Understanding React Context: A Kid-Friendly Guide
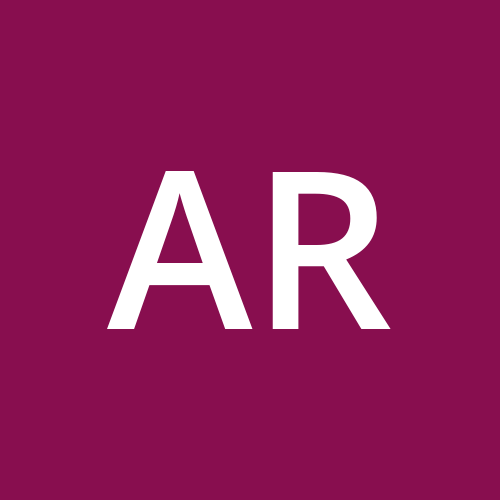
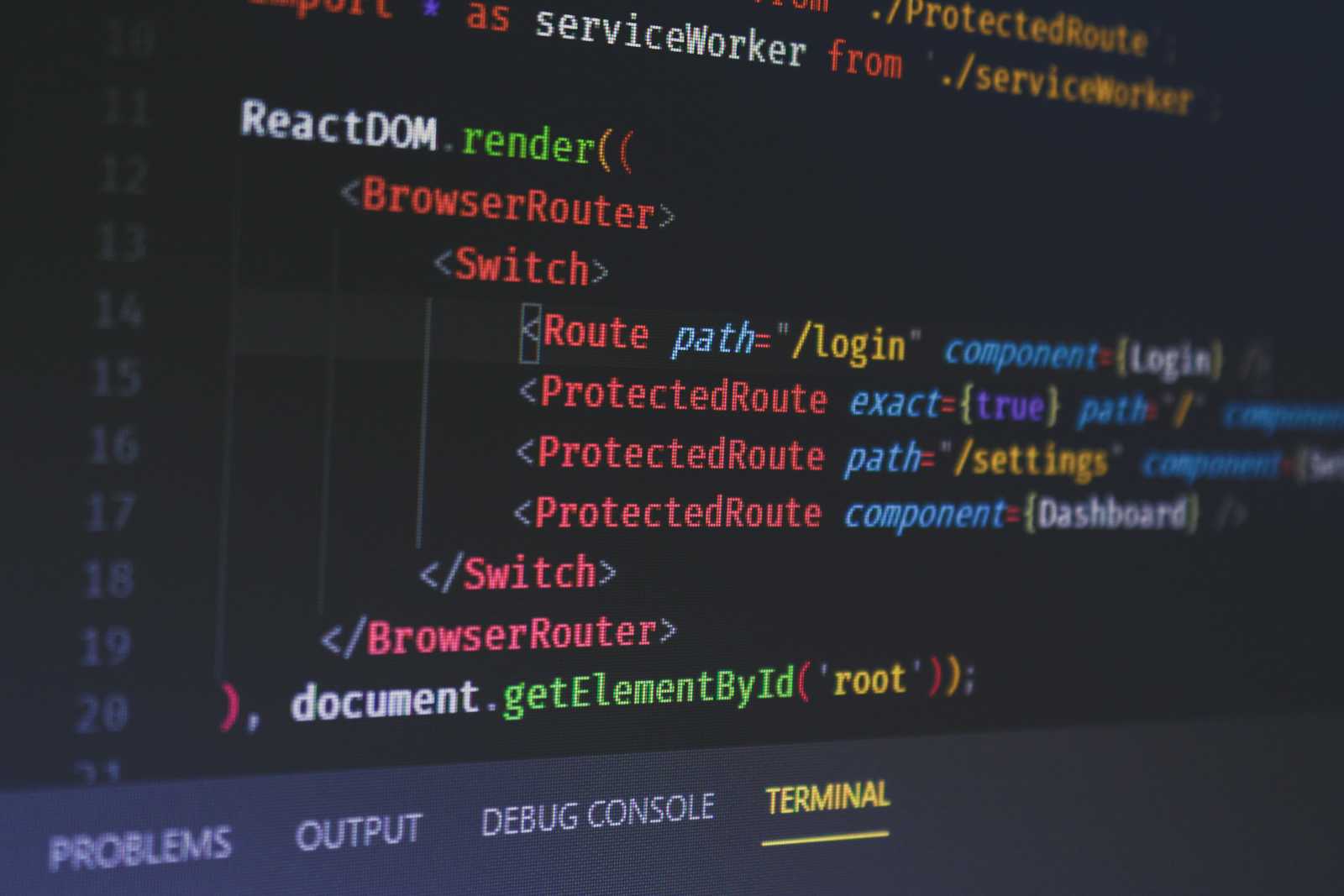
Sometimes in a React app, you have data that many components need to access. Passing this data through props can become cumbersome and messy. Imagine you have a big family and you need to pass a message to every member individually. It would take a lot of time and effort, right? This is what happens in a React app when you pass data through props.
