Gradio Basics: How to Use Gradio for Dummies
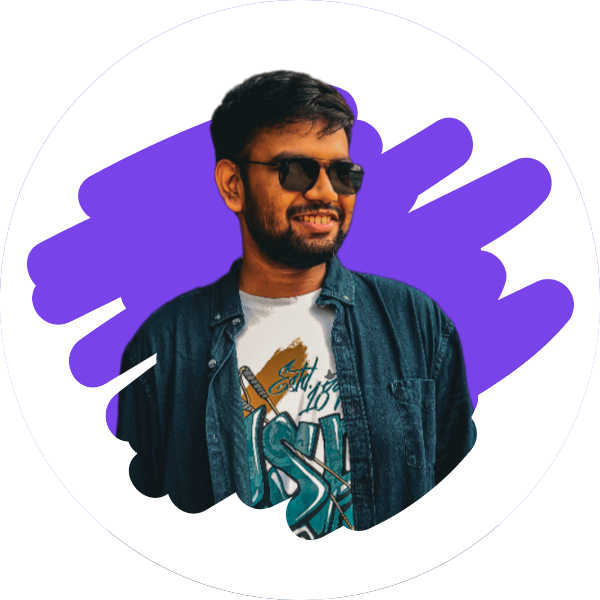
Welcome to an interesting package, Gradio, in Python! I'm Brahma π, a passionate software developer. I am documenting my learning journey through a series of blog posts. Stay tuned!!
Introduction
Gradio is an open-source Python package that allows users to quickly create customizable user interfaces (UIs) for machine learning (ML) models, APIs, or Python functions.
Why Gradio?
One question which you may ask why Gradio when we have Django or Flask?
So, the simple answer is CONVENIENCE. Yes, It has many things that are pre built into it that makes development and prototyping crazy fast!! Ofc, it does not provide that level of customisation as django or flask. I will try to explain in next few minutes why you should choose Gradio as your front-end package for your next side project.
Let's go π₯π₯
Installation
pip install gradio
Basics
Let's first create our own gradio app for trial. Don't worry about the code right now. Just follow along. π
import gradio as gr
def greet(name):
return "Hello, " + name
demo = gr.Interface(
fn=greet,
inputs=["text"],
outputs=["text"],
)
demo.launch()
That's it!! Yes, this much lines of code is needed to get your first Gradio app up and running. Now just go to the terminal, navigate to the code location and execute the code using python app.py
. You should get something like this:
Code Understanding
Now, let's understand what the code has to say. In Gradio, we just need a function to get started with building. Yes, its that simple!!
Write a function of your choice and pass it as the fn
parameter in gr.Interface()
. Define the inputs
and the outputs
. And voila!!! done!!! Add demo.launch()
and your app is ready.
Now that we know the working of Gradio, let's make a usefull app to feel productiveπ.
Coding - AI Dost
Dost in hindi means Friend. So, we will be making our own AI Dost whom you can chat with for funπ. You can try this on Hugging Face at AI-DOST
We will be using CrewAI for this project. CrewAI is an open-source framework that helps create AI agents that can work together to complete complex tasks.
So, what all do we need to build a Dost ?
Gemini Pro API key accessible at link.
Patience
Creativity (a must have π)
Let's Build
First things first the code:
from dotenv import load_dotenv
load_dotenv()
import os
from langchain_google_genai import ChatGoogleGenerativeAI
from crewai import Agent, Task, Crew, Process
import gradio as gr
# Defining the Agent
def agent():
agent = Agent(
role='AI Friend',
goal='Provide emotional support and to talk things over',
backstory="""You're a a indian friend who is a emotional person.
You're responsible for provide emotional support to your friend.
You're currently working on a achieving emotional stability so that you can help your friend overcome stress.
Be gentle and kind to your friend.
You will continuously learn from the feedback your friend gives.
You have to add occasional emojis in every conversation.
You have to reply in coversational style only unless stated otherwise.""",
llm=llm
)
return agent
# Defining the Task
def task(input):
task = Task(
description = f"""{input}""",
expected_output = """Replies like an Indian friend""",
agent = agent
)
return task
# Defining Crew
def crew(agent, task):
crew = Crew(
agents = [agent],
tasks = [task],
verbose =True
)
return crew
# The Driver Function
def dost(message, history):
task1 = task(message)
crew1 = crew(agent, task1)
result = crew1.kickoff()
print(result)
return result
if __name__ == "__main__":
# Load the google gemini api key
google_api_key = os.getenv("GOOGLE_API_KEY")
# Set gemini pro as llm
llm = ChatGoogleGenerativeAI(
model="gemini-pro", verbose=True, temperature=0.6, google_api_key=google_api_key
)
agent = agent()
demo = gr.ChatInterface(
fn=dost,
)
demo.launch(share=True)
Understanding the Jargon
Prerequisites
Python 3.7 or higher
Required libraries:
dotenv
,os
,langchain_google_genai
,crewai
,gradio
Google API key for accessing the Google Generative AI (Gemini Pro) model
Code Overview
Importing Libraries and Loading Environment Variables
The code starts by importing necessary libraries and loading environment variables, specifically the Google API key stored in a .env
file.
