Beginner's Guide to Docker: A Crash Course

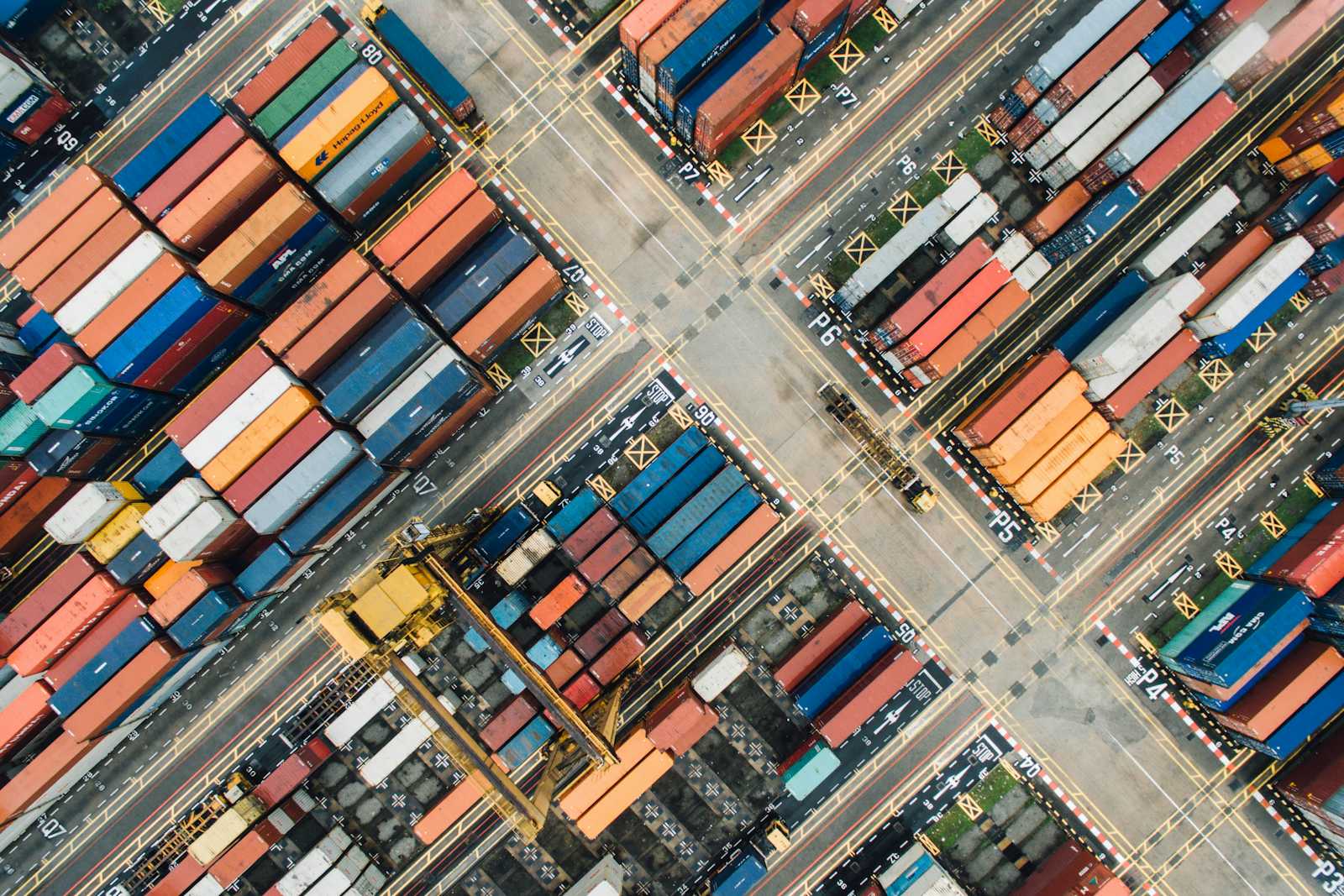
Welcome to our crash course on Docker! If you're new to Docker and want to learn how it can transform your development workflow, you're in the right place. This guide will introduce you to the basics of Docker, explain its benefits, and provide practical examples to help you get started.
