Tightly Coupled vs. Loosely Coupled Systems in Android Development
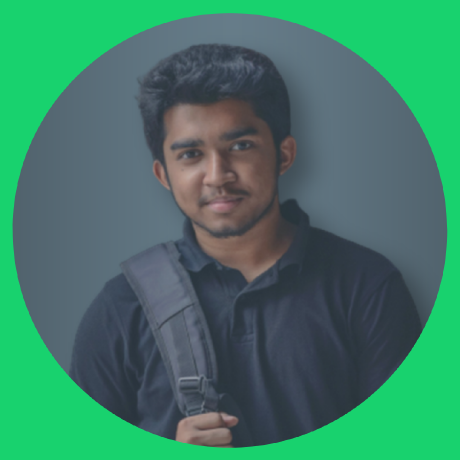
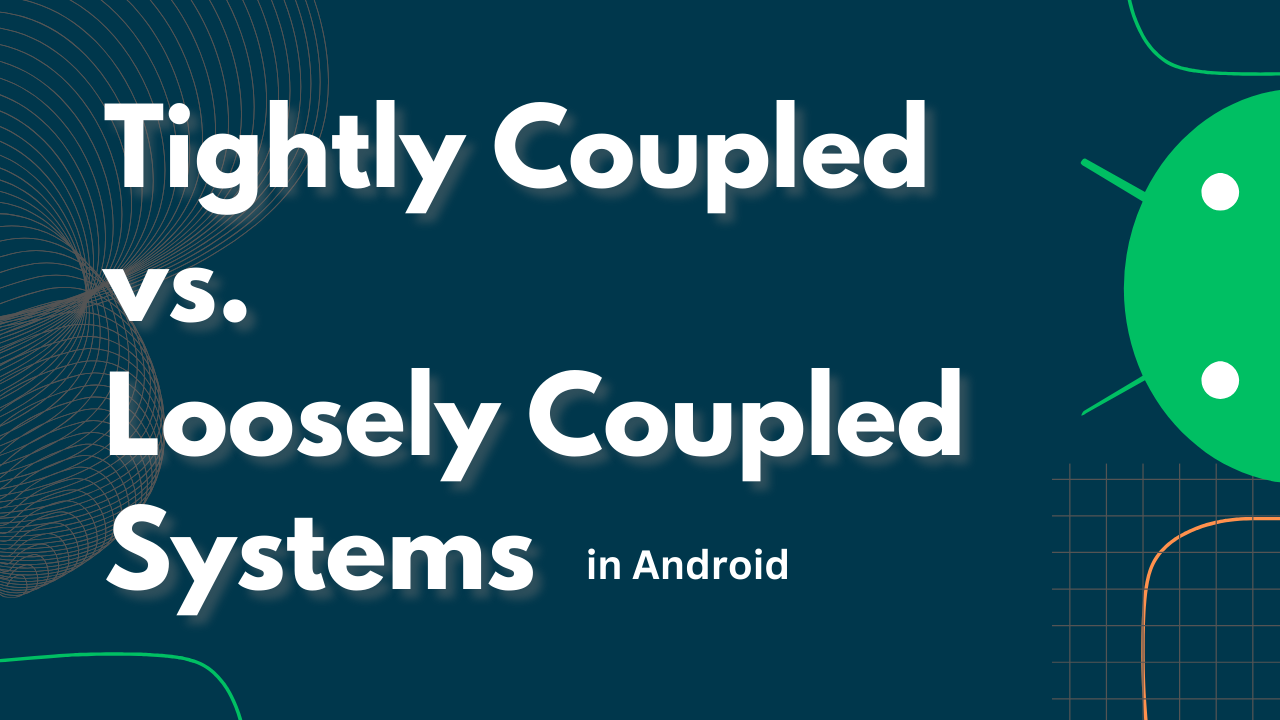
In Android development, the concepts of tightly coupled and loosely coupled systems play a significant role in determining the flexibility, maintainability, and scalability of an application. Understanding these concepts helps developers design more robust and efficient apps. This blog will delve into what tightly coupled and loosely coupled systems mean in the context of Android development, their advantages and disadvantages, and best practices for achieving loose coupling in Android applications.
Tightly Coupled Systems in Android
In tightly coupled Android systems, components such as Activities, Fragments, and other classes are heavily dependent on each other. This dependency can make the application less flexible and harder to maintain. Let's explore the characteristics of tightly coupled systems in Android:
Characteristics:
Direct Dependencies: Activities and Fragments directly reference each other or other components, leading to a web of dependencies.
Hard-coded Implementation: UI elements and business logic are often mixed within Activities or Fragments, making it difficult to reuse code.
Complex Maintenance: Changing one component requires changes in all dependent components, increasing the risk of introducing bugs.
Low Reusability: Components are often not reusable in different contexts due to their direct dependencies on specific implementations.
Advantages:
Performance: Tightly coupled systems can be more efficient in terms of performance because components can directly access each other's resources without the overhead of abstraction layers.
