Go Pointers Made Easy: Understanding Memory in Go
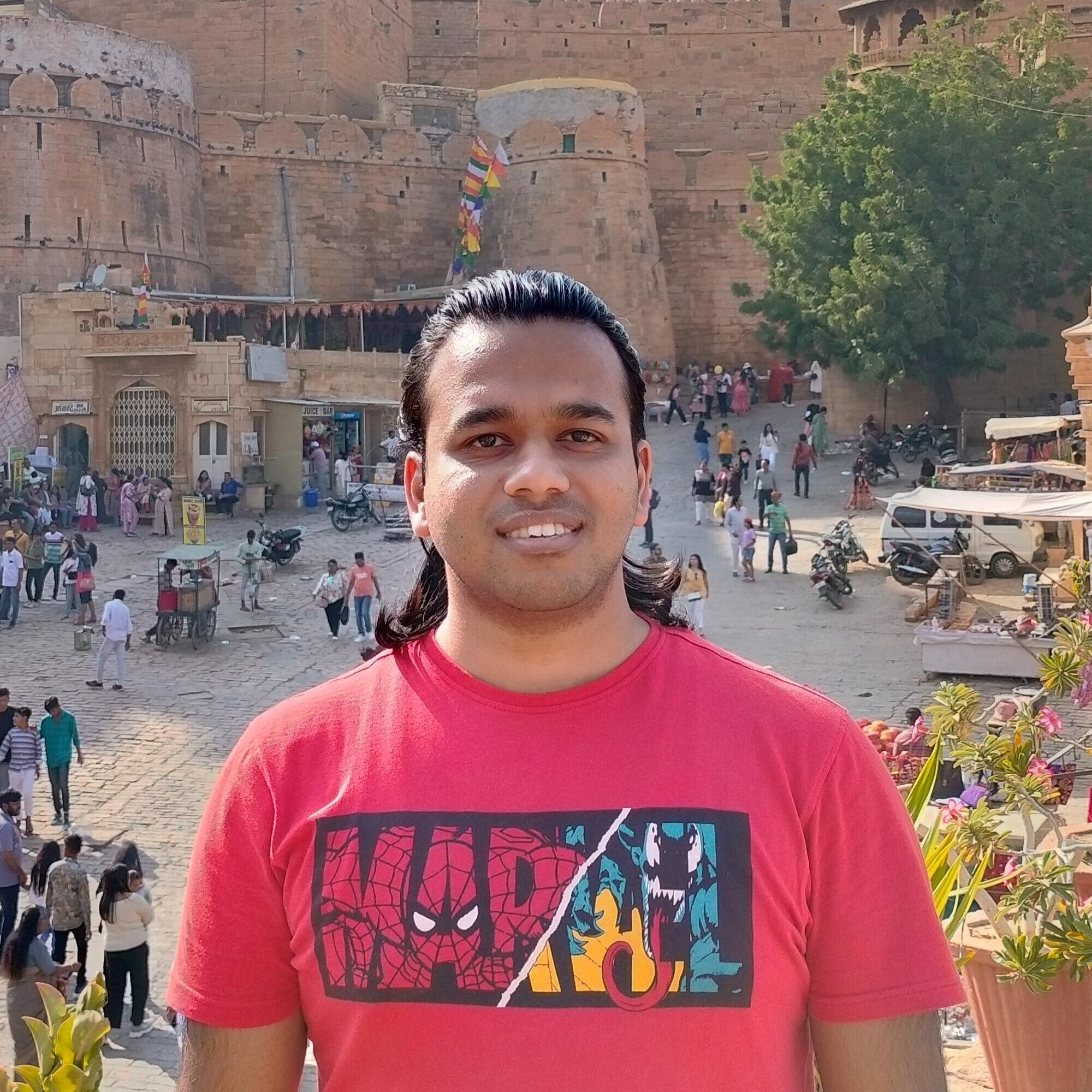
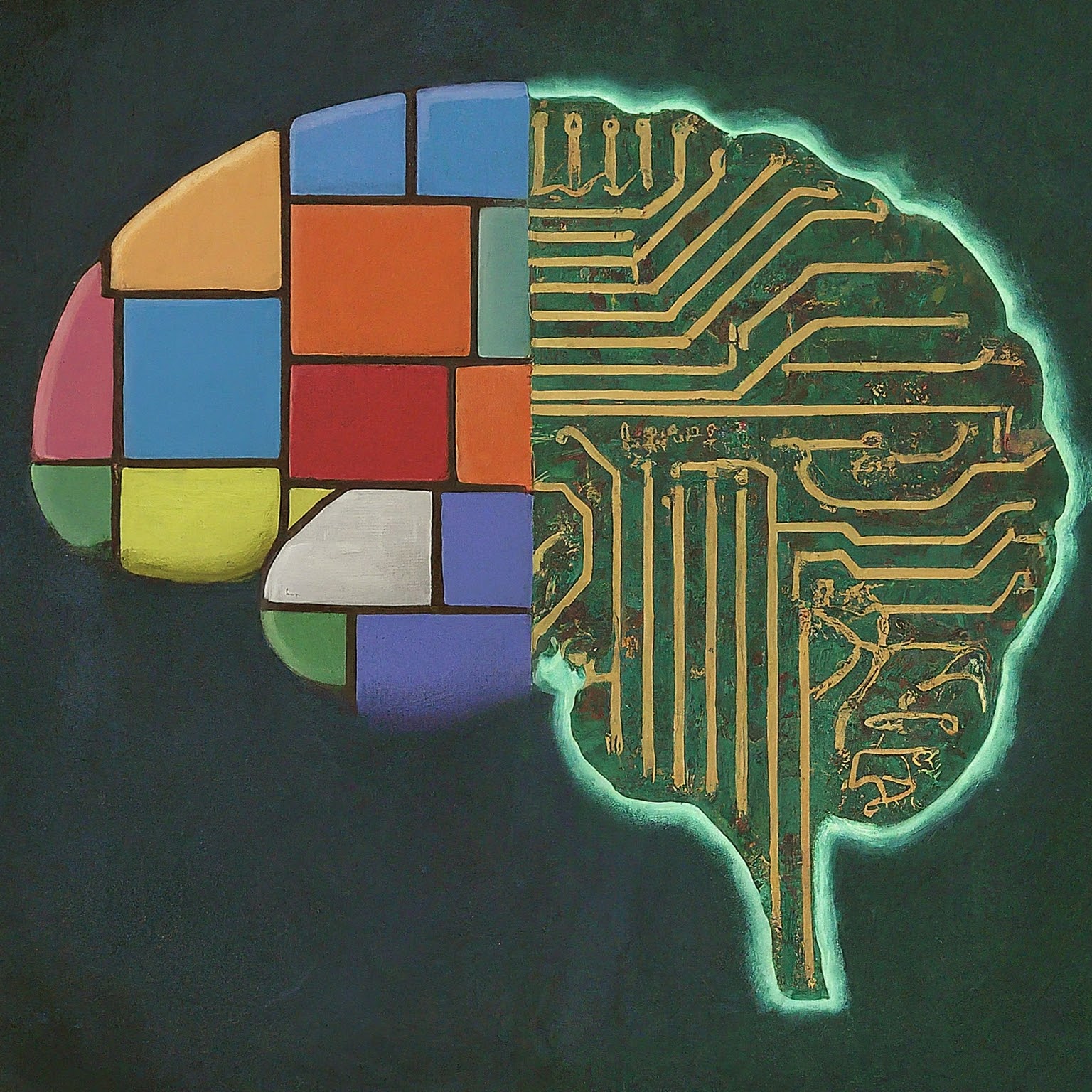
Go, a powerful and rapidly growing language, offers a clean and efficient syntax. However, one concept can often trip up newcomers: pointers. Pointers, though seemingly complex, are a fundamental building block for working with memory in Go and unlocking its full potential. This article delves into the world of Go pointers, explaining their core concepts, demonstrating their usage through practical examples, and highlighting the benefits they offer.
Understanding Variables and Memory
Before diving into pointers, let’s revisit the concept of variables. When you declare a variable in Go, you essentially reserve a space in memory to store a specific value. This memory location has an address, similar to a house address in the real world.
Imagine a variable named age
of type int
assigned the value 30. Here, age
acts as a label for a memory location that stores the integer value 30.
However, sometimes you might need to work directly with the memory address itself. This is where pointers come into play.
Pointers: Keys to Memory Locations
A pointer variable in Go is a variable that stores the memory address of another variable. It’s like a signpost pointing to the actual location where the data is stored. Here’s an analogy: Imagine you have a treasure chest buried somewhere, but instead of revealing the exact location, you provide a map with directions to the chest. The map acts as a pointer, guiding you to the actual treasure (data) stored in memory.
In Go, pointers are declared using the asterisk (*
) operator before the variable name. Let's see how to declare a pointer variable:
var agePtr *int
This code declares a pointer variable named agePtr
of type *int
. It means agePtr
can hold the memory address of an integer variable.
