Series - in Java
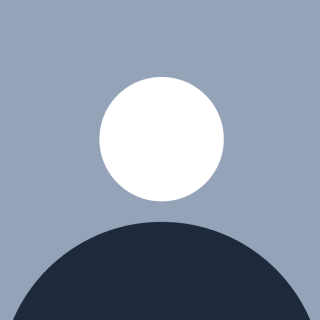
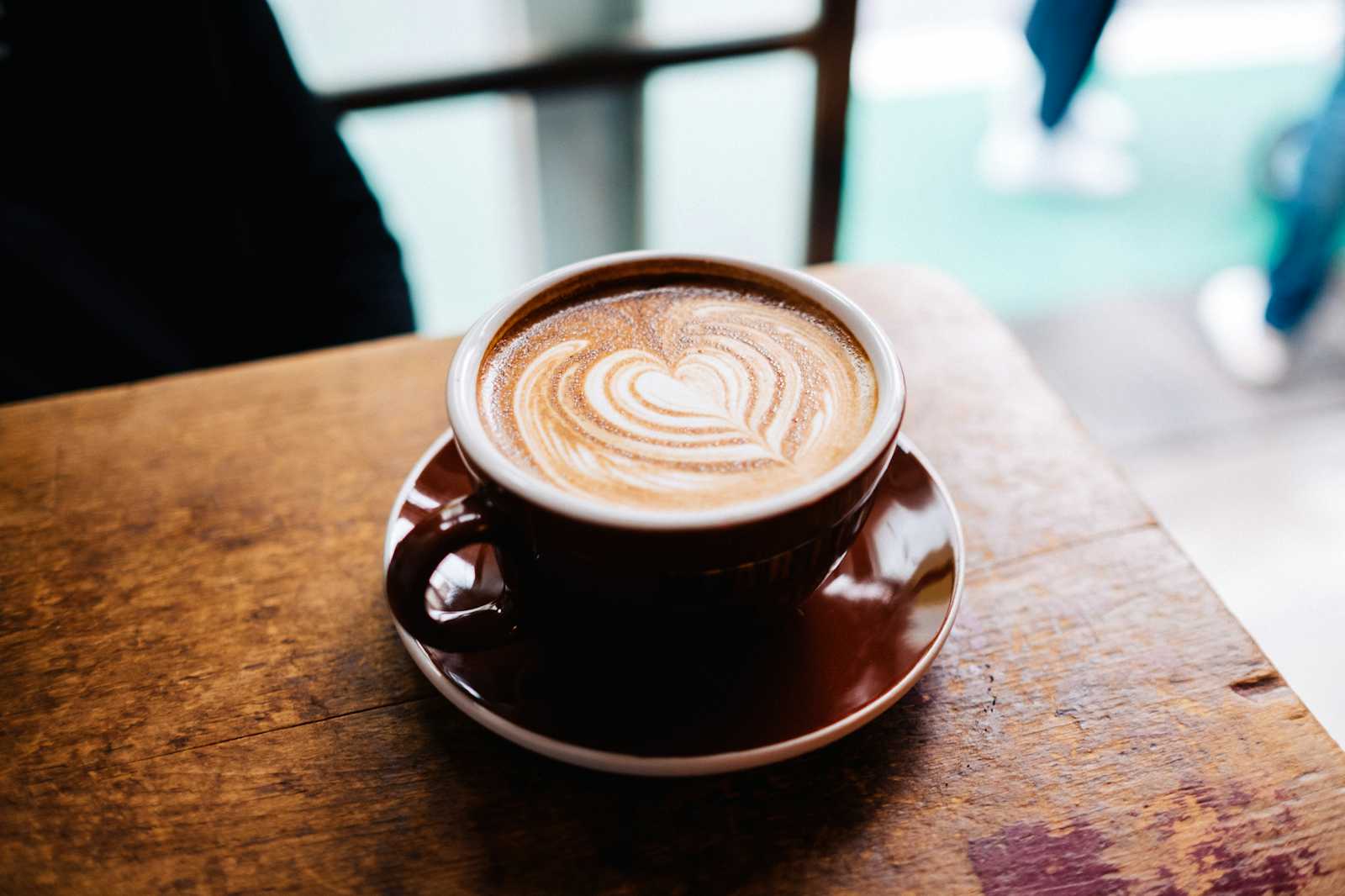
Understanding the Series: 4.0, 16.0, and Beyond
Series - 4.0, 16.0, ..., n
In the realm of programming, generating sequences and series is a common exercise to understand loops, conditional statements, and mathematical operations. The provided Java code snippet is an excellent example of how to generate a specific numerical series: 4.0, 16.0, and so forth. Let's delve into the mechanics of the code and explore the underlying mathematical concept.
The Java Program
Here’s a breakdown of the provided Java code:
import java.util.Scanner;
import java.lang.Math;
public class shounak {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double a;
int i, n;
System.out.print("Enter the number: ");
n = sc.nextInt();
for (i = 1; i <= n; i++) {
if (i % 2 == 0) {
a = Math.pow(i, 2);
System.out.println(a);
}
}
sc.close();
}
}
Explanation of the Code
Import Statements:
import java.util.Scanner;
: Imports the Scanner class for taking user input.import java.lang.Math;
: Imports the Math class to use mathematical functions, particularlyMath.pow()
.
Main Method: The entry point of the program where execution begins.
Variable Declaration:
double a;
: A variable to store the square of the number.int i, n;
: Loop counteri
and the numbern
which is the upper limit provided by the user.
User Input: The program prompts the user to enter a number
n
. This determines how many terms in the series the program will evaluate.Loop and Conditional Check:
for (i = 1; i <= n; i++)
: A loop that runs from 1 ton
.if (i % 2 == 0)
: Checks ifi
is even.a = Math.pow(i, 2);
: Computes the square ofi
wheni
is even.System.out.println(a);
: Prints the computed square.
Close Scanner:
sc.close();
closes the scanner to prevent resource leakage.
The Series: 4.0, 16.0, and Beyond
The series generated by this code snippet consists of the squares of even numbers. Let's look at the mathematical basis:
Even Numbers: Numbers divisible by 2, i.e., 2, 4, 6, 8, etc.
Squares of Even Numbers: The series we obtain by squaring these even numbers is:
( 2^2 = 4 )
( 4^2 = 16 )
( 6^2 = 36 )
( 8^2 = 64 )
and so on...
Thus, the series is 4.0, 16.0, 36.0, 64.0, ..., which represents the squares of the even numbers starting from 2.
Output Example
If the user inputs 8, the program will generate the squares of all even numbers from 2 to 8:
Enter the number: 8
4.0
16.0
36.0
64.0
Conclusion
The given Java program effectively demonstrates how to generate a series of squares of even numbers using fundamental programming constructs. By understanding and modifying this basic template, one can explore a vast array of numerical sequences and deepen their grasp of both programming and mathematical principles.
Subscribe to my newsletter
Read articles from TechReview_SS directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
TechReview_SS
TechReview_SS
Sharing about tech - reviews, comparisons, customizations of products.