React Context helps by providing a way to share data without props. It's like having a family notice board where you can pin a message, and everyone in the family can read it whenever they need to. This makes sharing information much easier and more efficient.
In this blog post, we'll explore what React Context is, why it's useful, and how to use it in your React applications. We'll break it down into simple terms and provide examples to help you understand how to implement and benefit from React Context. Whether you're new to React or looking to simplify your state management, this guide will help you get started with React Context in no time.
Understanding React Context
Imagine you have a special storage box in your house where you keep your favorite snacks. Instead of giving a snack to each family member individually, you put the snacks in this storage box. Now, whenever someone in the family wants a snack, they can go to the box and take what they need. This way, everyone knows where to find the snacks, and you don't have to run around distributing them.
In a React application, React Context works similarly. It's a way to create a central place where you can store data (like the snacks) and make it accessible to multiple components without having to pass it through props manually each time.
React Context acts like a centralized storage box. It allows you to keep certain pieces of information (like user data, theme settings, or application state) in one place. Any component that needs access to the stored data can simply "look into the storage box" and use the data. This is done without the need for passing the data down through every component level manually, which can be cumbersome and error-prone.
How to use Context in React?
Using React Context involves three main steps: creating a context, providing the context to your components, and consuming the context in the components that need it. Let's break down each step and see how it all comes together.
Creating a Context
To create a context, you use React.createContext
. This function returns a context object that you can use to store and provide data. It contains two main components: Provider
and Consumer
.
- Provider: This component allows you to supply the context value to its children.
- Consumer: This component allows you to access the context value.
You can optionally provide a default value that will be used if no Provider
is found above in the component tree. Let's create a context to store a favorite candy.
import React from 'react';
const FavoriteCandyContext = React.createContext();
// OR React.createContext('default value')
export default FavoriteCandyContext;
This FavoriteCandyContext
can now be used to share the favorite candy across different components.
Providing Context
To make the context available to all components, you use a Provider
. A Provider
is a special component that comes with the context you create. Think of it as the person who fills the candy jar (context) and places it in the living room for everyone to access. The Provider
component wraps around the parts of your application that need access to the context, and it supplies the value you want to share.
import React from 'react';
import FavoriteCandyContext from './FavoriteCandyContext';
const App = () => {
return (
<FavoriteCandyContext.Provider value="chocolate">
<ChildComponent />
</FavoriteCandyContext.Provider>
);
};
export default App;
In this example, the App
component wraps ChildComponent
with FavoriteCandyContext.Provider
and sets the value to "chocolate". Now, ChildComponent
and any nested components can access this context value.
