Mastering Laravel API Development: A Comprehensive Guide to Seamless Integration with Frontend

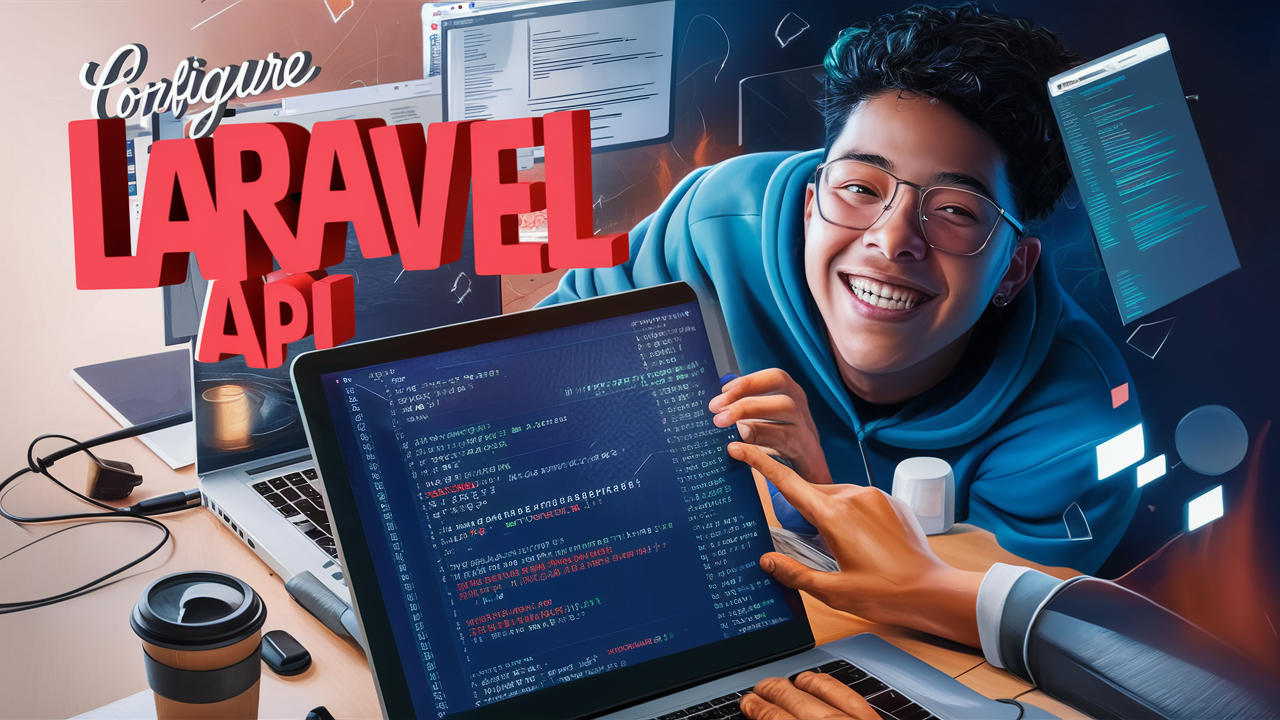
Connecting a Laravel API with a front-end framework can be challenging, and deploying the API often adds to the complexity. But don't worry; in this article, I'll guide you through the steps to seamlessly link your Laravel API with a front-end framework. While we’ll be using ReactJS for the front end, the process is quite similar for other frameworks as well.
ℹ️ Laravel updates frequently, and you might be working with a version different from the latest release. This can sometimes make things seem more complicated. Don’t worry, though—I’m here to help. If you have any questions, feel free to reach out to me on Instagram; I will be more than happy to assist. ℹ️
1- Create Laravel Project :
use the command Laravel new projectName
set up the Laravel project link it to a database and run the migrationphp artisan migrate
2- Setting up the .env file
In your .env
file, you will see various configurations that you need to set up.
A- Set the URLs
APP_URL=http://localhost:8000
FRONTEND_URL=http://localhost:3000
APP_URL
: This is the URL of your backend API, which by default is localhost:8000
. If your app is hosted or you have a custom domain, you can replace it with your domain, like so: APP_URL=https://learnLaravel.org
FRONTEND_URL
: This specifies the frontend URL and tells Laravel to only accept requests coming from this specific URL. For instance, if a request to your backend is sent from another domain, such as http://localhost:3001
, it will be blocked.