What is Docker?
Docker is a platform that lets developers automate the deployment, scaling, and management of applications in lightweight, portable containers. Containers include everything an application needs to run: code, runtime, system tools, libraries, and settings. This ensures consistency across different environments, from development to production.
Why Use Docker?
Consistency: Docker ensures that your application runs the same way, no matter where it's deployed.
Isolation: Each container runs in its own isolated environment, preventing conflicts.
Efficiency: Containers share the host OS kernel, making them more efficient and lightweight compared to virtual machines.
Portability: Docker containers can run on any system that supports Docker.
Installing Docker
Before we dive into Docker commands, let's get Docker installed. You can find installation instructions for your operating system on the Docker documentation site.
Installation on Ubuntu
Here's a quick guide to installing Docker on Ubuntu:
# Update your existing list of packages
sudo apt update
# Install prerequisite packages
sudo apt install apt-transport-https ca-certificates curl software-properties-common
# Add Docker’s official GPG key
curl -fsSL https://download.docker.com/linux/ubuntu/gpg | sudo apt-key add -
# Add Docker APT repository
sudo add-apt-repository "deb [arch=amd64] https://download.docker.com/linux/ubuntu $(lsb_release -cs) stable"
# Update the package database with Docker packages from the newly added repo
sudo apt update
# Install Docker
sudo apt install docker-ce
# Verify that Docker is installed correctly by running a hello-world container
sudo docker run hello-world
Docker Basics
Docker Images and Containers
Images: An image is a read-only template with instructions to create a Docker container. It often builds on another image with added customizations.
Containers: A container is a runnable instance of an image. You can create, start, stop, move, or delete a container using Docker commands.
Pulling an Image
To pull an image from Docker Hub (a repository of Docker images), use the docker pull
command:
docker pull ubuntu
This command downloads the latest Ubuntu image to your local machine.
Running a Container
Once you have an image, you can create and run a container from it:
docker run -it ubuntu
-it
runs the container in interactive mode with a terminal.ubuntu
is the name of the image.
Basic Docker Commands
Here are some essential Docker commands to manage containers:
List Running Containers:
docker ps
List All Containers (including stopped ones):
docker ps -a
Stop a Container:
docker stop <container_id>
Remove a Container:
docker rm <container_id>
Creating Your First Dockerfile
A Dockerfile is a text document that contains all the commands to assemble an image. Here's a simple example:
# Use the official Ubuntu image as a base
FROM ubuntu:latest
# Install a package
RUN apt-get update && apt-get install -y nginx
# Expose port 80
EXPOSE 80
# Define the command to run when the container starts
CMD ["nginx", "-g", "daemon off;"]
Building an Image
To build an image from a Dockerfile, navigate to the directory containing the Dockerfile and run:
docker build -t my-nginx-image .
-t my-nginx-image
tags the image with a name..
specifies the build context (current directory).
Running Your Custom Container
Now, run a container from your newly created image:
docker run -d -p 80:80 my-nginx-image
-d
runs the container in detached mode (in the background).-p 80:80
maps port 80 on your host to port 80 on the container.
Visit http://localhost
in your browser, and you should see the default Nginx page.
Docker Compose
Docker Compose is a tool for defining and running multi-container Docker applications. With Compose, you use a YAML file to configure your application’s services.
Example docker-compose.yml
Here's a simple example for a web application with Nginx and Redis:
version: '3'
services:
web:
image: nginx
ports:
- "80:80"
redis:
image: redis
Running Docker Compose
To start your application, navigate to the directory containing your docker-compose.yml
and run:
docker-compose up
Conclusion
Docker makes development and deployment easier, ensuring consistent application management across environments. This guide covered Docker basics, including installation, commands, Dockerfiles, and Docker Compose.
For more, visit the official Docker documentation to explore advanced topics like Docker Swarm and Docker Hub.
Happy Dockering!
Subscribe to my newsletter
Read articles from Sajid Shaikh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
