(math.log(someNumber)) #prints the natural logarithm of a number
print(math.radians(someNumber)) #converts a value in degrees to radians
print(math.isclose(x, y)) #checks if two numbers are close to each other
print(math.sqrt(x)) #prints the square root of x
print(math.tan(y)) #prints the tangent of y
Check out an extensive list here.
I think I’ll write short posts like these where each one is about a module. It is important to know these in Python as they could make you less prone to pulling out your hair. However, try building some of these functionalities yourself by creating your own modules for the sake of being a better programmer. Don’t know how? We would have a look at that next time.
Subscribe to my newsletter
Read articles from Amanda Ene Adoyi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Amanda Ene Adoyi
Amanda Ene Adoyi
I am a Software Developer from Nigeria. I love learning about new technologies and talking about coding. I talk about coding so much that I genuinely think I could explode if I don't . I have been freelance writing for years and now I do the two things I love the most: writing and talking about tech.