Beginner's Guide to NestJs Framework
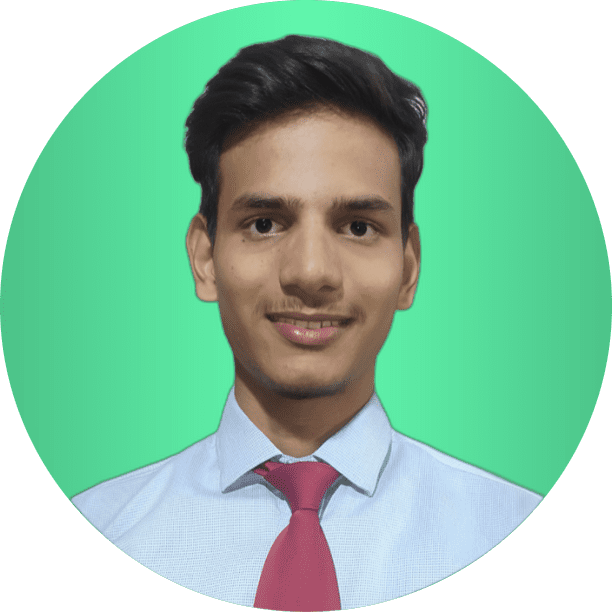
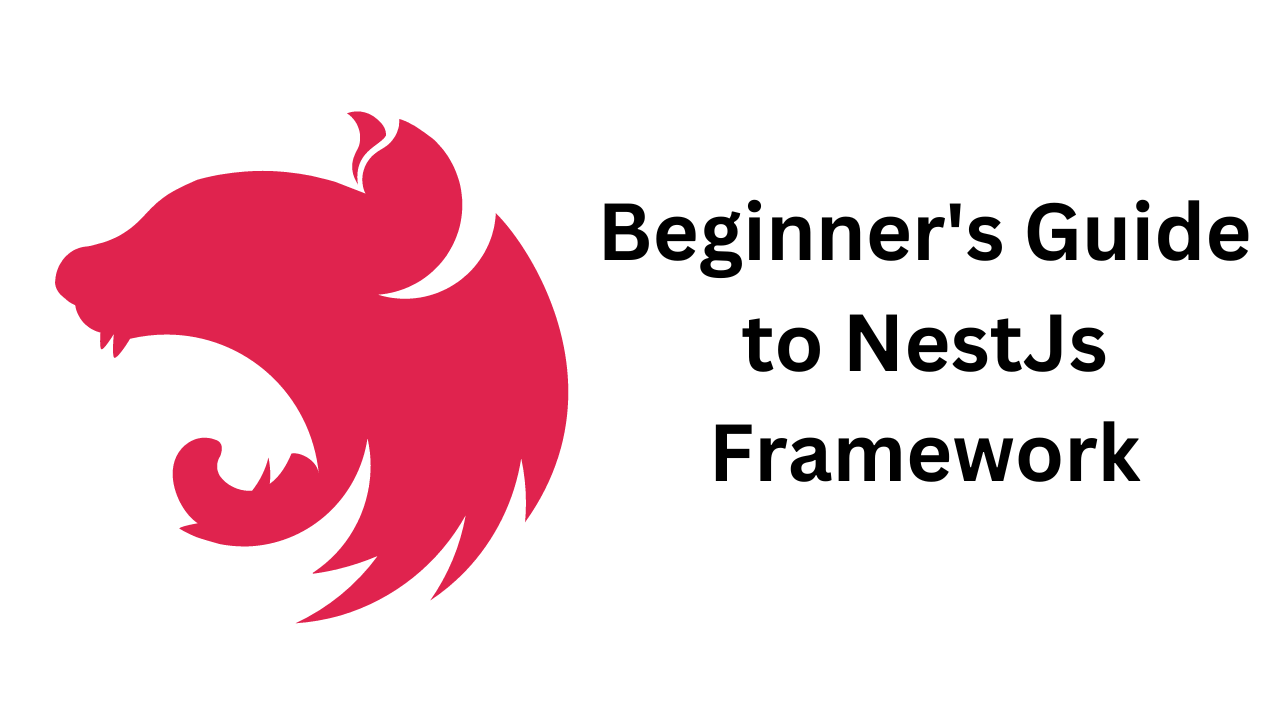
Introduction
Among the myriad of options available, NestJS has emerged as a standout choice for building efficient, reliable, and scalable server-side applications. This article will explore what NestJS is, its core features, and why developers should consider using it for their next project.
