A Comprehensive Guide to AWS SAM Template for Lambda Functions: Events and Environment Variables
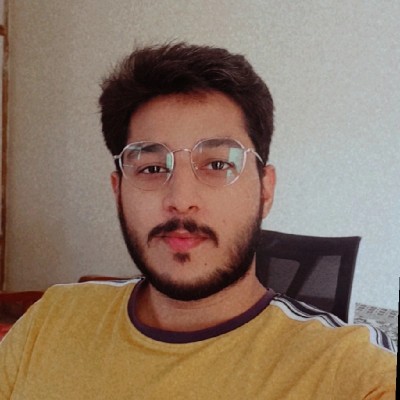

AWS SAM (Serverless Application Model) simplifies building and deploying serverless applications on AWS. In this guide, we’ll dive into the details of how to define different types of events in an AWS SAM template that trigger Lambda functions, such as API Gateway, SQS, SNS, and S3. Additionally, we'll explore how to set environment variables for these Lambda functions.
Understanding AWS SAM and Its Core Components
AWS SAM is an extension of AWS CloudFormation that provides a simplified way to define the Amazon API Gateway APIs, AWS Lambda functions, Amazon DynamoDB tables, and Amazon S3 buckets needed by your serverless application. The SAM template uses simple, declarative syntax and supports all the same resource types and properties as AWS CloudFormation.
Defining Events in AWS SAM
Events are configurations that specify how a Lambda function gets triggered. Here are examples of different event sources supported by AWS SAM:
1. API Gateway Event
The API Gateway event allows a Lambda function to be invoked via HTTP requests. Here’s how to define an API Gateway event in your SAM template:
Resources:
MyApiFunction:
Type: AWS::Serverless::Function
Properties:
Handler: index.handler
Runtime: nodejs14.x
Environment:
Variables:
MY_ENV_VAR: my-value
Events:
ApiEvent:
Type: Api
Properties:
Path: /myresource
Method: get
Handler: Specifies the file and function where the Lambda code is written (
index.handler
).Runtime: Defines the runtime environment for the Lambda function (
nodejs14.x
).Environment: Sets environment variables for the function.
Events: Configures the events that trigger the Lambda function.
ApiEvent: The name of the event.
Type: The event type (Api).
Properties: Defines the API Gateway specifics.
Path: The path of the API endpoint (
/myresource
).Method: The HTTP method (
get
).
2. SQS Event
The SQS event triggers a Lambda function when messages are sent to an SQS queue:
Resources:
MySqsFunction:
Type: AWS::Serverless::Function
Properties:
Handler: index.handler
Runtime: nodejs14.x
Environment:
Variables:
MY_ENV_VAR: my-value
Events:
SQSEvent:
Type: SQS
Properties:
Queue: !GetAtt MyQueue.Arn
BatchSize: 10
MyQueue:
Type: AWS::SQS::Queue
Queue: The ARN of the SQS queue that triggers the Lambda function.
BatchSize: Maximum number of records to retrieve from the queue at once.
3. SNS Event
The SNS event invokes a Lambda function when a message is published to an SNS topic:
Resources:
MySnsFunction:
Type: AWS::Serverless::Function
Properties:
Handler: index.handler
Runtime: nodejs14.x
Environment:
Variables:
MY_ENV_VAR: my-value
Events:
SNSEvent:
Type: SNS
Properties:
Topic: !Ref MyTopic
MyTopic:
Type: AWS::SNS::Topic
- Topic: The reference to the SNS topic that triggers the Lambda function.
4. S3 Event
The S3 event triggers a Lambda function when an object is created or deleted in an S3 bucket:
Resources:
MyS3Function:
Type: AWS::Serverless::Function
Properties:
Handler: index.handler
Runtime: nodejs14.x
Environment:
Variables:
MY_ENV_VAR: my-value
Events:
S3Event:
Type: S3
Properties:
Bucket: !Ref MyBucket
Events: s3:ObjectCreated:*
MyBucket:
Type: AWS::S3::Bucket
Bucket: The reference to the S3 bucket that triggers the Lambda function.
Events: The S3 event type that triggers the function (e.g.,
s3:ObjectCreated:*
).
5. Scheduled Event (CloudWatch Events)
The Scheduled event triggers a Lambda function on a scheduled basis using CloudWatch Events:
Resources:
MyScheduledFunction:
Type: AWS::Serverless::Function
Properties:
Handler: index.handler
Runtime: nodejs14.x
Environment:
Variables:
MY_ENV_VAR: my-value
Events:
ScheduleEvent:
Type: Schedule
Properties:
Schedule: rate(5 minutes)
- Schedule: The schedule expression (e.g.,
rate(5 minutes)
).
