Taming the Queue Beast: Mastering Background Jobs with Laravel Horizon
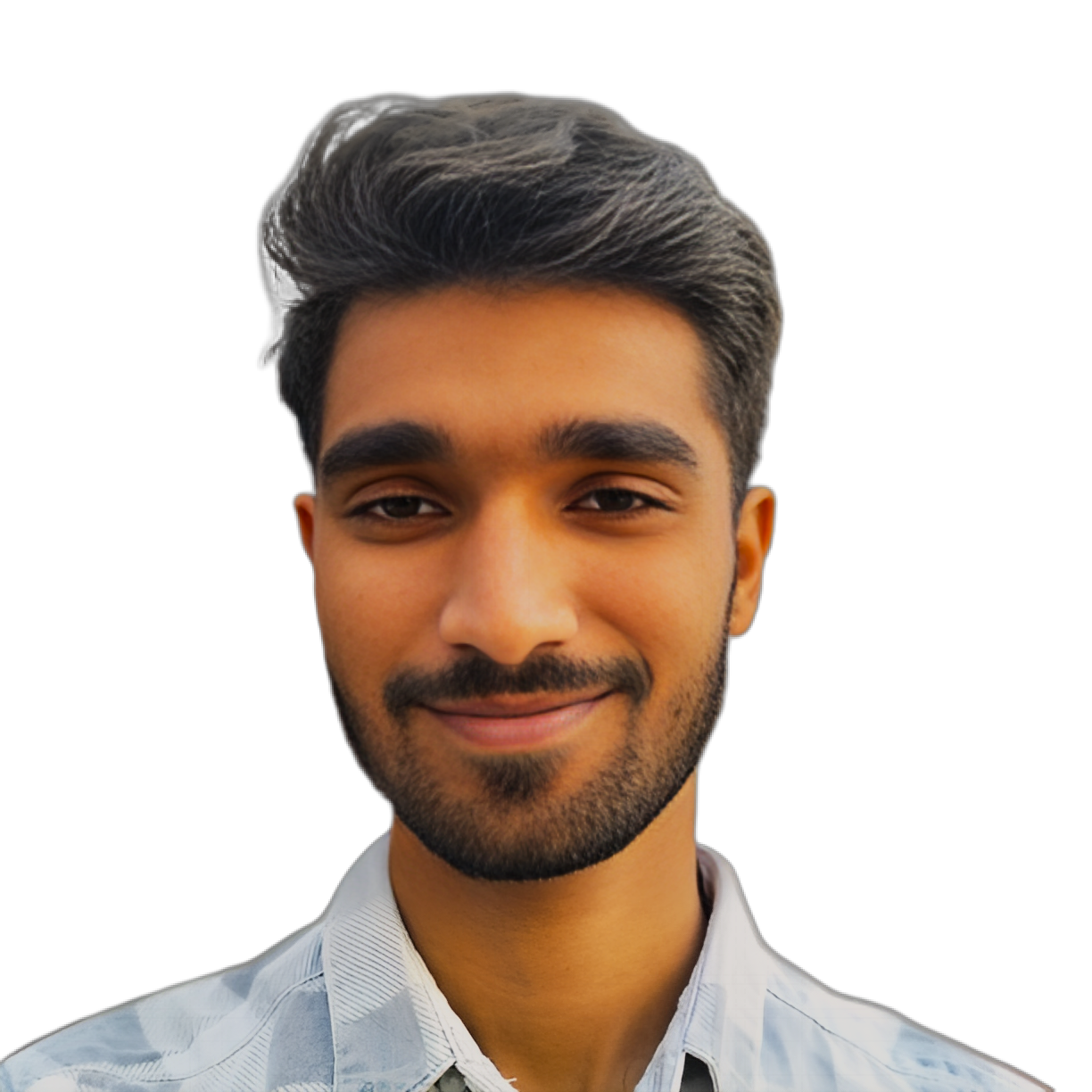
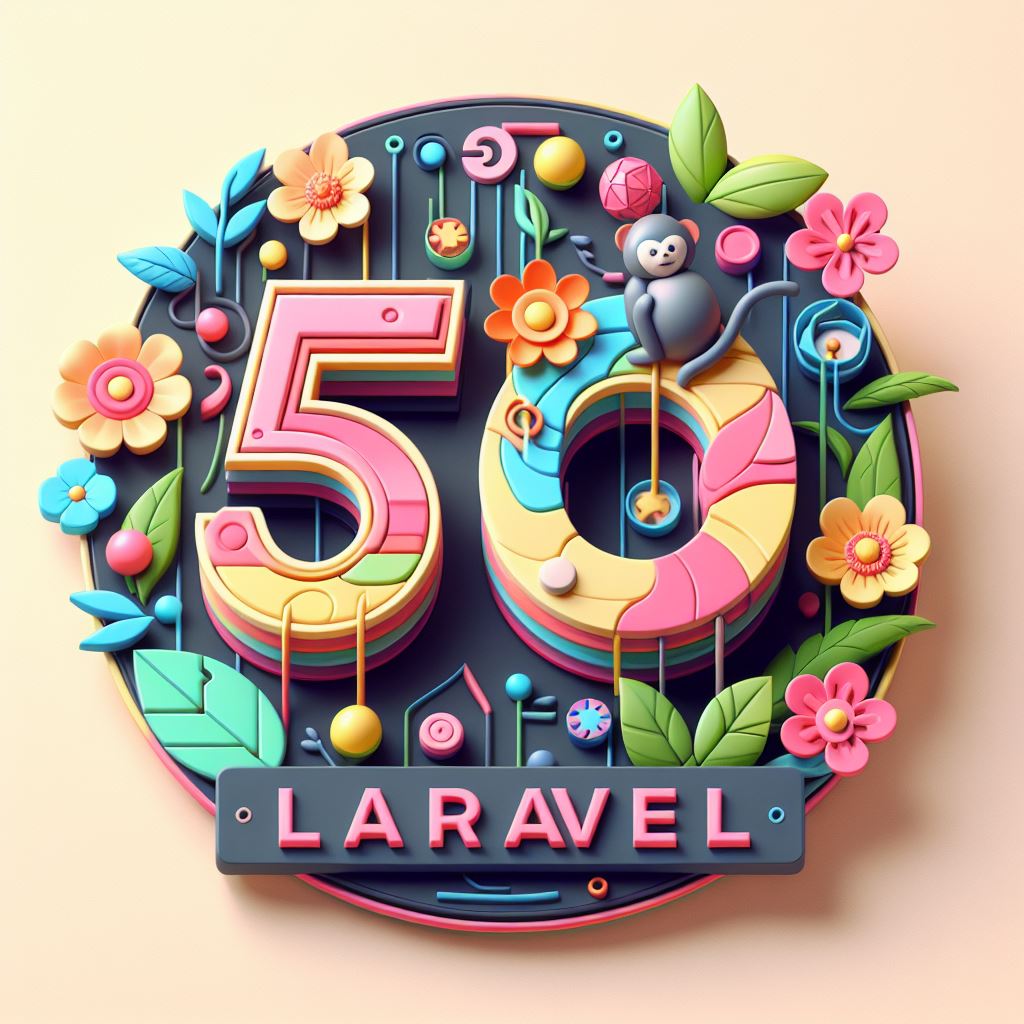
In the bustling world of web applications, background jobs are the unsung heroes that handle asynchronous tasks, keeping your app responsive and efficient. But managing these jobs, especially as your application scales, can quickly become a juggling act. Enter Laravel Horizon, your knight in shining armor for queue management in Laravel.
What is Laravel Horizon?
Laravel Horizon is a must-have tool for any Laravel developer looking to streamline and visualize background job processing. It offers a beautifully designed dashboard that grants you real-time insights into your queues, providing invaluable information like:
Queue Status: See which queues are currently processing jobs, giving you a clear overview of your application's workload.
Job Performance: Gain insights into how long jobs are taking to execute, helping you identify potential bottlenecks and optimize performance.
Failure Tracking: Quickly identify and troubleshoot any failed jobs that might be causing issues in your application.
Benefits of Using Laravel Horizon
Enhanced Visibility: Gain a bird's-eye view of your background jobs, allowing you to make informed decisions about queue management and resource allocation.
Effortless Debugging: No more digging through cryptic logs! Horizon's intuitive dashboard makes it easy to pinpoint the root cause of failed jobs, saving you precious time and frustration.
Simplified Scaling: As your application grows, Horizon empowers you to effortlessly adjust worker processes (the processes that execute jobs) to handle increased queue volume, ensuring optimal performance.