Think of it like instructing your child at home to only open the door when they hear your voice. In this analogy, your backend is the child, and you (the frontend) are the voice. If someone with a different voice asks your child to open the door, it won't happen.
🛑 Note: In my case, I want my API to be accessible to everyone, so I will remove the FRONTEND_URL
configuration. 🛑
If you want to specify your frontend host, you can do so like this:
FRONTEND_URL=http://localhost:3001
B- Set the domains
When building a Laravel API that will be accessed by various front-end applications, it's important to configure session and authentication settings properly. Two key environment variables in Laravel that control these settings are SESSION_DOMAIN
and SANCTUM_STATEFUL_DOMAINS
.
Understanding SESSION_DOMAIN
The SESSION_DOMAIN
environment variable defines the domain for which the session cookie is valid. This setting is crucial for security, ensuring that session cookies are only sent to the specified domain, thereby preventing cross-site request forgery (CSRF) attacks.
However, if you want your API to accept requests from any source, you can leave this variable empty:
SESSION_DOMAIN=
By leaving SESSION_DOMAIN
empty, Laravel will default to using the host of the incoming request as the session domain. This is useful in development environments or when your API needs to be accessed by multiple front-end applications without restriction.
Example: If your application is hosted on example.com
, you would set SESSION_DOMAIN=.
example.com
in your .env
file.
SESSION_DOMAIN=.example.com
Understanding SANCTUM_STATEFUL_DOMAINS
The SANCTUM_STATEFUL_DOMAINS
environment variable is used by Laravel Sanctum to specify which domains should receive stateful API authentication cookies. This setting is essential for Single Page Applications (SPAs) where the front-end and back-end might be hosted on different domains.
For scenarios where your API should accept requests from any domain, you can also leave this variable empty:
SANCTUM_STATEFUL_DOMAINS=
By leaving SANCTUM_STATEFUL_DOMAINS
empty, Sanctum will treat all incoming requests as stateful. This means that authentication cookies will be sent and received regardless of the requesting domain, facilitating easier integration with multiple front-end applications.
Example: If your frontend application is hosted on frontend.example.com
and your backend API is hosted on api.example.com
, you would set SANCTUM_STATEFUL_DOMAINS=
frontend.example.com
in your .env
file.
SANCTUM_STATEFUL_DOMAINS=frontend.example.com
Multiple Domains:
SANCTUM_STATEFUL_DOMAINS=frontend.example.com,another-frontend.example.com
Practical Example
Let's say you're building an article about creating a Laravel API and linking it with various front-end frameworks. To simplify the setup and allow unrestricted access during development, you can configure your .env
file as follows:
SESSION_DOMAIN=
SANCTUM_STATEFUL_DOMAINS=
Benefits and Considerations
Flexibility: This configuration allows your API to interact seamlessly with any front-end application, whether it’s a React app, Vue.js, or even a mobile app.
Ease of Development: It simplifies the development process, especially when dealing with multiple front-end clients or when the front-end domain is not fixed.
Security: While this setup is convenient for development and testing, it’s crucial to reconsider these settings in a production environment. Restricting session and stateful domains to specific trusted domains enhances security by preventing unauthorized access and mitigating CSRF attacks.
🛑 You can set the Sanctum Configuring in
config/sanctum.php
without the need to set it in .env file especially if you are building an accessible APIC- Configuring Sanctum in Laravel
Sanctum's configuration file is located at config/sanctum.php
. This file allows you to define various settings, including the domains that should receive stateful API authentication cookies. Here's an example of how to configure this file:
Stateful Domains:
The
stateful
key defines which domains should be considered stateful, meaning they will receive authentication cookies. This is crucial for SPAs that rely on cookie-based authentication.The configuration uses the
SANCTUM_STATEFUL_DOMAINS
environment variable. If this variable is empty, a default set of domains, including localhost and common local development URLs, is used.replace them with your domains if you have different ones.
