Arrays and Slices in Go: Basic Operations and Capabilities

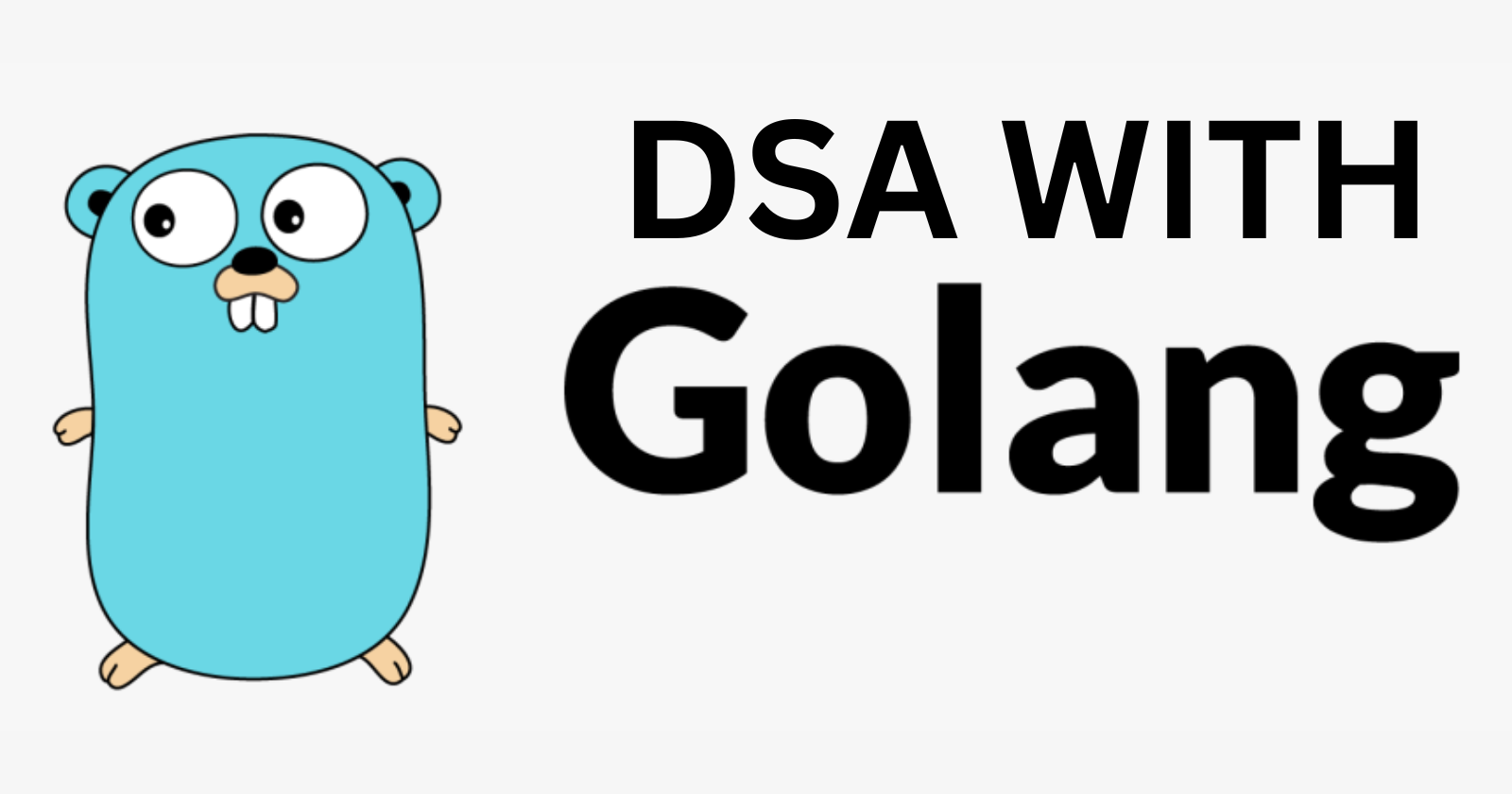
In the Go programming language, arrays and slices are fundamental data structures that offer unique ways to handle collections of data. This blog will walk you through basic operations such as insertion and deletion, as well as slicing and managing capacity. By the end, you'll have a solid understanding of how to work with these constructs in Go.
