Boosting Angular Performance: Essential Optimization Techniques
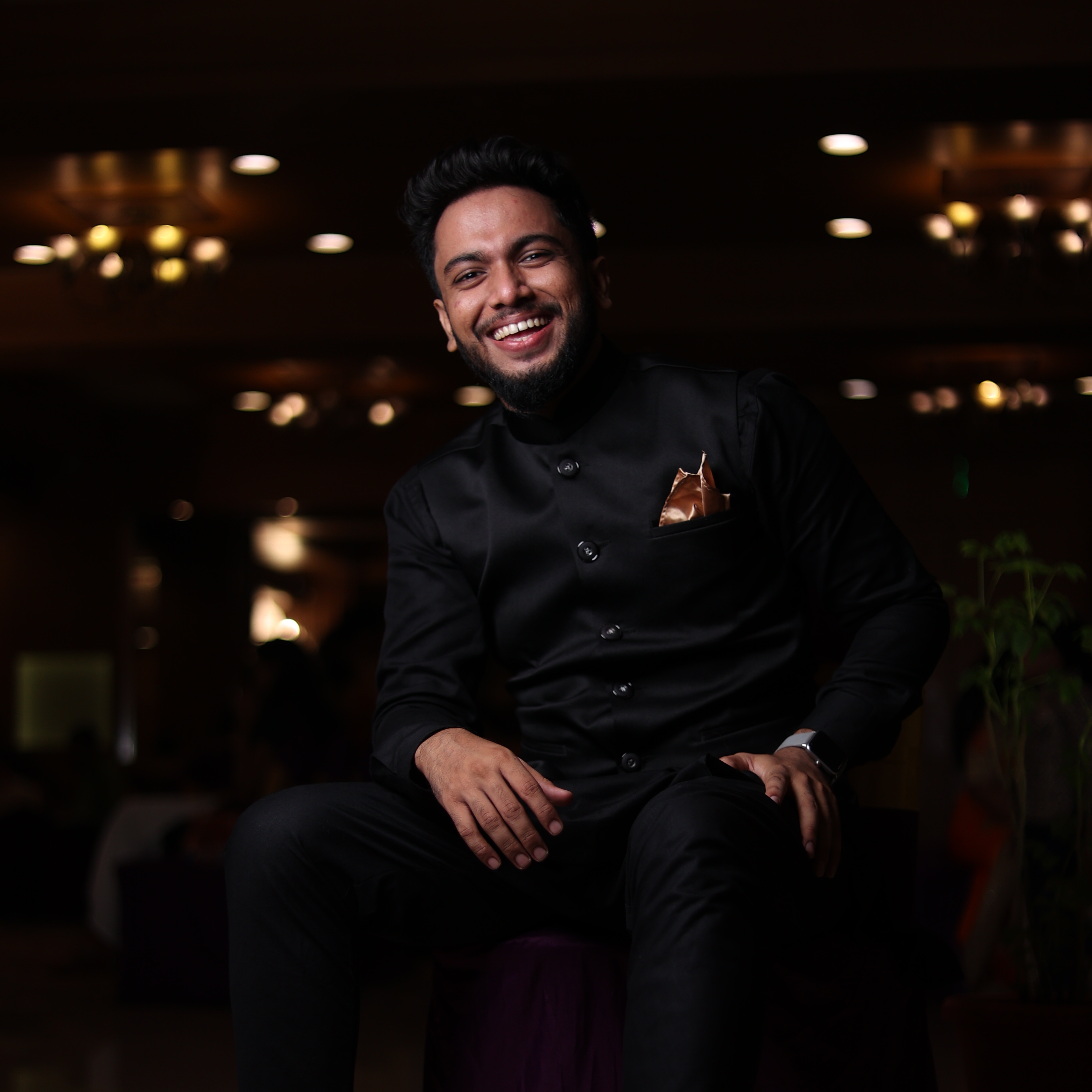
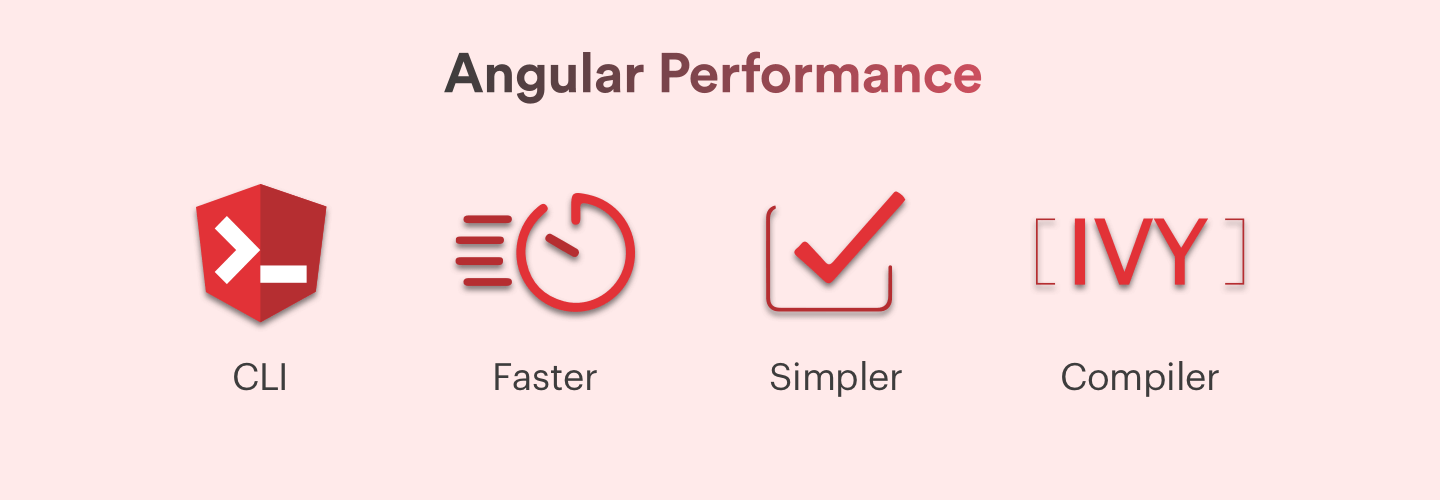
Introduction: Angular is a powerful front-end framework for building modern web applications, but as applications grow in size and complexity, ensuring optimal performance becomes increasingly crucial. In this article, we'll explore some essential optimization techniques that can help boost the performance of Angular applications, resulting in faster load times, smoother user experiences, and better overall efficiency.
Lazy Loading Modules:
Lazy loading allows you to load Angular modules asynchronously, improving initial load times by only loading the modules required for the current view.
By breaking down your application into feature modules and lazy loading them as needed, you can reduce the initial bundle size and improve time-to-interactivity.
Implement lazy loading using Angular's built-in routing module by defining lazy-loaded routes with loadChildren.
Tree Shaking:
Tree shaking is a technique for eliminating dead code or unused modules from your application bundle during the build process.
Ensure that your codebase is properly structured and use ES6 imports to allow the build tools to perform effective tree shaking.
Minimize the use of third-party libraries or only import the specific modules that are needed to reduce the size of the final bundle.
Ahead-of-Time (AOT) Compilation:
Angular supports both Just-in-Time (JIT) and Ahead-of-Time (AOT) compilation modes.
AOT compilation compiles your Angular templates and components during the build process, resulting in smaller bundle sizes and faster rendering in the browser.
Enable AOT compilation by default in your Angular CLI configuration to take advantage of these benefits.
