Laravel Queues: Background Jobs and Workers
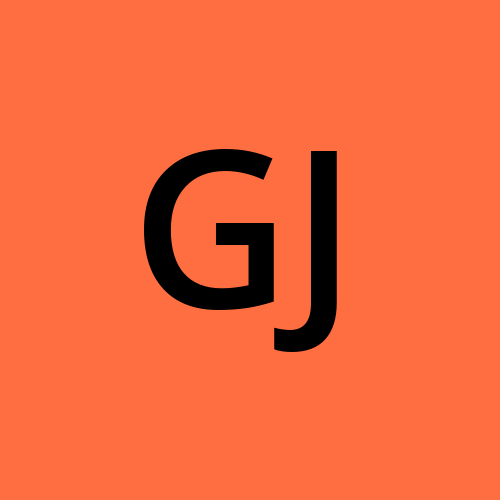
Laravel, a powerful and elegant PHP framework, provides robust tools for managing background jobs and task queues. This is essential for building scalable and efficient web applications. In this article, we'll explore the fundamentals of Laravel queues, how to set up and manage background jobs, and the role of workers in this process.
Introduction to Queues
Queues allow you to defer the processing of a time-consuming task, such as sending an email or processing a video, to a later time. This offloading helps in improving the performance of your application by freeing up resources for more immediate tasks. Laravel supports several queue backends, including:
Database
Redis
Beanstalkd
Amazon SQS
Redis
Setting Up Queues in Laravel
To get started with queues, you first need to configure your queue connection. Laravel provides a configuration file located at config/queue.php
. By default, the configuration is set to use the sync
driver, which executes jobs immediately.
To use a different driver, such as database
, update the default
setting in the config/queue.php
file:
'default' => env('QUEUE_CONNECTION', 'database'),
Next, you need to create a migration for the jobs table:
php artisan queue:table
php artisan migrate
This creates the necessary table to store queued jobs handled by Laravel out of the box.
Creating Jobs
Laravel makes it easy to create a new job class. You can generate a job class using the Artisan command:
php artisan make:job ProcessPodcast
This command creates a new job class in the app/Jobs
directory. Here's an example of what the generated job class might look like:
<?php
namespace App\Jobs;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Foundation\Bus\Dispatchable;
use Illuminate\Queue\InteractsWithQueue;
use Illuminate\Queue\SerializesModels;
class ProcessPodcast implements ShouldQueue
{
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
protected $podcast;
/**
* Create a new job instance.
*
* @return void
*/
public function __construct($podcast)
{
$this->podcast = $podcast;
}
/**
* Execute the job.
*
* @return void
*/
public function handle()
{
// Process the podcast...
}
}
The ShouldQueue
interface tells Laravel that this job should be pushed onto the queue instead of being executed synchronously.
Dispatching Jobs
You can dispatch jobs using the dispatch
method from anywhere in your application, such as a controller or a service class:
use App\Jobs\ProcessPodcast;
// Dispatch the job with necessary data
ProcessPodcast::dispatch($podcast);
Working with Queue Workers
Queue workers are responsible for processing the jobs in the queue. Laravel provides a simple way to start a worker using the queue:work
Artisan command:
php artisan queue:work
This command starts a long-running process that will keep checking the queue for new jobs and process them as they arrive.
To run the worker as a daemon, which is more suitable for production environments, you can use the --daemon
flag:
php artisan queue:work --daemon
Supervising Queue Workers
In a production environment, you should use a process monitor like Supervisor to ensure that your queue workers are always running. Supervisor can be installed on Ubuntu using the following command:
sudo apt-get install supervisor
After installation, you can create a configuration file for your Laravel queue worker:
[program:laravel-worker]
process_name=%(program_name)s_%(process_num)02d
command=php /path/to/your/app/artisan queue:work --sleep=3 --tries=3
autostart=true
autorestart=true
user=your-username
numprocs=8
redirect_stderr=true
stdout_logfile=/path/to/your/app/worker.log
After configuring Supervisor, you need to update the configuration and start the process:
sudo supervisorctl reread
sudo supervisorctl update
sudo supervisorctl start laravel-worker:*
Handling Failed Jobs
Laravel also provides an elegant way to handle failed jobs. You can create a migration for the failed_jobs
table using the Artisan command:
php artisan queue:failed-table
php artisan migrate
To handle failed jobs, you can define a failed
method on your job class:
public function failed(Exception $exception)
{
// Handle the failure...
}
You can also retry failed jobs using the queue:retry
command:
php artisan queue:retry all
Conclusion
Laravel queues are a powerful feature for managing background tasks in a scalable and efficient way. By offloading time-consuming processes, you can significantly improve the performance and responsiveness of your applications. With a variety of supported queue backends and robust tools for managing jobs and workers, Laravel makes it easy to integrate queues into your application.
By setting up queues, creating and dispatching jobs, and managing workers, you can take full advantage of Laravel's capabilities to build high-performance, scalable web applications.
Subscribe to my newsletter
Read articles from Godstime John directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
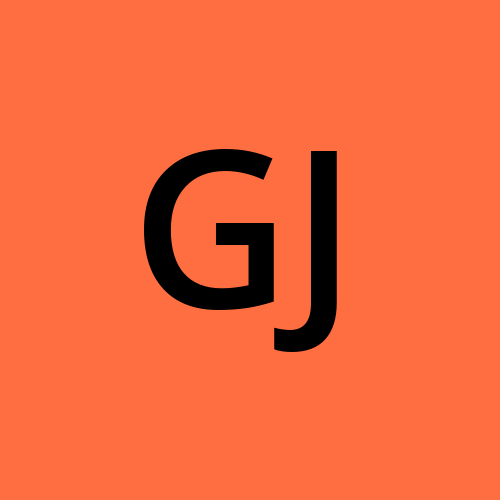