Unleash the Power of Laravel and Redis: A Performance Boosting Tutorial
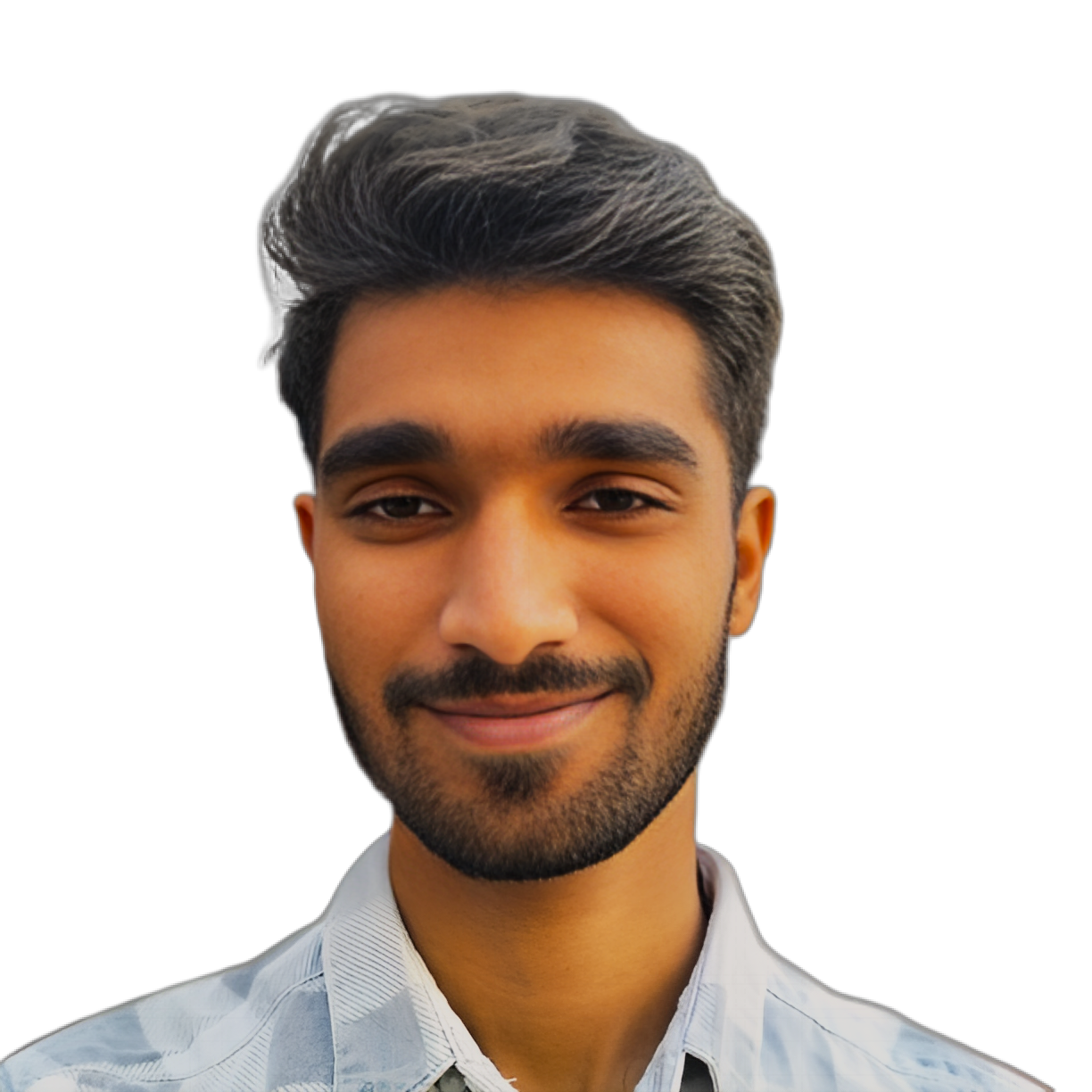

Introduction
In today's digital landscape, website speed is paramount. Users expect lightning-fast responses, and even slight delays can lead to frustration and lost conversions. While Laravel, a phenomenal PHP framework, excels in building dynamic web applications, sometimes you need an extra edge. That's where Redis comes in – a powerful in-memory data store that can significantly enhance your Laravel app's performance and scalability.
