How to setup different database for development and testing in NodeJS
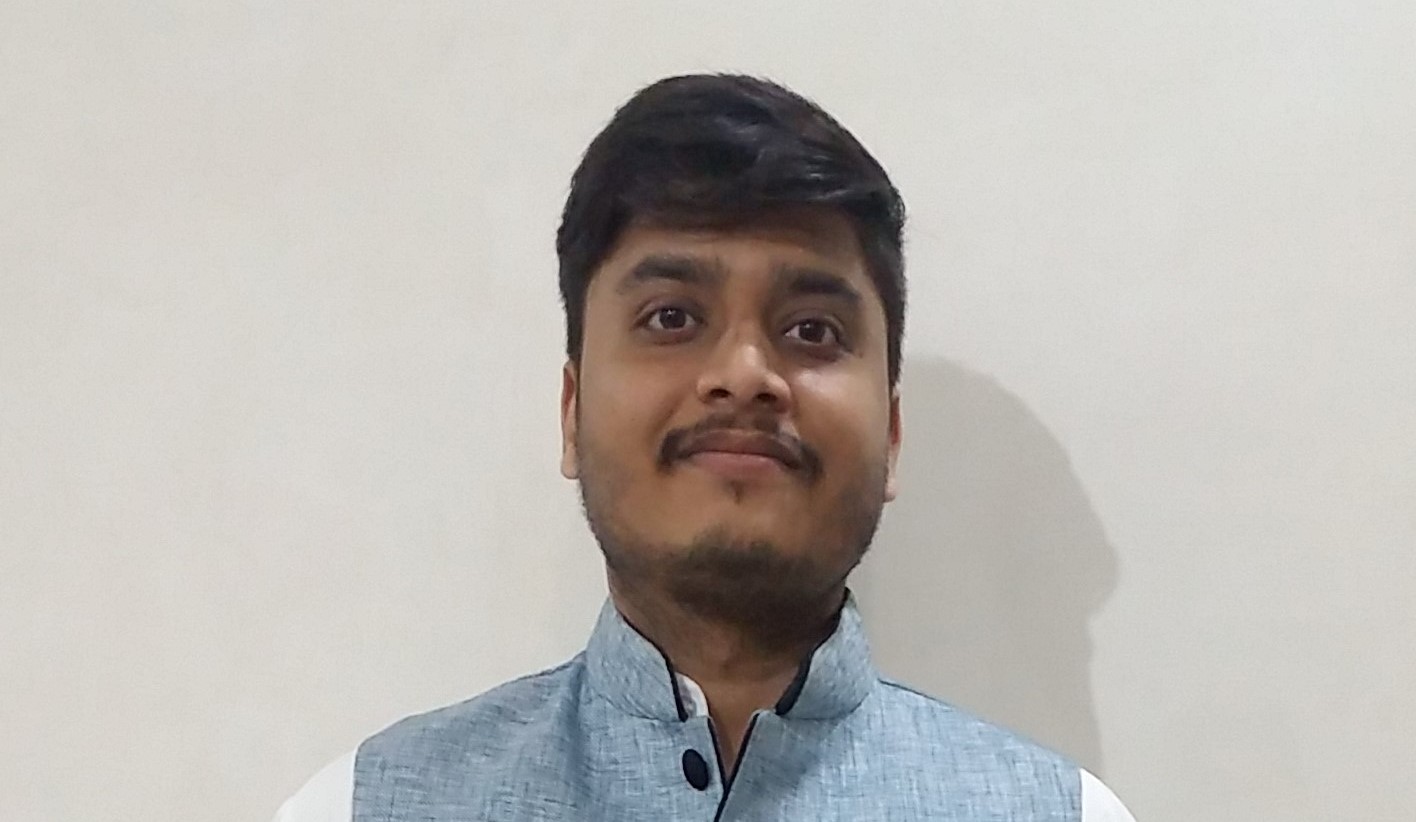
Obviously we do not want to mess up our development and test data and hence want to setup a different database for both the purposes. I faced this issue when performing unit and integration testing in my node application using chai and mocha. Below are the steps that you need to follow in order to do the setup.
Make a
.env
: To store all your development variablesMONGO_URI_DEV=mongodb://localhost:27017/eventsDB NODE_ENV=development
Make a
.env.test
: To store all your test variablesMONGO_URI_TEST=mongodb://localhost:27017/eventsTestDB NODE_ENV=test
Now you must be connecting to mongo when you are starting the server (this might be in your index.js or app.js). modify this code slightly so that it only connects to this database when
NODE_ENV === development
:if (process.env.NODE_ENV === "development") { try { mongoose.connect(process.env.MONGO_URI_DEV); console.log("cwejncewnejfncwjk"); } catch (error) { console.log("Failed while trying to establish connection with mongodb"); } }
Lastly modify your package.json. The below code indicates that whenever I run
npm run test
, this script will run and first it will set the NODE_ENV as test and then mocha will recursively run all the js files (where tests are written) present in the test folder."scripts": { "test": "NODE_ENV=test mocha --recursive test/**/*.js --exit" }
and finally connect to the test database in the before clause in your tests.
before((done) => { console.log("Running before all unit tests"); mongoose .connect(process.env.MONGO_URI_TEST) .then(() => { console.log("successfully connected to DB"); done(); }) .catch((error) => { console.log("Failed while connecting with MongoDB"); done(error); }); });
The above steps will make sure that whenever you run npm run test
, you’ll be connected to the test database and whenever you do node index.js
, you’ll be connected to the development database. The same concept can be extended to integrate production database as well.
