Step-by-Step Guide to Adding Tailwind to a Bootstrap Project

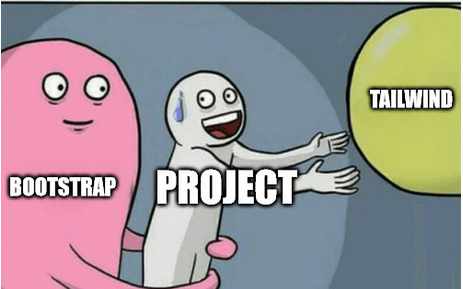
I've been working with a template that uses Bootstrap. Later on, I wanted the ability to extend the template, not with Bootstrap, but with tailwindCSS.
For some context, the template had 175 sass files and at least 1000 lines inside the configuration file, plus all the styles inside Javascript.
Let's get to it.
Install TailwindCSS.
Follow the instructions on Tailwind's official website https://tailwindcss.com/docs/installation or follow the instructions below.
Install Tailwind
npm install -D tailwindcss
oryarn add tailwindcss --dev
Add a tailwind config file with this command.
npx tailwindcss init
Add the tailwind base file.
Add the tailwind directives below to a CSS file inside your source folder. This CSS file will be the base/entry point. (e.g. inside the.src/styles/tailwind.css
file.)
@tailwind base;
@tailwind components;
@tailwind utilities;
Remove tailwinds reset-styles
CSS reset styles ensure that all HTML elements appear consistently across all browsers. And because your project already uses a different CSS framework, it already has an existing set of rest styles.
Bootstrap and TailwindCSS each have their reset styles: Reboot for Bootstrap and Preflight for Tailwind. The table below shows a few styles that could conflict.
Bootstrap | TailwindCSS | |
Headings | margin: 0 0 0.5rem 0; | margin:0; |
All tags * | No border styles | border: 0 solid #e7e5e4 |
Paragraph | margin: 0 0 1rem 0; | margin:0; |
And many more... |
Since this project is based on Bootstrap, we will remove tailwinds resets. There are two ways to do it.
Remove the
@tailwind base;
a directive inside the base file. (This directive inserts tailwinds reset styles.)/* @tailwind base; <-- remove this directive */ @tailwind components; @tailwind utilities;
Add the
preflight: false
property to thetailwind.config.js
file.module.exports = { content: ["./src/**/*.{html,js}"], // add the corePlugins property corePlugins: { // then add the preflight property preflight: false // and set it to false }, theme: { extend: {}, }, plugins: [], }
Add a prefix to the tailwind classes
Some classes in bootstrap and tailwind have similar names, for example.
Bootstrap | Tailwind |
.container | .container |
padding .p-0 .p-1 .p-2 .p-3 .p-4 .p-5 | padding .p-0 .p-1 .p-2 .p-3 .p-4 .p-5 |
margin .p-0 to .p-5 | margin .p-0 to .p-5 |
And other classes |
To avoid conflicts, we can add a prefix to all tailwind classes. The prefix can be the initials of your project e.g. me-
or tw-
for tailwind. TIP: make the prefix short; two letters should be the maximum; otherwise, your class list will grow long.
module.exports = {
content: ["./src/**/*.{html,js}"],
corePlugins: {
preflight: false
},
// add the prefix property
prefix: 'tw-', // set prefix to 'tw-'
theme: {
extend: {},
},
plugins: [],
}
Now the tailwind classes will be different from the bootstrap classes
Bootstrap | Tailwind |
.container | .tw-container |
padding .p-0 to .p-5 | padding .tw-p-0 to .tw-p-5 |
margin .p-0 to .p-5 | margin .tw-p-0 to .tw-p-5 |
And other classes |
Matching breakpoints
Your current project uses predefined breakpoints, which may differ from the tailwindCSS breakpoints.