What is Redis?
Redis is an open-source, in-memory data store that excels in storing various data structures like strings, hashes, lists, sets, and sorted sets. Its blazing-fast performance, significantly exceeding traditional databases, makes it an ideal choice for caching and other performance-sensitive operations.
How Laravel and Redis Work Together
Laravel and Redis form a dynamic duo, offering a potent combination for optimizing your web application. Here are key ways they collaborate:
Caching: Store frequently accessed data like user sessions, product details, or API responses in Redis for rapid retrieval. This reduces database load and accelerates page rendering.
Code Example:
PHP
// Install the Laravel Redis package composer require laravel/redis // Within your controller or model: use Illuminate\Support\Facades\Cache; $userId = 1; $user = Cache::get('user:' . $userId); if (!$user) { $user = User::find($userId); Cache::put('user:' . $userId, $user, 60); // Cache for 60 minutes } return view('user.profile', compact('user'));
Queueing: Utilize Redis lists to handle asynchronous tasks like sending emails, processing images, or generating reports. This ensures background tasks don't impede user requests, maintaining a smooth user experience.
Code Example:
PHP
// Install the Laravel Queue package composer require laravel/framework // Within your controller or model: use Illuminate\Support\Facades\Queue; Queue::push(new SendWelcomeEmail($user));
Real-time Features: Leverage Redis Pub/Sub for real-time updates in applications like chat rooms, live leaderboards, or stock tickers. When a user publishes a message, Redis instantly broadcasts it to all subscribed clients, enabling seamless real-time communication.
Code Example:
PHP
// Install the Laravel Broadcasting package composer require laravel/broadcasting // Within your controller or model: use Illuminate\Support\Facades\Redis; Redis::publish('chat-channel', json_encode(['message' => 'Hello, world!']));
Benefits of Using Laravel and Redis
By incorporating Redis into your Laravel application, you can reap the following advantages:
Enhanced Performance: Redis's in-memory nature significantly speeds up data retrieval, leading to faster page loads and quicker user interactions.
Improved Scalability: Easily scale your application to handle increased traffic by adding more Redis instances.
Reduced Database Load: Offload frequent database queries to Redis, lessening the burden on your primary database and improving overall system efficiency.
Real-time Capabilities: Implement real-time features like chat applications or live updates, enhancing user engagement.
Getting Started with Laravel and Redis
Install Redis: Download and install Redis from the official website (https://redis.io/downloads/).
Configure Laravel: Update your Laravel configuration (
.env
file) with the Redis server details:REDIS_HOST=localhost REDIS_PORT=6379 REDIS_DATABASE=0
Utilize Laravel's Redis Facade: Laravel provides a convenient Redis facade (
Illuminate\Support\Facades\Redis
) for interacting with Redis from your code.
Beyond the Basics
For more advanced use cases, explore these Redis features:
Persistence: Configure Redis for persistence to ensure data is not lost upon restarts.
Sentinel and Cluster: Employ Redis Sentinel for high availability and Redis Cluster for horizontal scaling.
Conclusion
The combination of Laravel and Redis provides a powerful arsenal for crafting high-performance, scalable web applications. By implementing caching, queueing, and real-time features, you can elevate your user experience and ensure your application thrives in today's demanding web environment. Start experimenting, and witness the transformative power of Laravel and Redis!
