Introduction to Next.js: The Ultimate React Framework for Production
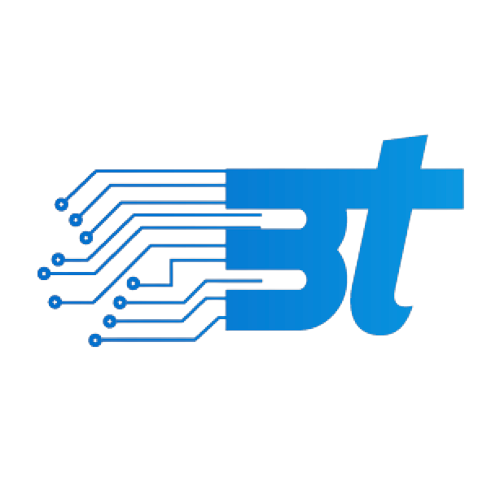
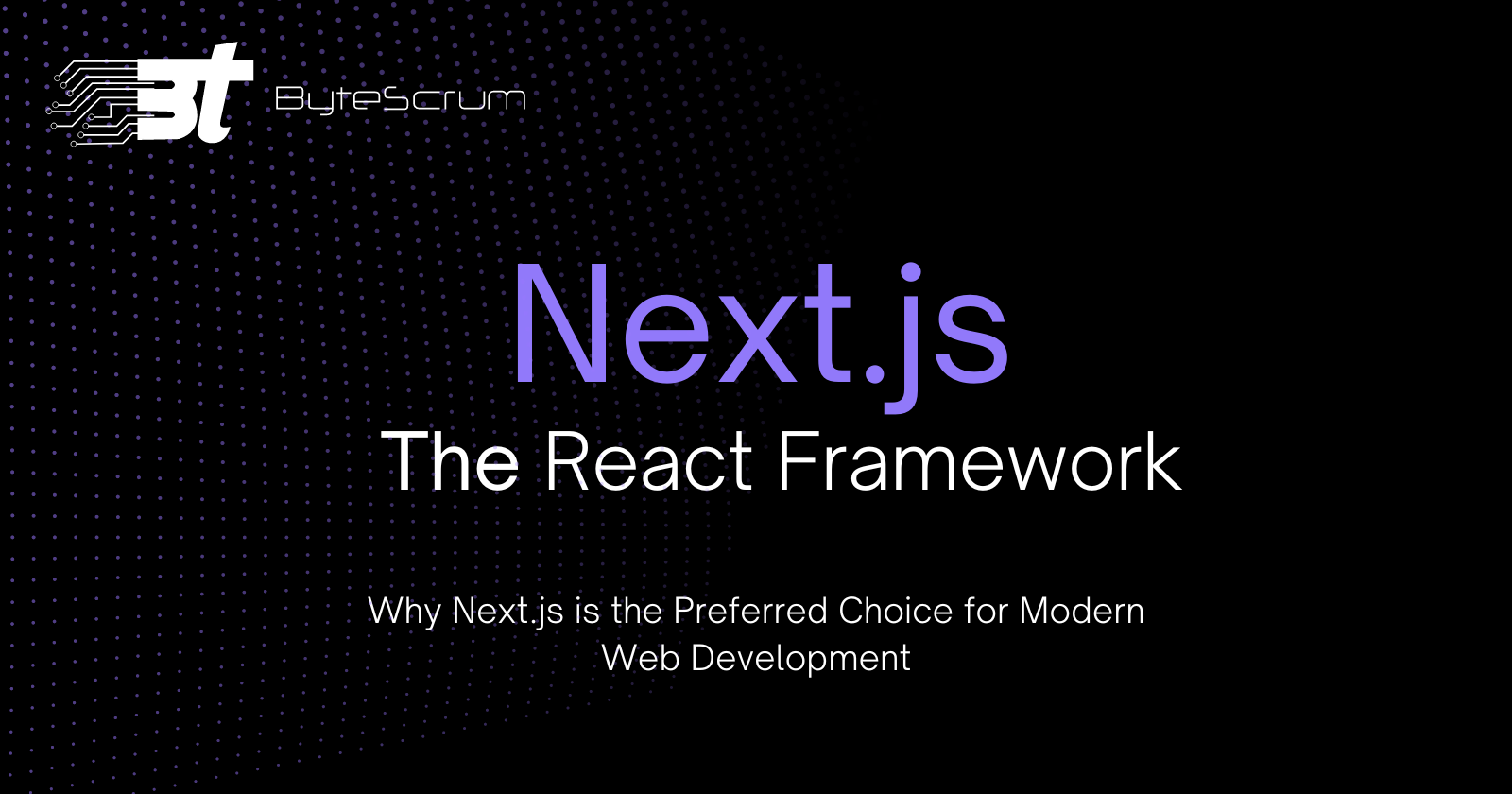
In the ever-evolving landscape of web development, frameworks and libraries play a crucial role in simplifying the development process and enhancing performance. React, a popular JavaScript library for building user interfaces, has paved the way for a multitude of frameworks that extend its capabilities. Among these, Next.js has emerged as a standout choice for developers looking to create production-ready React applications. In this blog, we'll explore what Next.js is, its key features, and why it's considered the ultimate React framework for production.