What is NestJS?
NestJS is a progressive Node.js framework for building server-side applications. It is built with TypeScript, leveraging the latest JavaScript features and bringing a strongly typed and modern approach to Node.js development. NestJS aims to provide an out-of-the-box application architecture that helps developers build and maintain server-side applications more effectively.
NestJS stands out for several reasons:
TypeScript First: While it supports JavaScript, NestJS is designed to work seamlessly with TypeScript, offering type safety and advanced IDE support.
Modular Architecture: Inspired by Angular, NestJS promotes a modular architecture, enabling developers to create highly testable, scalable, and loosely coupled applications.
Dependency Injection: NestJS uses a powerful and flexible dependency injection system, making managing the application's dependencies easier.
Extensive Ecosystem: With support for various libraries and tools, NestJS integrates well with popular databases, testing frameworks, and other essential tools.
Decorators and Metadata: Utilizing TypeScript decorators, NestJS provides a declarative approach to defining routes, middleware, and other components, enhancing code readability and maintainability.
Core Features of NestJS
TypeScript Support: TypeScript brings static typing to JavaScript, which helps catch errors early in development, leading to more robust and maintainable code. NestJS is built with TypeScript, providing built-in support and encouraging best practices.
Modular Architecture: NestJS encourages a modular structure, where each module encapsulates related components such as controllers, services, and repositories. This modularity facilitates code organization, reusability, and scalability, making it easier to manage large applications.
Dependency Injection: The built-in dependency injection system allows developers to manage dependencies efficiently, promoting loose coupling and enhancing testability. It also simplifies the creation and management of service instances.
Decorators and Metadata: NestJS utilizes TypeScript decorators for defining routes, middleware, guards, pipes, and interceptors. This approach provides a clean and declarative way to configure application components, improving code readability and maintainability.
Rich Ecosystem: NestJS seamlessly integrates with various libraries and frameworks, including TypeORM, Mongoose, GraphQL, and WebSockets. This rich ecosystem ensures that developers have the tools they need to build comprehensive applications without reinventing the wheel.
Scalability and Performance: Designed with scalability in mind, NestJS can handle applications of all sizes, from small projects to enterprise-level applications. Its architecture and design principles ensure that applications remain performant and responsive under heavy load.
Why use Nestjs over Nodejs
NestJS is a progressive Node.js framework designed for building efficient, reliable, and scalable server-side applications. Here's a detailed comparison and explanation of why you might choose NestJS over plain Node.js:
1. Architectural Patterns and Best Practices
NestJS:
Modular Architecture: Encourages splitting applications into modules, which helps in organizing code and improving maintainability.
Dependency Injection: Facilitates better code organization and testing by injecting dependencies rather than hardcoding them.
MVC Pattern: Provides a Model-View-Controller structure, which is a widely adopted design pattern that separates concerns within the application.
Plain Node.js:
No inherent structure or patterns; developers need to set up and enforce their architectural patterns.
Dependency injection and modularization are not built-in; developers need to implement their solutions or use third-party libraries.
2. Built-in Features and Libraries
NestJS:
Comprehensive CLI: The Command Line Interface (CLI) speeds up the development process by generating boilerplate code and managing tasks.
Out-of-the-box Support for TypeScript: Provides a more robust development experience with strong typing, modern JavaScript features, and better tooling support.
Integrated Middleware and Guards: Simplifies the implementation of authentication, authorization, and other cross-cutting concerns.
Support for WebSockets and GraphQL: Built-in modules for handling real-time communication and GraphQL APIs.
Plain Node.js:
Requires manual setup and configuration of tools like TypeScript.
Middleware and other features like authentication need to be manually integrated, often requiring several third-party libraries.
Developers need to manually set up WebSocket or GraphQL support.
3. Performance and Scalability
NestJS:
Efficient Request Handling: Uses Express.js or Fastify under the hood, allowing for high-performance request handling.
