Stop the Bots in Their Tracks: Mastering Laravel Rate Limiting
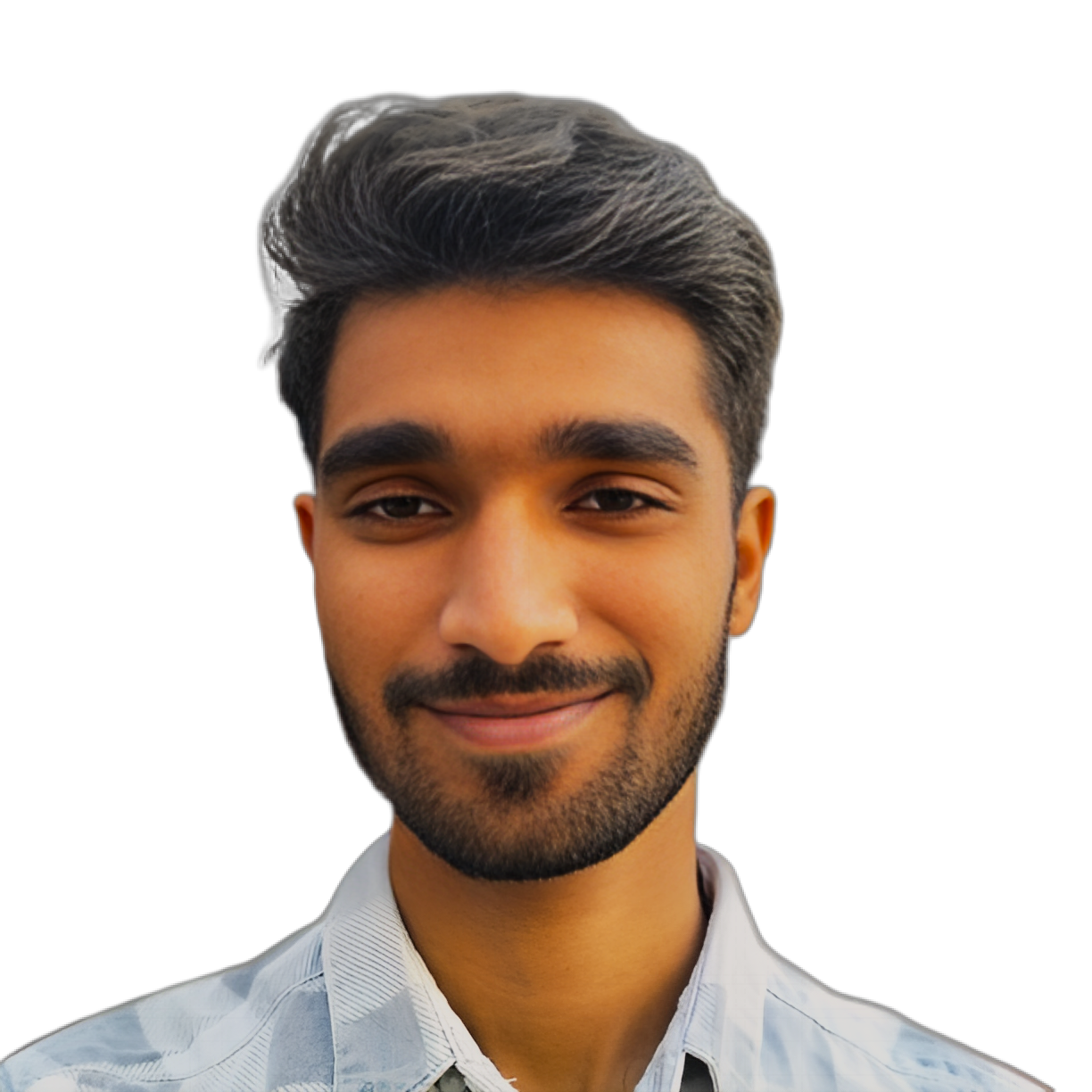
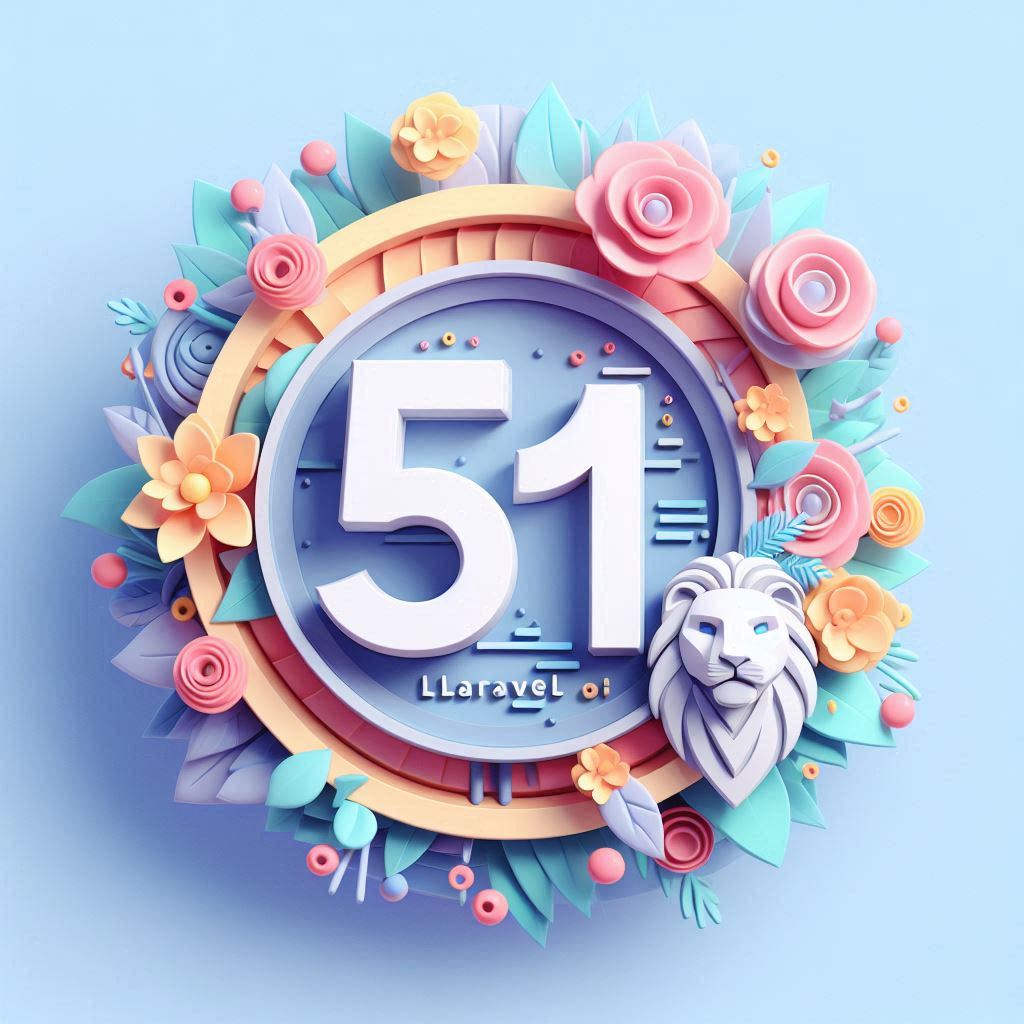
Introduction
In the fast-paced world of web development, protecting your Laravel application from malicious attacks and excessive resource usage is paramount. Here's where Laravel's built-in rate limiting feature comes to the rescue. By controlling the number of requests an application receives within a specific timeframe, you can create a robust shield against denial-of-service (DoS) attacks, prevent abusive user behavior, and ensure a fair and smooth experience for all legitimate users.
Why Use Rate Limiting?
The benefits of implementing rate limiting in your Laravel application are numerous:
Thwart DoS Attacks: Malicious actors often attempt to overwhelm your server with a barrage of requests, aiming to take it offline. Rate limiting acts as a gatekeeper, mitigating such attacks by setting a threshold on the number of requests allowed per user or IP address within a given time frame.
Curb Abusive Users: If a user is making excessive requests that could potentially slow down your application (e.g., repeatedly attempting to log in or exploit functionalities), rate limiting can automatically block them, safeguarding your system's integrity.
Guarantee Fair Access: By regulating the request flow, you create a level playing field for all users. No single user can monopolize resources, ensuring a seamless experience for everyone.
Optimize Resource Allocation: Rate limiting helps prevent spikes in requests that could overload your server. This optimizes resource usage, allowing your application to function efficiently for all legitimate users.