Streamlined Collaboration: With worker configuration stored in a central, source-controlled file, your entire team can stay in sync and collaborate effectively on queue-related tasks.
Getting Started with Laravel Horizon
1. Installation:
Let's get Horizon up and running in your Laravel project! Open a terminal and navigate to your project's root directory. Then, run the following Composer command:
Bash
composer require laravel/horizon
2. Configuration (Optional):
While Horizon works great out of the box with Redis as the default queue connection, you can customize certain settings in the config/horizon.php
file. Here are some common adjustments:
Supervisor: Define supervisor configuration details if you're using a supervisor process manager.
Metrics: Configure the metrics driver (e.g., Prometheus) for detailed performance monitoring.
Queue Configuration: Specify queues and their worker processes to tailor Horizon's behavior to your specific application needs.
3. Artisan Commands:
Now it's time to unleash the power of Horizon! Use the following Artisan commands to start and manage Horizon:
php artisan horizon:start
: Starts the Horizon dashboard and worker processes.php artisan horizon:terminate
: Gracefully stops the Horizon processes.php artisan horizon:cleanup
: Removes any orphaned Horizon processes.
4. Accessing the Dashboard:
Once Horizon is running, open your web browser and navigate to the URL http://localhost:8000/horizon
(replace localhost:8000
with your actual application URL if needed). You'll be greeted by the Horizon dashboard, ready to provide you with valuable insights into your background jobs.
Code Example: Enqueuing a Job for Processing
Let's see how to enqueue a simple job for processing in Laravel using Horizon. Here's an example job class:
PHP
<?php
namespace App\Jobs;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Foundation\Bus\Dispatchable;
use Illuminate\Queue\InteractsWithQueue;
use Illuminate\Queue\SerializesModels;
class SendWelcomeEmail implements ShouldQueue
{
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
protected $user;
/**
* Create a new job instance.
*
* @param \App\Models\User $user
* @return void
*/
public function __construct(\App\Models\User $user)
{
$this->user = $user;
}
/**
* Execute the job.
*
* @return void
*/
public function handle()
{
// Send a welcome email to the user
// ... (your email sending logic)
}
}
To enqueue this job, use the dispatch
method on your job class:
PHP
SendWelcomeEmail::dispatch($user);
Monitoring Job Progress in Horizon:
Once you've enqueued jobs, the Horizon dashboard will display them in real-time. Here's what you can see:
Pending Jobs: These jobs are waiting to be processed by a worker.
Running Jobs: These jobs are currently being executed by a worker.
Failed Jobs: These jobs encountered an error during execution and need attention.
Monitored Jobs: Jobs added through Horizon's UI for monitoring purposes.
By clicking on a specific job, you can delve deeper into its details, including:
Job class name
Queue name
Date and time of enqueuing
Execution time (if applicable)
Stack trace of any errors (for failed jobs)
This detailed information empowers you to effectively manage your background jobs and ensure smooth application operation.
Advanced Horizon Features:
Beyond basic monitoring, Horizon offers some powerful features to further enhance your queue management experience:
Supervising Workers: Easily view and manage worker processes directly from the Horizon dashboard.
Supervisory Configuration: If you're using a process supervisor like Supervisor or Laravel Forge, you can configure Horizon to integrate seamlessly.
Metrics Integration: Integrate Horizon with metrics drivers like Prometheus for detailed performance tracking and analysis.
Monitoring Queues from Different Applications: You can even configure Horizon to manage queues from multiple Laravel applications running on the same server.
Conclusion
Laravel Horizon is an invaluable tool for any Laravel developer looking to tame the complexity of background jobs. Its intuitive interface and powerful features provide comprehensive visibility, streamline debugging, and simplify scaling. By embracing Horizon, you can ensure your Laravel application remains efficient and responsive as your queue volume grows.
Additional Tips:
Consider Error Handling: Implement proper error handling mechanisms within your jobs to gracefully handle exceptions and prevent cascading failures.
Monitor Job Retries: Configure job retries in your queue configuration to automatically retry failed jobs a certain number of times before permanently marking them as failed.
Optimize Worker Processes: Experiment with the number of worker processes to find the optimal balance for your application's workload and resource availability.
With these tips and Laravel Horizon at your disposal, you can confidently manage your background jobs and take your Laravel application's performance to the next level!