Arrays in Go
An array is a fixed-size sequence of elements of a specific type. The size of an array is determined when it's declared and cannot be changed.
Declaring Arrays
goCopy codevar arr [5]int // An array of 5 integers
Inserting Elements
Elements can be assigned to specific positions within the array.
goCopy codearr[0] = 10
arr[1] = 20
fmt.Println(arr) // Output: [10 20 0 0 0]
Deleting Elements
You cannot "delete" an element from an array as its size is fixed. Instead, you can set the element to its zero value.
goCopy codearr[1] = 0
fmt.Println(arr) // Output: [10 0 0 0 0]
Slices in Go
Slices provide a more flexible and powerful way to work with sequences of elements. They are built on top of arrays and provide dynamic sizing.
Creating Slices
Slices can be created in various ways, including from an array or using the make
function.
goCopy codeslice := []int{1, 2, 3, 4, 5}
fmt.Println(slice) // Output: [1 2 3 4 5]
anotherSlice := make([]int, 5) // A slice of length 5
fmt.Println(anotherSlice) // Output: [0 0 0 0 0]
Inserting Elements
To insert elements into a slice, you can use the append
function. It automatically resizes the slice if needed.
goCopy codeslice = append(slice, 6)
fmt.Println(slice) // Output: [1 2 3 4 5 6]
Deleting Elements
Deleting elements from a slice involves slicing and appending. Here’s how you can remove the element at index 2
:
goCopy codeslice = append(slice[:2], slice[3:]...)
fmt.Println(slice) // Output: [1 2 4 5 6]
Slicing and Capacity
Slicing
Slicing allows you to create a new slice from an existing one, specifying a start and end index.
goCopy codesubSlice := slice[1:4]
fmt.Println(subSlice) // Output: [2 4 5]
Capacity
The capacity of a slice is the number of elements in the underlying array, starting from the first element in the slice. You can check the capacity using the cap
function.
goCopy codefmt.Println(len(slice)) // Output: 5
fmt.Println(cap(slice)) // Output: 5
When you slice a slice, the capacity changes to reflect the underlying array's remaining capacity from the start of the new slice.
goCopy codenewSlice := slice[1:3]
fmt.Println(len(newSlice)) // Output: 2
fmt.Println(cap(newSlice)) // Output: 4
Example: Working with Slices
Let's put everything together with a practical example.
goCopy codepackage main
import "fmt"
func main() {
// Create a slice
numbers := []int{1, 2, 3, 4, 5}
fmt.Println("Initial slice:", numbers)
// Append elements
numbers = append(numbers, 6)
fmt.Println("After appending 6:", numbers)
// Delete element at index 2
numbers = append(numbers[:2], numbers[3:]...)
fmt.Println("After deleting element at index 2:", numbers)
// Slicing
subSlice := numbers[1:4]
fmt.Println("Sub-slice from index 1 to 4:", subSlice)
// Capacity
fmt.Println("Length of sub-slice:", len(subSlice))
fmt.Println("Capacity of sub-slice:", cap(subSlice))
}
Conclusion
Arrays and slices are essential tools for handling collections of data in Go. Arrays offer fixed-size sequences, while slices provide dynamic sizing and flexibility. Understanding their basic operations and how to manage slicing and capacity will empower you to handle data more efficiently in your Go programs. Happy coding!
Feel free to experiment with the provided examples and explore further to deepen your understanding of arrays and slices in Go.
Subscribe to my newsletter
Read articles from Sagar Mohanty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sagar Mohanty
Sagar Mohanty
Developer from India experienced in C#, React, and Python. Diving into the world of Go, and loving every bit of the simple yet powerful language.