The value provided to a Provider
can be any type of data, including strings, numbers, objects, arrays, and even state managed by hooks like useState
or useReducer
.
import React, { useState } from 'react';
import FavoriteCandyContext from './FavoriteCandyContext';
const App = () => {
const [candy, setCandy] = useState("chocolate");
return (
<FavoriteCandyContext.Provider value={{ candy, setCandy }}>
<ChildComponent />
</FavoriteCandyContext.Provider>
);
};
export default App;
Consuming Context
To use the context in a component, you can use the useContext
hook. This hook allows the component to access the context value directly.
import React, { useContext } from 'react';
import FavoriteCandyContext from './FavoriteCandyContext';
const ChildComponent = () => {
const favoriteCandy = useContext(FavoriteCandyContext);
return <div>My favorite candy is {favoriteCandy}</div>;
};
export default ChildComponent;
In ChildComponent
, we use the useContext
hook to access the FavoriteCandyContext
. The favoriteCandy
variable now holds the value "chocolate", and we can use it in the component's JSX.
Example: Sharing a Candy Jar
Let's create a more practical example where we share a candy jar across different components.
Create the Context:
import React, { createContext } from 'react'; const CandyJarContext = createContext(); export default CandyJarContext;
Provide the Context:
import React, { useState } from 'react'; import CandyJarContext from './CandyJarContext'; import LivingRoom from './LivingRoom'; import Kitchen from './Kitchen'; const App = () => { const [candy, setCandy] = useState("gummy bears"); return ( <CandyJarContext.Provider value={{ candy, setCandy }}> <LivingRoom /> <Kitchen /> </CandyJarContext.Provider> ); }; export default App;
Consume the Context:
import React, { useContext } from 'react'; import CandyJarContext from './CandyJarContext'; const LivingRoom = () => { const { candy } = useContext(CandyJarContext); return <h1>In the living room, we have {candy}!</h1>; }; export default LivingRoom; ____________________________________________________________ import React, { useContext } from 'react'; import CandyJarContext from './CandyJarContext'; const Kitchen = () => { const { candy } = useContext(CandyJarContext); return <p>In the kitchen, we enjoy {candy} as a treat.</p>; }; export default Kitchen;
In this example, CandyJarContext
is created to hold a type of candy. The App
component provides the candy "gummy bears" to the LivingRoom
and Kitchen
components. Both components consume the context to display the type of candy.
Why is React Context Useful?
Simplifies Prop Drilling: When you have to pass data through multiple layers of components, it can get messy and complicated. This is called "prop drilling." React Context helps you avoid this by allowing you to provide the data once and consume it wherever needed.
Global State Management: For small to medium-sized applications, React Context can be used as a simple state management tool. You can store global states like user information, theme settings, or language preferences in a context.
Consistency: By centralizing important data, you ensure that all components have consistent access to the same data, reducing the risk of errors and inconsistencies.
The context provided by a Provider
component can be accessed by any component within its tree, regardless of how deeply nested those components are. As long as a component is a descendant of the Provider
, it can access the context value using the useContext
hook. This makes context a powerful tool for sharing data across multiple levels of a component tree without needing to pass props manually at each level.
Conclusion
Using React Context, we can easily share data across many components without the hassle of passing props down the tree. It's like having a central place where you can keep important things and let everyone in your app use them.
Remember, React Context is a powerful tool but should be used wisely. For simple state management, props are still a great choice. But when you need to share data across many components, React Context can make your life a lot easier.
If you found this post helpful, please give it a like and share it with others who might benefit from it. Happy coding!
Subscribe to my newsletter
Read articles from Artur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
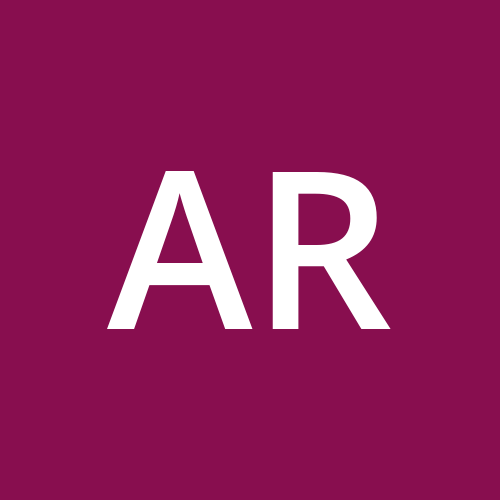