Bundle Size Reduction:
Analyze and optimize the size of your application bundles to improve load times and reduce bandwidth usage.
Use tools like Webpack Bundle Analyzer to identify large dependencies or modules that can be further optimized or removed.
Consider code splitting and dynamic imports to load critical resources asynchronously and reduce the initial bundle size.
Optimizing Change Detection:
Angular's change detection mechanism is powerful but can become a performance bottleneck in large applications with frequent updates.
Optimize change detection by using OnPush change detection strategy for components that don't rely on external inputs.
Use Immutable.js or Object.freeze() to ensure that objects are immutable and avoid unnecessary change detection cycles.
Caching and Memoization:
Implement caching and memoization techniques to reduce redundant computations and improve performance, especially for expensive operations or data fetching.
Cache HTTP requests and responses using Angular's HttpClient or a service worker to minimize network requests and latency.
Memoize function results to avoid recalculating values that have already been computed.
Conclusion: By applying these optimization techniques, you can significantly improve the performance of your Angular applications, resulting in faster load times, better user experiences, and increased efficiency. However, performance optimization is an ongoing process, and it's essential to continuously monitor and measure the performance of your application and apply further optimizations as needed. With a proactive approach to performance optimization, you can ensure that your Angular applications deliver optimal performance across various devices and network conditions.
