Send e-mails using NodeJS
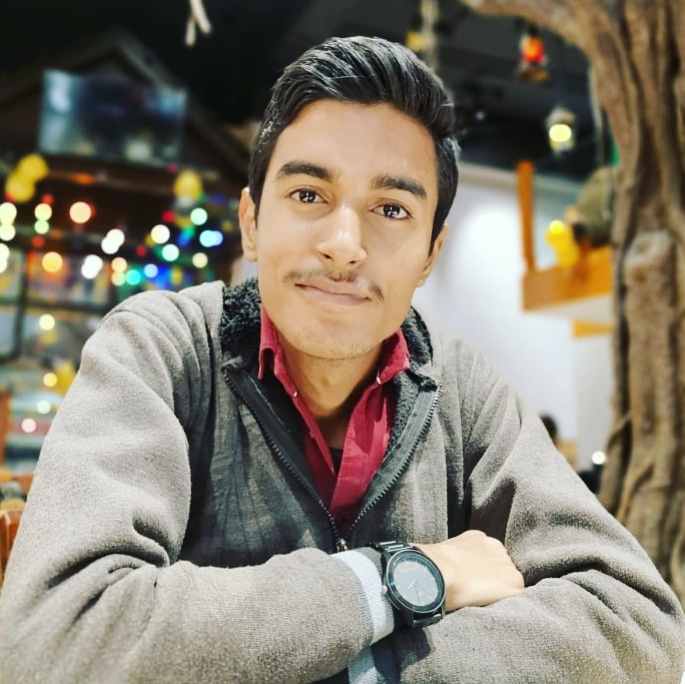
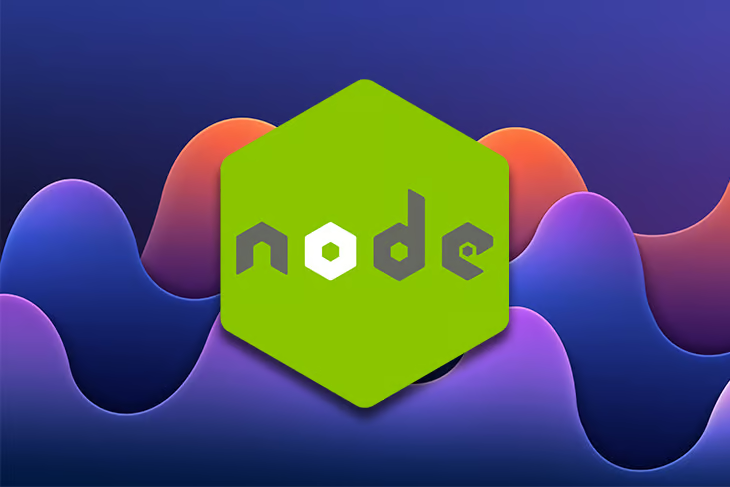
In the world of MERN stack development (MongoDB, Express, React, Node.js), building dynamic and interactive web applications is a breeze. But what if your application needs to send emails to users? NodeJS comes to the rescue with powerful libraries like Nodemailer that streamline the email sending process.
This blog post will guide you through setting up Nodemailer and crafting code to send emails from your NodeJS application.
Setting Up Nodemailer
The first step is to install the Nodemailer package using npm (Node Package Manager):
npm install nodemailer
This command will download the Nodemailer library and make it available for use in your project.
Creating a Transporter Object
Nodemailer utilizes a transporter object to handle communication with your email server. This object requires configuration details like the SMTP server address, port number, and authentication credentials.
Here's an example of creating a transporter object for Gmail:
const nodemailer = require('nodemailer');
const transporter = nodemailer.createTransport({
service: 'gmail',
auth: {
user: 'your_email@gmail.com',
pass: 'your_password'
}
});
Important Note: Avoid storing your email password directly in your code. A more secure approach is to use environment variables or a configuration file to manage sensitive information.
Crafting Your Email Message
The transporter.sendMail
method takes a mailOptions
object that defines the content of your email. This object allows you to specify details like sender address, recipient address, subject line, and email body.
Here's an example of crafting a basic email message:
const mailOptions = {
from: 'your_email@gmail.com',
to: 'recipient_email@example.com',
subject: 'Sending Email with NodeJS!',
text: 'This is a test email sent from a NodeJS application.'
};
The text
property defines the message content in plain text format. You can also use the html
property to create emails with HTML formatting.
Sending the Email
Once you have configured the transporter and defined your email message, you can use the transporter.sendMail
method to send the email:
transporter.sendMail(mailOptions, (error, info) => {
if (error) {
console.error(error);
} else {
console.log('Email sent: ' + info.response);
}
});
This code snippet sends the email defined in mailOptions
and logs the response to the console. The response will contain information about the success or failure of the email delivery.
Conclusion
By following these steps and incorporating Nodemailer into your NodeJS application, you can easily send emails to users and enhance the functionality of your MERN stack projects. Remember to explore Nodemailer's additional features to customize your emails further, such as adding attachments or sending emails with BCC (blind carbon copy) recipients.
Happy Coding!
Subscribe to my newsletter
Read articles from Abhishek Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
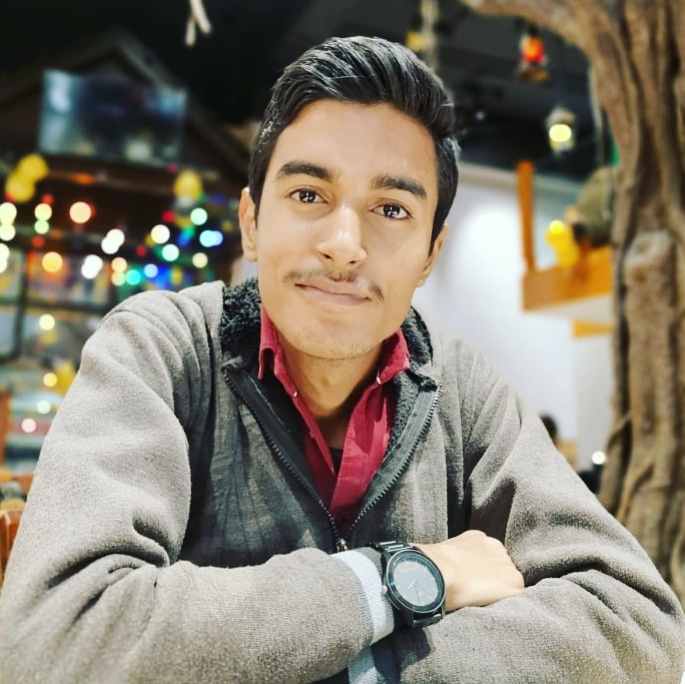
Abhishek Sharma
Abhishek Sharma
Abhishek is a designer, developer, and gaming enthusiast! He love creating things, whether it's building websites, designing interfaces, or conquering virtual worlds. With a passion for technology and its impact on the future, He is curious about how AI can be used to make work better and play even more fun.