Harnessing the Power of Tanstack Query: useQuery and useMutation

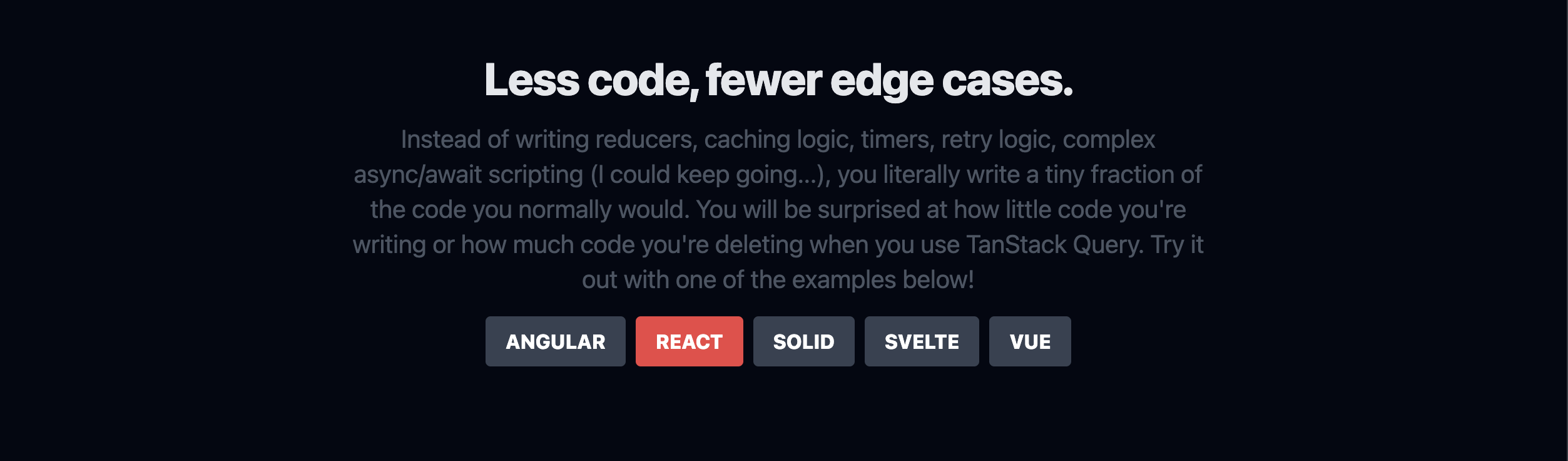
Tanstack Query (previously known as React Query) is a powerful data-fetching library that simplifies data synchronization in React applications. In this article, we will delve into two of its essential hooks: useQuery
and useMutation
. These hooks enable efficient data fetching, caching, synchronization, and more.
What is Tanstack Query?
Tanstack Query provides hooks for fetching, caching, synchronizing, and updating server state in your React applications without the need to manage complex state logic manually. It offers a robust solution for handling asynchronous operations in a declarative manner.
useQuery
: Fetching Data Made Easy
The useQuery
hook is designed for fetching and caching data. It allows you to declaratively fetch data from an API and handles the caching and updating of data seamlessly.
Here's a basic example of how to use useQuery
to fetch data from an API:
import React from 'react';
import { useQuery } from '@tanstack/react-query';
import axios from 'axios';
const fetchTodos = async () => {
const { data } = await axios.get('https://jsonplaceholder.typicode.com/todos');
return data;
};
const Todos = () => {
const { data, error, isLoading } = useQuery({ queryKey: ['todos'], queryFn: fetchTodos });
if (isLoading) return <div>Loading...</div>;
if (error) return <div>Error: {error.message}</div>;
return (
<div>
<h1>Todos</h1>
<ul>
{data.map(todo => (
<li key={todo.id}>{todo.title}</li>
))}
</ul>
</div>
);
};
export default Todos;
Explanation
Define a fetch function: The
fetchTodos
function usesaxios
to fetch data from an API.Use
useQuery
: TheuseQuery
hook takes an object withqueryKey
andqueryFn
properties.queryKey
ensures unique identification for the query, andqueryFn
is the function to fetch the data.Handle different states: The hook returns an object with
data
,error
, andisLoading
. You can use these to handle loading states, errors, and to display the fetched data.
useMutation
: Simplifying Data Mutations
While useQuery
is for fetching data, useMutation
is used for creating, updating, or deleting data. It provides a way to perform side effects and integrates seamlessly with Tanstack Query's cache.
Here's an example of how to use useMutation
to create a new todo item:
import React from 'react';
import { useMutation, useQueryClient } from '@tanstack/react-query';
import axios from 'axios';
const addTodo = async (newTodo) => {
const { data } = await axios.post('https://jsonplaceholder.typicode.com/todos', newTodo);
return data;
};
const AddTodo = () => {
const queryClient = useQueryClient();
const mutation = useMutation({
mutationFn: addTodo,
onSuccess: () => {
queryClient.invalidateQueries({ queryKey: ['todos'] });
},
});
const handleAddTodo = () => {
mutation.mutate({ title: 'New Todo', completed: false });
};
return (
<div>
<button onClick={handleAddTodo}>Add Todo</button>
</div>
);
};
export default AddTodo;
Explanation
Define a mutation function: The
addTodo
function usesaxios
to post a new todo item.Use
useMutation
: TheuseMutation
hook takes an object withmutationFn
and anonSuccess
callback.Handle success: The
onSuccess
callback invalidates the['todos']
query to ensure the list is refetched with the new data.Trigger the mutation: Call
mutation.mutate
with the new todo item when the button is clicked.
Conclusion
Tanstack Query's useQuery
and useMutation
hooks provide a robust and declarative approach to data fetching and mutations in React applications. They help manage server state efficiently, allowing developers to focus on building rich, interactive UIs without worrying about the complexities of data synchronization.
By leveraging these hooks, you can ensure that your application remains responsive, reliable, and maintainable. Happy coding!
Subscribe to my newsletter
Read articles from Atharva Mulgund directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Atharva Mulgund
Atharva Mulgund
Software Developer| Passionate about MERN | React.js | Angular | Javascript | Typescript