What is Next.js?
Next.js is an open-source React framework created by Vercel that enables developers to build server-side rendered (SSR) and statically generated web applications with ease. It provides a robust set of features out of the box, such as file-based routing, automatic code splitting, and built-in support for API routes, making it a powerful tool for modern web development.
Key Features of Next.js
Server-Side Rendering (SSR) and Static Site Generation (SSG):
- Next.js supports both SSR and SSG, allowing developers to choose the best rendering method for their application. SSR improves SEO and performance by rendering pages on the server, while SSG generates static HTML at build time for fast load times.
File-Based Routing:
- Next.js uses a file-based routing system where each file in the
pages
directory automatically becomes a route in the application. This simplifies navigation and routing without the need for additional configuration.
- Next.js uses a file-based routing system where each file in the
API Routes:
- Next.js allows you to create API endpoints within your application, making it easy to build full-stack applications with serverless functions. Simply create a file in the
pages/api
directory, and it becomes an endpoint.
- Next.js allows you to create API endpoints within your application, making it easy to build full-stack applications with serverless functions. Simply create a file in the
Automatic Code Splitting:
- Next.js automatically splits your code into smaller bundles, ensuring that only the necessary code is loaded for each page. This improves the performance and speed of your application.
Static Export:
- Next.js can export your application as a static site, which can be deployed to any static hosting service. This combines the benefits of a static site with the dynamic capabilities of React.
Built-In CSS and Sass Support:
- Next.js includes built-in support for CSS and Sass, allowing you to import styles directly into your components. It also supports CSS modules for scoped styling.
Image Optimization:
- Next.js provides an optimized Image component that automatically handles image resizing, lazy loading, and serving images in the most appropriate format, improving the performance of your application.
TypeScript Support:
- Next.js has excellent TypeScript support out of the box, enabling developers to build type-safe applications with minimal configuration.
Getting Started with Next.js
To get started with Next.js, you'll need to have Node.js and npm (or Yarn) installed on your machine. You can create a new Next.js application using the following commands:
npx create-next-app my-next-app
cd my-next-app
npm run dev
This will set up a new Next.js project and start the development server. You can access your application at http://localhost:3000
.
Creating Your First Page
Creating a new page in Next.js is as simple as adding a file to the pages
directory. For example, let's create a new about.js
page:
// pages/about.js
const About = () => {
return (
<div>
<h1>About Us</h1>
<p>This is the about page.</p>
</div>
);
};
export default About;
You can now navigate to http://localhost:3000/about
to see your new page.
Adding API Routes
Next.js allows you to create API endpoints with ease. Let's add a simple API route that returns a list of users:
// pages/api/users.js
export default function handler(req, res) {
res.status(200).json([
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Doe' },
]);
}
You can access this API at http://localhost:3000/api/users
.
Deploying Your Next.js Application
Next.js applications can be deployed to a variety of hosting providers, including Vercel, the creators of Next.js. Vercel offers seamless integration with Next.js and provides features like automatic builds, deployments, and custom domains.
To deploy your application to Vercel, you can use the Vercel CLI:
npm install -g vercel
vercel
Follow the prompts to deploy your application. Once deployed, Vercel will provide you with a live URL for your application.
Conclusion
Feel free to explore Next.js's extensive documentation to discover more features and best practices. Happy coding!
Check out the website we built in Next.js (Merlin Hunter).
Thank for reading the blog!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
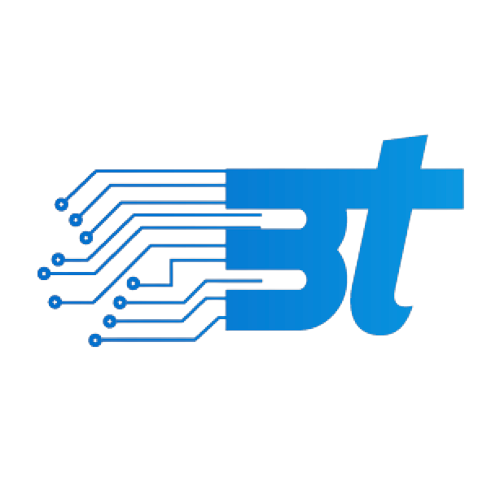
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.