Subscribe to my newsletter
Read articles from Rajat Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
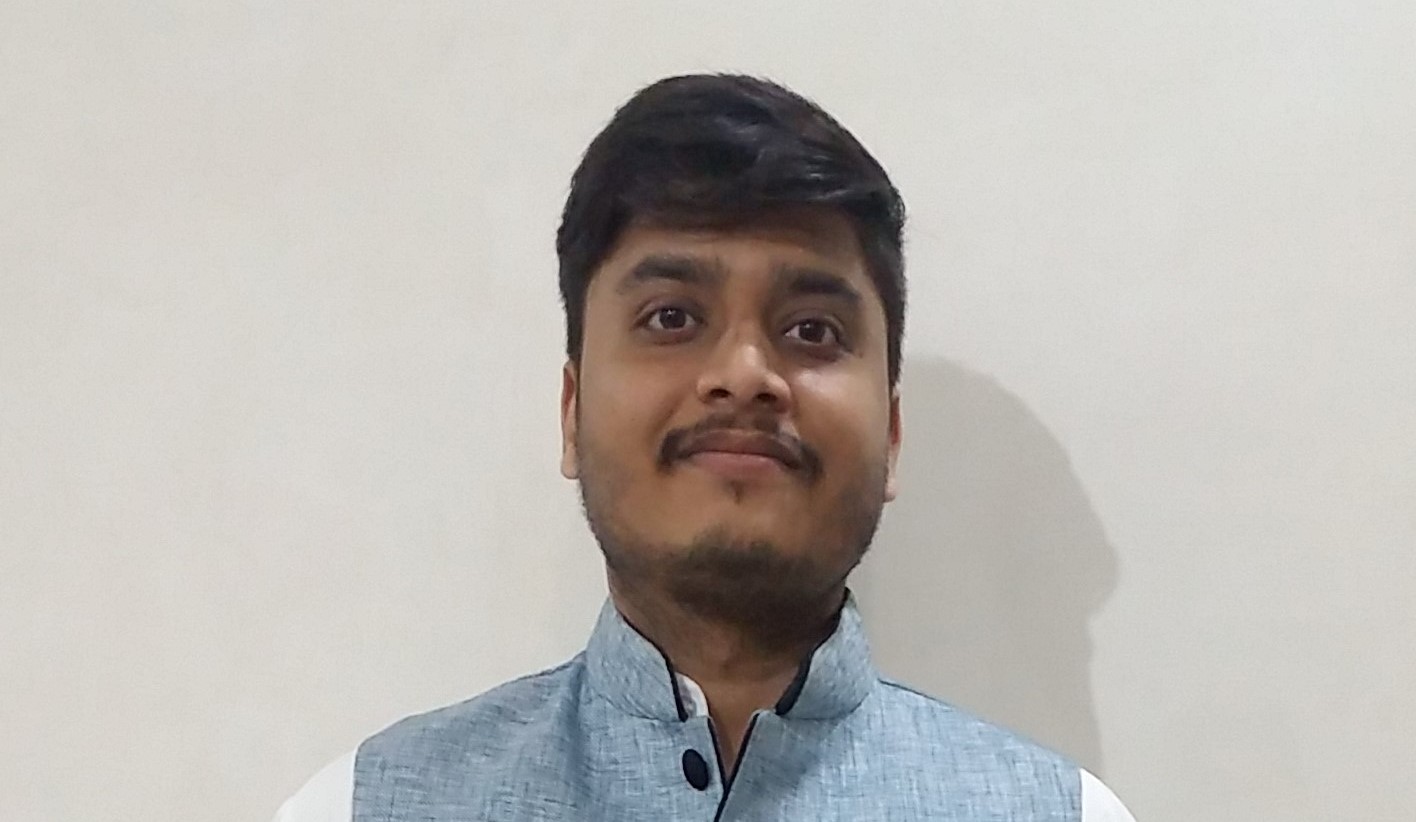
Rajat Gupta
Rajat Gupta
Hi, I am rajat. I write about react, javascript or css 4 times a week. One more thing: I would love to work as a frontend engineer (focused on ReactJS). If you have any such role, ping me at dguptarajat@gmail.com.