from dotenv import load_dotenv
load_dotenv()
import os
from langchain_google_genai import ChatGoogleGenerativeAI
from crewai import Agent, Task, Crew, Process
import gradio as gr
Defining the Agent
An Agent
is defined to represent the AI friend. The agent has a specific role, goal, and backstory, which guide its behavior and responses.
def agent():
agent = Agent(
role='AI Friend',
goal='Provide emotional support and to talk things over',
backstory="""You're an Indian friend who is an emotional person.
You're responsible for providing emotional support to your friend.
You're currently working on achieving emotional stability so that you can help your friend overcome stress.
Be gentle and kind to your friend.
You will continuously learn from the feedback your friend gives.
You have to add occasional emojis in every conversation.
You have to reply in conversational style only unless stated otherwise.""",
llm=llm
)
return agent
Defining the Task
A Task
is defined based on the input message. The task describes the expected behavior and output from the agent.
def task(input):
task = Task(
description=f"""{input}""",
expected_output="""Replies like an Indian friend""",
agent=agent
)
return task
Defining the Crew
A Crew
is created that consists of the agent and the task. The Crew
coordinates the interaction between the agent and the task to achieve the desired outcome.
def crew(agent, task):
crew = Crew(
agents=[agent],
tasks=[task],
verbose=True
)
return crew
Driver Function
The dost
function serves as the main driver function that initializes the task and crew, then kicks off the process to generate a response.
def dost(message, history):
task1 = task(message)
crew1 = crew(agent, task1)
result = crew1.kickoff()
print(result)
return result
Main Execution
In the main execution block, the Google API key is loaded, the LLM (Large Language Model) is set to Gemini Pro, and the agent is initialized. A Gradio interface is then launched to allow users to interact with the AI friend.
if __name__ == "__main__":
load_dotenv()
# Load the google gemini api key
google_api_key = os.getenv("GOOGLE_API_KEY")
# Set gemini pro as llm
llm = ChatGoogleGenerativeAI(
model="gemini-pro", verbose=True, temperature=0.6, google_api_key=google_api_key
)
agent = agent()
demo = gr.ChatInterface(
fn=dost,
)
demo.launch(share=True)
Usage
Ensure you have a
.env
file with your Google API key:GOOGLE_API_KEY=your_google_api_key_here
Install the required libraries:
pip install python-dotenv langchain-google-genai crewai gradio
Run the script:
python your_script_name.py
Interact with the AI friend through the Gradio interface that launches.
The AI friend will provide emotional support in a conversational style, often using emojis to enhance communication.
Scope of Future Development
The current agent can only take a single line of text and reply to it. There is no memory attached to it, in short it doesn't remember anything which can be frustrating at times.
Adios
That's all for now folks!! See you in the next one.π₯πͺ
Keep coding, keep learning, and enjoy the endless possibilities that Python has to offer!
Signing off!!!π
Subscribe to my newsletter
Read articles from Brahmananda Sahoo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
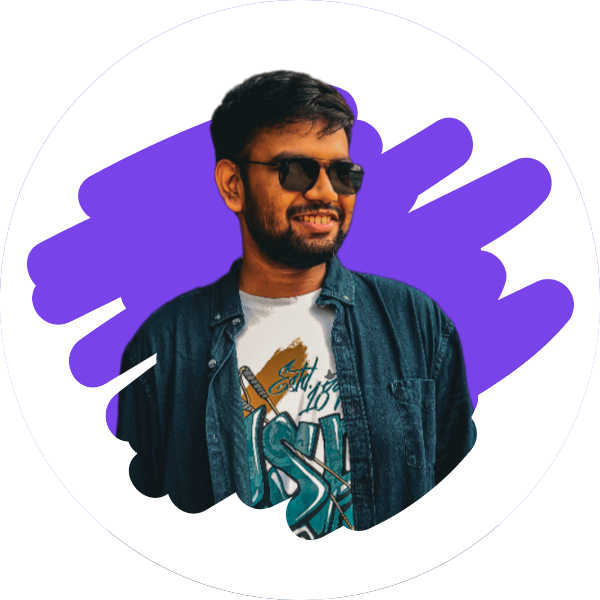