Subscribe to my newsletter
Read articles from Asis Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
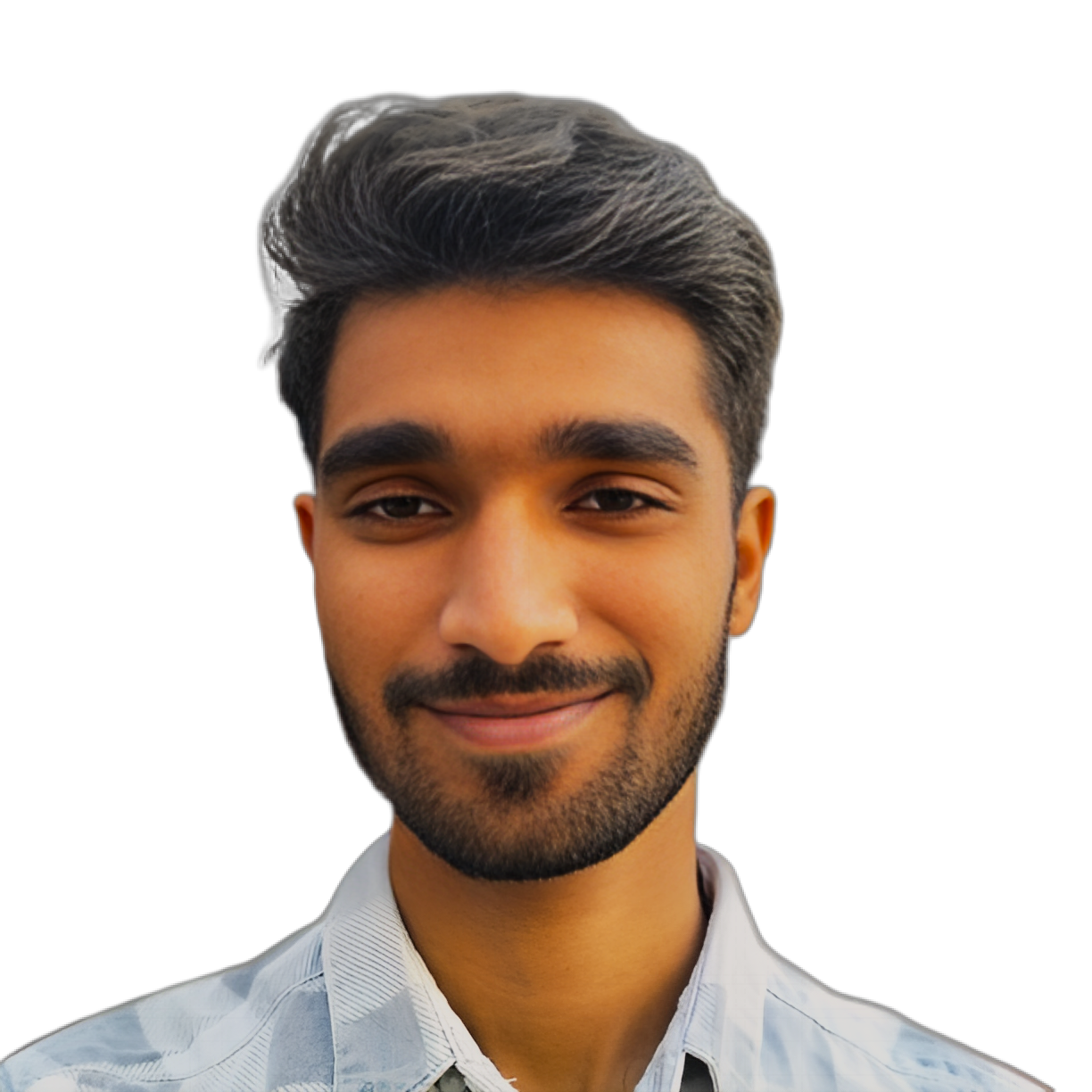
Asis Sharma
Asis Sharma
I'm an ambitious and motivated software developer, eager to apply my knowledge and skills to contribute to the development of innovative and cutting-edge software solutions. I'm well-versed in a variety of programming languages, including Javascript, C++, and Node.js, and have a understanding of object-oriented programming and algorithms. I'm passionate about developing and I am eager to learn and collaborate with others.