SceneKit - iOS Apps with 3D Content
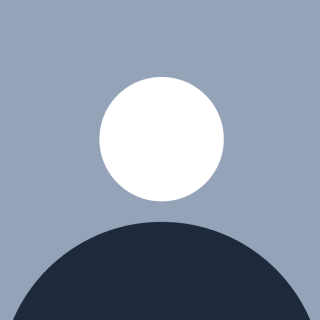
Table of contents
- Why SceneKit?
- Getting Started: Setting Up SceneKit
- The Scene Graph: Organizing Your 3D World π
- Lighting Up Your Scene π‘
- Action! Animating Your Objects π¬
- Materials and Textures: Dressing Up Your Geometries
- Integrating Physics: Making Things Fall (or Fly) πͺοΈ
- Getting Real with ARKit πΆοΈ
- Resources to Keep You Going π
- Conclusion π

Ever wanted to add a splash of 3D magic to your iOS app? Enter SceneKit, your friendly neighborhood framework for creating rich 3D content. Whether you're a seasoned developer or just starting, SceneKit makes it easy to dive into the world of 3D graphics. So, grab your favorite caffeinated beverage and let's explore how you can create stunning 3D experiences with SceneKit! π
Why SceneKit?
Before we jump into code, letβs talk about why SceneKit is awesome:
High-Level Framework: SceneKit is built on top of OpenGL and Metal, so you get all the power without the headache. πͺ
Easy to Use: With its intuitive API, even newbies can get started quickly. π
Cross-Platform: Supports iOS, macOS, watchOS, and tvOS. π±π»πΉοΈ
ARKit Integration: Perfect for creating augmented reality apps. πΆοΈ
Getting Started: Setting Up SceneKit
Alright, let's set up a SceneKit project in Xcode. No wizards or magical incantations required. π§ββοΈβ¨
Create a New Xcode Project:
Open Xcode and create a new project.
Choose the "Single View App" template.
Add SceneKit framework to your project by navigating to your project settings, selecting your target, and then going to the "General" tab. Under "Frameworks, Libraries, and Embedded Content", click the "+" button and add SceneKit.
Add a SCNView:
- In your storyboard or SwiftUI view, replace the default view with
SCNView
.
- In your storyboard or SwiftUI view, replace the default view with
Here's a simple setup in SwiftUI:
import SwiftUI
import SceneKit
struct ContentView: View {
var body: some View {
SceneView(
scene: createScene(),
options: [.allowsCameraControl, .autoenablesDefaultLighting]
)
.frame(width: 300, height: 300)
}
func createScene() -> SCNScene {
let scene = SCNScene()
// Camera setup
let cameraNode = SCNNode()
cameraNode.camera = SCNCamera()
cameraNode.position = SCNVector3(x: 0, y: 0, z: 10)
scene.rootNode.addChildNode(cameraNode)
// Light setup
let lightNode = SCNNode()
lightNode.light = SCNLight()
lightNode.light?.type = .omni
lightNode.position = SCNVector3(x: 0, y: 10, z: 10)
scene.rootNode.addChildNode(lightNode)
// Geometry setup
let box = SCNBox(width: 1, height: 1, length: 1, chamferRadius: 0)
let boxNode = SCNNode(geometry: box)
scene.rootNode.addChildNode(boxNode)
return scene
}
}
The Scene Graph: Organizing Your 3D World π
Imagine you're a director setting up a stage for a play. Each object on your stage (actors, props, lights) is a SCNNode
. These nodes can have child nodes, creating a hierarchical tree structure. Hereβs a quick rundown:
SCNScene: Your stage. π
SCNNode: The actors and props. π©βπ€π¨βπ€
SCNGeometry: The shapes (e.g., boxes, spheres). π¦β½
SCNMaterial: The costumes (textures and colors). ππΆοΈ
SCNLight: The stage lighting. π‘
SCNCamera: The audience's viewpoint. πΈ
Lighting Up Your Scene π‘
SceneKit supports various light types to make your scene look realistic (or spooky, if that's your thing). Hereβs how to add a simple light:
let lightNode = SCNNode()
lightNode.light = SCNLight()
lightNode.light?.type = .omni // Think of it as a bare light bulb
lightNode.position = SCNVector3(x: 0, y: 10, z: 10)
scene.rootNode.addChildNode(lightNode)
Action! Animating Your Objects π¬
Animations in SceneKit are as easy as pie. Want to make a box bounce up and down? Use SCNAction
:
let moveUp = SCNAction.moveBy(x: 0, y: 2, z: 0, duration: 1)
let moveDown = SCNAction.moveBy(x: 0, y: -2, z: 0, duration: 1)
let sequence = SCNAction.sequence([moveUp, moveDown])
boxNode.runAction(SCNAction.repeatForever(sequence))
Now you have a bouncing box. Imagine the possibilities: bouncing balls, flying saucers, or even a dancing banana! ππ
Materials and Textures: Dressing Up Your Geometries
Geometries are cool, but without materials, they're just plain shapes. Letβs add some flair:
let material = SCNMaterial()
material.diffuse.contents = UIImage(named: "texture.png")
box.materials = [material]
Now your box isnβt just a boxβitβs a fancy box with a texture! π
Integrating Physics: Making Things Fall (or Fly) πͺοΈ
Ever wanted to create a realistic game where objects fall, bounce, or crash into each other? SceneKitβs physics engine has got you covered:
boxNode.physicsBody = SCNPhysicsBody(type: .dynamic, shape: nil)
boxNode.physicsBody?.mass = 1.0
boxNode.physicsBody?.friction = 0.5
Now your box will react to gravity and other physical forces. Drop the micβer, box! π€π¦
Getting Real with ARKit πΆοΈ
Integrate SceneKit with ARKit for augmented reality experiences that will blow your mind (and your users' minds too):
import ARKit
// Create an ARSCNView
let arView = ARSCNView(frame: .zero)
arView.scene = scene
// Configure AR session
let configuration = ARWorldTrackingConfiguration()
arView.session.run(configuration)
Now, your 3D content can interact with the real world. Cue the βoohsβ and βaahsβ. π²π
Resources to Keep You Going π
- Official Documentation: Apple Developer Documentation - SceneKit
Conclusion π
SceneKit is your gateway to creating amazing 3D experiences in your iOS apps. From simple animations to complex augmented reality, itβs all possible with a bit of creativity and some SceneKit magic. So, what are you waiting for? Start creating and may your 3D worlds be ever immersive and mind-blowing!
Happy coding! π
Image in blog cover by Patrick Schneider on Unsplash
Subscribe to my newsletter
Read articles from Himanshu Goyal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Himanshu Goyal
Himanshu Goyal
Software Engineer | Mobile App Developer | iOS