Simplicity: In small, straightforward systems, tight coupling can simplify design and implementation by reducing the number of abstraction layers.
Disadvantages:
Scalability Issues: Scaling a tightly coupled system can be challenging because changes to one part of the system require changes to others.
Flexibility Issues: Tightly coupled systems are less flexible in accommodating new requirements or integrating with other systems.
Complexity in Testing: Testing tightly coupled systems is complex due to the interdependencies between components.
Example:
public class MainActivity extends AppCompatActivity {
private DetailFragment detailFragment;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
detailFragment = new DetailFragment();
getSupportFragmentManager().beginTransaction()
.add(R.id.fragment_container, detailFragment)
.commit();
}
public void updateDetail(String data) {
detailFragment.updateContent(data);
}
}
In this example, MainActivity directly depends on DetailFragment, leading to tight coupling.
Loosely Coupled Systems in Android
Loosely coupled systems in Android, on the other hand, aim to minimize dependencies between components. This makes the application more modular, flexible, and easier to maintain.
Characteristics:
Minimal Dependencies: Components interact through well-defined interfaces or communication mechanisms, reducing direct dependencies.
Separation of Concerns: UI, business logic, and data handling are separated, often using patterns like MVVM (Model-View-ViewModel).
Easier Maintenance: Changes in one component do not significantly affect others, simplifying the maintenance process.
High Reusability: Components are designed to be reused in different parts of the application or even in different applications.
Advantages:
Scalability: Loosely coupled systems can scale more easily as components can be modified or replaced without significant changes to the entire system.
Flexibility: Such systems are more adaptable to change, making it easier to integrate new features or technologies.
Simplified Testing: Independent components can be tested in isolation, leading to more straightforward and reliable testing processes.
Disadvantages:
Performance Overhead: The abstraction layers that enable loose coupling can introduce performance overhead due to additional communication or processing steps.
Complexity in Design: Designing loosely coupled systems requires careful planning and adherence to best practices, which can initially be more complex and time-consuming.
Example:
public class MainActivity extends AppCompatActivity {
private MainViewModel viewModel;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
viewModel = new ViewModelProvider(this).get(MainViewModel.class);
DetailFragment detailFragment = new DetailFragment();
getSupportFragmentManager().beginTransaction()
.add(R.id.fragment_container, detailFragment)
.commit();
viewModel.getDetailData().observe(this, data -> {
// Update the UI with the new data
detailFragment.updateContent(data);
});
}
}
public class MainViewModel extends ViewModel {
private MutableLiveData<String> detailData = new MutableLiveData<>();
public LiveData<String> getDetailData() {
return detailData;
}
public void setDetailData(String data) {
detailData.setValue(data);
}
}
In this example, MainActivity observes changes in MainViewModel's data without directly depending on the internal implementation of the ViewModel.
Best Practices for Achieving Loose Coupling in Android
To design loosely coupled systems in Android, developers can follow these best practices:
Use ViewModel and LiveData: Utilize Android's ViewModel and LiveData to manage UI-related data lifecycle-aware, reducing direct dependencies between UI components.
Apply Dependency Injection: Use dependency injection frameworks like Dagger or Hilt to inject dependencies rather than creating them directly within components.
Adopt MVVM or MVP Patterns: Use architectural patterns like MVVM (Model-View-ViewModel) or MVP (Model-View-Presenter) to separate concerns and reduce direct dependencies.
Utilize Interfaces: Define interfaces for communication between components. This allows different implementations to be swapped without changing the dependent components.
Modularization: Break the application into smaller, feature-specific modules. This modular approach enhances reusability and maintainability.
Conclusion
Understanding and implementing tightly coupled versus loosely coupled systems is crucial for developing maintainable and scalable Android applications. While tightly coupled systems might offer simplicity in small-scale projects, they can become problematic as the application grows. On the other hand, loosely coupled systems provide flexibility, ease of maintenance, and scalability, which are essential for modern Android applications. By following best practices such as using ViewModel, LiveData, dependency injection, and architectural patterns like MVVM, developers can achieve loose coupling and build robust Android applications ready to meet evolving requirements.
Subscribe to my newsletter
Read articles from Emran Khandaker Evan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
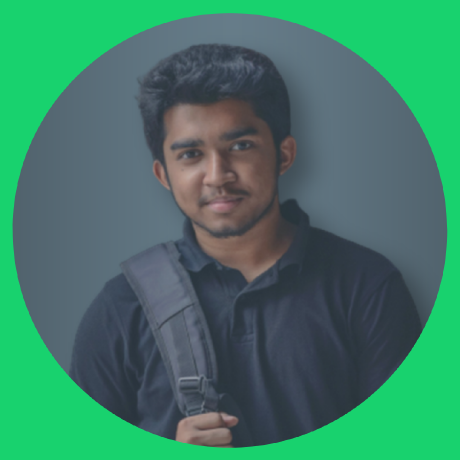
Emran Khandaker Evan
Emran Khandaker Evan
Software Engineer | Content Creator