Series - Java code
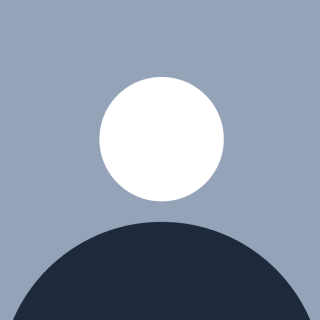
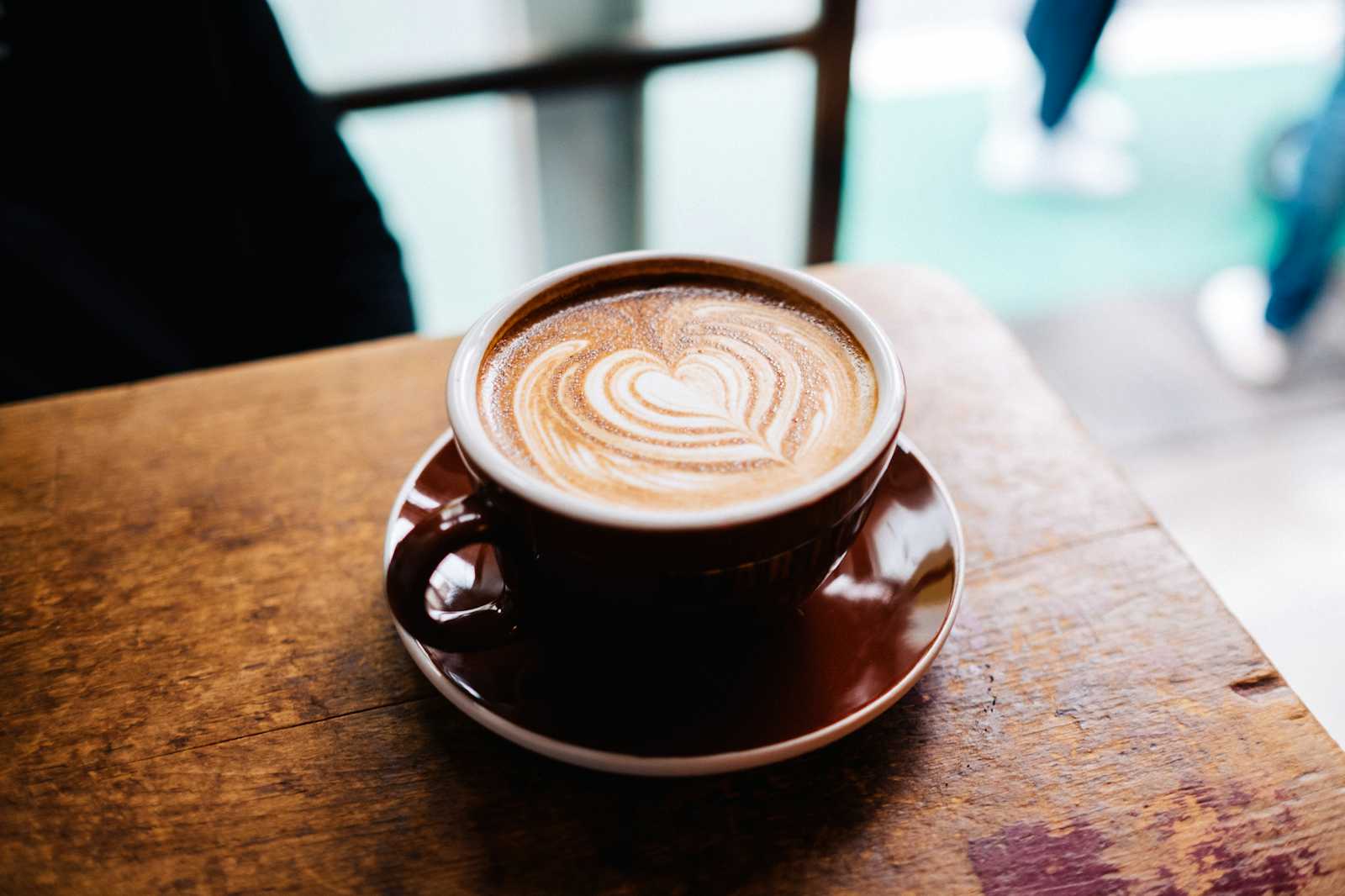
Creating a Series Generator in Java
In this tutorial, we will create a simple Java program to generate and print a series where each term is double the previous term, starting from 3. The series looks like this: 3, 6, 12, 24, 48, ...
Step-by-Step Instructions
Set Up Your Environment:
Ensure you have Java Development Kit (JDK) installed on your computer.
Use an Integrated Development Environment (IDE) like IntelliJ IDEA, Eclipse, or a simple text editor to write your Java code.
Write the Java Code:
Create a new Java file named
shounak.java
.Import the
Scanner
class for user input.
Here is the complete code for generating the series:
import java.util.Scanner;
public class shounak {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n;
int e = 3; // Starting value of the series
System.out.print("Enter the range: ");
n = sc.nextInt();
for (int i = 1; i <= n; i++) {
System.out.println(e); // Print the current value
e *= 2; // Double the value for the next iteration
}
}
}
Explanation of the Code
Importing Scanner:
import java.util.Scanner;
This line imports the
Scanner
class, which is used to take input from the user.Class Definition:
public class shounak {
This line defines a public class named
shounak
.Main Method:
public static void main(String[] args) {
This line defines the main method, which is the entry point of the program.
Creating Scanner Object:
Scanner sc = new Scanner(System.in);
This line creates a
Scanner
object to read input from the user.Variable Initialization:
int n; int e = 3; // Starting value of the series
n
is used to store the range of the series, ande
is initialized to 3, the starting value of the series.Taking User Input:
System.out.print("Enter the range: "); n = sc.nextInt();
These lines prompt the user to enter the range and store the input in the variable
n
.Generating and Printing the Series:
for (int i = 1; i <= n; i++) { System.out.println(e); // Print the current value e *= 2; // Double the value for the next iteration }
This loop runs from 1 to
n
and prints each term in the series. The value ofe
is doubled in each iteration.
Running the Program
Compile the Code: Open your terminal or command prompt and navigate to the directory where
shounak.java
is saved. Run the following command to compile the code:javac shounak.java
Run the Program: After successful compilation, run the program using the following command:
java shounak
Input the Range: When prompted, enter the desired range for the series. For example, if you input
5
, the program will output:Enter the range: 5 3 6 12 24 48
Conclusion
This simple Java program demonstrates how to use a loop to generate a series based on a specified range. By following these instructions, you can create similar programs to generate different types of series or sequences. Experiment with different starting values and rules for generating the series to deepen your understanding of loops and arithmetic operations in Java.
Subscribe to my newsletter
Read articles from TechReview_SS directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
TechReview_SS
TechReview_SS
Sharing about tech - reviews, comparisons, customizations of products.