Series Java - part 3
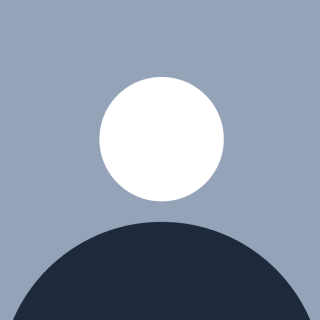
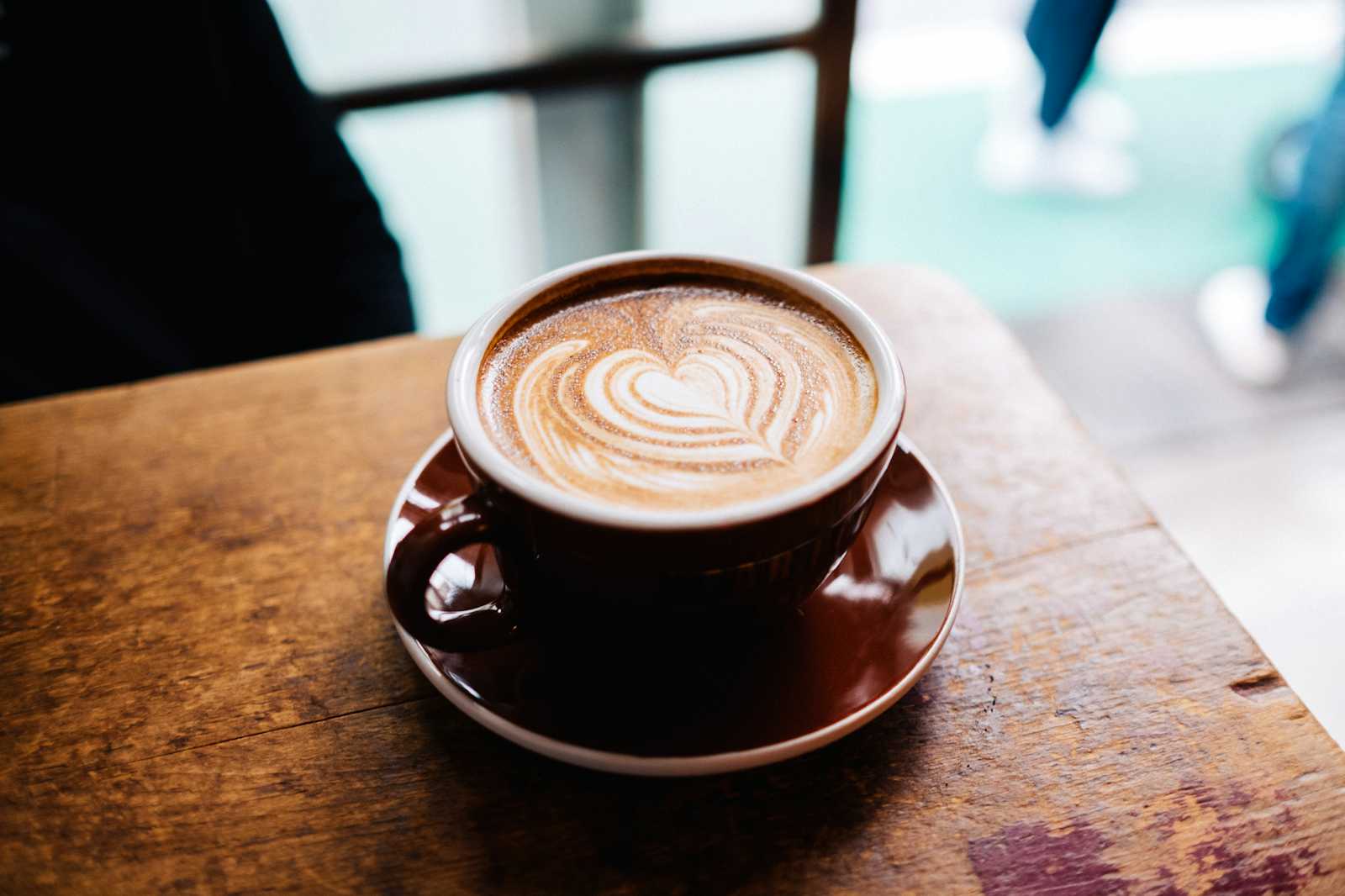
Understanding the Series: 1.5, 3.0, 4.5, 6.0, and Beyond
Generating numerical series is a fundamental aspect of programming that helps in understanding loops, arithmetic operations, and incremental values. The provided Java code snippet showcases how to generate a simple yet intriguing numerical series: 1.5, 3.0, 4.5, 6.0, and so on. Let’s break down the mechanics of the code and explore the underlying arithmetic concept.
The Java Program
Here’s a breakdown of the provided Java code:
import java.util.Scanner;
import java.lang.Math;
public class shounak {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double a;
int i, n;
double sum = 1.5;
System.out.print("Enter the number: ");
n = sc.nextInt();
for (i = 1; i <= n; i++) {
System.out.println(sum);
sum = sum + 1.5;
}
sc.close();
}
}
Explanation of the Code
Import Statements:
import java.util.Scanner;
: Imports the Scanner class for taking user input.import java.lang.Math;
: Though imported,Math
is not utilized in this specific code snippet.
Main Method: The entry point of the program where execution begins.
Variable Declaration:
double a;
: Declared but not used in this code.int i, n;
: Loop counteri
and the numbern
which is the upper limit provided by the user.double sum = 1.5;
: Initializes the starting value of the series.
User Input: The program prompts the user to enter a number
n
. This determines how many terms in the series the program will output.Loop and Increment:
for (i = 1; i <= n; i++)
: A loop that runs from 1 ton
.System.out.println(sum);
: Prints the current value ofsum
.sum = sum + 1.5;
: Incrementssum
by 1.5 for the next iteration.
Close Scanner:
sc.close();
closes the scanner to prevent resource leakage.
The Series: 1.5, 3.0, 4.5, 6.0, and Beyond
The series generated by this code snippet is an arithmetic progression where each term increases by a fixed amount (1.5). Let’s look at the mathematical basis:
Initial Term: The series starts at 1.5.
Common Difference: Each subsequent term increases by 1.5.
Thus, the series is 1.5, 3.0, 4.5, 6.0, ..., representing the terms of an arithmetic progression with a common difference of 1.5.
Output Example
If the user inputs 5, the program will generate the first five terms of the series:
Enter the number: 5
1.5
3.0
4.5
6.0
7.5
Conclusion
The given Java program effectively demonstrates how to generate an arithmetic series using fundamental programming constructs. By understanding and modifying this basic template, one can explore a vast array of numerical sequences and deepen their grasp of both programming and mathematical principles. This exercise not only reinforces the concept of loops and arithmetic operations but also provides a stepping stone to more advanced numerical and algorithmic challenges.
Subscribe to my newsletter
Read articles from TechReview_SS directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
TechReview_SS
TechReview_SS
Sharing about tech - reviews, comparisons, customizations of products.