ArrayList in Java: A Comprehensive Guide
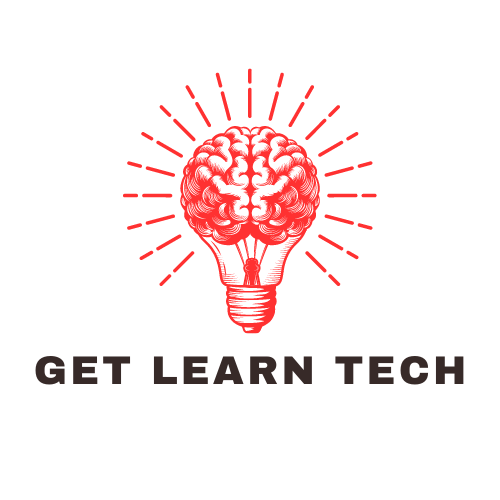
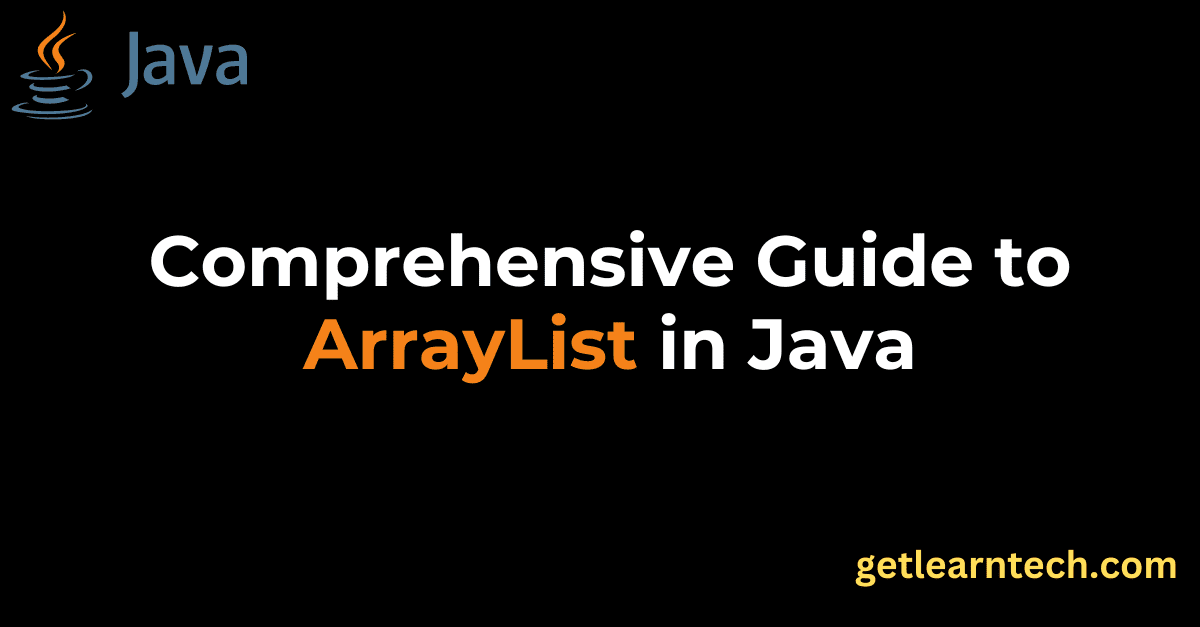
Java, a widely-used programming language, offers various data structures to manage collections of objects. Among these, ArrayList
stands out due to its dynamic nature and ease of use. In this article, we delve into the fundamentals of ArrayList
, its advantages, and how to effectively use it in your Java applications.
What is an ArrayList?
An ArrayList
in Java is a part of the java.util
package and provides a resizable array, also known as a dynamic array. Unlike standard arrays in Java, which have a fixed size, ArrayList
can grow and shrink dynamically as elements are added or removed. This flexibility makes it a popular choice for many Java developers.
Key Features of ArrayList
Dynamic Sizing: Unlike regular arrays,
ArrayList
can change size automatically.Non-Synchronized: By default,
ArrayList
is not thread-safe, which means it's not synchronized.Random Access: Provides fast access to elements using indexes.
Allows Duplicates:
ArrayList
allows storing duplicate elements.Maintains Insertion Order: Elements are maintained in the order they were added.
How to Use ArrayList in Java
Importing ArrayList
Before using ArrayList
, you need to import the java.util.ArrayList
package.
import java.util.ArrayList;
Creating an ArrayList
To create an ArrayList
, you simply instantiate it using its constructor.
ArrayList<String> list = new ArrayList<>();
Adding Elements
Use the add()
method to insert elements into the ArrayList
.
list.add("Apple");
list.add("Banana");
Accessing Elements
Access elements using the get()
method, which takes an index as an argument.
String fruit = list.get(0); // "Apple"
Modifying Elements
To modify elements, use the set()
method.
list.set(1, "Orange"); // Changes "Banana" to "Orange"
Removing Elements
Remove elements using the remove()
method, which can take either an index or an object as an argument.
list.remove(0); // Removes "Apple"
list.remove("Orange"); // Removes "Orange"
Iterating Over Elements
You can iterate over the elements in an ArrayList
using various methods, such as a for
loop, enhanced for
loop, or an Iterator
.
for (String fruit : list) {
System.out.println(fruit);
}
Size of the ArrayList
To get the number of elements in the ArrayList
, use the size()
method.
int size = list.size();
Advantages of Using ArrayList
Flexibility: Can dynamically grow and shrink.
Ease of Use: Provides methods to manipulate the data easily.
Performance: Offers O(1) time complexity for access operations.
Integration: Works seamlessly with Java's Collection Framework.
When to Use ArrayList
Dynamic Arrays: When you need a resizable array structure.
Random Access: When you require frequent access to elements by index.
Order Maintenance: When you need to maintain the order of insertion.
Limitations of ArrayList
Performance Overhead: Frequent resizing can lead to performance overhead.
Non-Synchronized: Not suitable for concurrent operations without external synchronization.
Memory Consumption: May consume more memory compared to linked lists for large datasets.
Conclusion
ArrayList
is an integral part of Java's Collection Framework, offering a robust and flexible solution for managing dynamic arrays. Its simplicity and efficiency make it a go-to choice for developers. By understanding its features, methods, and appropriate use cases, you can leverage ArrayList
to write more efficient and maintainable Java code.
Explore ArrayList
in your next Java project and experience the convenience of dynamic arrays!
For further reading, refer to the official Java Documentation on ArrayList
.
Subscribe to my newsletter
Read articles from getlearntech directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
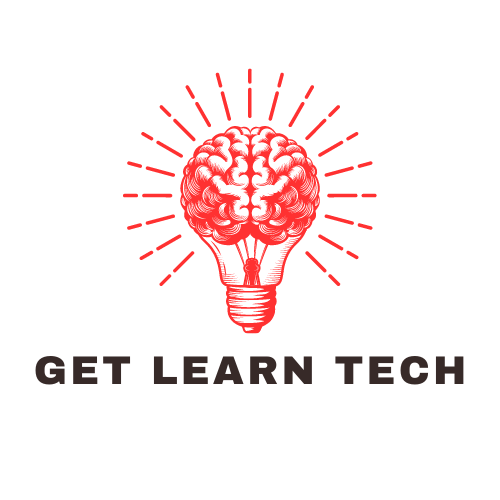
getlearntech
getlearntech
Welcome to Get Learn Tech! It's the perfect spot for developers who want to learn programming and get ready for interviews. Whether you're a beginner starting from scratch or an experienced coder wanting to polish your skills, this is where you belong.