Understanding Laravel's Rate Limiting Mechanism
Laravel's rate limiting functionality leverages your application's cache to store data pertaining to user requests. You have the power to define custom rules for various actions within your app. These rules specify the maximum number of requests a user can make for a particular action within a defined time window. For example, you might choose to allow 10 login attempts per minute.
Step-by-Step Tutorial: Implementing Rate Limiting in Laravel
Configuration (Optional):
While Laravel's rate limiting is enabled by default, you can fine-tune it to your application's specific needs. To access the configuration options, head over to the
config/rate-limiting.php
file. Here, you can customize settings like the cache driver (cache.store
), the default throttle expiration time (default
), and more.Defining Rate Limit Rules:
The core of rate limiting lies in establishing rate limit rules for the actions you want to safeguard. Laravel provides two primary methods to achieve this:
Using the
RateLimiter
Facade:use Illuminate\Support\Facades\RateLimiter; Route::post('/login', function () { RateLimiter::attempt('login', 5, 60); // Allow 5 login attempts per minute // Login logic here });
In this example, the
RateLimiter::attempt
method defines a rate limit rule for the login action. It allows a maximum of 5 login attempts within a 60-minute (1 hour) window.Using Rate Limit Gates:
Route::post('/login', function () { if (RateLimiter::tooManyAttempts('login', 5)) { return back()->withErrors(['too_many_attempts' => 'Too many login attempts. Please try again later.']); } // Login logic here });
This approach utilizes the
RateLimiter::tooManyAttempts
method to check if the user has exceeded the rate limit for the login action. If so, it redirects the user back to the login page with an appropriate error message.
Customization: Tailoring Rate Limiting Rules
Laravel offers a plethora of options to tailor your rate limiting rules for different use cases:
Granular Control: You can define rate limits based on various factors, such as user ID, IP address, email address, or a combination of these.
Customizing Expirations: The default expiration time for rate limits is one minute. However, you can adjust this value to suit your application's requirements. For instance, you might set a shorter expiration for login attempts and a longer one for API calls.
Customizing Response Messages: Laravel provides default error messages when a user exceeds a rate limit. You can customize these messages to provide more informative feedback to your users.
Best Practices for Effective Rate Limiting
Here are some key considerations to ensure optimal implementation of rate limiting:
Identify Critical Actions: Prioritize the actions in your application that are most susceptible to abuse or overuse. These might include login attempts, API calls, form submissions, or any actions that could potentially impact your server resources.
Set Appropriate Limits: Carefully consider the limits you set for each action. Too low a limit can frustrate legitimate users, while too high a limit might leave your application vulnerable. Strike a balance between security and user experience.
Monitor and Fine-Tune: As your application evolves and usage patterns change, keep an eye on your rate limiting rules. Adjust them as needed to maintain a balance between security and user experience.
Provide Clear Feedback: Inform users when they've exceeded a rate limit. Offer clear and informative error messages that explain why their request was blocked and when they can try again. Consider offering different feedback for different actions (e.g., a more lenient message for login attempts compared to API access).
Consider Exemptions: In rare cases, you might consider exempting certain users or IP addresses from rate limiting. For instance, you could create an exception list for trusted administrative users or specific partner applications. However, exercise caution when implementing exemptions to avoid compromising the security of your application.
Conclusion
Laravel's rate limiting feature empowers you to safeguard your application from malicious attacks, prevent resource depletion, and guarantee fair access for all legitimate users. By understanding the fundamentals, following the step-by-step tutorial, and adhering to best practices, you can effectively implement rate limiting and protect your Laravel application. Remember, security is an ongoing process, so stay vigilant and adapt your strategies as needed to maintain a robust and secure application.
Bonus: Additional Considerations
Integration with Third-Party Services: If you're using external services or APIs within your Laravel application, consider their rate limiting policies. You might need to adjust your own rate limits accordingly to avoid exceeding theirs.
Advanced Rate Limiting with Packages: The Laravel ecosystem offers various third-party packages that provide additional functionalities and granular control over rate limiting. Explore these options if your application requires more sophisticated rate limiting strategies.
By following these guidelines and tailoring the approach to your specific application's needs, you can leverage Laravel's rate limiting to create a secure and efficient web development environment.