Microservices Support: Built-in support for creating microservice architecture, enabling the development of scalable and distributed systems.
Plain Node.js:
Performance depends on how well the developer implements request handling and optimizes the application.
Microservices architecture must be set up manually, requiring more effort and expertise.
4. Community and Ecosystem
NestJS:
Active Community and Ecosystem: A growing community with extensive documentation, tutorials, and third-party modules.
Enterprise Support: Increasing adoption by enterprises for large-scale applications due to its structured approach and maintainability.
Plain Node.js:
Larger overall ecosystem, but less structured; finding high-quality modules and ensuring their compatibility can be more challenging.
Community and resources are vast but can vary greatly in quality and relevance.
5. Testing and Maintenance
NestJS:
Testing: Built-in support for unit and end-to-end testing using popular frameworks like Jest.
Maintainability: A structured codebase with clear patterns makes maintenance and scaling easier.
Plain Node.js:
Testing is not built-in; developers must choose and configure their own testing frameworks.
Maintainability depends on how well the initial architecture and code organization are implemented.
6. Learning Curve
NestJS:
Steeper Initial Learning Curve: Due to its comprehensive set of features and architectural patterns, it might take longer for developers to learn initially.
Long-term Productivity: Once learned, the structured approach and built-in features can significantly enhance long-term productivity.
Plain Node.js:
Gentler Learning Curve: Easier to get started with for small projects due to its simplicity.
Potential for Complexity: As the project grows, the lack of structure can lead to increased complexity and technical debt.
Key Components in Nestjs
The key components in NestJS: are controllers, entities, modules, services, pipes, and DTOs. These components are crucial in building well-structured and maintainable applications with NestJS.
Controllers: Handle incoming requests and return responses.
Entities: Represent the data model and interact with the database.
Modules: Group related components together and manage dependencies.
Services: Contain business logic and handle data operations.
Pipes: Transform and validate data in request handling.
DTOs: Define and validate the shape of data transferred over the network.
By using these components, NestJS promotes a highly modular, maintainable, and scalable application architecture, allowing developers to build robust server-side applications efficiently.
1. Controllers
Role: Controllers in NestJS are responsible for handling incoming requests and returning responses to the client. They define the routes (endpoints) and contain the business logic that gets executed when a specific route is accessed.
Features:
Routing: Controllers map HTTP requests to specific handler methods using decorators like
@Get()
,@Post()
,@Put()
,@Delete()
, etc.Request Handling: They process incoming data, call the appropriate service methods, and send back the response.
Example:
import { Module } from '@nestjs/common';
import { UsersController } from './users.controller';
import { UsersService } from './users.service';
import { TypeOrmModule } from '@nestjs/typeorm';
import { User } from './user.entity';
@Module({
imports: [TypeOrmModule.forFeature([User])],
controllers: [UsersController],
providers: [UsersService],
})
export class UsersModule {}
2. Services
Role: Services contain the business logic of the application and are typically used to handle data operations, such as querying the database, processing data, and implementing complex logic.
Features:
Reusability: Services can be injected into controllers or other services.
Business Logic: Encapsulate the core functionalities of the application.
Example:
import { Injectable } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository } from 'typeorm';
import { User } from './user.entity';
import { CreateUserDto } from './dto/create-user.dto';
@Injectable()
export class UsersService {
constructor(
@InjectRepository(User)
private usersRepository: Repository<User>,
) {}
findAll(): Promise<User[]> {
return this.usersRepository.find();
}
create(createUserDto: CreateUserDto): Promise<User> {
const user = this.usersRepository.create(createUserDto);
return this.usersRepository.save(user);
}
findOne(id: string): Promise<User> {
return this.usersRepository.findOne(id);
}
}
3. Pipes
Role: Pipes are used for transforming and validating data. They can be applied to route handlers to process incoming requests before they reach the controller.
Features:
Transformation: Modify the data before passing it to the handler.
Validation: Ensure the incoming data meets certain criteria.