Bootstrap | Tailwind | |
small | min-width: 576px | min-width: 640px |
medium | min-width: 768px | min-width: 768px |
large | min-width: 992px | min-width: 1024px |
And more... |
To match the breakpoints, add a screen
property inside the theme
property
module.exports = {
content: ["./src/**/*.{html,js}"],
corePlugins: {
preflight: false
},
prefix: 'tw-',
// inside the theme property,
theme: {
extend: {},
// add a screen property
screens: {
/*
then add the bootstrap breakpoint key:values, some of the keys are different and some of the values are different. therefore, update the key:values to match Bootstraps key:values
*/
'sm': '576px',
'md': '768px',
'lg': '992px',
'xl': '1200px',
'xxl': '1400px', // key changed to match bootstrap's key 'xxl', or if you're used to tailwind you can leave it as '2xl'
}
},
plugins: [],
}
What about colours
Like breakpoints, we'd want the colours in tailwindCSS to match out project's colours. If our bootstrap project uses this as the primary color #ff6b11
, then we can add it to the config file.
module.exports = {
content: ["./src/**/*.{html,js}"],
corePlugins: {
preflight: false
},
prefix: 'tw-',
// inside the theme property,
theme: {
// inside the extend property
extend: {
// extend the theme with a color property
colors: {
primary: '#ff6b11', // primary
// you can add more colors
secondary: '#bb7700',
// ... more colors
}
},
screens: {
'sm': '576px',
'md': '768px',
'lg': '992px',
'xl': '1200px',
'xxl': '1400px',
}
},
plugins: [],
}
Now you can use the colour in your HTML
<div class="tw-bg-primary"><div>
For more options, you can add an object with a list of primary colors with different shades, e.g. when you want a lighter primary color for a component like in the example below.
You can use this handy tool to generate variations of the primary color.
module.exports = {
content: ["./src/**/*.{html,js}"],
corePlugins: {
preflight: false
},
prefix: 'tw-',
// extend the theme with a color
theme: {
extend: {
colors: {
primary: {
100: "#CBE6FB", // lightest primary
200: "#99CBF7",
300: "#64A4E7",
400: "#3C7FCF",
500: "#0A4EB0", // primary
600: "#073C97",
700: "#052C7E",
800: "#031F66",
900: "#011654", // daskest primary
} // primary colors with different shades
}
},
},
plugins: [],
}
Which can now be used like this
<div class="tw-bg-primary-100">
<h1 class='tw-text-primary-800'>alert</h1>
<div>
Compiling the tailwind file
You most likely have a source folder where all your pre-compiled source files are, and an output directory where all the compiled source files are.
Now you probably already have a build process that compiles the bootstrap files, if you have experience working with Tailwind you can integrate it to your build process. But here is a quick way to compile the tailwind classes.
Add this script
npx tailwindcss -i {path/to/tailwind/input/file} -o {path/to/tailwind/output/file} --watch
to thepackage.json
file that will compile the tailwind classes.I named the script 'tailwind' (just my preference).
--watch
watches for any changes in the files specified in thetailwind.config.js
file
-i
The input file or the entry point.
-o
The output file"scripts": { // ... other scripts "tailwind": "npx tailwindcss -i ./src/styles/tailwind.css -o ./build/css/tailwind.css --watch" }
Tips
When building a new page, it will be easy to exclude the bootstrap file. Then the whole page will be based on tailwind with zero conflicts.
If you try to change the styles of an existing component using bootstrap with a tailwind class and the tailwind style does not override, meaning the bootstrap classes have a higher specificity, you can add the important property in the tailwind config file just below the
prefix
property.important: true,
. Though I would recommend styling the template CSS file directly and using the 'important' as a last resort.You'll have to add more classes for border classes. e.g. to get a border-bottom, you'll need
s-border-t-0 s-border-b-[1px] s-border-solid s-border-gray-300
the preflight has some of the border settings, which we cannot access since we don't have the base styles.The buttons will lose some default styles (especially when you're using a library that doesn't include the preflight classes in the button components) e.g.
cursor-pointer, transform-none, margin-0, family-inherit
Working with the
divide-x
classes which uses the border utility
s-border-t-0 s-border-b-[1px] s-border-solid s-border-gray-300References
Subscribe to my newsletter
Read articles from Kanyi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