Subscribe to my newsletter
Read articles from Asis Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
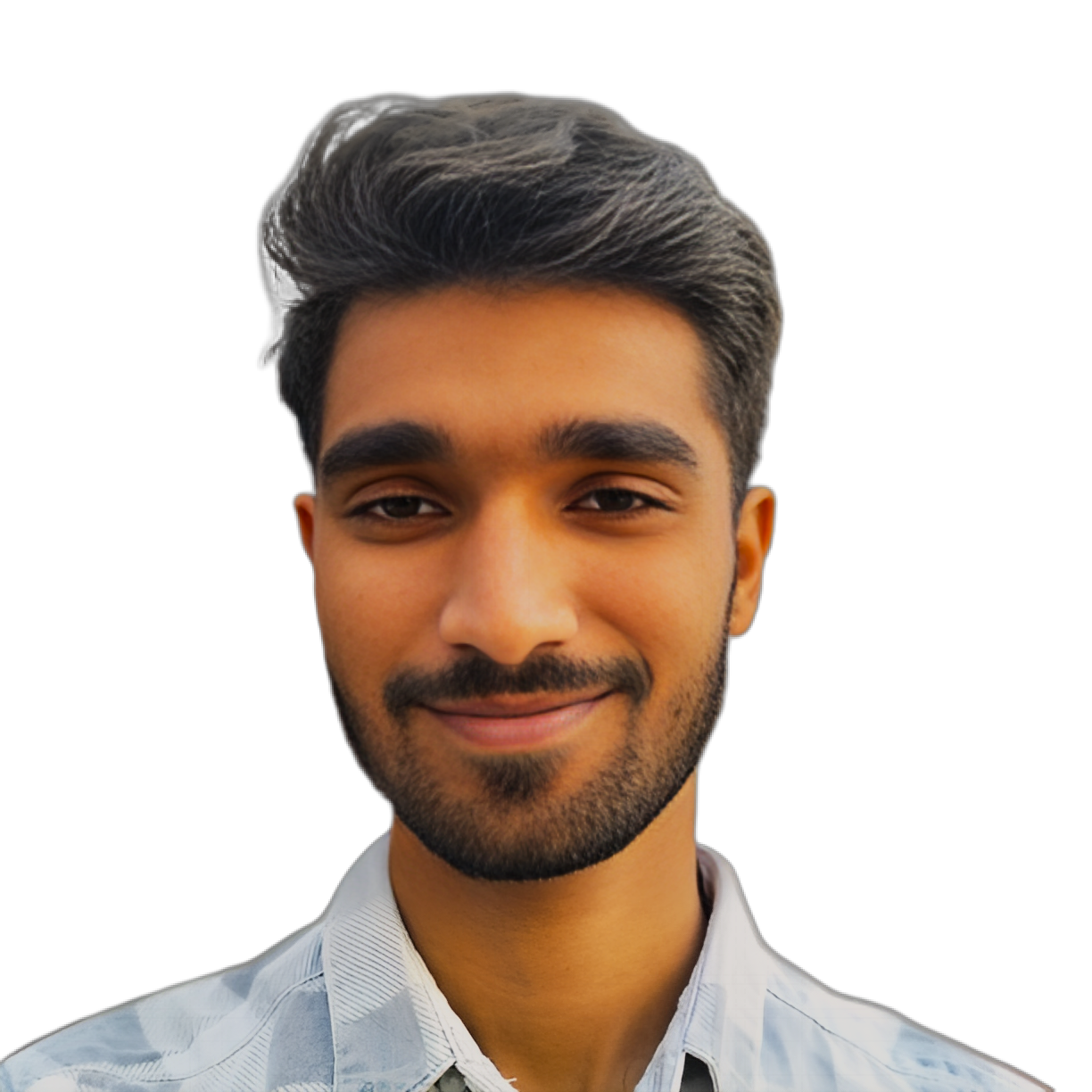
Asis Sharma
Asis Sharma
I'm an ambitious and motivated software developer, eager to apply my knowledge and skills to contribute to the development of innovative and cutting-edge software solutions. I'm well-versed in a variety of programming languages, including Javascript, C++, and Node.js, and have a understanding of object-oriented programming and algorithms. I'm passionate about developing and I am eager to learn and collaborate with others.