'stateful' => explode(',', env('SANCTUM_STATEFUL_DOMAINS', sprintf(
'%s,%s%s',
'localhost,localhost:3000,127.0.0.1,127.0.0.1:8000,::1',
Sanctum::currentApplicationUrlWithPort(),
env('FRONTEND_URL') ? ','.parse_url(env('FRONTEND_URL'), PHP_URL_HOST) : ''
))),
In my case, I will use the below stateful
key to make my API accessible by any domain
'stateful' => ['*'],
3. Eliminating the CSRF Token Mismatch Error
When working with Laravel, you might encounter a CSRF token mismatch error. Here are two methods to resolve this issue:
Method 1: ModifyVerifyCsrfToken
Middleware
In some Laravel versions, you can find the VerifyCsrfToken
file at app/Http/Middleware/VerifyCsrfToken.php
. You can add the api/*
path to the $except
array:
<?php
namespace App\Http\Middleware;
use Illuminate\Foundation\Http\Middleware\VerifyCsrfToken as Middleware;
class VerifyCsrfToken extends Middleware
{
/**
* The URIs that should be excluded from CSRF verification.
*
* @var array
*/
protected $except = [
'api/*',
];
}
Method 2: Validate CSRF Tokens
You have two options for this method:
- Modify
ValidateCsrfTokens
Middleware: Navigate toValidateCsrfTokens.php
and look for the$except
array. You can add specific endpoints you want to exclude from CSRF verification:
protected $except = ['api/*']; // replace the api/* with the end point you want
- Modify
bootstrap/app.php
: This is the most recommended approach. You can add all the endpoints and URLs that will interact with your API:
->withMiddleware(function (Middleware $middleware) {
$middleware->validateCsrfTokens(except: [
'api/*',
'http://example.com/foo/bar',
'http://example.com/foo/*',
]);
})
There you go—congratulations! You've successfully configured the backend. Piece of cake, isn't it? 🥳
4. Frontend Axios Configuration (Optional)
While not strictly necessary, setting up Axios configuration is a good practice for a cleaner and more manageable codebase.
Setting Up Axios
Create Axios Configuration File: In your
src
folder, create a new folder namedAPI
. Inside this folder, create a file namedaxiosConfig.js
.Add Axios Configuration Code: Paste the following code into
axiosConfig.js
:
import axios from "axios";
export const axiosConfig = axios.create({
baseURL: "http://127.0.0.1:8000/api", // replace localhost 127.0.0.1:8000 with your domain
withCredentials: true,
xsrfCookieName: "XSRF-TOKEN",
xsrfHeaderName: "X-XSRF-TOKEN",
headers: {
Accept: "application/json",
},
});
axios.interceptors.response.use(null, (err) => {
console.log(err);
});
This configuration sets the base URL for all your API requests, handles CSRF tokens, and adds a response interceptor to log any errors.
- Using Axios Configuration: Instead of calling Axios directly, you can now import your custom
axiosConfig
.
Example of Creating a POST Request:
import { axiosConfig } from 'Api/axiosConfig';
const post = {
title: 'post title',
description: 'post description',
imageUrl: 'image.jpeg'
}
const handleSubmit = async (e) => {
e.preventDefault();
console.log(user);
axiosConfig
.post("/create", post)
.then((response) => alert(response.data.message))
.catch((error) => console.log(error));
};
5. Conclusion and Recap
Congratulations on reaching the end of this article! If you've made it this far, give yourself a pat on the back for your dedication and hard work. 💪🫡
Recap of What You've Learned:
Sanctum and Its Role: You've learned about Laravel Sanctum and its crucial role in handling API authentication and token management. Sanctum provides a simple yet powerful way to secure your API endpoints and manage user authentication.
Laravel Configuration: We've covered various aspects of Laravel configuration, including setting up the
.env
file, configuring URLs, and dealing with CSRF token mismatches. Understanding these configurations is essential for a smooth development experience.Middleware in Laravel: Middleware plays a vital role in Laravel's request lifecycle. You've explored how to modify middleware settings, exclude routes from CSRF verification, and handle API requests efficiently.
Avoiding CSRF Token Mismatch Errors: CSRF token mismatches can be a common issue, but you've learned effective methods to handle them, such as modifying the
VerifyCsrfToken
middleware or configuring Axios on the frontend.
Looking Ahead:
Stay tuned for the next article, where we'll dive into the exciting world of deploying a Laravel application. Deploying your application is a crucial step towards making it accessible to users worldwide, and we'll guide you through the process with ease. 😎
Keep coding, keep learning, and keep building amazing projects! If you have any questions or need further assistance, feel free to reach out. Happy coding! 🚀
Subscribe to my newsletter
Read articles from AMINE ABAIDI directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