Dereferencing: Accessing the Treasure
Now that you have a pointer, how do you access the actual data it points to? This process is called dereferencing. You use the asterisk operator (*
) again, but this time before the pointer variable. Here's how:
age := 30
agePtr := &age // Assign the memory address of age to agePtr
// Dereference agePtr to access the value stored at the address
dereferencedValue := *agePtr
fmt.Println(dereferencedValue) // This will print 30
In this example, we first declare an integer variable age
with the value 30. Then, we declare a pointer variable agePtr
and assign the memory address of age
to it using the address-of operator (&
). Finally, we dereference agePtr
using the asterisk (*
) to access the value stored at the memory location pointed to by agePtr
, which is 30.
Key Takeaway: When you dereference a pointer, you’re essentially retrieving the value stored at the memory address it points to.
Practical Use Cases for Pointers
Pointers offer several advantages in Go programming:
- Modifying Values: Pointers allow you to modify the value of a variable indirectly. This is useful when you want to change the data within a function without affecting the original variable.
func updateAge(age *int) {
*age = *age + 1 // Dereference the pointer to modify the value at the pointed memory location
}
age := 30
updateAge(&age) // Pass the address of age to the function
fmt.Println(age) // This will print 31 (age has been modified)
- Passing Large Data Structures: When working with large data structures like arrays or slices, copying the entire structure can be inefficient. By passing pointers to these structures, you only pass the memory address, avoiding unnecessary copying.
func printArray(arr *[]int) {
for _, value := range *arr { // Dereference the pointer to access the array elements
fmt.Println(value)
}
}
myArray := []int{1, 2, 3}
printArray(&myArray)
- Building Advanced Data Structures: Pointers are essential for building complex data structures like linked lists, trees, and graphs, where nodes need to reference each other.
package main
import (
"fmt"
)
// Node represents a single element in the linked list
type Node struct {
Value int
Next *Node // Pointer to the next node in the list
}
// LinkedList represents the Singly Linked List data structure
type LinkedList struct {
Head *Node // Pointer to the head (first) node of the list
}
// NewNode creates a new Node with the given value
func NewNode(value int) *Node {
return &Node{Value: value}
}
// InsertAtBeginning inserts a new node at the beginning of the linked list
func (list *LinkedList) InsertAtBeginning(value int) {
newNode := NewNode(value)
newNode.Next = list.Head
list.Head = newNode
}
// PrintList iterates through the linked list and prints the value of each node
func (list *LinkedList) PrintList() {
currentNode := list.Head
for currentNode != nil {
fmt.Print(currentNode.Value, " -> ")
currentNode = currentNode.Next
}
fmt.Println("nil")
}
func main() {
// Create a new linked list
linkedList := &LinkedList{}
// Insert elements at the beginning
linkedList.InsertAtBeginning(5)
linkedList.InsertAtBeginning(3)
linkedList.InsertAtBeginning(1)
// Print the linked list
fmt.Println("Linked List:")
linkedList.PrintList()
}
When to Use Pointers
While pointers offer flexibility and efficiency, it’s important to use them judiciously. Here are some scenarios where pointers are beneficial:
When you need to modify the value of a variable passed to a function: As demonstrated in the previous example, pointers allow you to modify the original variable within a function by dereferencing the pointer and changing the value at the memory location it points to.
When working with large data structures: Passing pointers to large data structures like arrays or slices avoids unnecessary copying of the entire structure, improving performance.
Building complex data structures: Linked lists, trees, and graphs rely on pointers to establish relationships between nodes, enabling efficient navigation and manipulation of these structures.
Implementing low-level system interactions: When interacting with system resources or libraries written in C, pointers are often necessary for memory management and data exchange.
Common Pitfalls with Pointers
Understanding pointers can take practice, and there are potential pitfalls to be aware of:
- Nil Pointers: A pointer variable can be nil, meaning it doesn’t point to any valid memory location. Dereferencing a nil pointer leads to a runtime panic. Always check if a pointer is nil before dereferencing it.
var unknownPtr *int
if unknownPtr != nil { // Check if the pointer is nil before dereferencing
fmt.Println(*unknownPtr) // This line might cause a panic if unknownPtr is nil
}
Memory Leaks: If you create a pointer but don’t manage its lifetime properly, it can lead to memory leaks. Garbage collection in Go automatically reclaims unused memory, but understanding pointer ownership helps prevent leaks.
Dangling Pointers: When the memory location pointed to by a pointer is deallocated, the pointer becomes a dangling pointer. Dereferencing a dangling pointer can lead to unexpected behaviour or crashes. Be mindful of the lifetime of the data pointed to by your pointers.
Tips for Effective Pointer Usage
Here are some tips to ensure you’re using pointers effectively in your Go code:
Start simple: Begin by using pointers for basic tasks like modifying function arguments. Gradually progress to more complex scenarios as your understanding deepens.
Document pointer usage: Clearly document the purpose and usage of pointers in your code to improve readability and maintainability.