Example:
import { PipeTransform, Injectable, ArgumentMetadata, BadRequestException } from '@nestjs/common';
@Injectable()
export class ParseIntPipe implements PipeTransform<string, number> {
transform(value: string, metadata: ArgumentMetadata): number {
const val = parseInt(value, 10);
if (isNaN(val)) {
throw new BadRequestException('Validation failed');
}
return val;
}
}
// Usage in a controller
@Get(':id')
findOne(@Param('id', ParseIntPipe) id: number) {
return this.usersService.findOne(id);
}
4. DTO (Data Transfer Object)
Role: DTOs are used to define the shape of data that is sent over the network. They help in validating and transforming the data that comes into the application.
Features:
Validation: Use class-validator decorators to enforce data constraints.
Typing: Provide a structured way to handle data with TypeScript.
Example:
import { IsString, IsEmail, IsNotEmpty } from 'class-validator';
export class CreateUserDto {
@IsString()
@IsNotEmpty()
readonly name: string;
@IsEmail()
readonly email: string;
@IsString()
@IsNotEmpty()
readonly password: string;
}
// Usage in a controller
@Post()
create(@Body() createUserDto: CreateUserDto) {
return this.usersService.create(createUserDto);
}
Conclusion
NestJS offers a robust framework for building server-side applications, leveraging the power of TypeScript, modular architecture, and a rich ecosystem of tools and libraries. By providing built-in support for key features such as dependency injection, decorators, and TypeScript, NestJS ensures that developers can create scalable, maintainable, and high-performance applications efficiently.
The detailed comparison with plain Node.js highlights NestJS's advantages in terms of architectural patterns, built-in features, scalability, and maintainability. NestJS not only facilitates the development of complex applications but also enhances productivity and long-term maintainability.
Key components like controllers, entities, modules, services, pipes, and DTOs form the backbone of NestJS applications, promoting a well-organized and modular structure. These components ensure that developers can handle requests, manage data, and validate inputs effectively, leading to robust and reliable server-side solutions.
Overall, NestJS stands out as a comprehensive framework that simplifies the development process while providing the flexibility and scalability needed for modern web applications. For developers seeking a structured, efficient, and scalable solution for building server-side applications, NestJS is an excellent choice.
Subscribe to my newsletter
Read articles from Hitesh Mishra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
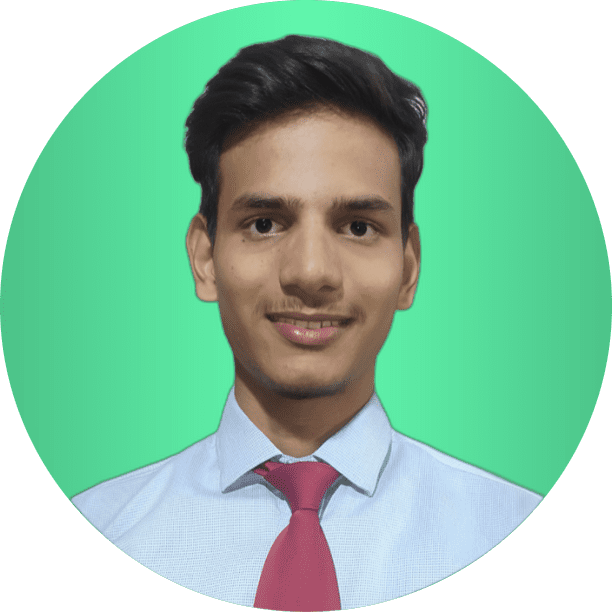
Hitesh Mishra
Hitesh Mishra
I am Hitesh Mishra, currently pursuing my bachelor of technology in computer science engineering from Graphic Era Hill University, Dehradun. I am always ready to have new experiences, meet new people and learn new things. 🔹Passionate about coding(problem solving) and web development. Currently learning web development. I have also created some projects like a full stack ecommerce website, full blog in which a user can create, edit, delete and update blog posts, forkfiy project in which users can find a recipe and instructions on how to make them! 🔹Currently learning ReactJS 🔹SKILLS: HTML, CSS, JAVASCRIPT, NODEJS, EXPRESSJS, MYSQL, MONGODB, C, C++. 🔹Check out my GitHub profile: https://github.com/hiteshmishra2103/ 🔹Linkedin: https://www.linkedin.com/in/hiteshmishra21/ Want to contact me?.📞 Just leave me a message on linkedin.