Troubleshooting background-attachment: fixed Bug in iOS Safari
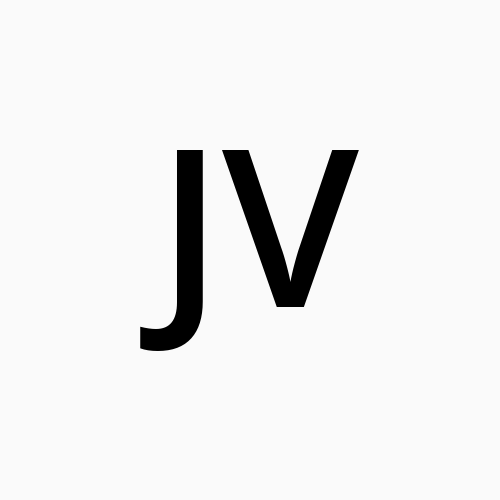
When developing for the web, cross-browser compatibility is always a major concern. One common issue developers face is with the background-attachment: fixed
property in iOS Safari. This feature often doesn't behave as expected, causing background images to fail in creating a fixed position effect. In this article, we will discuss this bug and provide a practical workaround using position: fixed
.
Understanding the Bug
The background-attachment: fixed
property allows you to fix the background image relative to the viewport, meaning the background image stays in place while the rest of the content scrolls. Unfortunately, iOS Safari has had longstanding issues with properly supporting this feature, often leading to undesirable behavior like the background image scrolling with the content.
The Workaround
To achieve a similar effect to background-attachment: fixed
, we can use a combination of CSS and JavaScript. The idea is to change the positioning of a div
containing the background image to position: fixed
once it reaches the edge of the viewport during scrolling.
Step-by-Step Guide
1. HTML Structure
First, we need to set up our HTML structure. We'll create a div
for the content and another div
for the background image.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>iOS Safari Background Fixed Workaround</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="background"></div>
<div class="content">
<h1>Content Heading</h1>
<p>Lots of content here...</p>
<!-- Add enough content to make the page scroll -->
</div>
<script src="script.js"></script>
</body>
</html>
2. CSS Styling
Next, we style our div
elements. Initially, the background will be positioned absolutely.
/* styles.css */
body, html {
margin: 0;
padding: 0;
height: 100%;
overflow-x: hidden;
}
.background {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100vh;
background: url('path/to/your/image.jpg') no-repeat center center;
background-size: cover;
z-index: -1;
}
.content {
position: relative;
z-index: 1;
padding: 20px;
background: rgba(255, 255, 255, 0.8);
}
3. JavaScript for Scroll Event
Now, we need JavaScript to handle the scroll event. The script will check the scroll position and change the background div to position: fixed once the top of the viewport reaches the top of the background div.
// script.js
document.addEventListener('scroll', function() {
const background = document.querySelector('.background');
const content = document.querySelector('.content');
const scrollPosition = window.scrollY;
const contentTop = content.offsetTop;
if (scrollPosition >= contentTop) {
background.style.position = 'fixed';
background.style.top = '0';
} else {
background.style.position = 'absolute';
background.style.top = '0';
}
});
Explanation
HTML Structure: We have two primary div elements, one for the background and one for the content.
CSS Styling: The .background div is positioned absolutely with the background image, covering the viewport initially. The .content div has relative positioning and some padding.
JavaScript: The scroll event listener checks the window's scroll position. If the scroll position is greater than or equal to the top of the content, the background is set to position: fixed. Otherwise, it reverts to position: absolute.
Conclusion
This workaround effectively simulates the background-attachment: fixed behavior in iOS Safari. By dynamically changing the positioning of the background image based on the scroll position, we achieve the desired fixed background effect. While not a perfect one-line CSS solution, this method ensures a smooth and compatible user experience across all devices.
Feel free to experiment with the provided code and adjust it to fit your specific needs. Happy coding!
Subscribe to my newsletter
Read articles from Juan Diego Urena Vazquez directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
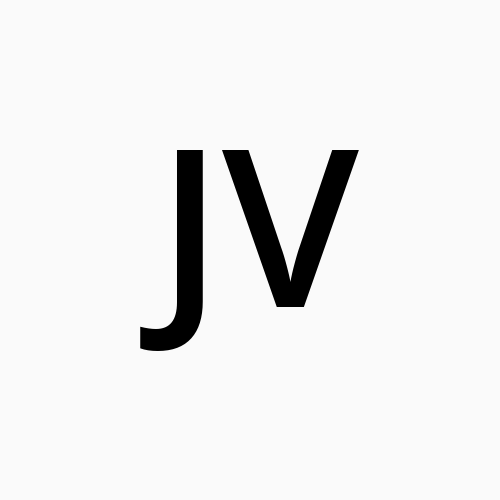