Use tools and libraries: Go offers tools like
go tool pprof
for profiling memory usage and identifying potential leaks. Utilise libraries that manage memory efficiently, reducing the need for manual pointer manipulation.
Conclusion
Pointers in Go, while initially appearing complex, offer a powerful tool for memory manipulation and building efficient programs. By understanding their core concepts, common pitfalls, and effective practices, you can leverage pointers to write cleaner, more performant, and flexible Go code. Remember to use pointers judiciously and prioritise clarity over complexity whenever possible. As you gain experience with Go, pointers will become a valuable asset in your coding arsenal.
Happy Coding 🚀
Thank you for reading until the end. Before you go:
Please consider liking and following! 👏
You can also go through my other article on Enums in Go
Subscribe to my newsletter
Read articles from Srijan Rastogi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
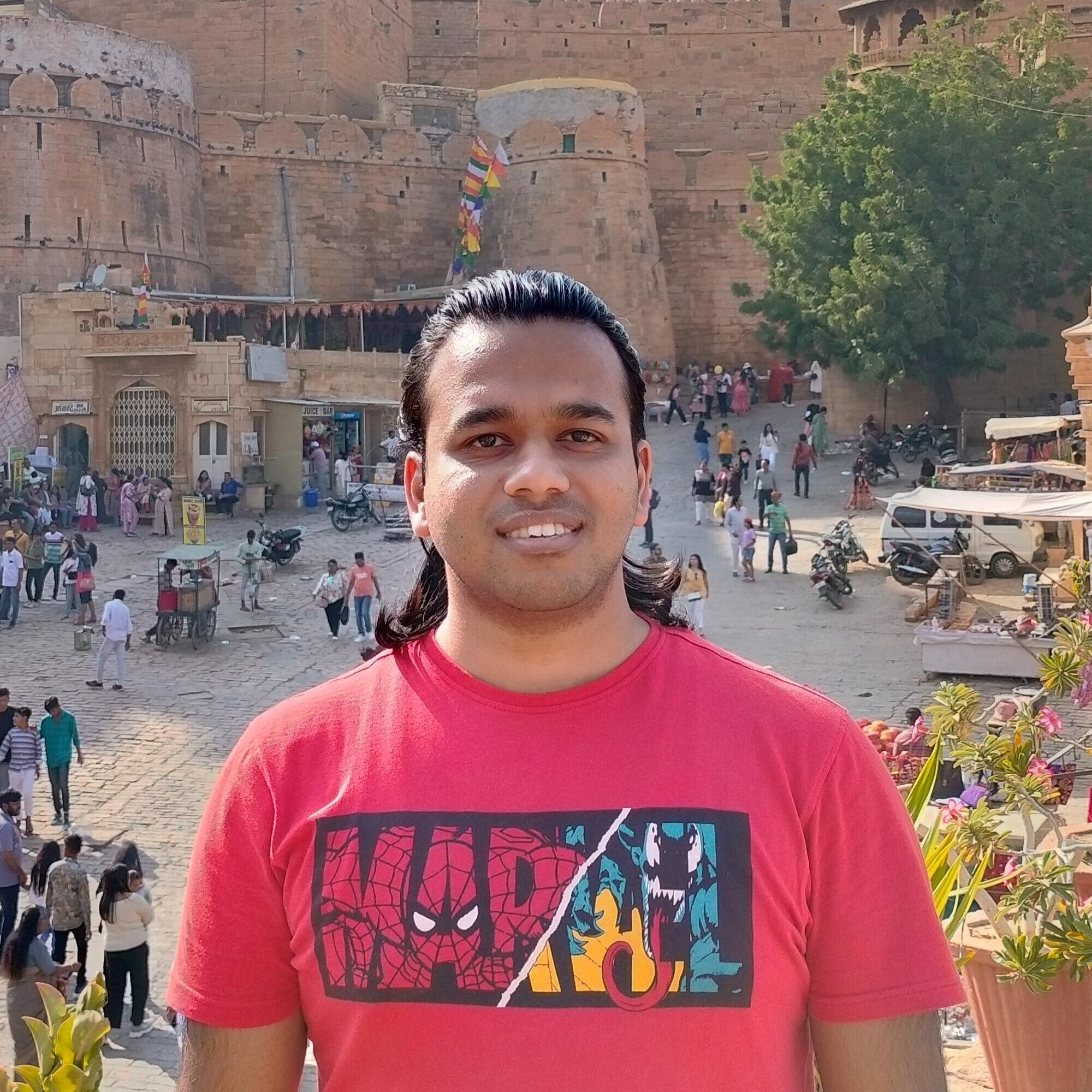
Srijan Rastogi
Srijan Rastogi
GoLang apprentice by day, sensei by night. Learning and building cool stuff, one commit at a time.