Subscribe to my newsletter
Read articles from Ashay Sawarkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
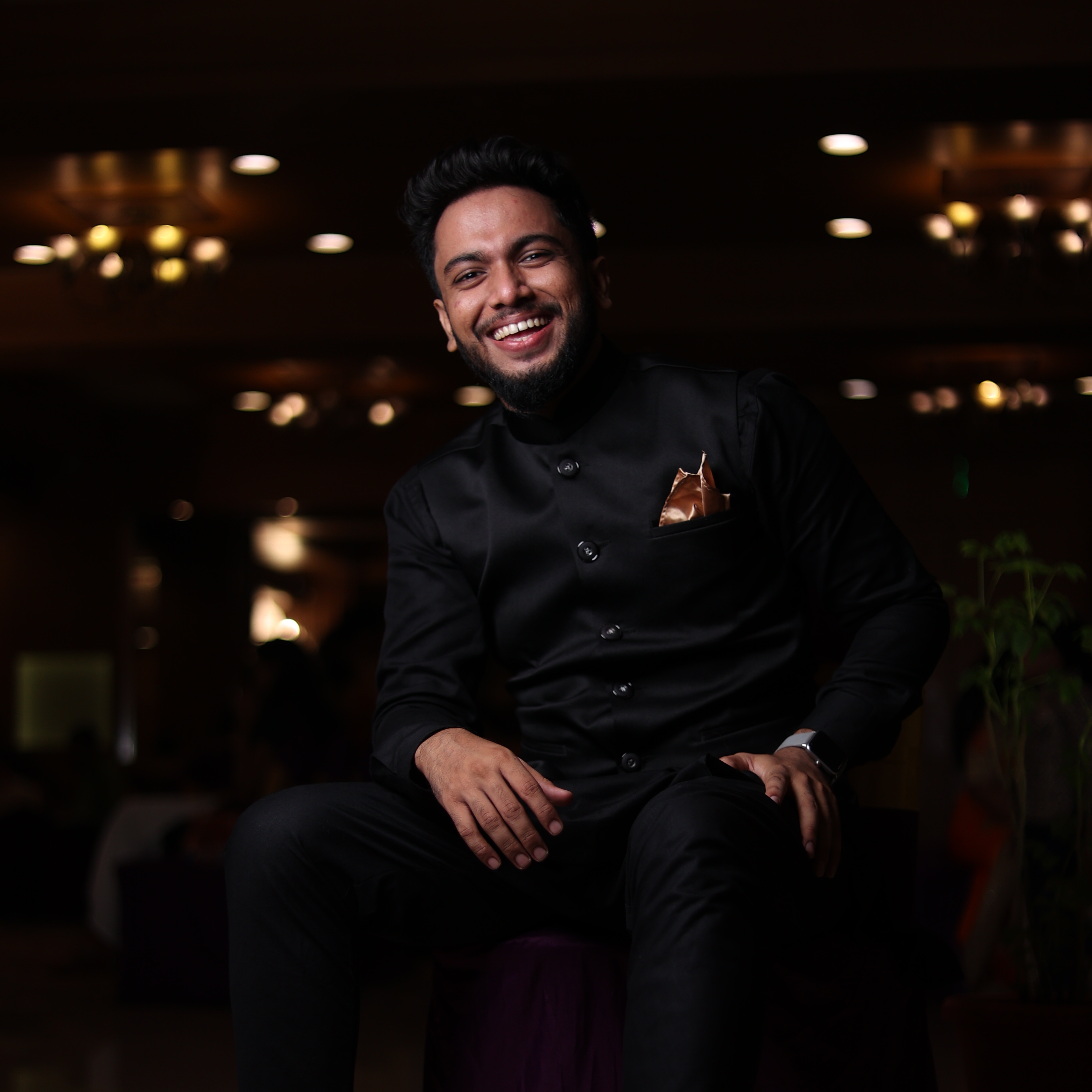
Ashay Sawarkar
Ashay Sawarkar
Passionate software developer and tech enthusiast on a journey to unlock the limitless possibilities of the digital realm. With a love for clean code and captivating design, I'm here to share my insights, experiences, and tips to help you embark on your own web development adventure. Let's transform your coding dreams into pixel-perfect reality!