6. DynamoDB Event
The DynamoDB event triggers a Lambda function when there are changes in a DynamoDB table:
Resources:
MyDynamoDBFunction:
Type: AWS::Serverless::Function
Properties:
Handler: index.handler
Runtime: nodejs14.x
Environment:
Variables:
MY_ENV_VAR: my-value
Events:
DynamoDBEvent:
Type: DynamoDB
Properties:
Stream: !GetAtt MyTable.StreamArn
BatchSize: 5
StartingPosition: TRIM_HORIZON
MyTable:
Type: AWS::DynamoDB::Table
Properties:
AttributeDefinitions:
- AttributeName: id
AttributeType: S
KeySchema:
- AttributeName: id
KeyType: HASH
BillingMode: PAY_PER_REQUEST
StreamSpecification:
StreamViewType: NEW_IMAGE
Stream: The ARN of the DynamoDB stream.
BatchSize: The maximum number of records to retrieve from the stream at once.
StartingPosition: The position in the stream where Lambda should start reading (e.g.,
TRIM_HORIZON
).
Setting Environment Variables for Lambda Functions
Environment variables allow you to pass configuration values to your Lambda function. These variables can be set in the AWS SAM template under the Properties
section of the function definition.
Here is a generic example showing how to set environment variables for a Lambda function:
Resources:
MyLambdaFunction:
Type: AWS::Serverless::Function
Properties:
Handler: index.handler
Runtime: nodejs14.x
Environment:
Variables:
ENV_VAR_ONE: value1
ENV_VAR_TWO: value2
Why Use Environment Variables?
Environment variables are useful for:
Managing configuration values without changing the code.
Securing sensitive information such as API keys.
Differentiating settings between environments (e.g., development, staging, production).
Example: Combining Events and Environment Variables
Here’s a more comprehensive example combining multiple events and environment variables:
Resources:
MyMultiEventFunction:
Type: AWS::Serverless::Function
Properties:
Handler: index.handler
Runtime: nodejs14.x
Environment:
Variables:
ENV_VAR_ONE: value1
ENV_VAR_TWO: value2
Events:
ApiEvent:
Type: Api
Properties:
Path: /myresource
Method: get
SQSEvent:
Type: SQS
Properties:
Queue: !GetAtt MyQueue.Arn
BatchSize: 10
SNSEvent:
Type: SNS
Properties:
Topic: !Ref MyTopic
S3Event:
Type: S3
Properties:
Bucket: !Ref MyBucket
Events: s3:ObjectCreated:*
ScheduleEvent:
Type: Schedule
Properties:
Schedule: rate(5 minutes)
DynamoDBEvent:
Type: DynamoDB
Properties:
Stream: !GetAtt MyTable.StreamArn
BatchSize: 5
StartingPosition: TRIM_HORIZON
MyQueue:
Type: AWS::SQS::Queue
MyTopic:
Type: AWS::SNS::Topic
MyBucket:
Type: AWS::S3::Bucket
MyTable:
Type: AWS::DynamoDB::Table
Properties:
AttributeDefinitions:
- AttributeName: id
AttributeType: S
KeySchema:
- AttributeName: id
KeyType: HASH
BillingMode: PAY_PER_REQUEST
StreamSpecification:
StreamViewType: NEW_IMAGE
In this example:
The Lambda function
MyMultiEventFunction
is configured to handle multiple types of events (API Gateway, SQS, SNS, S3, Schedule, DynamoDB).Environment variables
ENV_VAR_ONE
andENV_VAR_TWO
are set for use within the Lambda function.
Conclusion
AWS SAM simplifies the process of defining and deploying serverless applications on AWS. By using SAM templates, you can easily configure Lambda functions to be triggered by various event sources and manage configuration through environment variables. This guide covered the most common event sources and how to set environment variables, providing a robust foundation for building scalable serverless applications.
Feel free to experiment with these examples and adjust them to fit your specific use cases. Happy coding!
Subscribe to my newsletter
Read articles from Waleed Javed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
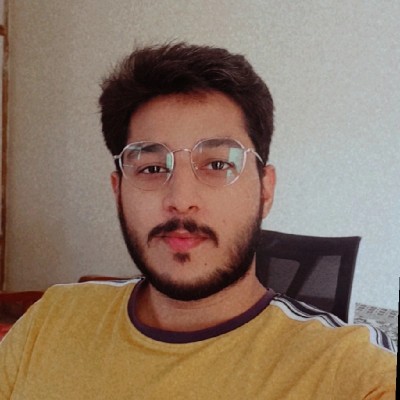
Waleed Javed
Waleed Javed
Building Enterprise Softwares🚀 | Sharing insights on Linkedin/in/Waleed-javed