Learn Python Programming: A beginner's Guide
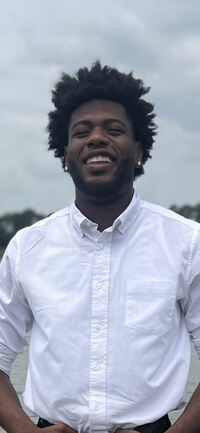

When I first encountered Python, it felt like deciphering an ancient language. Yet, it quickly revealed its elegant simplicity, akin to learning a straightforward recipe.
Why Python?
Because Python combines readability with power, making it an ideal choice for both novices and experts.
Getting Started with Python
Starting with Python requires installing the interpreter, which translates your code into machine language. You can download it from the official Python website. After installation, verifying the setup by running python --version
in your command line ensures everything is correctly configured.
Installing Python
To get started, you need to install Python on your system.
Python has an extensive library collection, making it suitable for a variety of development tasks.
Visit the official Python website to download the latest version. The installation process is straightforward, with a guided setup that caters to most operating systems, including Windows, macOS, and Linux.
After downloading and running the installer, verify the installation by opening your terminal and executing python --version
. This command confirms that Python is correctly installed and will display the version number.
Setting Up IDE
Choosing the right Integrated Development Environment (IDE) can vastly improve your efficiency and coding experience.
PyCharm: Feature-rich, with support for web development.
Visual Studio Code (VS Code): Lightweight, highly customizable through extensions.
Atom: Open-source IDE with a vast library of packages.
Sublime Text: Known for its speed and simplicity.
Each IDE offers unique features and benefits, so evaluate them based on your specific needs and project requirements.
Consider IDEs with strong community support and regular updates to stay up-to-date with the latest Python advancements.
Basic Python Syntax
Understanding Python's basic syntax is foundational for writing error-free code that executes as expected.
In Python, each statement typically occupies one line and indentation (usually 4 spaces) is used to define code blocks, unlike curly braces {}
in many other languages.
Keywords such as if
, for
, and while
are essential to control the flow of your program.
Variables and Data Types
Variables in Python act as placeholders for storing data that can be referenced and manipulated.
A variable is created by assigning a value to it with the =
operator. For example, x = 5
assigns the integer 5
to the variable x
.
Python supports various data types including integers (int
), floating-point numbers (float
), strings (str
), and booleans (bool
).
For instance:
codex = 5 # int
y = 3.14 # float
name = "Alice" # str
is_student = True # bool
Understanding these data types and how to use them effectively is crucial for writing robust and flexible Python code.
Basic Operators
Operators are essential in Python programming.
Python's operators can be categorized into several types. These include:
Arithmetic operators:
+
,-
,*
,/
Comparison operators:
==
,!=
,<
,>
,<=
,>=
Logical operators:
and
,or
,not
Assignment operators:
=
,+=
,-=
,*=
,/=
These operators serve as the building blocks for performing various operations within your code, enabling you to manipulate data and execute logic.
Each category serves a distinct purpose.
For example, arithmetic operators are used to perform basic mathematical operations. Logical operators are pivotal in decision-making processes by allowing boolean logic tests.
Having a strong grasp of these operators will significantly enhance your ability to write efficient Python code. Understanding how to use them not only simplifies complex expressions but also contributes to producing more readable and maintainable code.
Control Flow
Control flow dictates the order in which code executes. It is foundational for creating dynamic, multi-functional programs.
In Python, control flow is managed using constructs such as if
statements for conditional execution, for
and while
loops for iteration, and functions for creating reusable blocks of code. Understanding these constructs is crucial for writing effective Python programs that can handle various tasks dynamically.
The terms “control flow” and “flow of control” are often used interchangeably.
Conditional Statements
Conditional statements allow Python programs to make decisions and execute code based on certain conditions. They are essential for implementing logic and controlling the flow of a program.
Python uses if
, elif
, and else
statements for conditional decision-making.
The if
statement evaluates a given condition, and if the condition is true, the associated code block is executed.
If the condition in the if
statement isn't met, the elif
(else if) statement can check additional conditions.
Alternatively, an else
statement can provide a fallback option, executed when none of the previous conditions are met.
codex = 10
if x > 5:
print("x is greater than 5")
elif x == 5:
print("x is 5")
else:
print("x is less than 5")
Mastering conditional statements is pivotal for creating responsive and intelligent Python applications. They are used everywhere, from simple scripts to complex systems, making them an indispensable part of the language.
For further depth, review the official Python documentation on control flow for comprehensive examples and advanced techniques.
Loops
In Python, loops are used to execute a block of code repeatedly as long as a specified condition is met.
for
loop: Iterates over a sequence (like a list or string) and executes a block of code for each element.while
loop: Repeatedly executes a block of code as long as the given condition remains true.Nested loops: Allows embedding of one loop within another to handle complex iterating tasks.
# For loop example
for i in range(5):
print(i)
# While loop example
count = 0
while count < 5:
print(count)
count += 1
Loops are fundamental for tasks that involve repetitive actions, such as traversing data structures.
Mastering loops enhances efficiency in coding, automating repetitive tasks seamlessly.
Functions and Modules
Functions are blocks of reusable code designed to perform a specific task, created using the def
keyword. They enable modular programming, improving code readability and maintainability.
codedef greet(name):
return f"Hello, {name}!"
print(greet("Alice"))
Modules are files containing Python code: functions, variables, and classes. By importing modules, you can leverage pre-written code, promoting code reuse and organization.
code# Importing a module example
import math
print(math.sqrt(16))
Using functions and modules efficiently is fundamental to creating scalable and maintainable Python applications, while also promoting a clear separation of concerns within your codebase.g Functions
In Python, defining functions is a cornerstone of creating organized, reusable code.
Functions are defined using the keyword followed by the function name and parentheses. Inside the parentheses, you can specify parameters which act as placeholders for the data inputs. The indented block following the colon is the function body, where the actual code resides.
Functions can return a value using the statement, but it's not mandatory. When a function is invoked, the code inside its body executes, leveraging the input parameters if any are provided.
Defining functions promotes modularity and enhances code readability. Functions can encapsulate specific tasks, making it easier to debug, extend, and understand code. Additionally, well-defined functions facilitate testing and reusability, ultimately contributing to more efficient and maintainable Python programs.
Importing Modules
In Python, modules are simply Python files containing definitions and statements.
Basic Import: Use the keyword to bring an entire module into your script.
From Import: Use to import specific objects from a module.
Alias Import: Use to assign an alias to a module for ease of use.
Wildcard Import: Use to import everything from a module, though this is generally discouraged.
Modules provide a way to logically organize your Python code by functionality.
Organized code with modules enhances maintainability and readability of complex projects.
Connect with Me
Stay updated with my journey and connect with me on social media:
LinkedIn: Fradley Joseph
Twitter/X: @FradleyPrograms
Reddit: u/NeverBackDrown
Feel free to reach out, share your own coding experiences, and join the conversation!
Subscribe to my newsletter
Read articles from Fradley Joseph directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
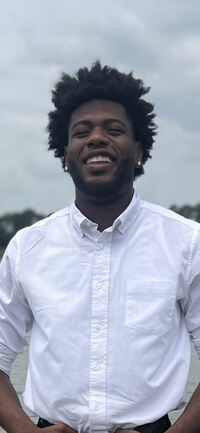