Detailed JavaScript learning roadmap with points and subpoints
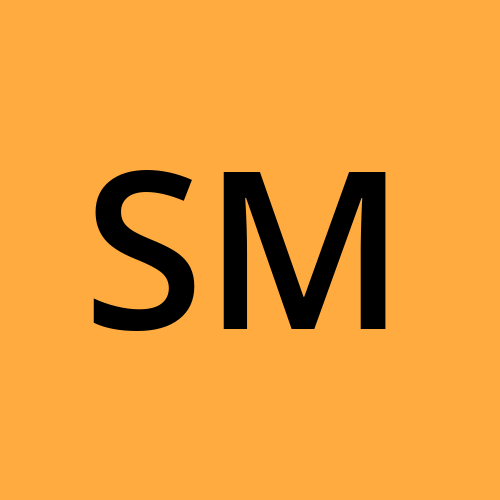
Table of contents
- Introduction
- Week 1-2: Fundamentals (20 hours)
- Week 3-4: Core JavaScript Concepts (20 hours)
- Week 5-6: Advanced JavaScript (20 hours)
- Week 7-8: Frameworks and Libraries (20 hours)
- Week 9-10: Tools and Testing (20 hours)
- Week 11-12: Performance Optimization (20 hours)
- Week 13-14: Advanced Topics (20 hours)
- Week 15-16: Advanced Topics (continued) (20 hours)
- Ongoing: Learning Resources and Practice

Introduction
JavaScript is a versatile and powerful language essential for web development. Whether you're a beginner or looking to deepen your understanding, following a structured learning path can significantly enhance your skills. This guide provides a comprehensive JavaScript roadmap (https://roadmap.sh/javascript), detailing what to learn, when to learn it, and how to progress efficiently.
Week 1-2: Fundamentals (20 hours)
Basics of Programming (10 hours)
Variables:
var
,let
,const
(2 hours)Data Types: String, Number, Boolean, Null, Undefined, Symbol (2 hours)
Operators: Arithmetic, Comparison, Logical, Assignment (2 hours)
Control Structures:
if
,else
,switch
(2 hours)Loops:
for
,while
,do-while
(2 hours)
Functions (10 hours)
Function Declaration and Expression (3 hours)
Arrow Functions (2 hours)
Function Scope and Closures (3 hours)
Higher-Order Functions and Callbacks (2 hours)
Week 3-4: Core JavaScript Concepts (20 hours)
DOM Manipulation (10 hours)
Selecting Elements:
getElementById
,querySelector
(2 hours)Changing Element Content and Attributes (2 hours)
Event Handling:
addEventListener
(2 hours)Creating and Removing Elements Dynamically (2 hours)
DOM Traversal (2 hours)
ES6+ Features (10 hours)
Template Literals (1 hour)
Destructuring (2 hours)
Spread and Rest Operators (2 hours)
Classes and Inheritance (3 hours)
Promises and
async/await
(2 hours)
Week 5-6: Advanced JavaScript (20 hours)
Asynchronous JavaScript (10 hours)
Callbacks (2 hours)
Promises (4 hours)
async/await
(4 hours)
JavaScript in the Browser (10 hours)
Browser APIs: Fetch, LocalStorage, SessionStorage (4 hours)
Understanding the Event Loop (3 hours)
Error Handling:
try
,catch
,finally
(3 hours)**
Week 7-8: Frameworks and Libraries (20 hours)
Frontend Frameworks (10 hours)
React.js: Components, State, Props, Hooks (5 hours)
Vue.js: Directives, Components, Vue CLI (3 hours)
Angular: Modules, Components, Services, Dependency Injection (2 hours)
Backend with Node.js (10 hours)
Setting up a Server (3 hours)
Working with Express.js (3 hours)
Middleware and Routing (2 hours)
Connecting to Databases: MongoDB, SQL (2 hours)
Week 9-10: Tools and Testing (20 hours)
Development Tools (10 hours)
Using npm/yarn (2 hours)
Code Linting with ESLint (2 hours)
Transpiling with Babel (2 hours)
Task Runners: Gulp (2 hours)
Module Bundlers: Webpack, Rollup (2 hours)
Testing (10 hours)
Unit Testing with Jest (4 hours)
End-to-End Testing with Cypress (4 hours)
Integration Testing (2 hours)
Week 11-12: Performance Optimization (20 hours)
Improving Load Times (10 hours)
Code Splitting (3 hours)
Lazy Loading (3 hours)
Caching Strategies (4 hours)
Optimizing Code (10 hours)
Debouncing and Throttling (3 hours)
Memory Leaks and Garbage Collection (3 hours)
Efficient DOM Manipulation (4 hours)
Week 13-14: Advanced Topics (20 hours)
TypeScript (10 hours)
Type Annotations (2 hours)
Interfaces and Types (3 hours)
Generics (2 hours)
Setting up a TypeScript Project (3 hours)
Progressive Web Apps (PWAs) (10 hours)
Service Workers (4 hours)
Web App Manifest (3 hours)
Offline Capabilities (3 hours)
Week 15-16: Advanced Topics (continued) (20 hours)
WebAssembly (10 hours)
Understanding WASM (4 hours)
Integrating WASM with JavaScript (6 hours)
Server-Side Rendering (SSR) (10 hours)
Next.js for React (5 hours)
Nuxt.js for Vue (5 hours)
Ongoing: Learning Resources and Practice
Books and Online Courses
"Eloquent JavaScript" by Marijn Haverbeke
"You Don't Know JS" by Kyle Simpson
FreeCodeCamp, Codecademy, Udemy courses
Practice Projects
Build small projects like to-do lists, weather apps, chat applications, etc.
Contribute to open-source projects on GitHub
Following this structured roadmap will help you master JavaScript efficiently and effectively. Happy coding!
Subscribe to my newsletter
Read articles from Saurabh Mehare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
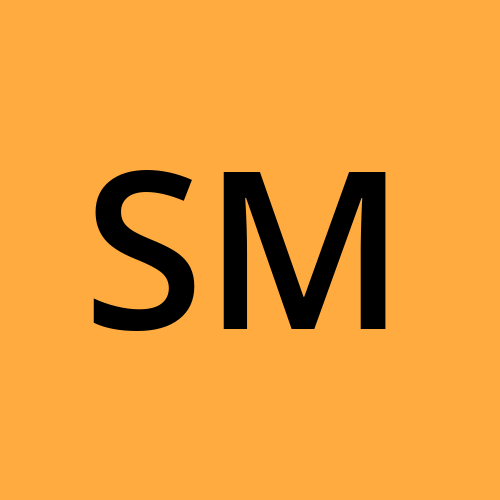