Subscribe to my newsletter
Read articles from Asis Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
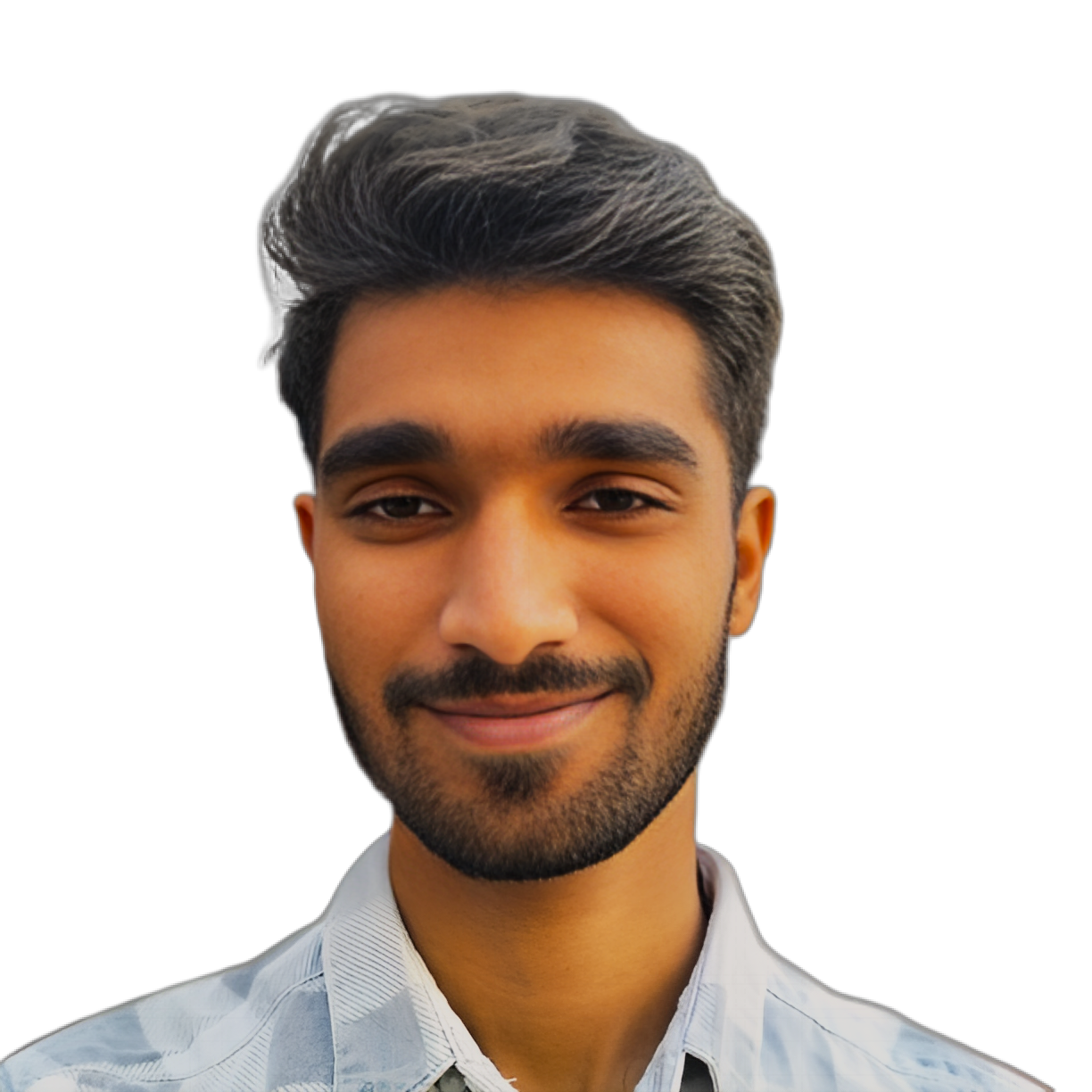
Asis Sharma
Asis Sharma
I'm an ambitious and motivated software developer, eager to apply my knowledge and skills to contribute to the development of innovative and cutting-edge software solutions. I'm well-versed in a variety of programming languages, including Javascript, C++, and Node.js, and have a understanding of object-oriented programming and algorithms. I'm passionate about developing and I am eager to learn and collaborate with others.